ESP32 - LED Matrix via Web
In this tutorial, we will learn how to control LED matrix signboard via web interface using ESP32. In detail, we will program ESP32 to become a web server that does the following:
Returns a web interface to users when receiving a request from a web browser.
Provides the web interface that users can send the message to ESP32
Displays the messages on the LED matrix once receiving the message.
Or you can buy the following sensor kits:
Disclosure: some of these links are affiliate links. We may earn a commission on your purchase at no extra cost to you. We appreciate it.
We can use a pure HTTP to send a text from web interface to ESP32. However, using WebSocket makes it looks more responsive and does not add much difficuity, So in this tutorial, we will use the WebSocket.
Unfamiliar with LED Matrix and Web Server and WebSocket, including their pinouts, functionality, and programming? Explore comprehensive tutorials on these topics below:
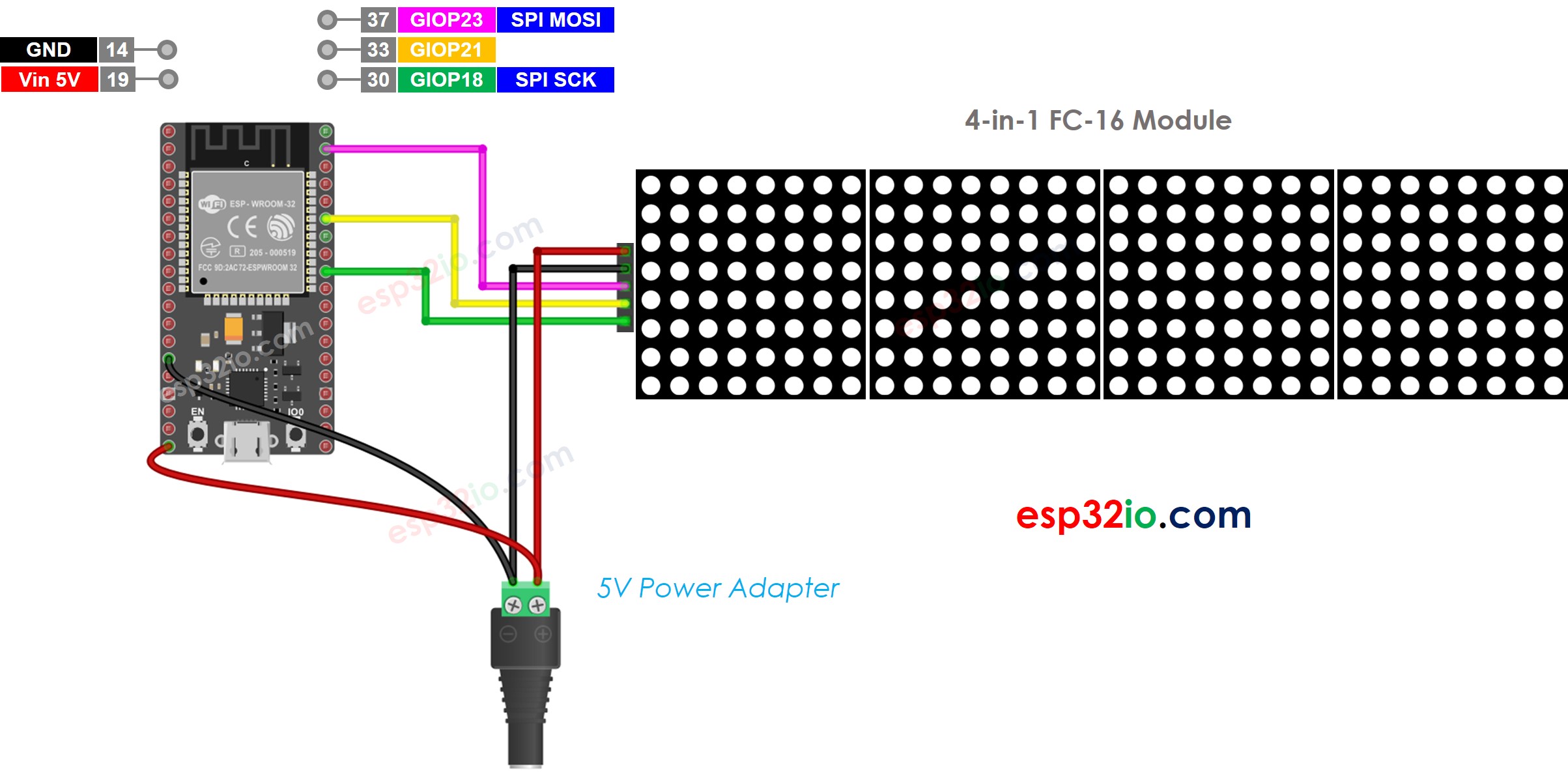
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: How to Power ESP32.
The webpage's content (HTML, CSS, JavaScript) are stored separately on an index.h file. So, we will have two code files on Arduino IDE:
An .ino file that is ESP32 code, which creates a web sever and WebSocket server
An .h file, which contains the webpage's content.
Connect the ESP32 board to your PC via a micro USB caweb server
Open Arduino IDE on your PC.
Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
Open the Library Manager by clicking on the Library Manager icon on the left navigation bar of Arduino IDE.
Search “ESPAsyncWebServer”, then find the ESPAsyncWebServer created by lacamera.
Click Install button to install ESPAsyncWebServer library.
Search “WebSockets”, then find the WebSockets created by Markus Sattler.
Click Install button to install WebSockets library.
On Arduino IDE, create new sketch, Give it a name, for example, esp32io.com.ino
Copy the below code and open with Arduino IDE
#include <WiFi.h>
#include <ESPAsyncWebServer.h>
#include <WebSocketsServer.h>
#include "index.h"
#include <MD_Parola.h>
#include <MD_MAX72xx.h>
#define HARDWARE_TYPE MD_MAX72XX::FC16_HW
#define MAX_DEVICES 4
#define CS_PIN 21
const char* ssid = "YOUR_WIFI_SSID";
const char* password = "YOUR_WIFI_PASSWORD";
AsyncWebServer server(80);
WebSocketsServer webSocket = WebSocketsServer(81);
MD_Parola ledMatrix = MD_Parola(HARDWARE_TYPE, CS_PIN, MAX_DEVICES);
void webSocketEvent(uint8_t num, WStype_t type, uint8_t* payload, size_t length) {
switch (type) {
case WStype_DISCONNECTED:
Serial.printf("[%u] Disconnected!\n", num);
break;
case WStype_CONNECTED:
{
IPAddress ip = webSocket.remoteIP(num);
Serial.printf("[%u] Connected from %d.%d.%d.%d\n", num, ip[0], ip[1], ip[2], ip[3]);
}
break;
case WStype_TEXT:
String text = String((char*)payload);
Serial.println("Received: " + text);
ledMatrix.displayClear();
ledMatrix.displayScroll(text.c_str(), PA_CENTER, PA_SCROLL_LEFT, 100);
webSocket.sendTXT(num, "Displayed: " + String((char*)payload) + "on LED Matrix");
break;
}
}
void setup() {
Serial.begin(9600);
ledMatrix.begin();
ledMatrix.setIntensity(0);
ledMatrix.displayClear();
ledMatrix.displayScroll("esp32io.com", PA_CENTER, PA_SCROLL_LEFT, 100);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
webSocket.begin();
webSocket.onEvent(webSocketEvent);
server.on("/", HTTP_GET, [](AsyncWebServerRequest* request) {
Serial.println("Web Server: received a web page request");
String html = HTML_CONTENT;
request->send(200, "text/html", html);
});
server.begin();
Serial.print("ESP32 Web Server's IP address: ");
Serial.println(WiFi.localIP());
}
void loop() {
webSocket.loop();
if (ledMatrix.displayAnimate()) {
ledMatrix.displayReset();
}
}
Create the index.h file On Arduino IDE by:
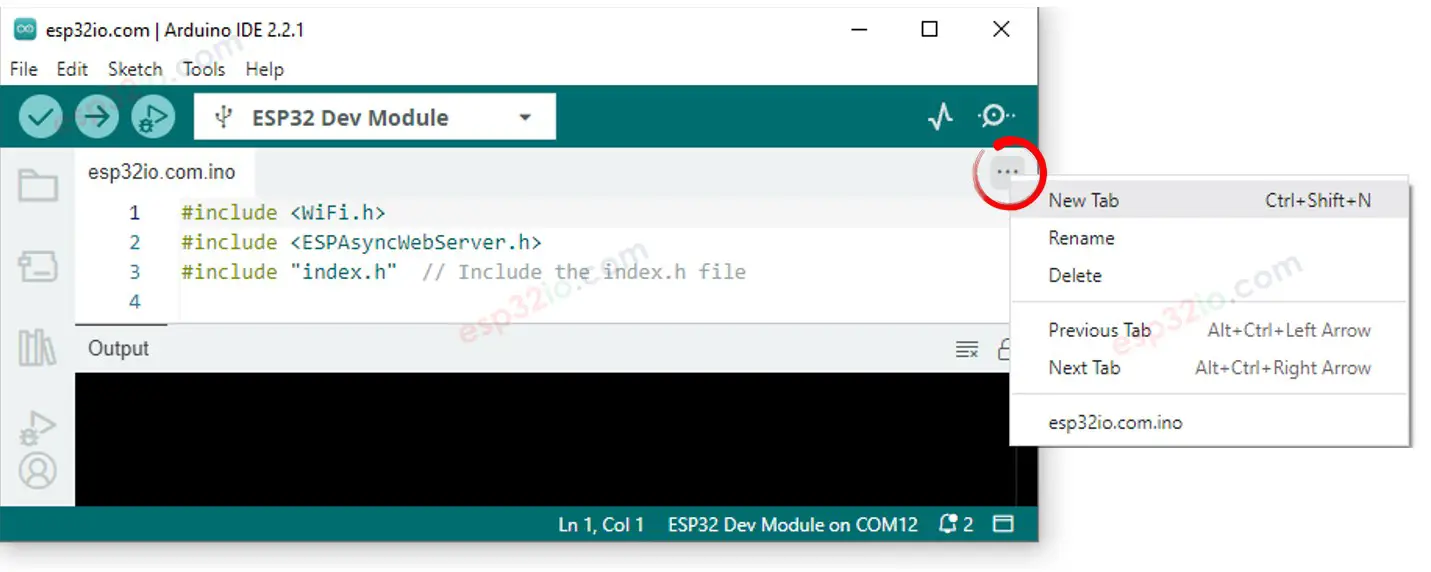
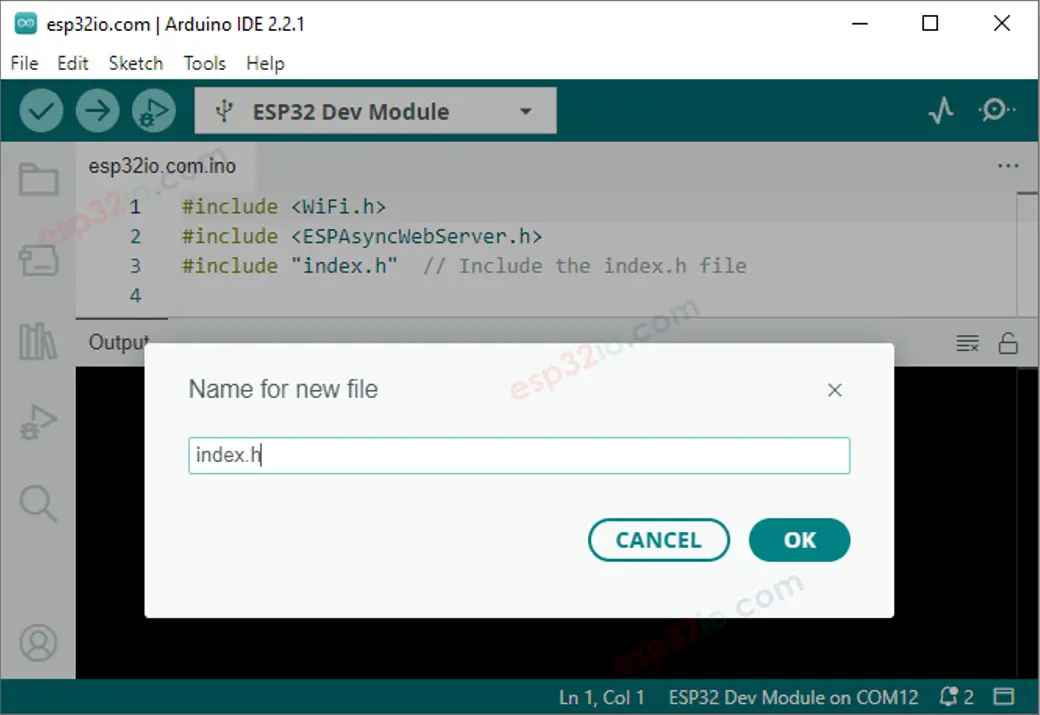
const char *HTML_CONTENT = R"=====(
<!DOCTYPE html>
<!-- saved from url=(0019)http://192.168.0.2/ -->
<html><head><meta http-equiv="Content-Type" content="text/html; charset=windows-1252">
<title>ESP32 WebSocket</title>
<meta name="viewport" content="width=device-width, initial-scale=0.7">
<link rel="icon" href="https://diyables.io/images/page/diyables.svg">
<style>
body {
font-size: 16px;
}
.chat-container {
width: 400px;
margin: 0 auto;
padding: 10px;
}
.chat-messages {
height: 250px;
overflow-y: auto;
border: 1px solid #444;
padding: 5px;
margin-bottom: 5px;
}
.user-input {
display: flex;
margin-bottom: 20px;
}
.user-input input {
flex: 1;
border: 1px solid #444;
padding: 5px;
}
.user-input button {
margin-left: 5px;
background-color: #007bff;
color: #fff;
border: none;
padding: 5px 10px;
cursor: pointer;
}
.websocket {
display: flex;
align-items: center;
margin-bottom: 5px;
}
.websocket button {
background-color: #007bff;
color: #fff;
border: none;
padding: 5px 10px;
cursor: pointer;
}
.websocket .label {
margin-left: auto;
}
</style>
<script>
var ws;
var wsm_max_len = 4096;
function update_text(text) {
var chat_messages = document.getElementById("chat-messages");
chat_messages.innerHTML += text + '<br>';
chat_messages.scrollTop = chat_messages.scrollHeight;
}
function send_onclick() {
if(ws != null) {
var message = document.getElementById("message").value;
if (message) {
document.getElementById("message").value = "";
ws.send(message + "\n");
update_text('<span style="color:navy">' + message + '</span>');
}
}
}
function connect_onclick() {
if(ws == null) {
ws = new WebSocket("ws:
document.getElementById("ws_state").innerHTML = "CONNECTING";
ws.onopen = ws_onopen;
ws.onclose = ws_onclose;
ws.onmessage = ws_onmessage;
} else
ws.close();
}
function ws_onopen() {
document.getElementById("ws_state").innerHTML = "<span style='color:blue'>CONNECTED</span>";
document.getElementById("bt_connect").innerHTML = "Disconnect";
document.getElementById("chat-messages").innerHTML = "";
}
function ws_onclose() {
document.getElementById("ws_state").innerHTML = "<span style='color:gray'>CLOSED</span>";
document.getElementById("bt_connect").innerHTML = "Connect";
ws.onopen = null;
ws.onclose = null;
ws.onmessage = null;
ws = null;
}
function ws_onmessage(e_msg) {
e_msg = e_msg || window.event;
console.log(e_msg.data);
update_text('<span style="color:blue">' + e_msg.data + '</span>');
}
</script>
</head>
<body>
<div class="chat-container">
<h2>ESP32 WebSocket</h2>
<div class="websocket">
<button class="connect-button" id="bt_connect" onclick="connect_onclick()">Connect</button>
<span class="label">WebSocket: <span id="ws_state"><span style="color:blue">CLOSED</span></span></span>
</div>
<div class="chat-messages" id="chat-messages"></div>
<div class="user-input">
<input type="text" id="message" placeholder="Type your message...">
<button onclick="send_onclick()">Send</button>
</div>
<div class="sponsor">Sponsored by <a href="https://amazon.com/diyables">DIYables</a></div>
</div>
</body></html>
)=====";
Now you have the code in two files: esp32io.com.ino and index.h
Click Upload button on Arduino IDE to upload code to ESP32.
Open the Serial Monitor
Check out the result on Serial Monitor.
Connecting to WiFi...
Connected to WiFi
ESP32 Web Server's IP address IP address: 192.168.0.2
Take note of the IP address displayed, and enter this address into the address bar of a web browser on your smartphone or PC.
You will see the webpage it as below:
Click the CONNECT button to connect the webpage to ESP32 via WebSocket.
Type some words and send them to ESP32.
You will see the response from ESP32.
※ NOTE THAT:
If you modify the HTML content in the index.h and does not touch anything in esp32io.com.ino file, when you compile and upload code to ESP32, Arduino IDE will not update the HTML content.
To make Arduino IDE update the HTML content in this case, make a change in the esp32io.com.ino file (e.g. adding empty line, add a comment....)
The above ESP32 code contains line-by-line explanation. Please read the comments in the code!