ESP32 - Joystick - Servo Motor
In this tutorial, we are going to learn how to use ESP32 and a joystick to control two servo motors or a pan-tilt kit with servos.
A joystick has two built-in potentiometers square with each other (called X-axis and Y-axis). These potentiometers output the analog values (called X-value and Y-value) on VRX and VRY pins. We will use these analog values to control two servo motors independently: X-value controls servo #1 and Y-value controls servo #2. In the case of the pan-tilt kit, the movement of two servo motors creates 3-D movement.
There are two application use cases:
- Use case 1: The servo motors rotate according to the movement of the joystick's thump:
- The angles of servo motors are in proportion to the values of the joystick's X-value and Y-value.
- When we release the joystick's thump ⇒ all values returns back to the center values ⇒ servo motors automatically returns back to the center position
- Use case 2: use the joystick to command servo motors (up/down/left/right commands)
- When joystick's thump is pushed to the left, move the servo motor #1 in a clockwise direction step-by-step
- When joystick's thump is pushed to the right, move the servo motor #1 in an anti-clockwise direction step-by-step
- When joystick's thump is pushed up, move the servo motor #2 in clockwise direction step-by-step
- When joystick's thump is pushed down, move the servo motor #2 in an anti-clockwise direction step-by-step
- When we release the joystick's thump ⇒ the servo motors will not return to the center position
- If the joystick is pressed (push button), The servo motors will return to the center position
- How to connect ESP32 with joystick and servo motor using breadboard
- How to connect ESP32 with joystick and servo motor using screw terminal block breakout board
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Connect the joystick and servo motor to the ESP32 board as above the wiring diagram.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Type ServoESP32 on the search box, then look for the servo library by Jaroslav Paral. Please be aware that both version 1.1.1 and 1.1.0 are affected by bugs. Kindly choose a different version.
- Click Install button to install servo motor library for ESP32.
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
- Open Serial Monitor
- Push the joystick in some direction
- See the servo motor's rotation
- See the result on Serial Monitor
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search “ezButton”, then find the button library by ArduinoGetStarted.com
- Click Install button to install ezButton library.
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
- Open Serial Monitor
- Push the joystick in some direction
- See the servo motor's rotation
- See the result on Serial Monitor
- The ESP32 ADC is not perfectly accurate and might need calibration for correct results. Each ESP32 board can be a bit different, so you need to calibrate the ADC for each individual board.
- Calibration can be difficult, especially for beginners, and might not always give the exact results you want.
We will explore the code for both use cases in the next parts.
This tutorial shows how to program the ESP32 using the Arduino language (C/C++) via the Arduino IDE. If you’d like to learn how to program the ESP32 with MicroPython, visit this ESP32 MicroPython - Joystick - Servo Motor tutorial.
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to Joystick and Servo Motor
If you do not know about joystick and servo motor (pinout, how it works, how to program ...), learn about them in the following tutorials:
Wiring Diagram
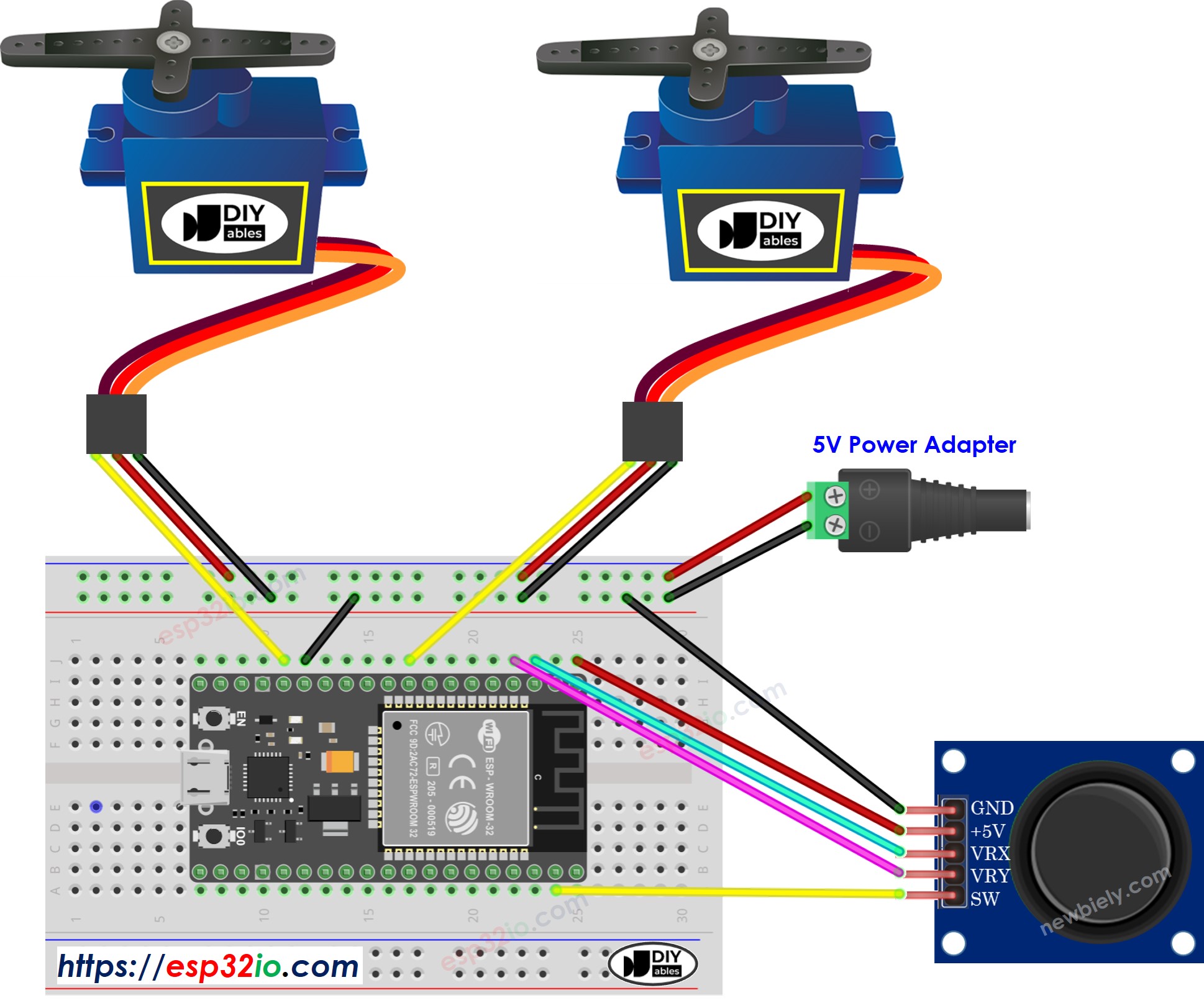
This image is created using Fritzing. Click to enlarge image
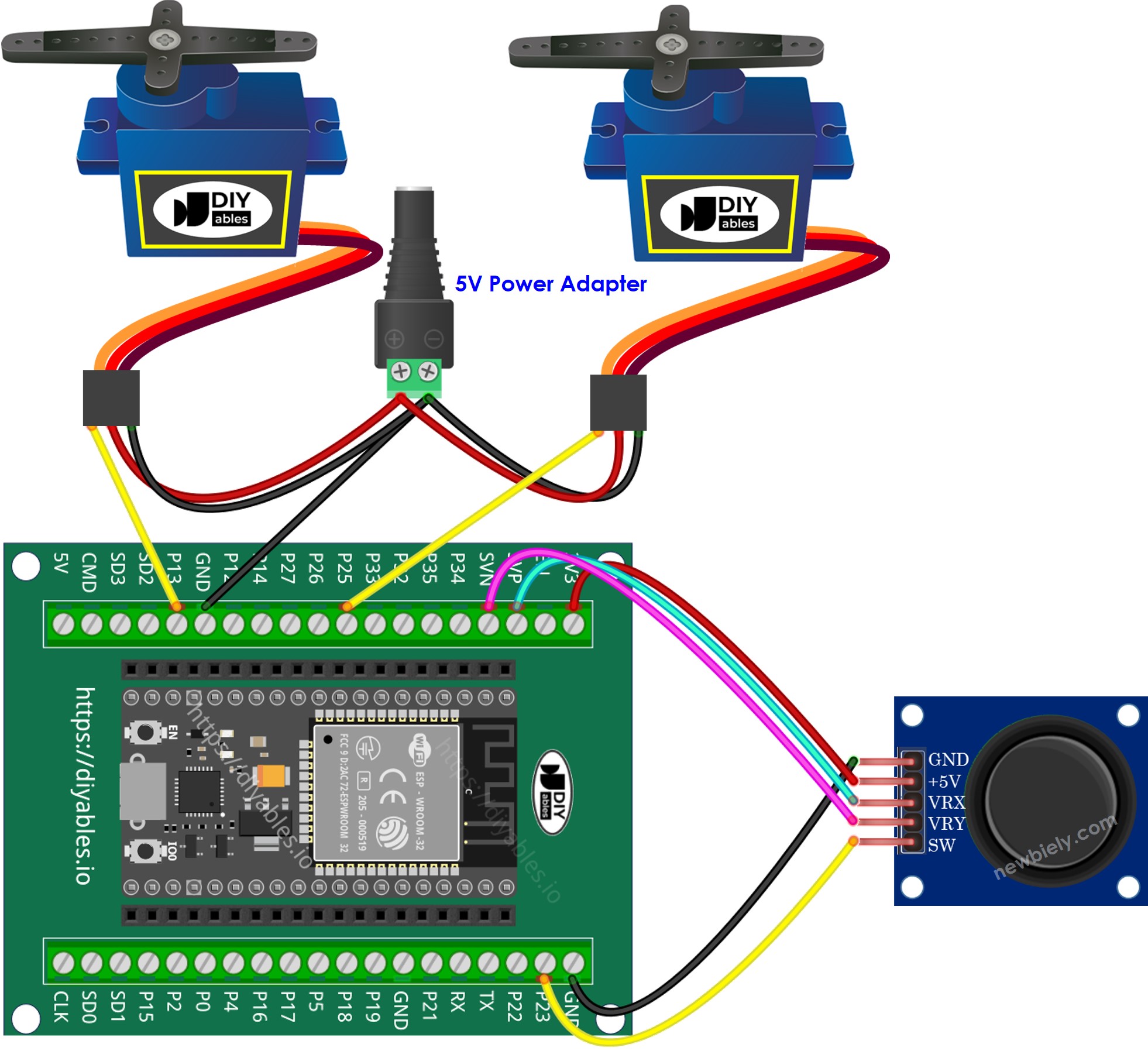
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
ESP32 Code
ESP32 Code - The servo motors rotate according to the movement of the joystick's thump
Quick Instructions
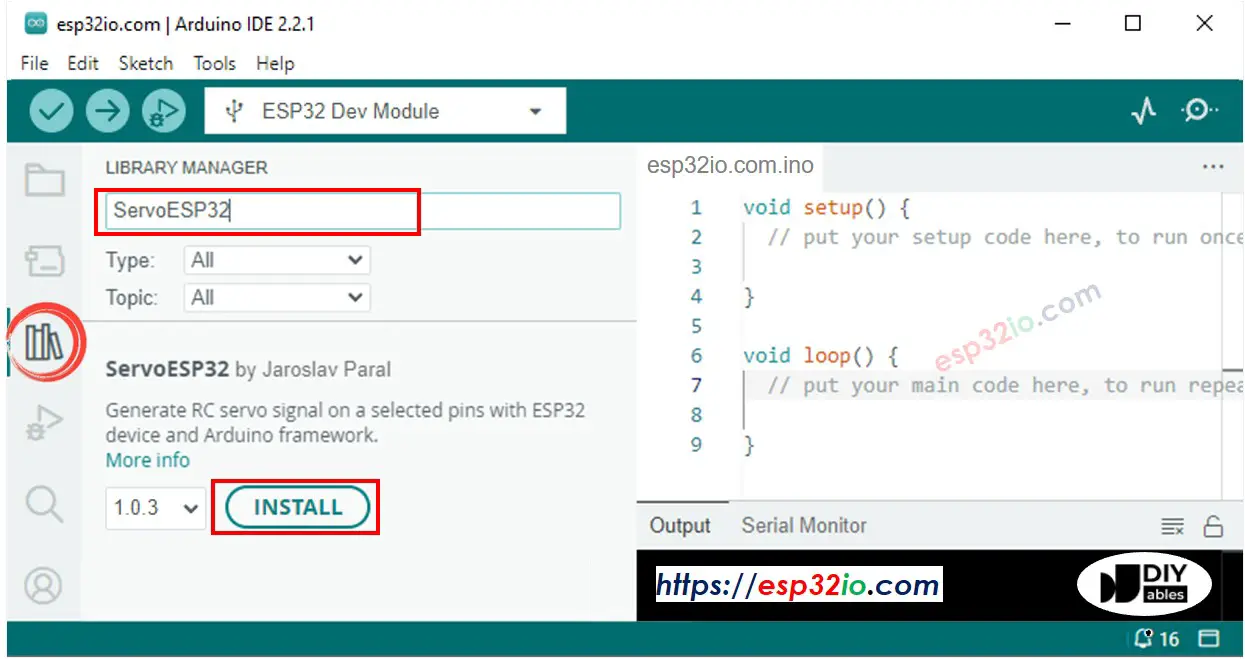
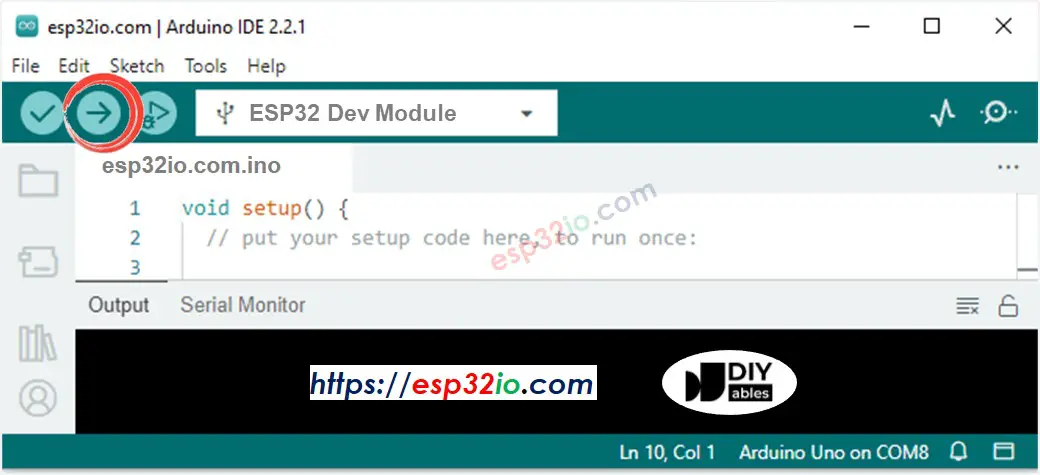
You may have noticed that the servo motor does not move proportionally to the joystick movement. This issue is not due to the joystick or the servo motor itself, but rather the ADC of the ESP32. The end of this tutorial will explain why this happens.
ESP32 Code - Use the joystick to command servo motors
Quick Instructions
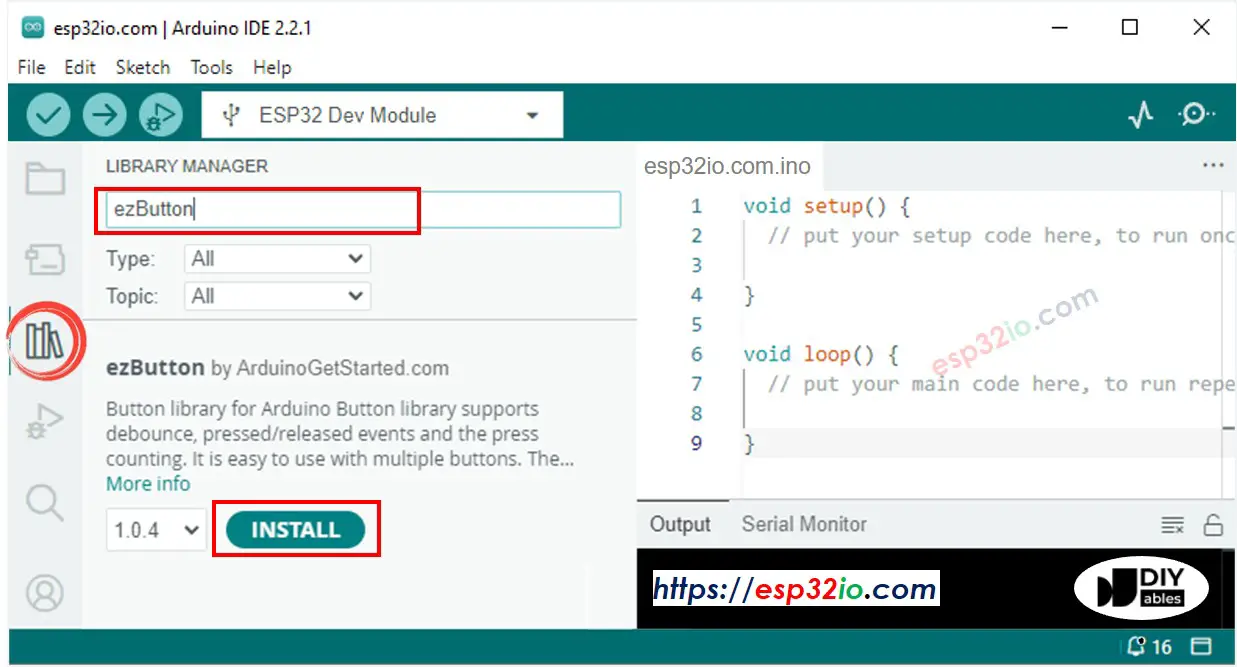
Code Explanation
Read the line-by-line explanation in comment lines of source code!
※ NOTE THAT:
This tutorial uses the analogRead() function to read values from an ADC (Analog-to-Digital Converter) connected to a joystick. The ESP32 ADC is good for projects that do NOT need high accuracy. However, for projects that need precise measurements, please note:
For projects that need high precision, consider using an external ADC (e.g ADS1115) with the ESP32 or using an Arduino, which has a more reliable ADC. If you still want to calibrate the ESP32 ADC, refer to ESP32 ADC Calibration Driver
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.