ESP32 - LED Strip
In this tutorial, we are going to learn how to program ESP32 to control a LED strip to emit the light.
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to LED Strip
A LED strip, also known as LED tape or LED ribbon, is a flexible circuit board with surface-mounted LEDs that emit light. These strips are versatile and commonly used for decorative lighting in various applications. LED strips come in a range of colors and are often used to provide ambient lighting, accent lighting, or decorative lighting effects.
LED strips come in two main types:
- Addressable LED Strips: In this type, the color and brightness of each individual LED on the strip can be independently controlled. This capability is due to the fact that each LED is assigned a specific address.
- Non-Addressable LED Strips: In contrast, non-addressable LED strips allow control over the color and brightness, but this control applies uniformly to all LEDs on the strip.
This tutorial will focus on the Non-Addressable LED Strips. For Addressable LED Strips, please refer to the following tutorials:
- ESP32 - NeoPixel LED Strip tutorial
- ESP32 - WS2812B LED Strip tutorial
- ESP32 - Dotstar LED Strip tutorial
Non-Addressable LED Strip Pinout
Non-Addressable LED Strip has two main types:
- Non-Addressable 1-color LED strip: Only one color defined by manufacturer.
- Non-Addressable RGB LED strip: any colors
A Non-Addressable 1-color LED Strip usually has two pins:
- 12V/24V pin: needs to be connected to the positive pin of 12V or 24V DC power supply
- GND pin: needs to be connected to the negative pin of 12V or 24V DC power supply
A Non-Addressable RGB LED Strip usually has four pins:
- 12V/24V pin: needs to be connected to the positive pin of 12V or 24V DC power supply
- R pin: This pin is used to control the red color. Connecting this pin to the negative pin of the power supply enables the red color
- G pin: This pin is used to control the green color. Connecting this pin to the negative pin of the power supply enables the green color
- B pin: This pin is used to control the blue color. Connecting this pin to the negative pin of the power supply enables the blue color
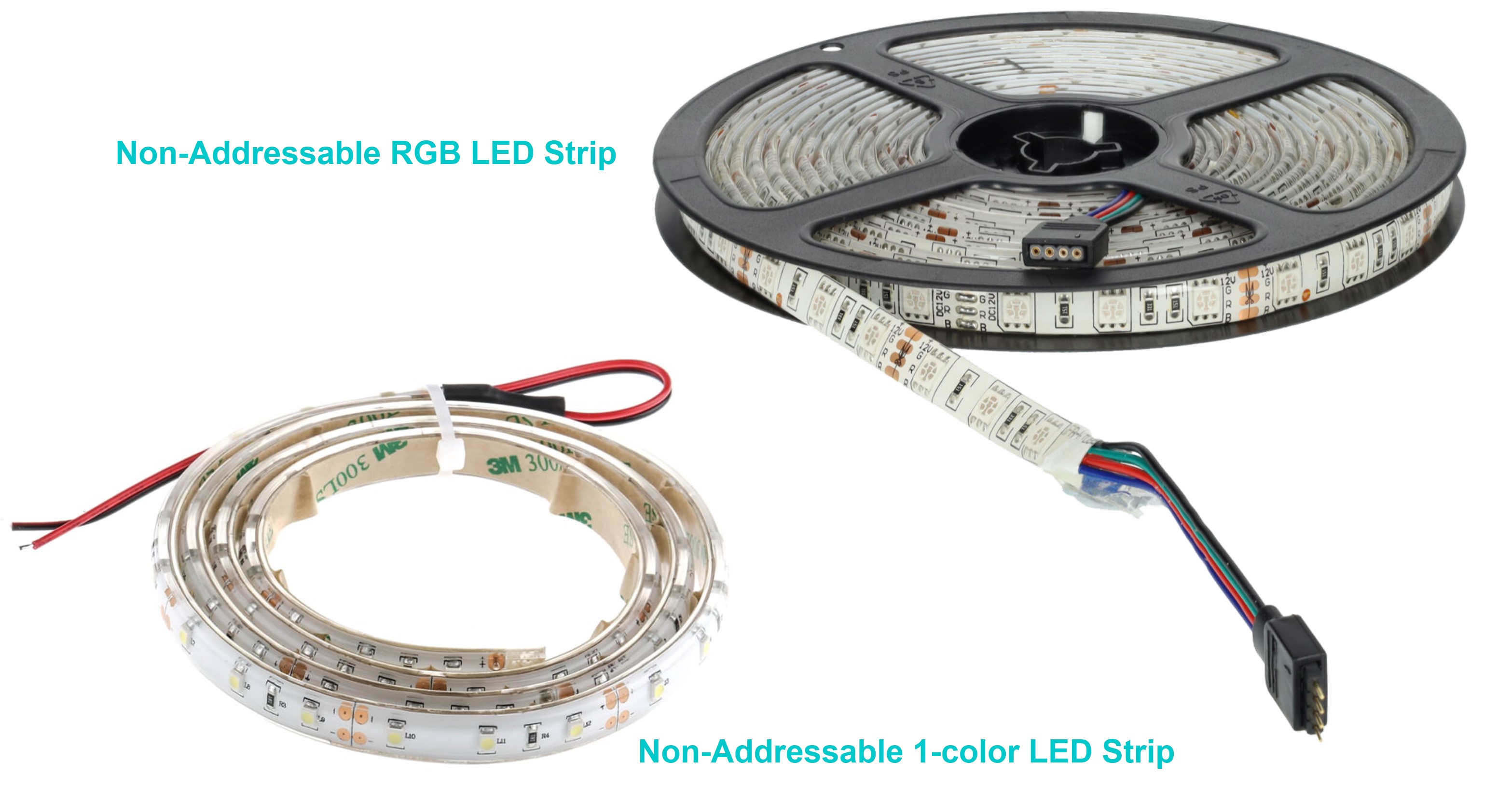
We will learn how to control the both types by ESP32 one-by-one.
How to Control a Non-Addressable 1-color LED strip.
If 12V LED strip is powered by 12V power supply, it emits light. To control a 12V LED strip, we need to use a relay in between ESP32 and 12V LED strip. ESP32 can control the 12V LED strip via the relay. Unfamiliar with relay, including their pinouts, functionality, and programming? learn about relay in the ESP32 - Relay tutorial
Wiring Diagram.
Wiring Diagram between ESP32 and Non-Addressable 1-color LED strip.
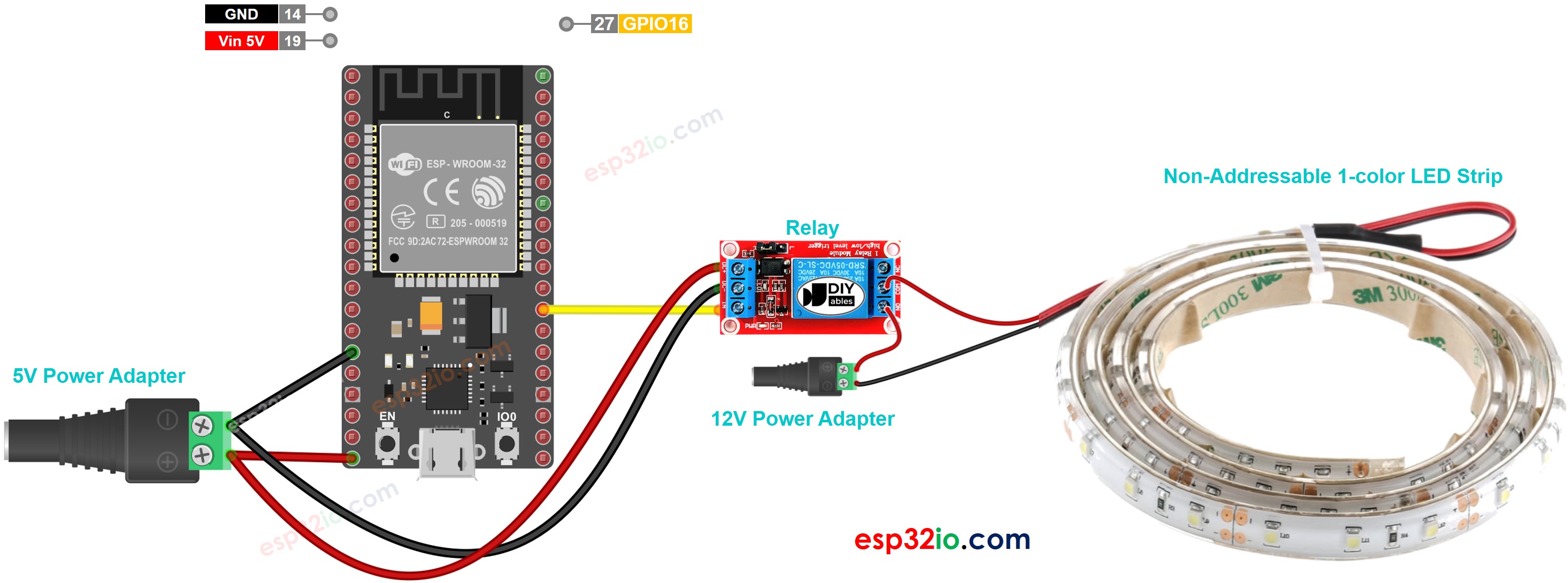
This image is created using Fritzing. Click to enlarge image
Wiring Diagram between ESP32 and Non-Addressable RGB LED strip.
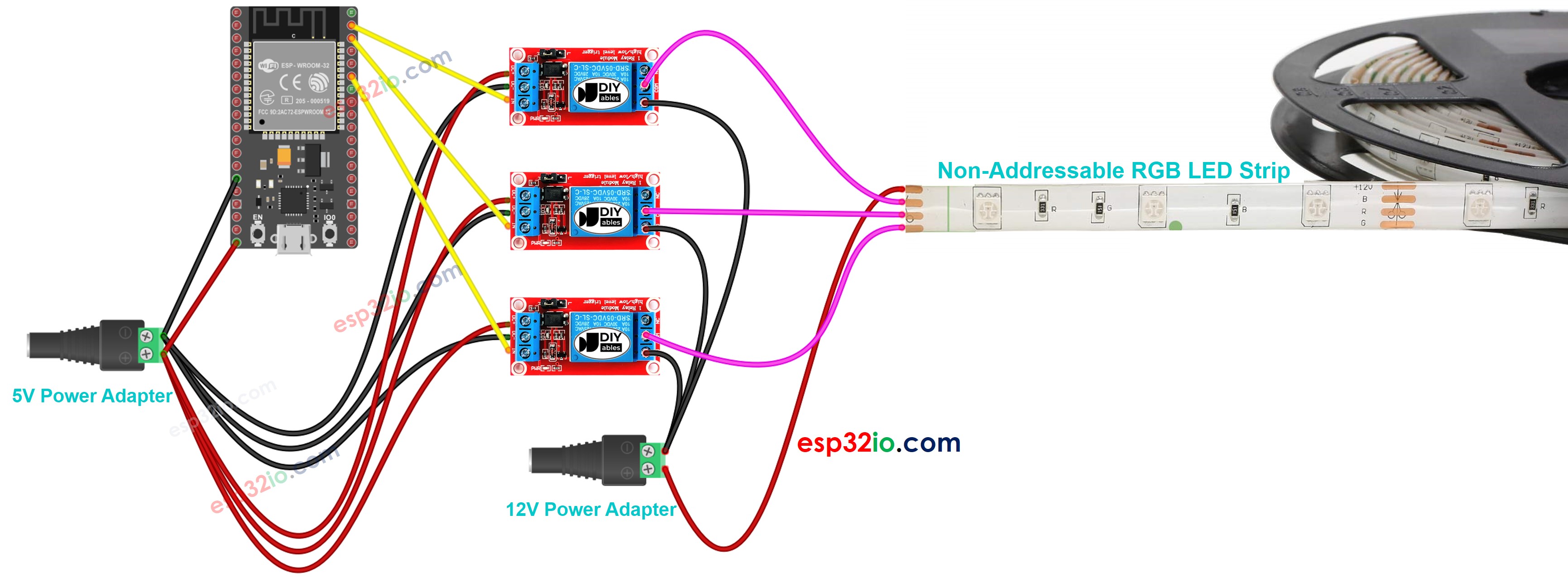
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
ESP32 Code
ESP32 Code for controlling Non-Addressable 1-color LED strip.
The below code repeatedly turns the LED strip ON in 5 seconds and OFF in 5 seconds,
ESP32 Code for controlling Non-Addressable RGB LED strip.
The below code repeatedly control the color of the RGB LED strip (red, green, blue, yellow, magenta, cyan and white)
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Connect ESP32 to PC via USB cable
- Open Arduino IDE, select the right board and port
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
- Check out the LED strip's state
Code Explanation
Read the line-by-line explanation in comment lines of code!
Please note that, to control the brightness an other colors of non-addressable LED strip, we need to use the L298N driver instead of relay
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.