ESP32 - Web Server Password
In this tutorial, we'll find out how to make ESP32 web server that is protected by username password for login. Before accessing web page on ESp32, user will be asked to input username/password
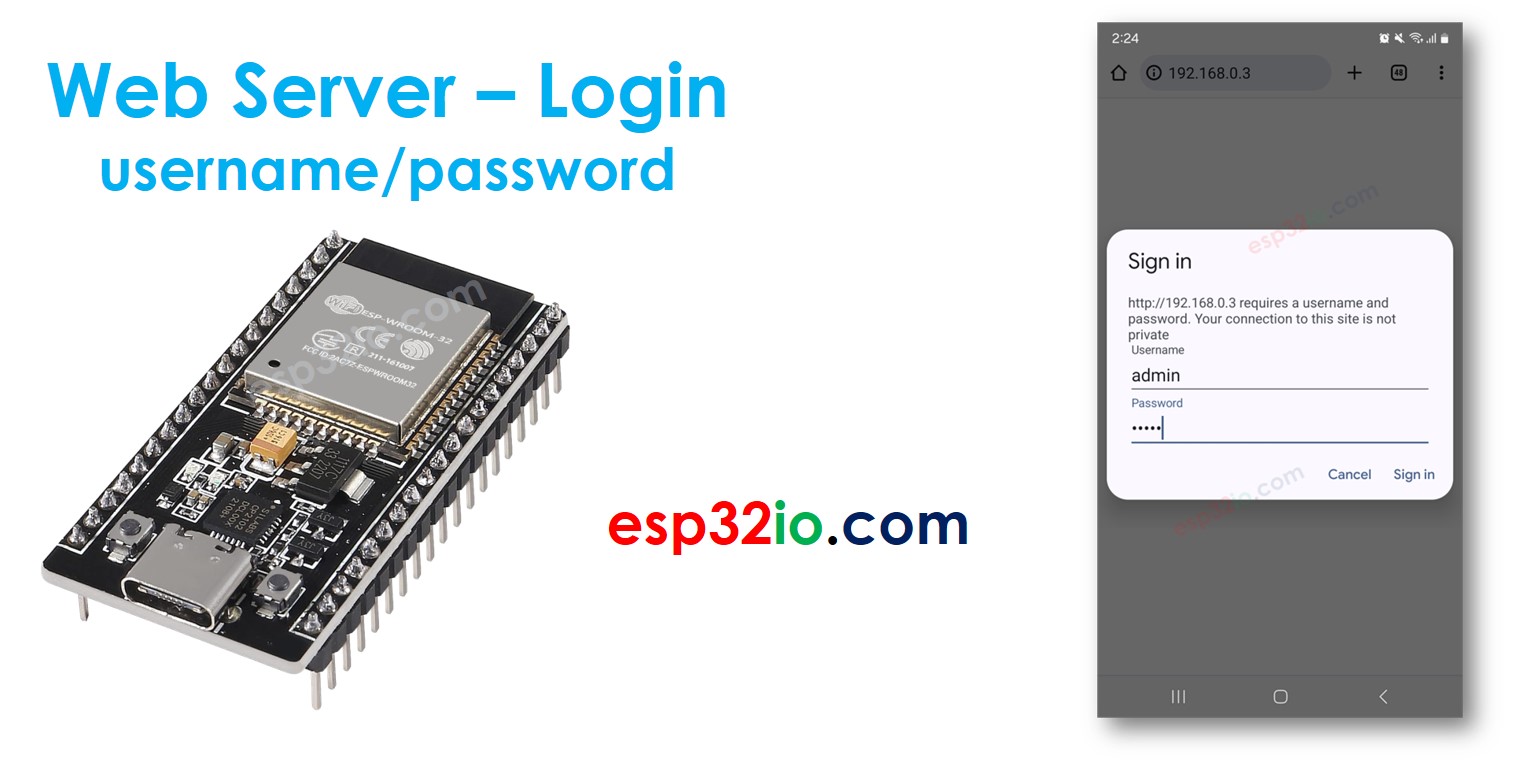
Hardware Used In This Tutorial
1 | × | ESP-WROOM-32 Dev Module | |
1 | × | USB Cable Type-C | |
1 | × | (Recommended) ESP32 Screw Terminal Adapter |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: some of these links are affiliate links. We may earn a commission on your purchase at no extra cost to you. We appreciate it.
Introduction to ESP32 and Web Server
If you're not familiar with ESP32 and Web Server (including pinout, how it works, and programming), you can learn about them through the following tutorials:
ESP32 Code - Web server Username/Password
/*
* This ESP32 code is created by esp32io.com
*
* This ESP32 code is released in the public domain
*
* For more detail (instruction and wiring diagram), visit https://esp32io.com/tutorials/esp32-web-server-password
*/
#include <WiFi.h>
#include <ESPAsyncWebServer.h>
const char* ssid = "YOUR_WIFI_SSID"; // WiFi SSID, change it to your network
const char* password = "YOUR_WIFI_PASSWORD"; // WiFi password, change it to your network
AsyncWebServer server(80);
const char* www_username = "admin"; // web page username
const char* www_password = "esp32"; // web page password
void handleRoot(AsyncWebServerRequest* request) {
if (!request->authenticate(www_username, www_password)) {
return request->requestAuthentication();
}
// The below is simplest form of web page, you can modify this
request->send(200, "text/html", "<html><body><h1>Login Successful!</h1><p>You are now logged in.</p></body></html>");
}
void handleNotFound(AsyncWebServerRequest* request) {
request->send(404, "text/plain", "Not found");
}
void setup() {
Serial.begin(9600);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
server.on("/", HTTP_GET, handleRoot);
server.onNotFound(handleNotFound);
server.begin();
// Print the ESP32's IP address
Serial.print("ESP32 Web Server's IP address: ");
Serial.println(WiFi.localIP());
}
void loop() {
}
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Open the Library Manager by clicking on the Library Manager icon on the left navigation bar of Arduino IDE.
- Search “ESPAsyncWebServer”, then find the ESPAsyncWebServer created by lacamera.
- Click Install button to install ESPAsyncWebServer library.
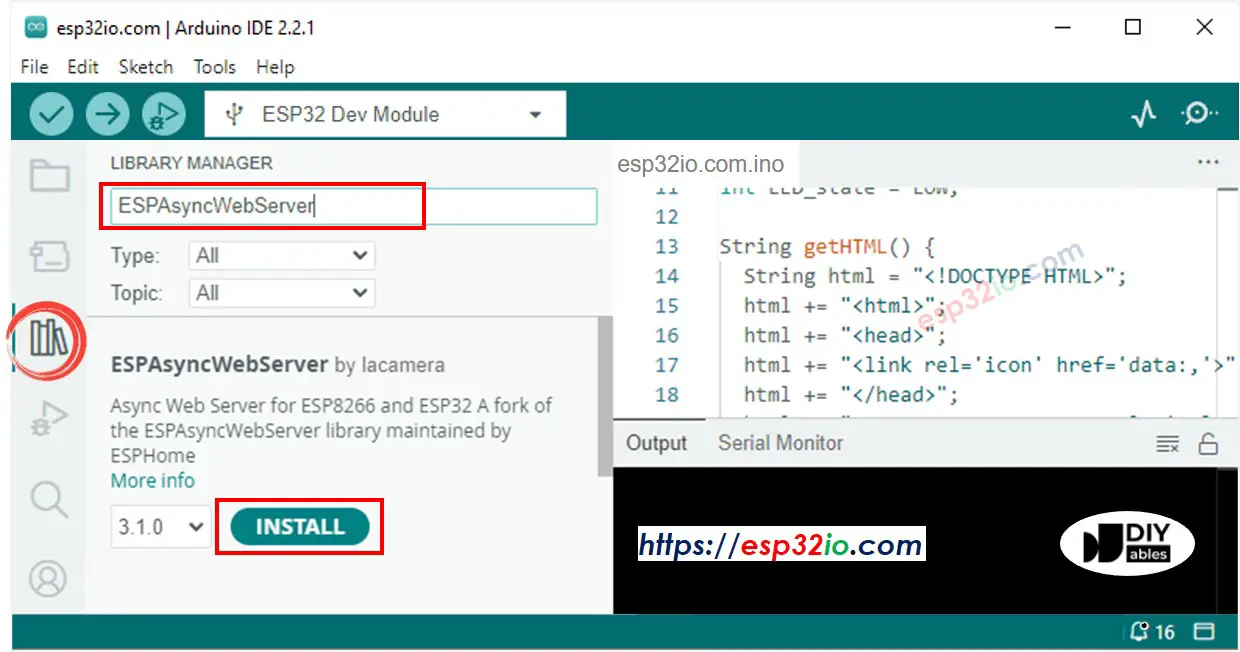
- You will be asked to install the dependency. Click Install All button.
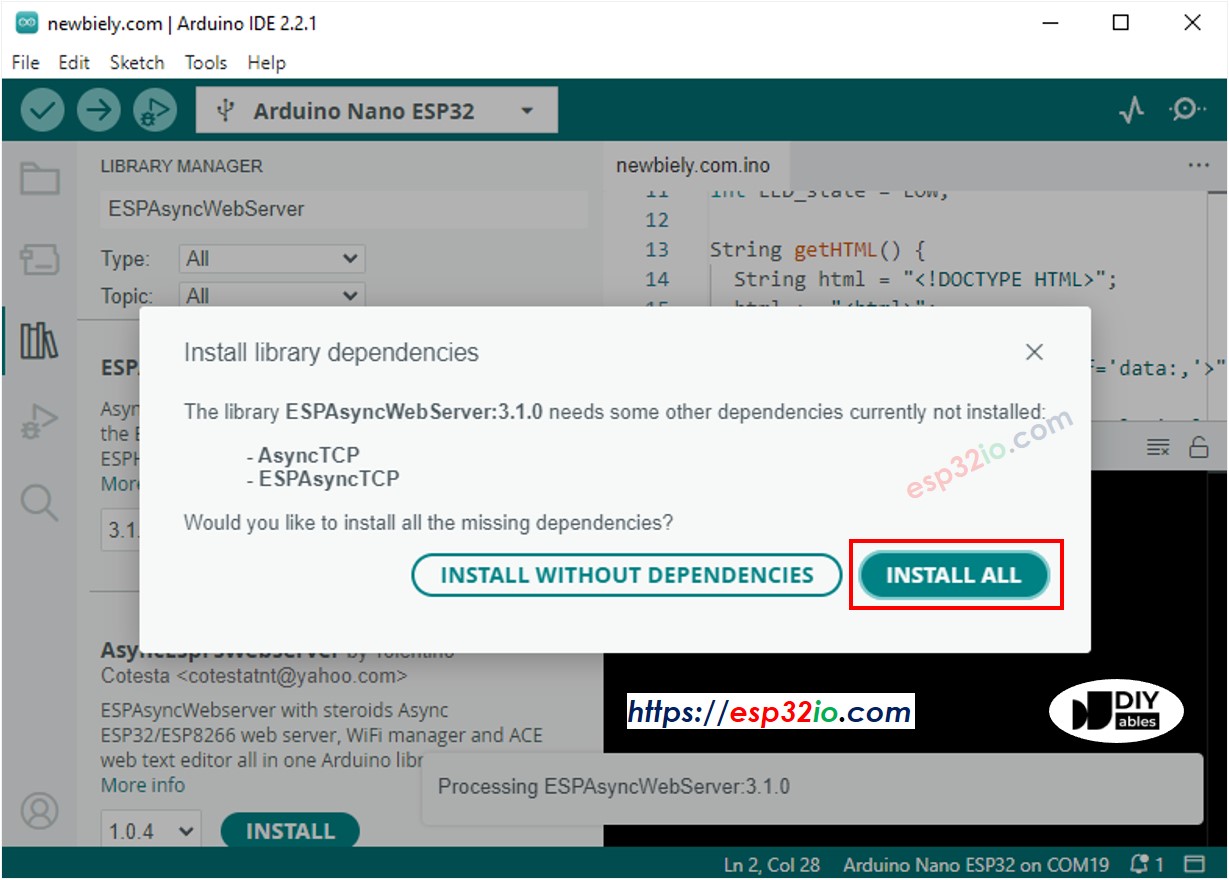
- Copy the above code and open with Arduino IDE
- Change the wifi information (SSID and password) in the code to yours
- Click Upload button on Arduino IDE to upload code to ESP32
- Open the Serial Monitor
- Check out the result on Serial Monitor.
COM6
Connecting to WiFi...
Connected to WiFi
ESP32 Web Server's IP address: 192.168.0.3
Autoscroll
Clear output
9600 baud
Newline
- You will see an IP address on the Serial Monitor, for example: 192.168.0.3
- Type the IP address on the address bar of a web browser on your smartphone or PC.
- Please note that you need to change the 192.168.0.3 to the IP address you got on Serial Monitor.
- You will see a page that prompt you to input username/password.
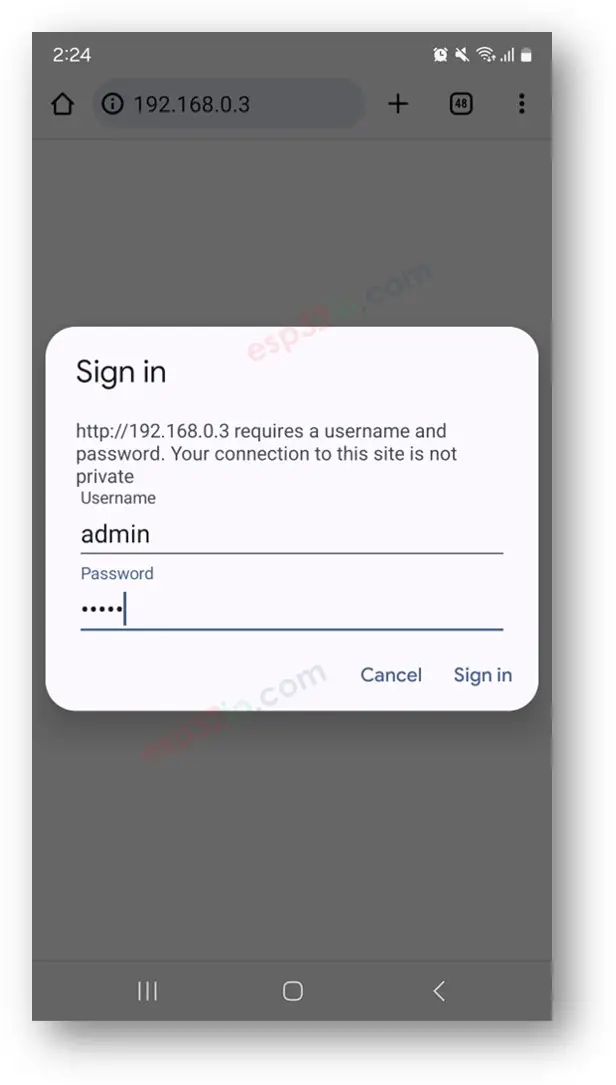
- Type username/password that are in the ESP32 code, in this case: admin as username, esp32 as password
- If you input the username/password correctly, the web content of ESP32 will be shown:
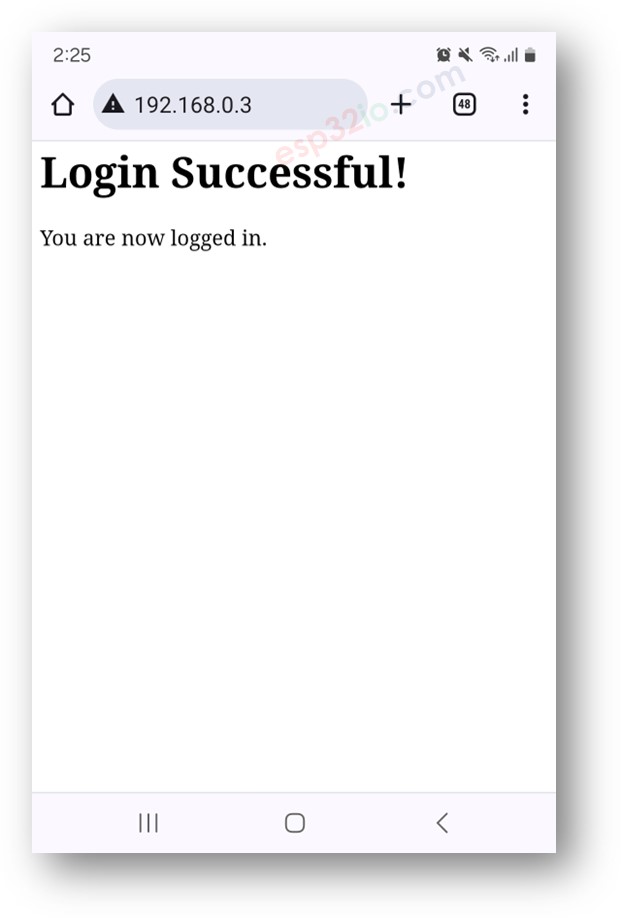
※ NOTE THAT:
- You can change web username/password in the code by changing the values of two variables: www_username and www_password
- You can modify this code to add the HTML/CSS/JavaScript code of your webpage
- In the code, there is no HTML code for the login form (username/password). Do not be suprised! The login form is created by Web Browser.
Advanced Knowledge
This section presents advanced knowledge on how the username/password system works without HTML for the login form.
- Initially, when you enter the IP address of the ESP32 into a web browser, the browser sends an HTTP request to the ESP32 without any username/password credentials.
- Upon receiving this request, the ESP32 code checks whether the username/password is included or not. If absent, the ESP32 doesn't respond the request for the page's content. Instead, it responds with an HTTP message containing headers instructing the browser to prompt the user for their username/password. Notably, this response doesn't contain HTML code for the login form.
- Upon receiving this response, the web browser parses the HTTP headers, recognizing the ESP32's request for username/password. Subsequently, the browser dynamically generates a login form allowing the user to input their credentials.
- The user then inputs their username/password into the form.
- The web browser includes the entered username/password within an HTTP request and sends it to the ESP32.
- The ESP32 verifies the username/password included in the HTTP request. If correct, it returns the requested page's content. If incorrect, it repeats the process, prompting the user to input the correct credentials again.
- Once the user inputs the correct username/password for the first time, subsequent request don't need to input username/password. This is because the web browser automatically saves the credentials, including them in subsequent requests.