ESP32 - Ethernet
This tutorial instructs you how to use W5500 Ethernet module to connect ESP32 to Internet or your LAN Network. In detail, we will learn:
How to connect ESP32 to W5500 Ethernet module
How to program ESP32 to make HTTP request via Ethernet
How to program ESP32 to create a simple web server via Ethernet
Or you can buy the following sensor kits:
Disclosure: Some of the links in this section are Amazon affiliate links, meaning we may earn a commission at no additional cost to you if you make a purchase through them. Additionally, some links direct you to products from our own brand, DIYables.
The W5500 Ethernet module has two interfaces:
RJ45 interface: to connect to router/switch via Ethernet cable
SPI interface: to connect to ESP32 board, including 10 pins:
NC pin: let this pin unconnected.
INT pin: let this pin unconnected.
RST pin: reset pin, connect this pin to the EN pin of ESP32.
GND pin: connect this pin to the ESP32's GND pin.
5V pin: let this pin unconnected.
3.3V pin: connect this pin to the ESP32's 3.3V pin.
MISO pin: connect this pin to the ESP32's SPI MISO pin.
MOSI pin: connect this pin to the ESP32's SPI MOSI pin.
SCS pin: connect this pin to the ESP32's SPI CS pin.
SCLK pin: connect this pin to the ESP32's SPI SCK pin.
image source: diyables.io
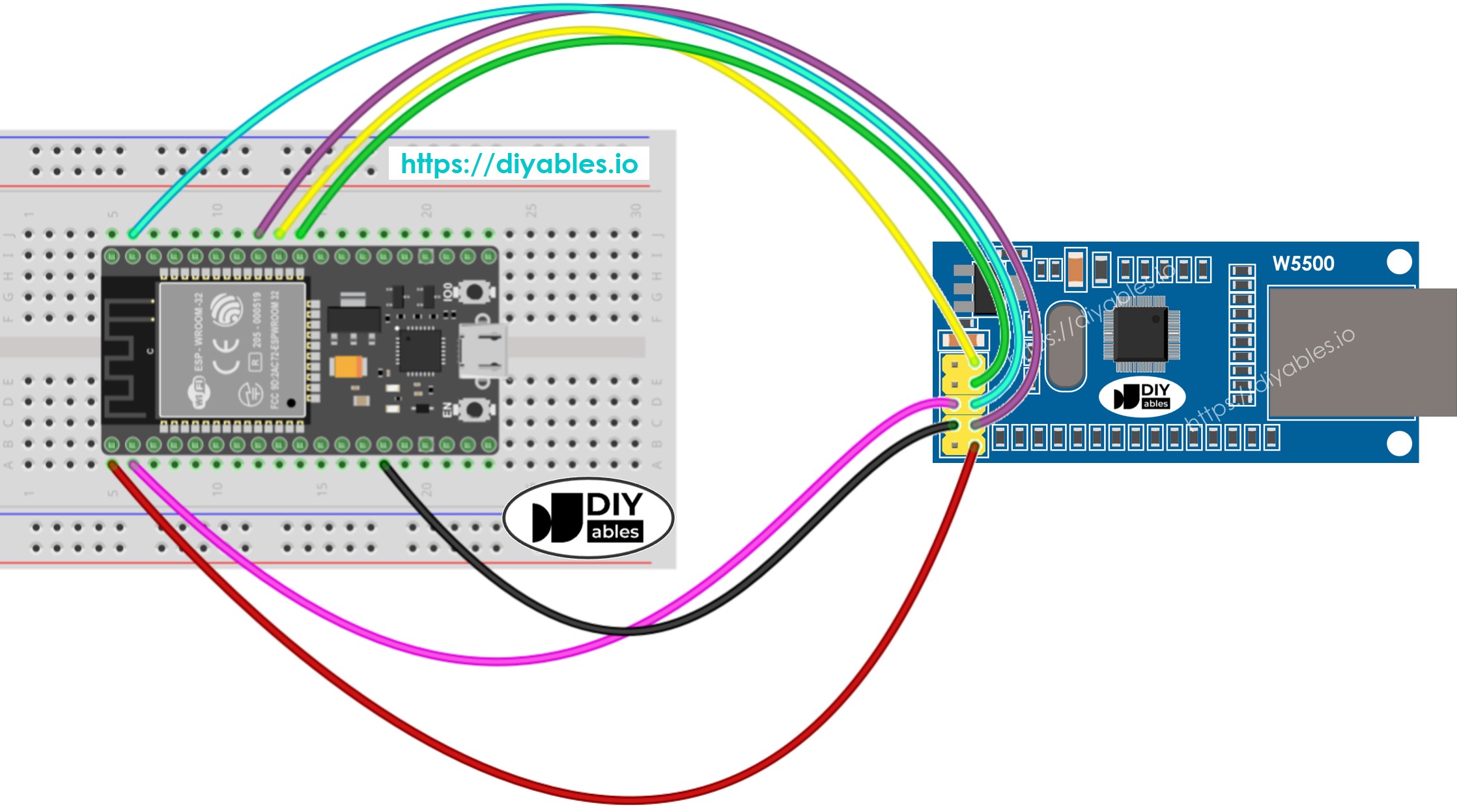
This image is created using Fritzing. Click to enlarge image
image source: diyables.io
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: How to Power ESP32.
The following code functions as a web client, making HTTP requests to the web server at http://example.com/.
#include <SPI.h>
#include <Ethernet.h>
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xEF };
EthernetClient client;
int HTTP_PORT = 80;
String HTTP_METHOD = "GET";
char HOST_NAME[] = "example.com";
String PATH_NAME = "/";
void setup() {
Serial.begin(9600);
delay(1000);
Serial.println("ESP32 - Ethernet Tutorial");
if (Ethernet.begin(mac) == 0) {
Serial.println("Failed to obtaining an IP address");
if (Ethernet.hardwareStatus() == EthernetNoHardware)
Serial.println("Ethernet shield was not found");
if (Ethernet.linkStatus() == LinkOFF)
Serial.println("Ethernet cable is not connected.");
while (true)
;
}
if (client.connect(HOST_NAME, HTTP_PORT)) {
Serial.println("Connected to server");
client.println(HTTP_METHOD + " " + PATH_NAME + " HTTP/1.1");
client.println("Host: " + String(HOST_NAME));
client.println("Connection: close");
client.println();
while (client.connected()) {
if (client.available()) {
char c = client.read();
Serial.print(c);
}
}
client.stop();
Serial.println();
Serial.println("disconnected");
} else {
Serial.println("connection failed");
}
}
void loop() {
}
Do the wiring between the Ethernet module and ESP32 as the above wiring diagram
Connect the ESP32 to PC via an USB cable
Connect the Ethernet module to your router/switch via an Ethernet cable
Open Arduino IDE on your PC.
Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
Click to the Libraries icon on the left bar of the Arduino IDE.
Search “Ethernet”, then find the Ethernet library by Various
Click Install button to install Ethernet library.
Open Serial On Arduino IDE
Copy the above code and paste it to Arduino IDE
Click Upload button on Arduino IDE to upload code to ESP32
Check out the result on Serial Monitor, it looks like below:
ESP32 - Ethernet Tutorial
Connected to server
HTTP/1.1 200 OK
Accept-Ranges: bytes
Age: 208425
Cache-Control: max-age=604800
Content-Type: text/html; charset=UTF-8
Date: Fri, 12 Jul 2024 07:08:42 GMT
Etag: "3147526947"
Expires: Fri, 19 Jul 2024 07:08:42 GMT
Last-Modified: Thu, 17 Oct 2019 07:18:26 GMT
Server: ECAcc (lac/55B8)
Vary: Accept-Encoding
X-Cache: HIT
Content-Length: 1256
Connection: close
<!doctype html>
<html>
<head>
<title>Example Domain</title>
<meta charset="utf-8" />
<meta http-equiv="Content-type" content="text/html; charset=utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
</head>
<body>
<div>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents. You may use this
domain in literature without prior coordination or asking for permission.</p>
<p><a href="https://www.iana.org/domains/example">More information...</a></p>
</div>
</body>
</html>
disconnected
※ NOTE THAT:
If there is another device on the same local network with the same MAC address, it might not work.
The following code transforms the ESP32 into a web server that responds to web browsers with a simple webpage.
#include <SPI.h>
#include <Ethernet.h>
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xEF };
EthernetServer server(80);
void setup() {
Serial.begin(9600);
delay(1000);
Serial.println("ESP32 - Ethernet Tutorial");
if (Ethernet.begin(mac) == 0) {
Serial.println("Failed to obtaining an IP address");
if (Ethernet.hardwareStatus() == EthernetNoHardware)
Serial.println("Ethernet shield was not found");
if (Ethernet.linkStatus() == LinkOFF)
Serial.println("Ethernet cable is not connected.");
while (true)
;
}
server.begin();
Serial.print("ESP32 - Web Server IP Address: ");
Serial.println(Ethernet.localIP());
}
void loop() {
EthernetClient client = server.available();
if (client) {
Serial.println("new client");
bool currentLineIsBlank = true;
while (client.connected()) {
if (client.available()) {
char c = client.read();
Serial.write(c);
if (c == '\n' && currentLineIsBlank) {
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html");
client.println("Connection: close");
client.println();
client.println("<!DOCTYPE HTML>");
client.println("<html>");
client.println("<body>");
client.println("<h1>ESP32 Web Server with Ethernet</h1>");
client.println("</body>");
client.println("</html>");
break;
}
if (c == '\n') {
currentLineIsBlank = true;
} else if (c != '\r') {
currentLineIsBlank = false;
}
}
}
delay(1);
client.stop();
Serial.println("client disconnected");
}
}
Copy the above code and paste it to Arduino IDE
Click Upload button on Arduino IDE to upload code to ESP32
Check out the result on Serial Monitor, it looks like below:
ESP32 - Ethernet Tutorial
ESP32 - Web Server IP Address: 192.168.0.2