ESP32 - DC Motor - Limit Switch
In this ESP32 tutorial, we are going to learn how to use ESP32 to control DC motor by limit switch and L298N driver. In detail, we are going to learn:
- How to stop DC motor when a limit switch is touched
- How to change the direction of DC motor when a limit switch is touched
- How to change the direction of DC motor when two limit switches is touched
In this tutorial on ESP32, we'll explore the process of utilizing the ESP32 to manage a DC motor using a limit switch and an L298N driver. Specifically, we'll cover the following aspects:
- Stopping the DC motor upon contact with a limit switch
- Changing the direction of the DC motor upon contact with a limit switch
- Changing the direction of the DC motor when two limit switches are activated
Hardware Used In This Tutorial
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to DC Motor and Limit Switch
If you do not know about DC motor and limit switch (pinout, how it works, how to program ...), learn about them in the following tutorials:
- ESP32 - Limit Switch tutorial
- ESP32 - Controls DC Motor tutorial
Wiring Diagram
This tutorial provides the ESP32 codes for two cases: One DC motor + one limit switch, One DC motor + two limit switches.
- Wiring diagram between the DC motor and a limit switch
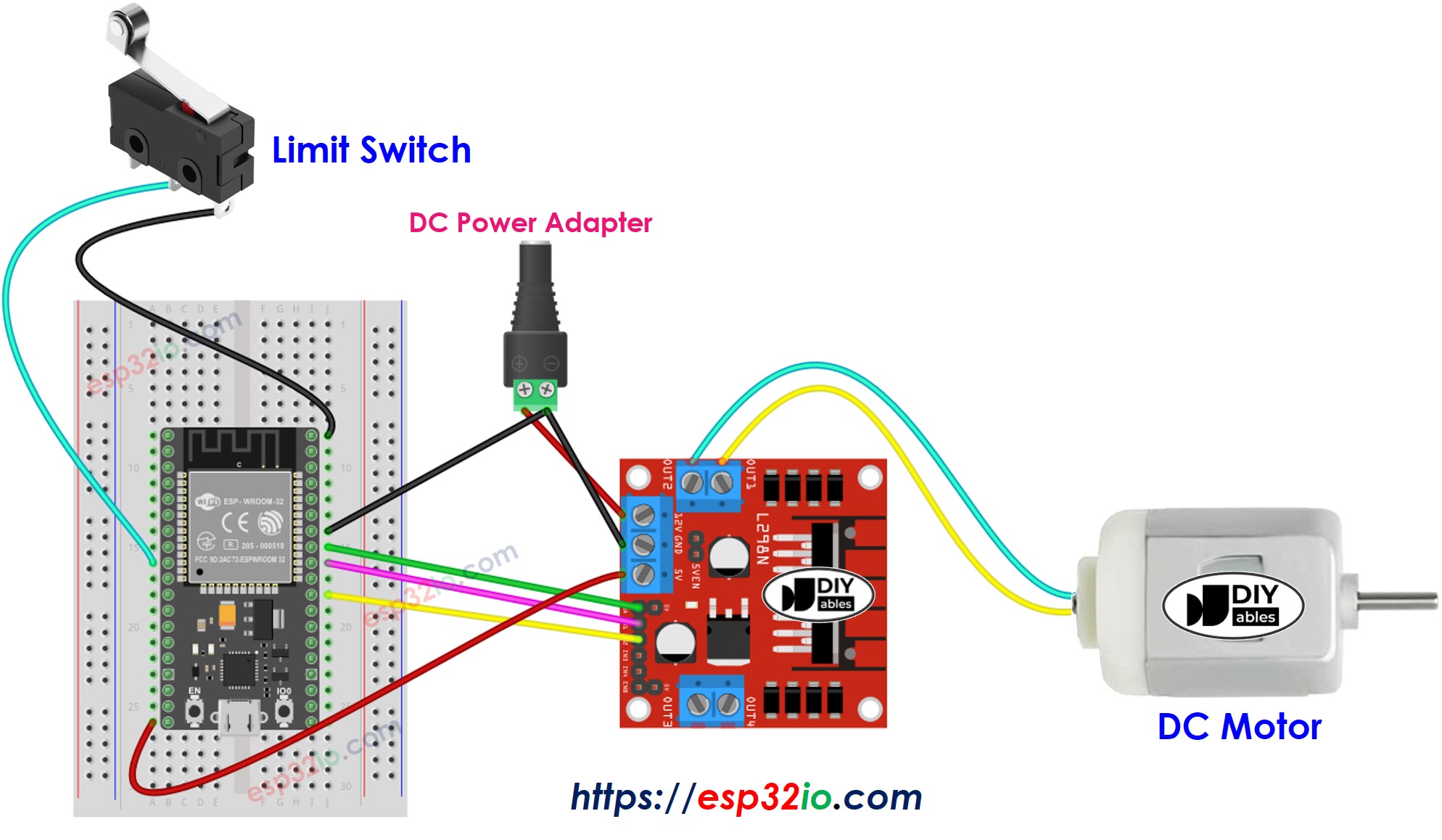
This image is created using Fritzing. Click to enlarge image
- Wiring diagram between the DC motor and two limit switches
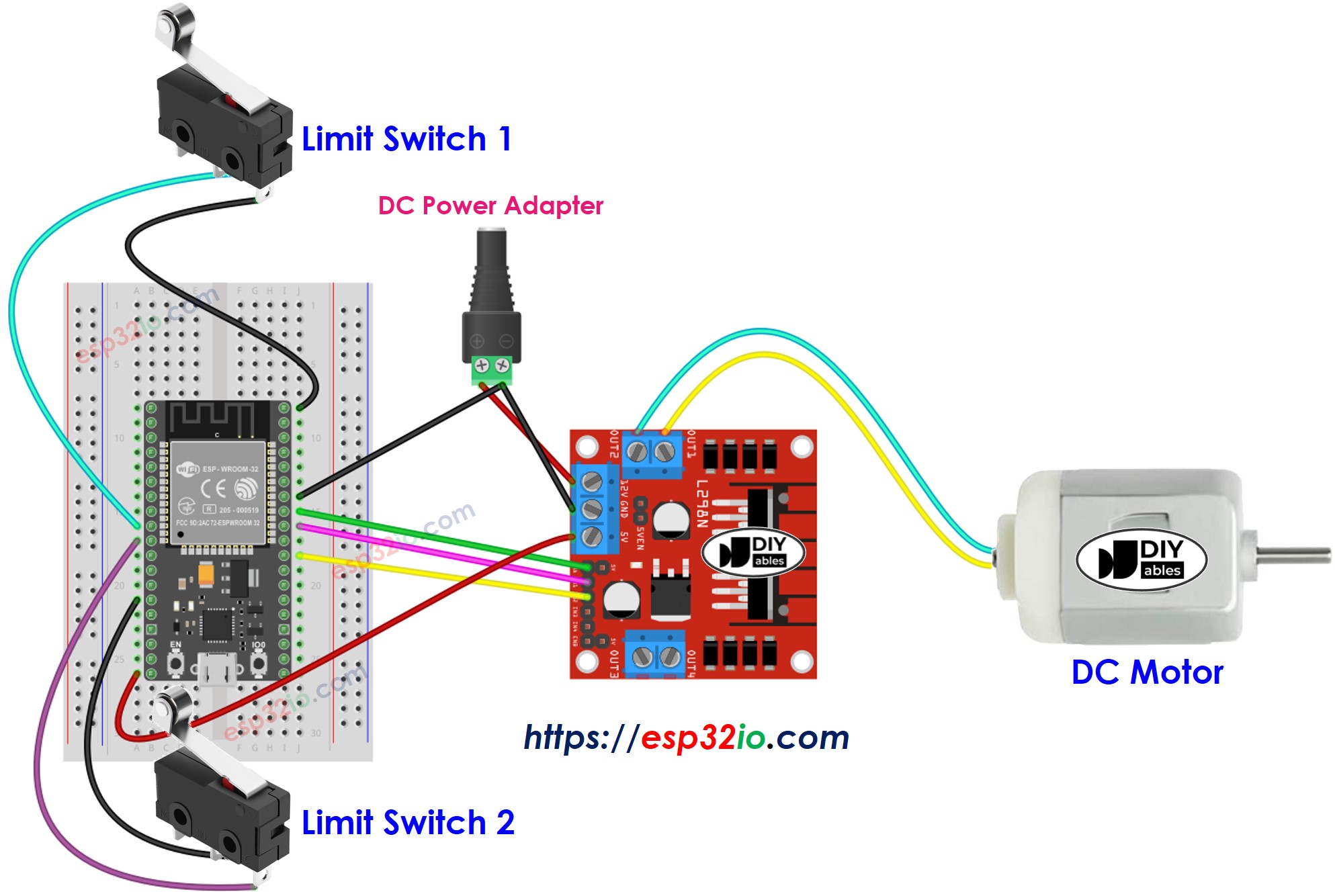
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
ESP32 Code - Stop DC Motor by a Limit Switch
The below code make a DC motor spin infinitely and stop immediately when a limit switch is touched
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Connect ESP32 to PC via USB cable
- Open Arduino IDE, select the right board and port
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search “ezButton”, then find the button library by ArduinoGetStarted.com
- Click Install button to install ezButton library.
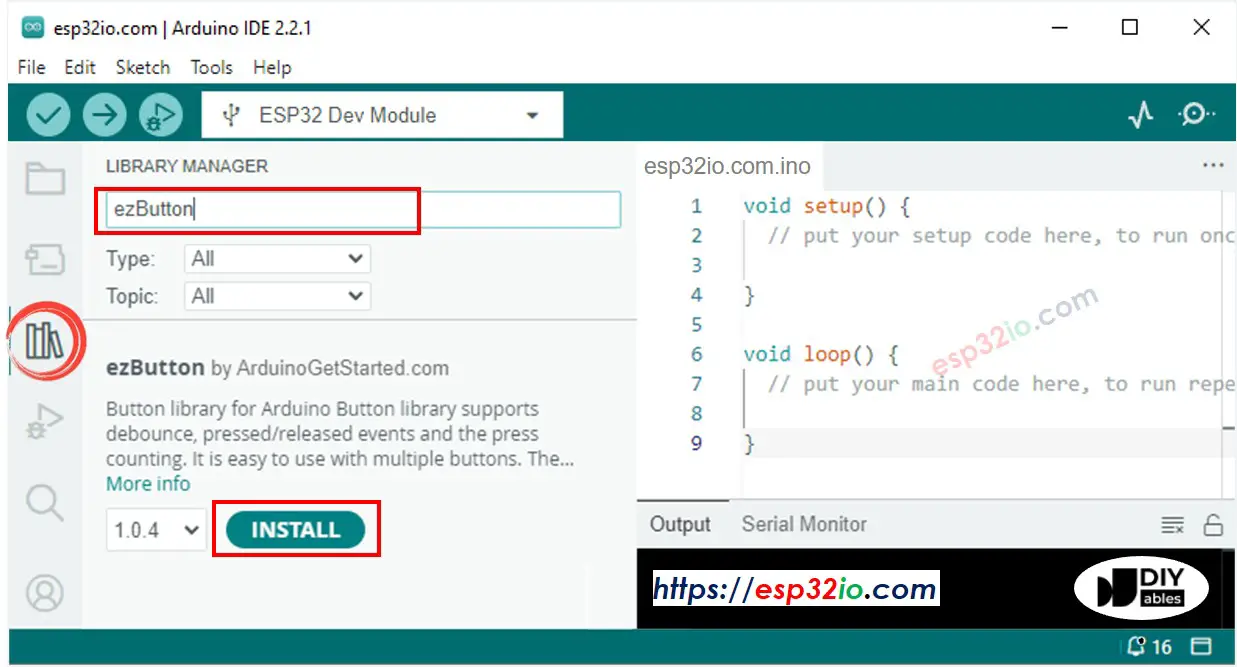
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
- If the wiring is correct, you will see the motor rotates clockwise direction.
- Touch the limit switch
- You will see the motor is stopped immediately
- The result on Serial Monitor looks like below
Code Explanation
Read the line-by-line explanation in comment lines of code!
ESP32 Code - Change Direction of DC Motor by a Limit Switch
The below code make a DC motor spin infinitely and change its direction when a limit switch is touched
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
- If the wiring is correct, you will see the motor spins in the clockwise direction.
- Touch the limit switch
- You will see the DC motor's direction is changed to the anti-clockwise
- Touch the limit switch again
- You will see the DC motor's direction is changed to clockwise
- The result on Serial Monitor looks like below
ESP32 Code - Change Direction of DC Motor by two Limit Switches
The below code make a DC motor spin infinitely and change its direction when one of two limit switches is touched
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
- If the wiring is correct, you will see the motor spins in the clockwise direction.
- Touch the limit switch 1
- You will see the DC motor's direction is changed to anti-clockwise
- Touch the limit switch 2
- You will see the DC motor's direction is changed to clockwise
- The result on Serial Monitor looks like below
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.