ESP32 - IR Remote Control
You've probably come across the infrared remote control, also called the IR remote control, when using devices like TVs and air conditioners at home... In this guide, we'll learn how to use the infrared (IR) remote control and an infrared receiver to control the ESP32. In detail, we will learn:
- How to connect an IR receiver to ESP32 board
- How to program ESP32 to read the command from IR remote controller via IR receiver
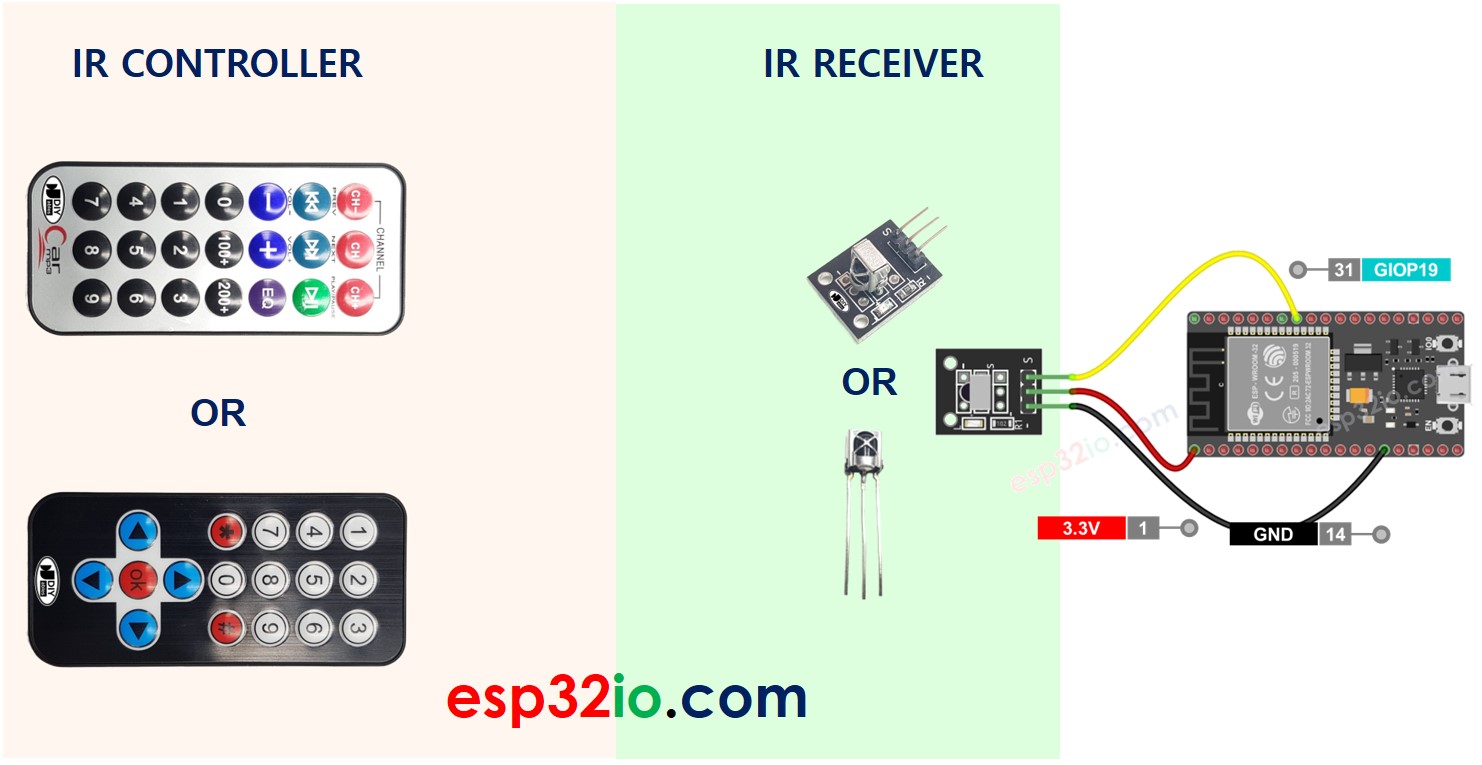
Hardware Used In This Tutorial
1 | × | ESP-WROOM-32 Dev Module | |
1 | × | USB Cable Type-C | |
1 | × | IR Remote Controller and Receiver Kit | |
1 | × | CR2025 Battery | |
1 | × | Breadboard | |
1 | × | Jumper Wires | |
1 | × | (Recommended) ESP32 Screw Terminal Adapter |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to IR Remote Control
An IR control system has two components: an IR remote controller and an IR receiver.
- The IR remote controller is used to send commands using infrared signals.
- The IR receiver captures and interprets these signals to control the device.
An IR kit typically consists of the two components mentioned above: an IR remote controller and an IR receiver.
IR remote controller
The IR remote controller is a portable device that emits infrared signals. It is designed with a keypad featuring multiple buttons:
- Each button on the remote controller is assigned a specific function or command.
- When a button is pressed, the remote emits an infrared signal containing a unique code or pattern linked to the pressed button.
- These infrared signals fall within the infrared spectrum and are not visible to the human eye.
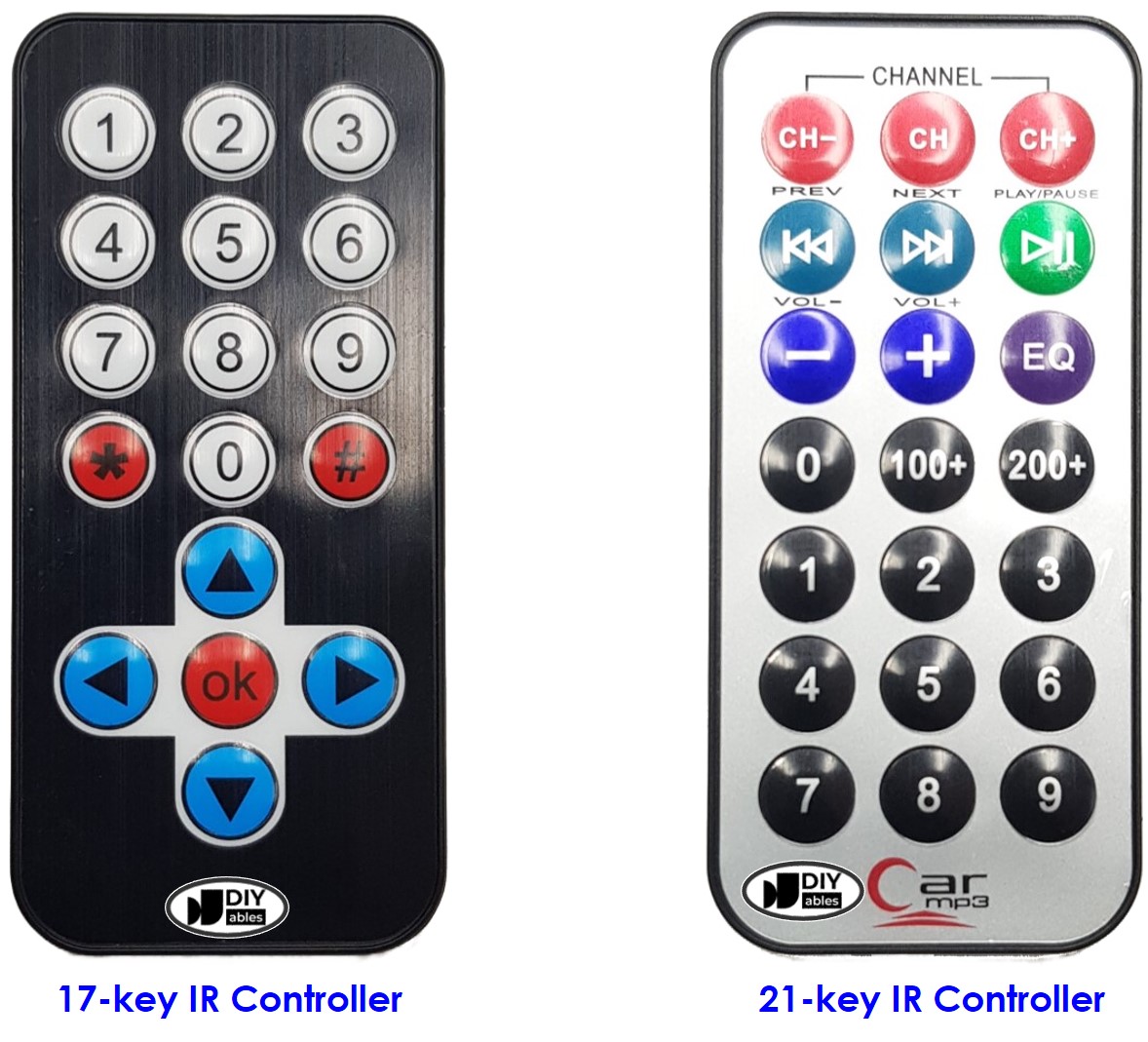
IR Receiver
The IR receiver module is a sensor that detects and receives the infrared signals emitted by the remote controller.
The infrared receiver detects the incoming infrared signals and converts them into the code (command) representing the button pressed on the remote controller.
The IR Receiver can be a sensor or a module. You can use the following choices:
- IR Receiver Module only
- IR Receiver Sensor only
- IR Receiver Sensor + Adapter
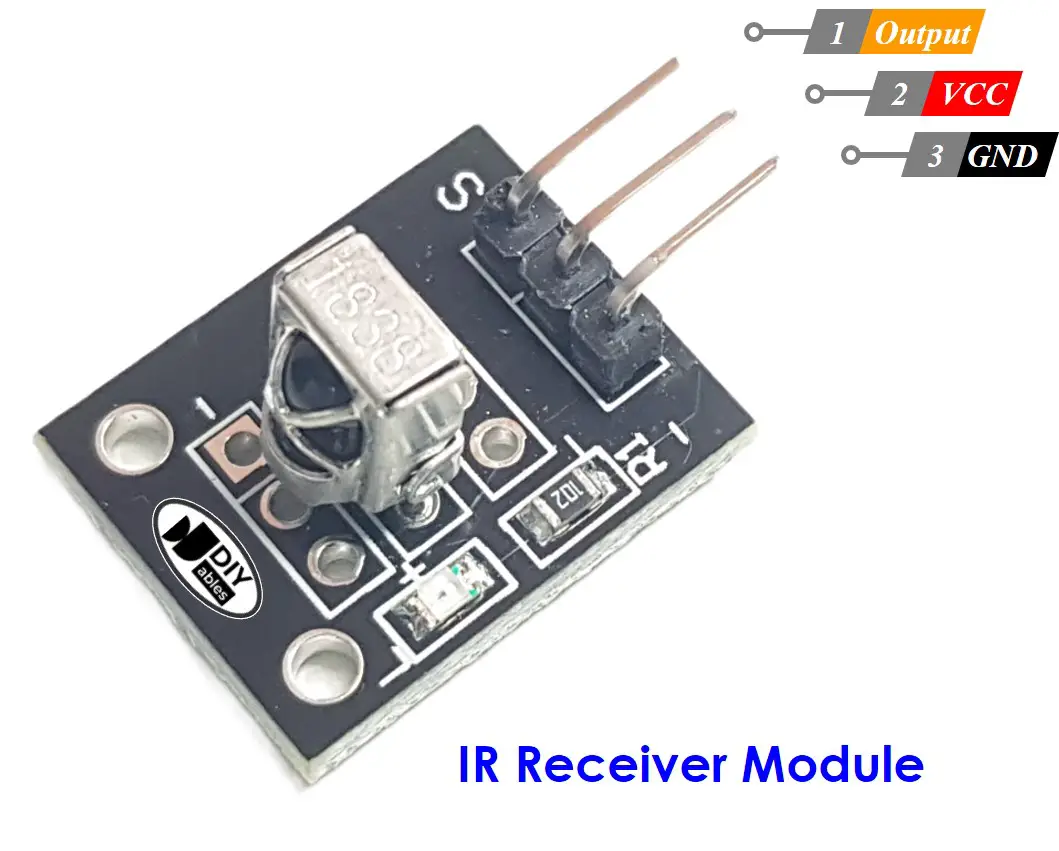
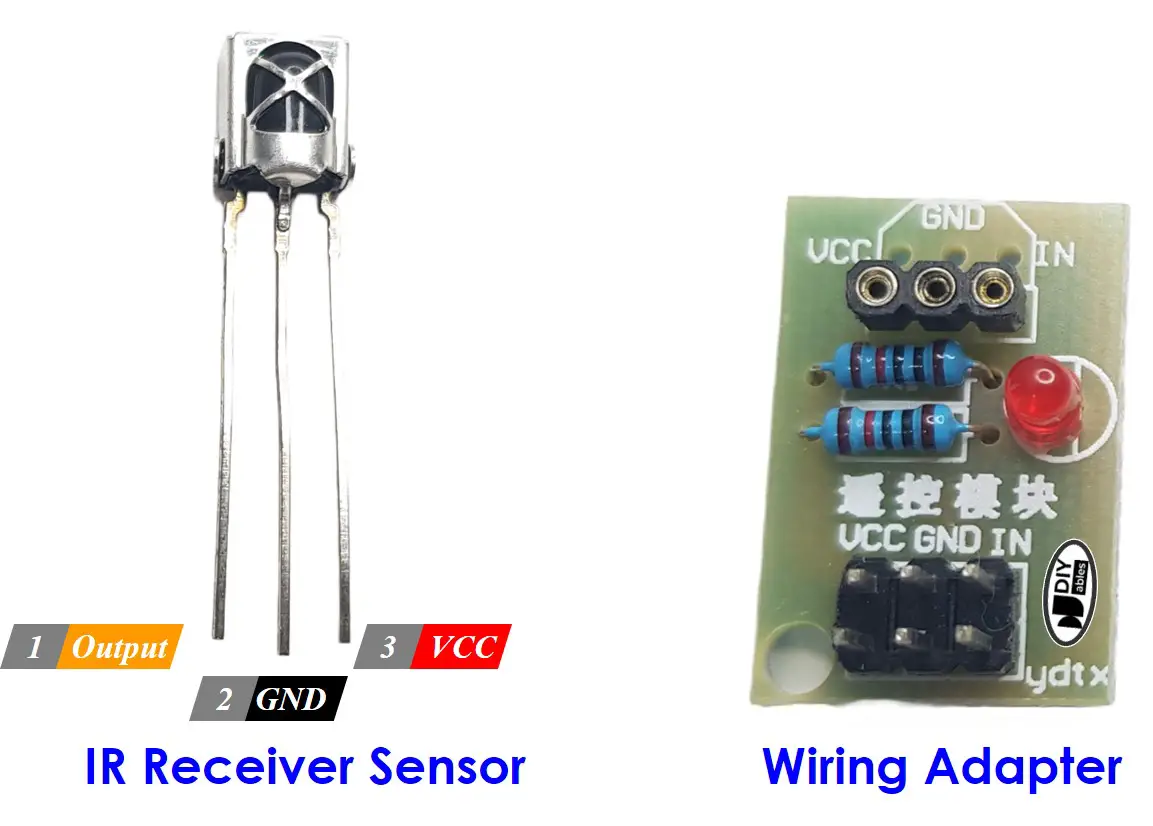
IR Receiver Pinout
IR receiver module or sensor has three pins:
- VCC pin: Connect this pin to the 3.3V or 5V pin of the ESP32 or external power source.
- GND pin: Connect this pin to GND pin of the ESP32 or external power source..
- OUT (Output) pin: This pin is the output pin of the IR receiver module. Connected to a digital input pin on the ESP32.
How It Works
When user presses a button on the IR remote controller
- The IR remote controller encodes the command corresponding to the button to the infrared signal via a specific protocol
- The IR remote controller emits the encoded infrared signal
- The IR receiver receives the encoded infrared signal
- The IR receiver decoded the encoded infrared signal in to the command
- The ESP32 reads the command from the IR receiver
- The ESP32 maps the command to the key pressed
When a button is pressed on the IR remote controller, the following sequence of events occurs:
- The IR remote controller encodes the button's command into an infrared signal using a specific protocol.
- The encoded infrared signal is emitted by the IR remote controller.
- The IR receiver captures and receives the encoded infrared signal.
- The IR receiver decodes the infrared signal, converting it back into the original command.
- The ESP32 reads and retrieves the command from the IR receiver.
- The ESP32 then maps the received command to the corresponding button that was pressed on the IR remote controller.
In essence, these steps outline how the IR remote controller's button press is transformed into a recognizable command that can be understood and processed by the ESP32.
Regenerate response
It may appear complicated, but don't worry. Thanks to the user-friendly DIYables_IRcontroller library, it becomes incredibly easy to accomplish.
Wiring Diagram
Wiring diagram between ESP32 and IR Receiver Module
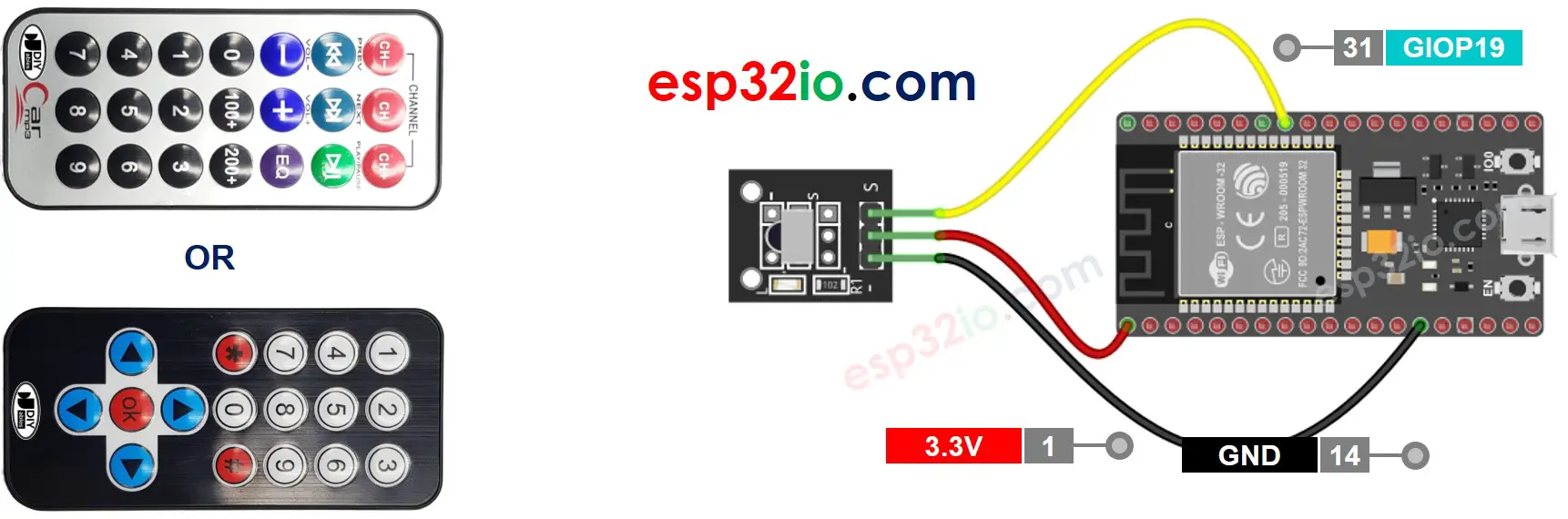
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: How to Power ESP32.
Wiring diagram between ESP32 and IR Receiver Sensor
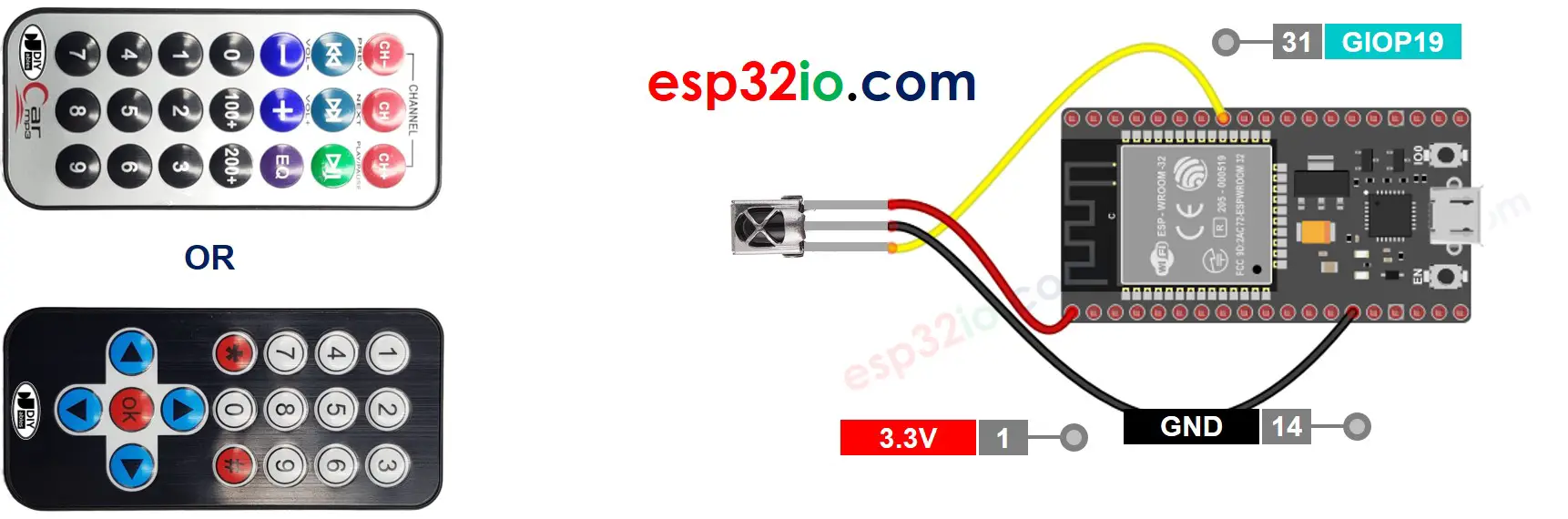
This image is created using Fritzing. Click to enlarge image
Wiring diagram between ESP32 and IR Receiver Sensor and Adapter
Before connecting the IR receiver sensor to the ESP32, you have the option to connect it to the adapter. This allows for easier integration and setup of the IR receiver sensor with the ESP32.
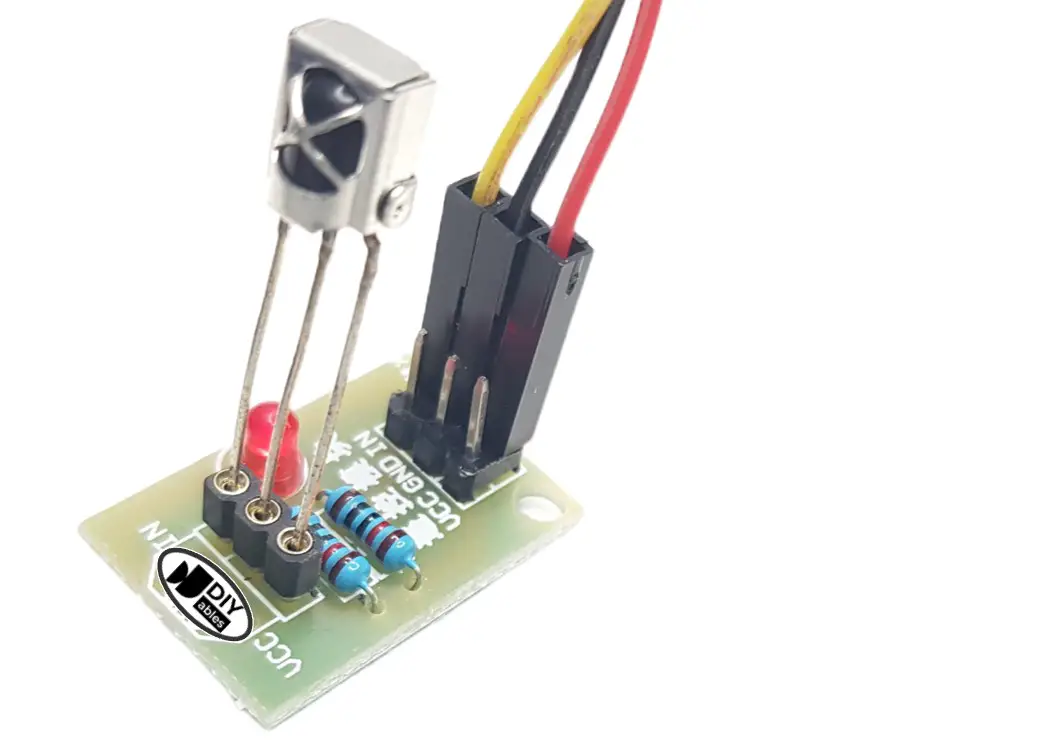
How To Program For IR Remote Controller
- Include the library:
- Declare a DIYables_IRcontroller_17 or DIYables_IRcontroller_21 object corresponds with 17-key or 21-key IR remote controllers:
- Initialize the IR Controller.
- In the loop, check if a key is pressed or not. If yes, get the key
- After detecting a key press, you can perform actions based on each key.
ESP32 Code
- ESP32 code for DIYables 17-key IR remote controller
- ESP32 code for DIYables 21-key IR remote controller
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search "DIYables_IRcontroller", then find the DIYables_IRcontroller library by DIYables
- Click Install button to install DIYables_IRcontroller library.
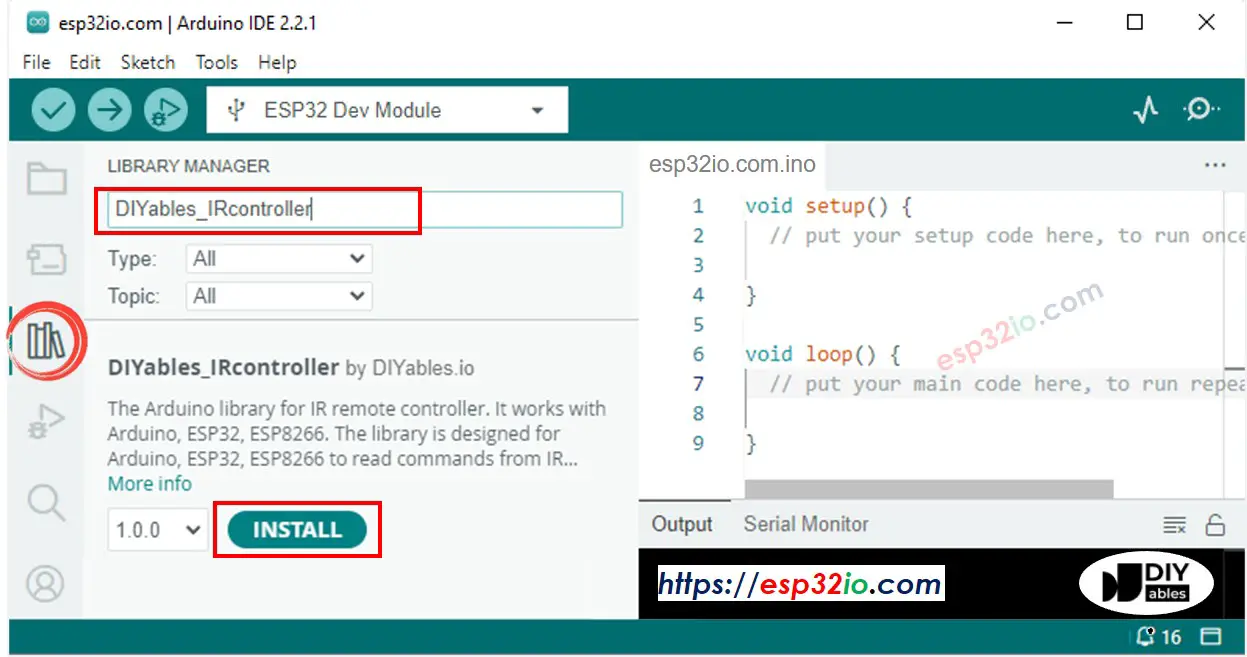
- You will be asked for installing the library dependency as below image:
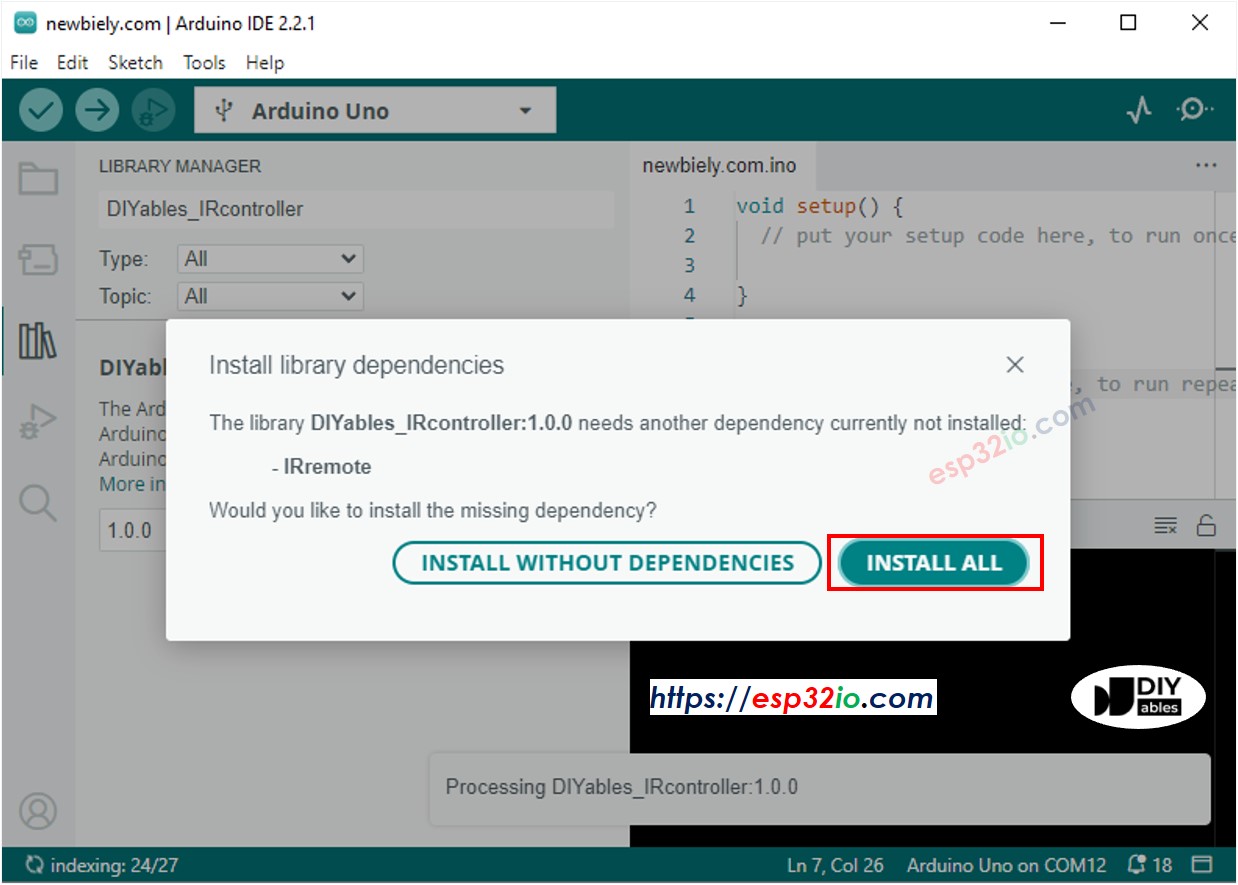
- Click Install all button to install the dependency
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
- Press keys on the remote controller one by one
- See the result on Serial Monitor.
- When you press the keys on a 21-key IR controller one by one, the following is the result:
You now have the ability to customize the code and control different devices like LEDs, fans, pumps, actuators, and more using IR remote controllers. This means you can make changes to the code to make these devices respond to your commands sent through the remote control.
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.