ESP32 - AWS IoT
This tutorial instructs you how to use ESP32 with AWS IoT Core. In detail, we will learn:
- How to connect the ESP32 to AWS IoT Core.
- How to program the ESP32 to send the data to AWS IoT Core.
- How to program the ESP32 to receive the data from AWS IoT Core.
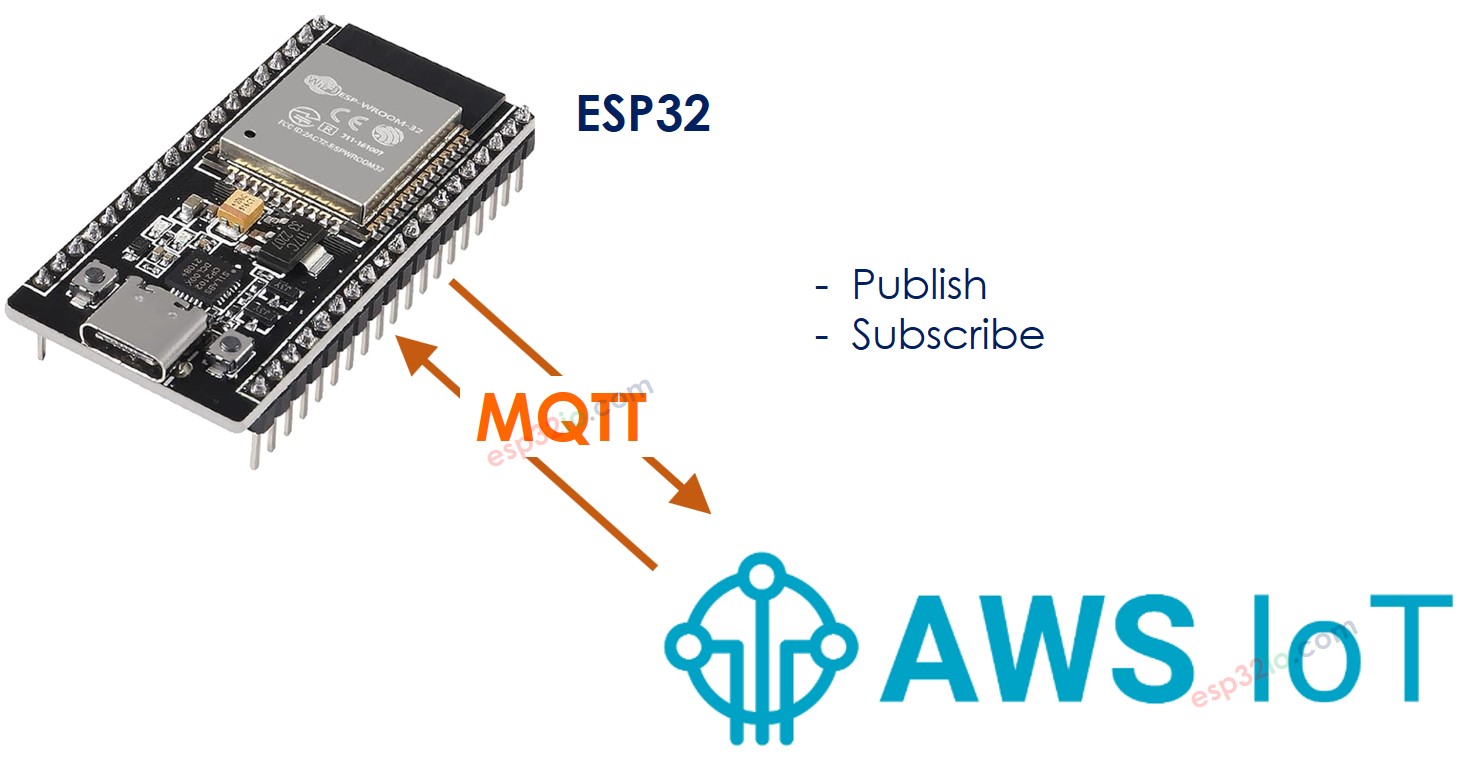
Hardware Used In This Tutorial
1 | × | ESP-WROOM-32 Dev Module | |
1 | × | USB Cable Type-C | |
1 | × | Breadboard | |
1 | × | Jumper Wires | |
1 | × | (Recommended) ESP32 Screw Terminal Adapter |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to ESP32 and AWS IoT
The ESP32 connects to AWS IoT Core using the MQTT protocol. While libraries are available to facilitate this connection, it's not as straightforward as connecting to a local MQTT broker like Mosquitto on your PC. This is because AWS IoT Core has strict security measures in place, requiring configuration steps to obtain authentication credentials and authorization before integrating them into the ESP32 code. In summary, the process involves two main steps:
- Step 1: Configuring AWS IoT Core - This involves setting up AWS IoT Core to generate the necessary authentication credentials, which will later be used in the ESP32 code.
- Step 2: Writing the ESP32 Code - Once the authentication credentials are obtained from AWS IoT Core, the next step is to write the ESP32 code, integrating the necessary authentication and communication protocols.
Let's take a closer look at each step to understand it better.
Configuring AWS IoT Core for use with ESP32
The objectives of this step include:
- Creating a representation of the ESP32 device on AWS IoT Core, referred to as a "Thing."
- Configuring the necessary authorization to allow the ESP32 device to connect, publish, and subscribe to/from AWS IoT Core, known as a "Policy."
- Generating the AWS credentials required for authentication, known as "Certificates." These credentials will be downloaded and integrated into the Arduino ESP32 code.
Below are instructions on configuring AWS IoT Core for use with ESP32 via the AWS IoT Console. Please note that while the User Interface may change over time, the process should remain similar to the instructions provided below:
- Sign in to the AWS IoT Console
- Create a Thing by going to Manage All devices Things
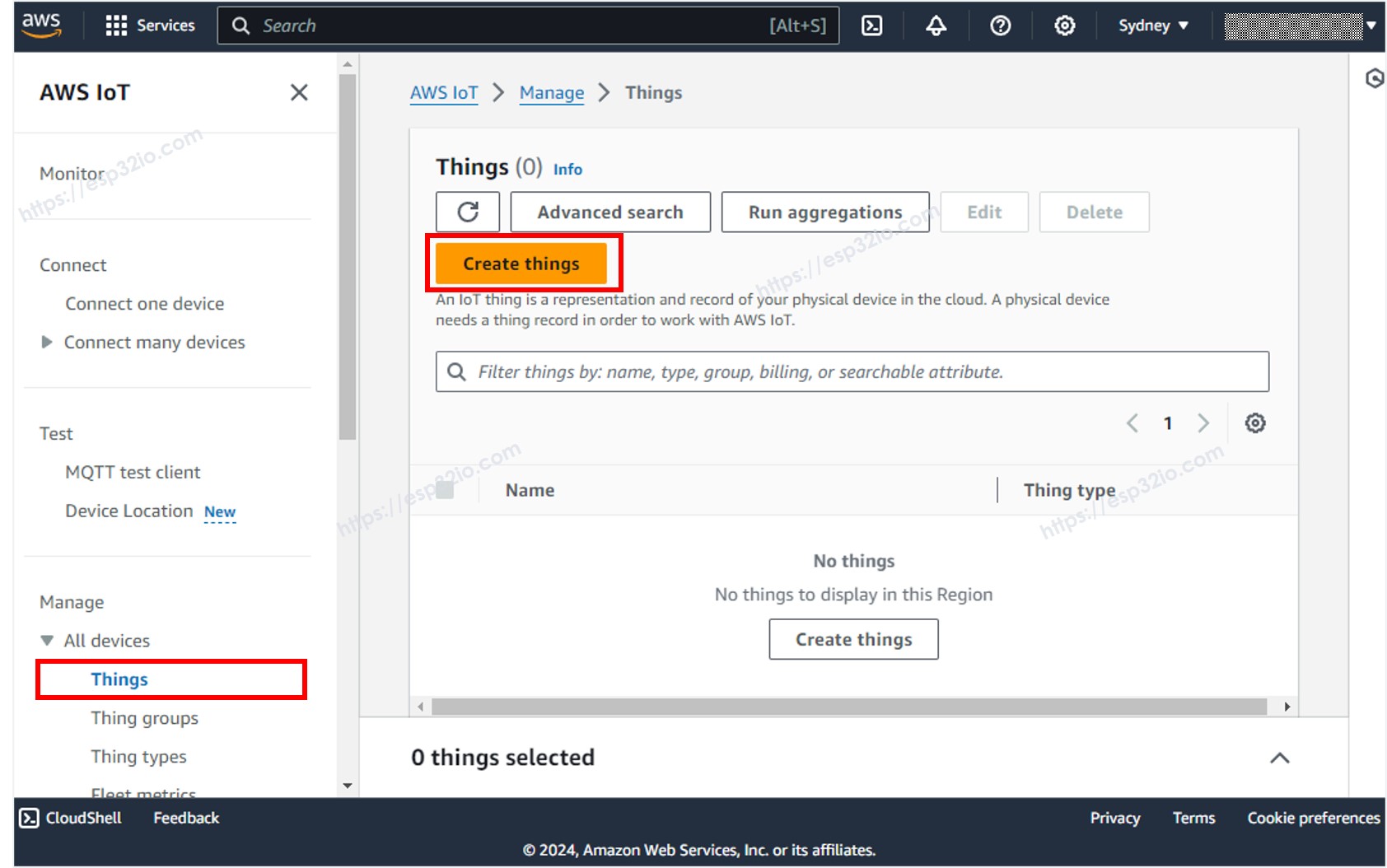
- Click the Create things button.
- Select Create single things and click the Next button.
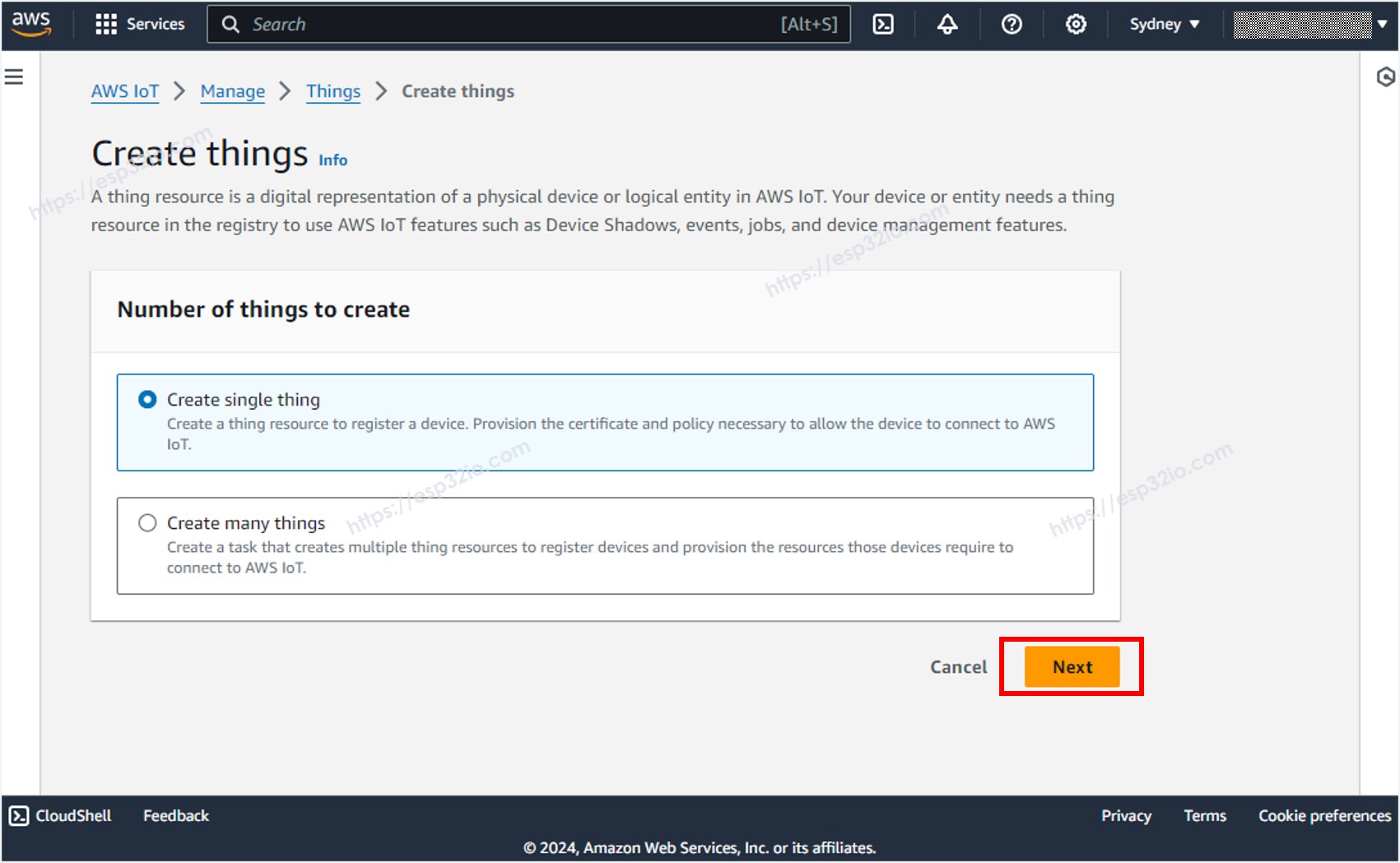
- Specify the Thing name, for example, ESP32-thing and click the Next button at the bottom of the page.
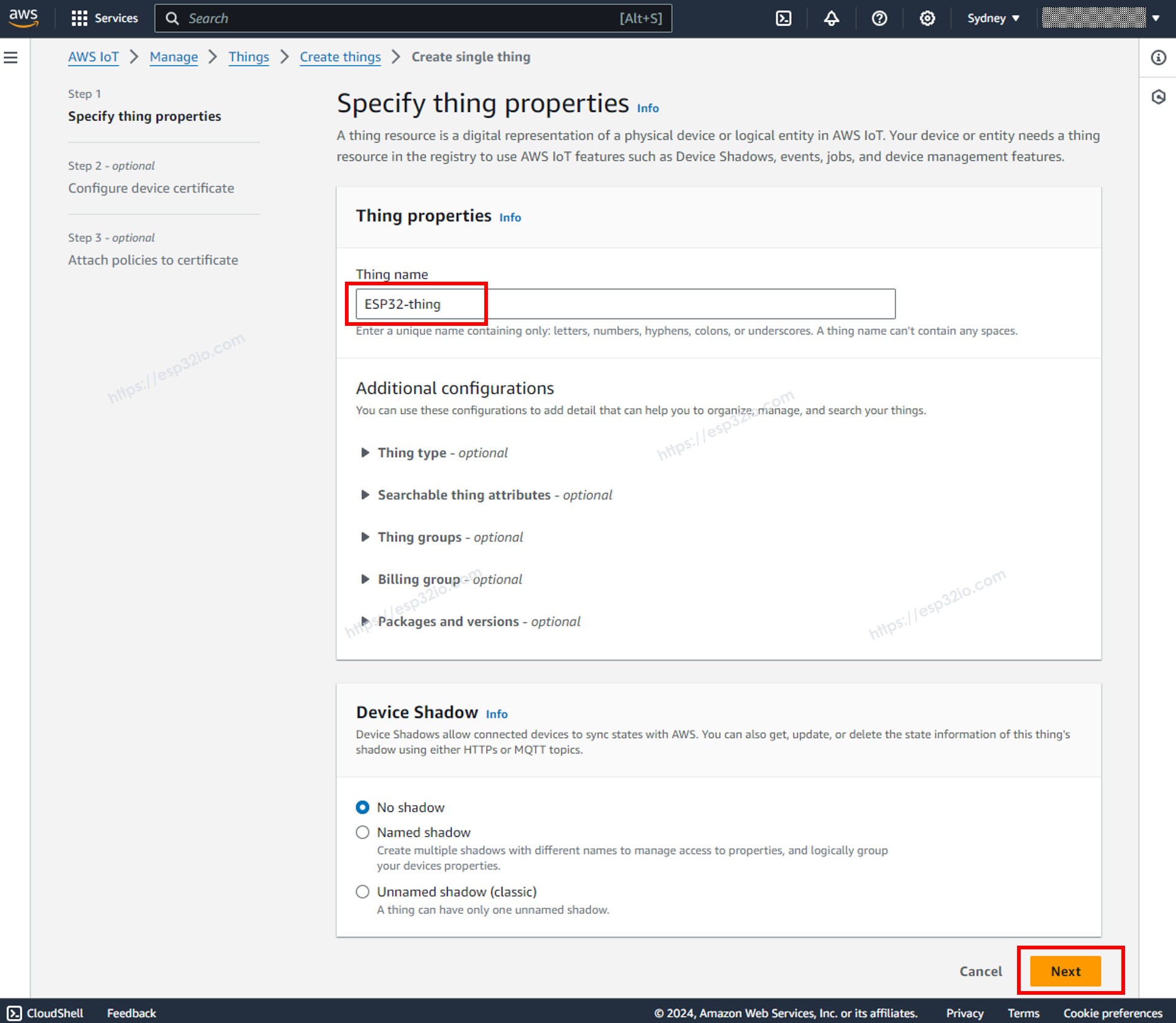
- Generate the credentials by selecting the Auto-generate a new certificate option, and click the Next button.
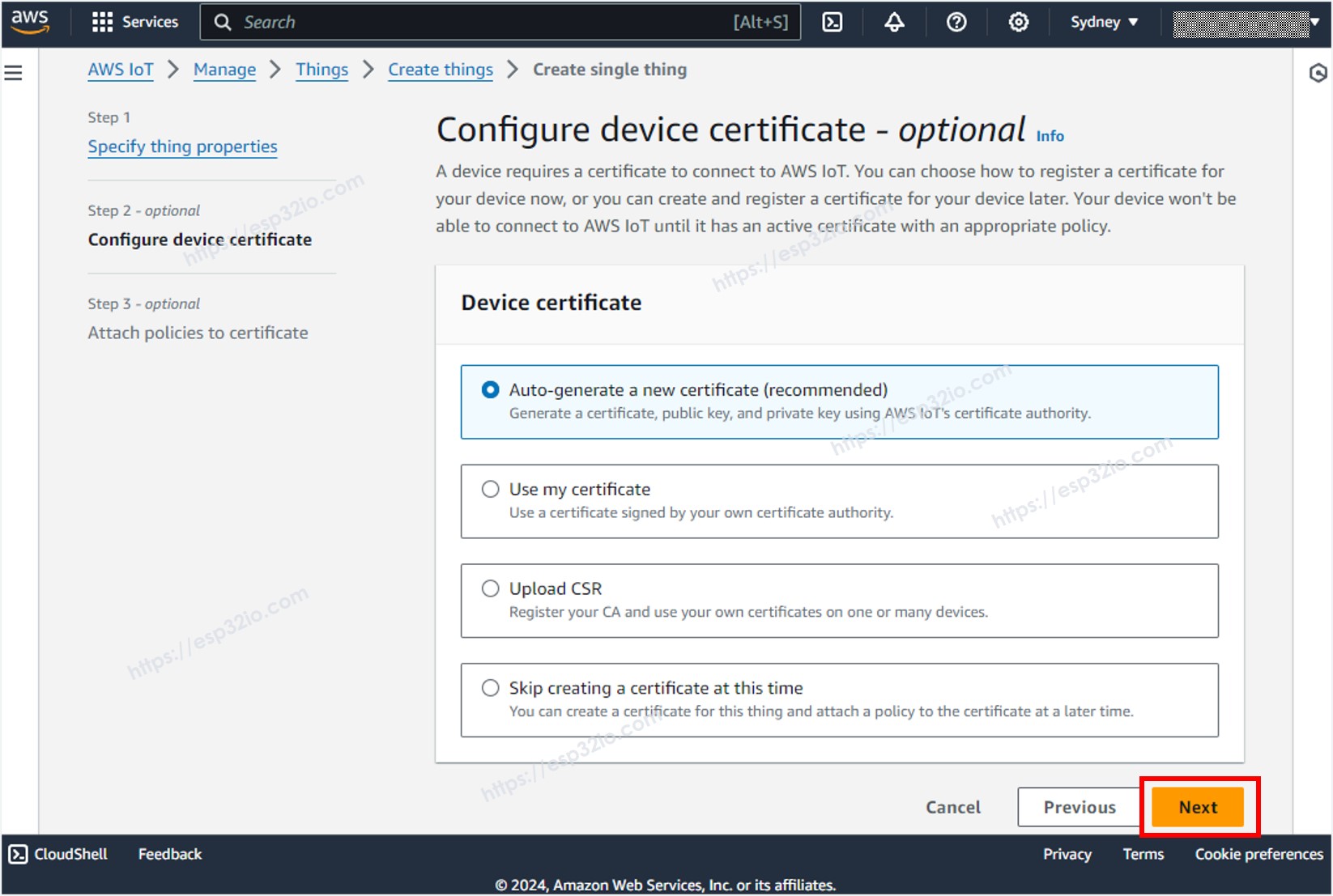
- Now, a Certificate is created and linked to the Thing.
- Create a policy by clicking the Create policy button.
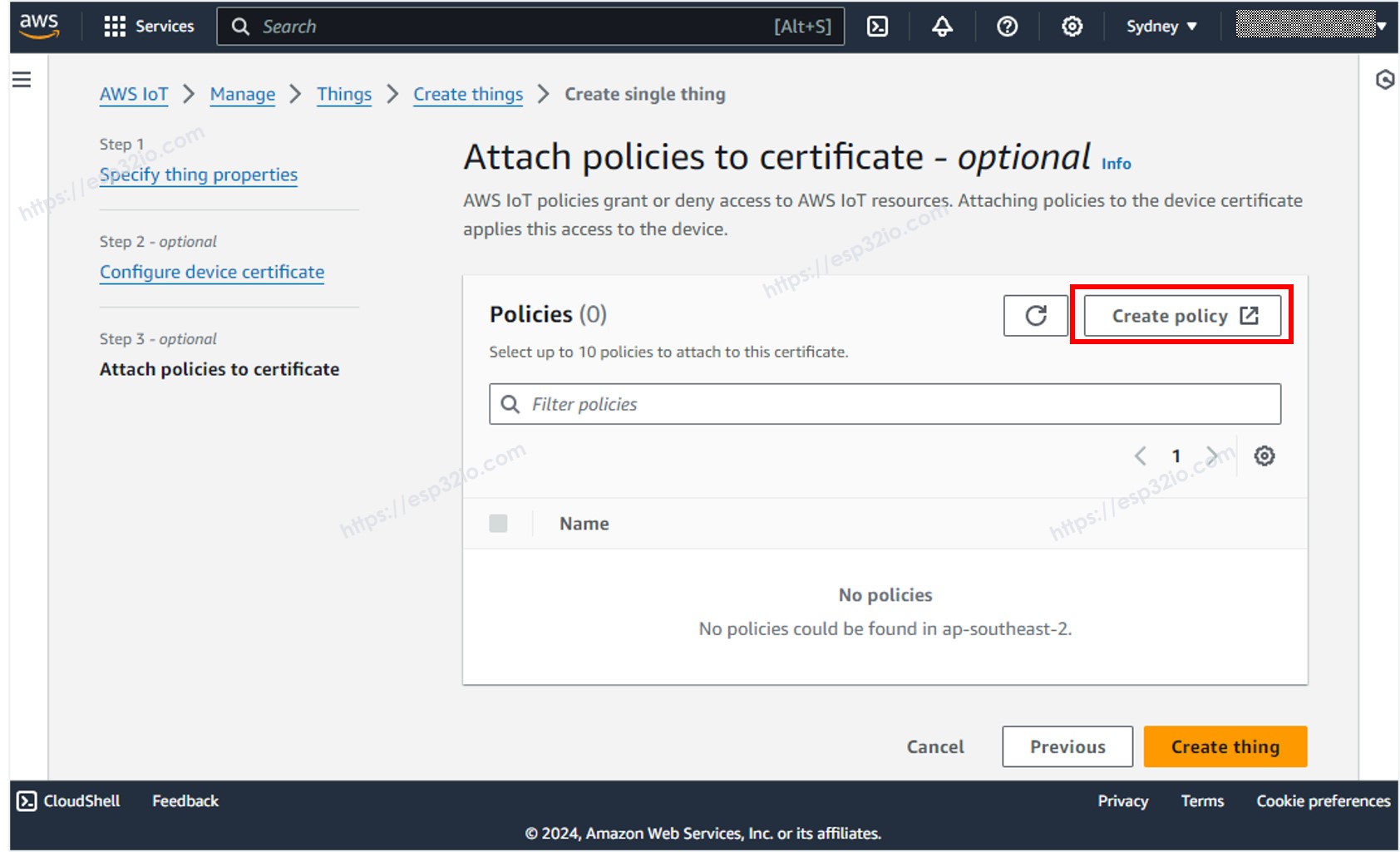
- A new tab will be opened
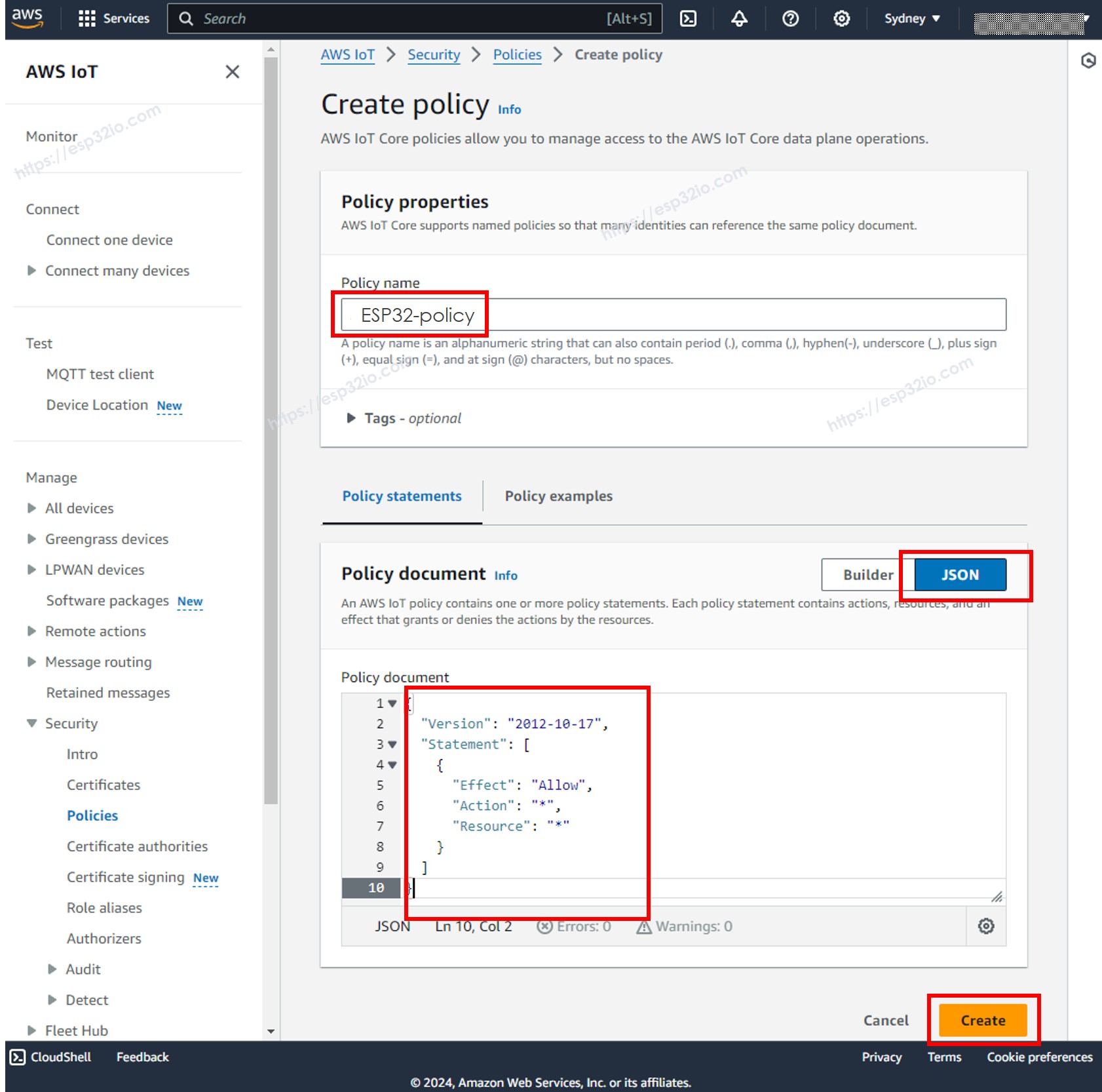
- Specify the Policy name, for example, ESP32-policy and click the JSON button.
- Copy the below JSON policy content and paste it to the Policy document area:
- Click the Create button at the bottom of the page to create the policy.
- Now, a Policy is created and attached to the Certificate. Close that page and back to the Thing page.
- Check the the ESP32-policy and Click the Create thing button to create the Thing.
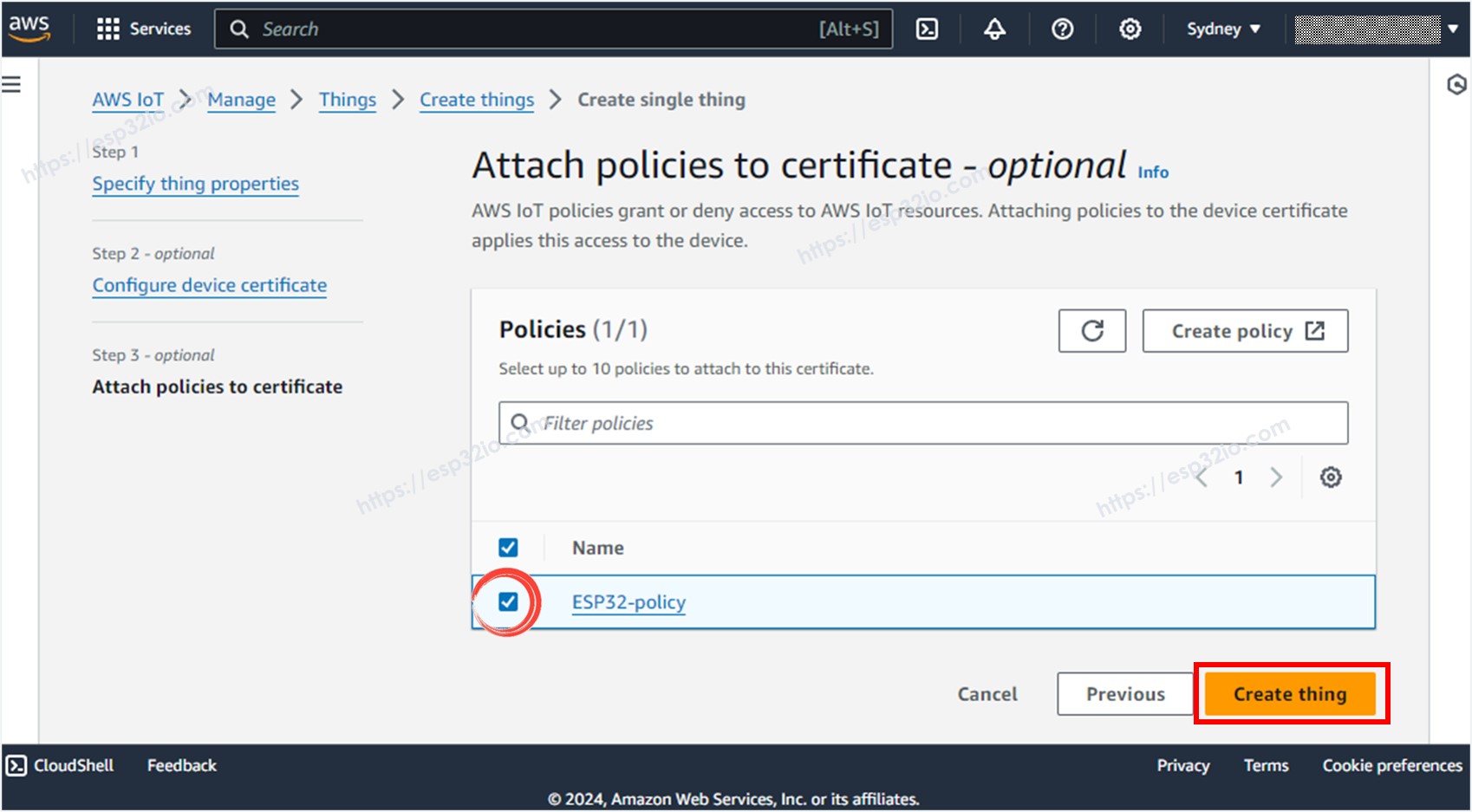
- A popup window appears that allows you to download the credentials files. Download all files and store them in a safe location on your PC and keep them confidentially.
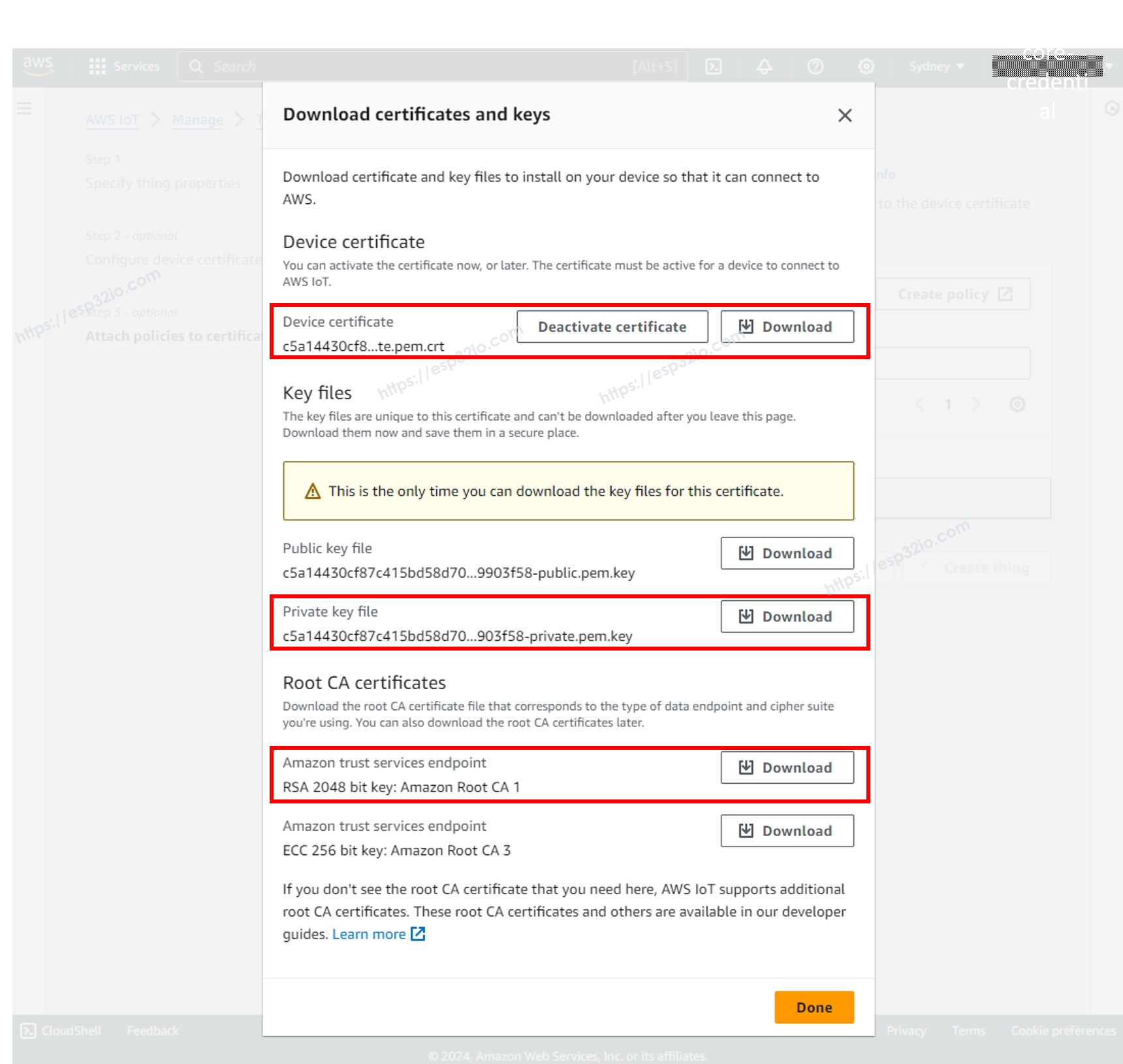
- Then, click the Done button.
Among downloaded files, there are three files will be used in the ESP32 code in the next steps:
- AmazonRootCA1.pem
- xxxxxxxxxx-certificate.pem.crt
- xxxxxxxxxx-private.pem.key
These files can be opened by any text editor such as Notepad, or Notepad++
Writing the ESP32 code to connect to AWS IoT Core
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Open the Library Manager by clicking on the Library Manager icon on the left navigation bar of Arduino IDE
- Type MQTT on the search box, then look for the MQTT library by Joel Gaehwiler.
- Click Install button to install MQTT library.
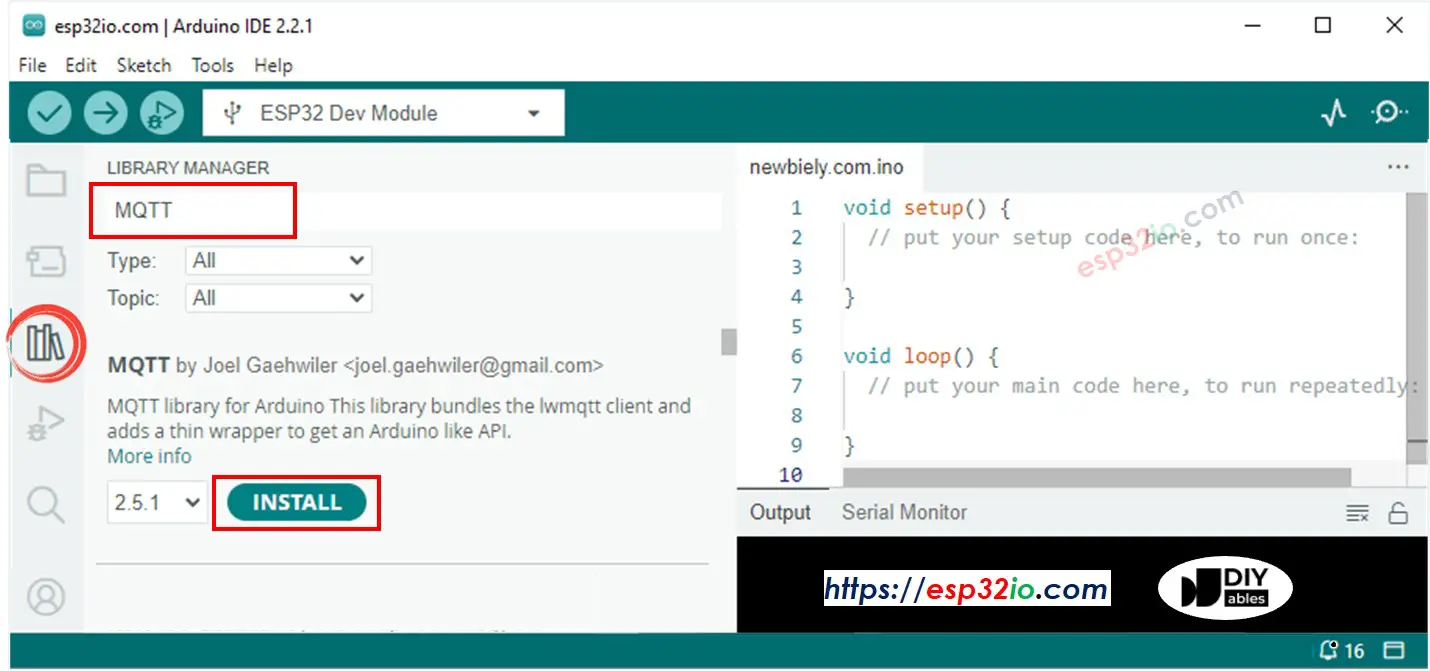
- Type ArduinoJson on the search box, then look for the ArduinoJson library by Benoit Blanchon.
- Click Install button to install ArduinoJson library.
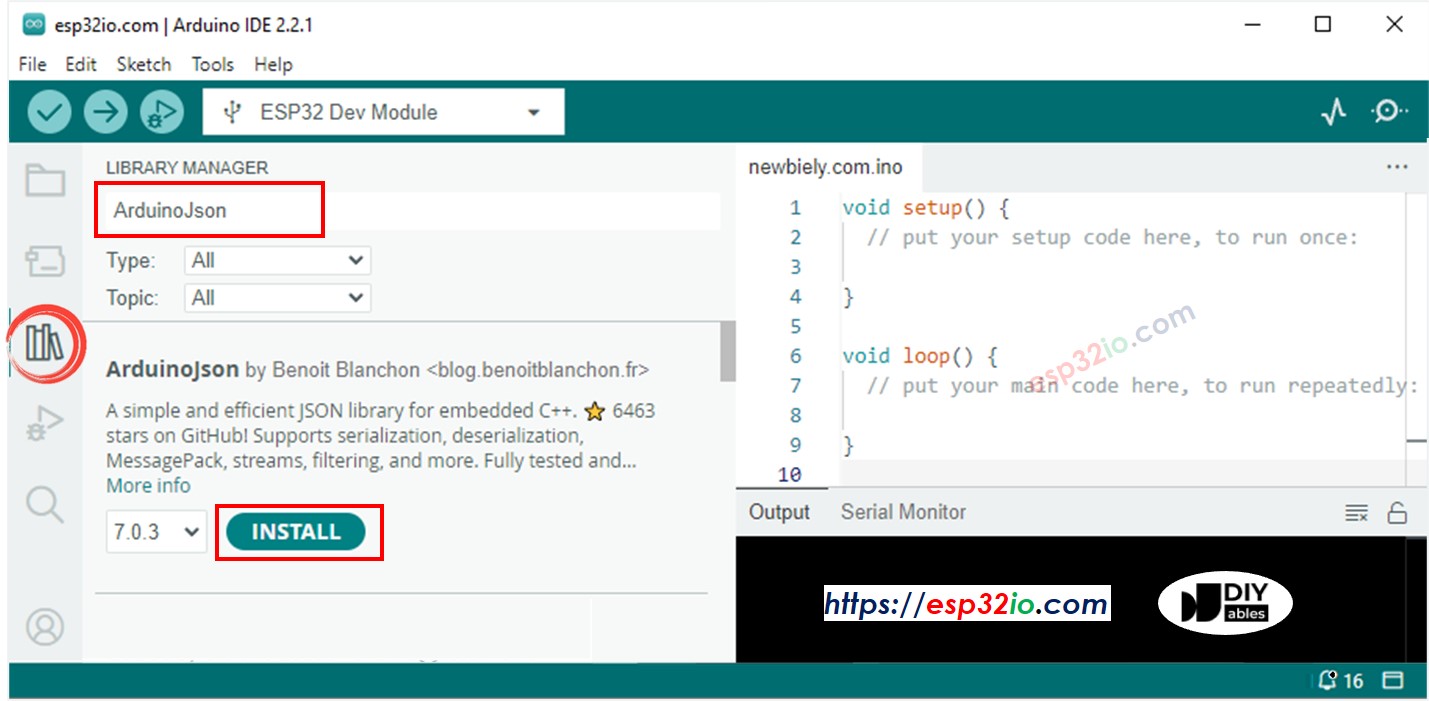
- Copy the above code and paste it to Arduino IDE.
- Create the secrets.h file On Arduino IDE by:
- Either click on the button just below the serial monitor icon and choose New Tab, or use Ctrl+Shift+N keys.
- Give file's name secrets.h and click OK button
- Copy the below code and paste it to the created secrets.h file.
- Update the following information in the secrets.h
- The WIFI_SSID and WIFI_PASSWORD of your WiFi network
- The AWS_CERT_CA, AWS_CERT_CRT, and AWS_CERT_PRIVATE. These infornation are in the files you downloaded in the previous step.
- The AWS_IOT_ENDPOINT. This information can be found on AWS IoT Console by going to Setting as below image:
- Compile and upload code to ESP32 board by clicking Upload button on Arduino IDE
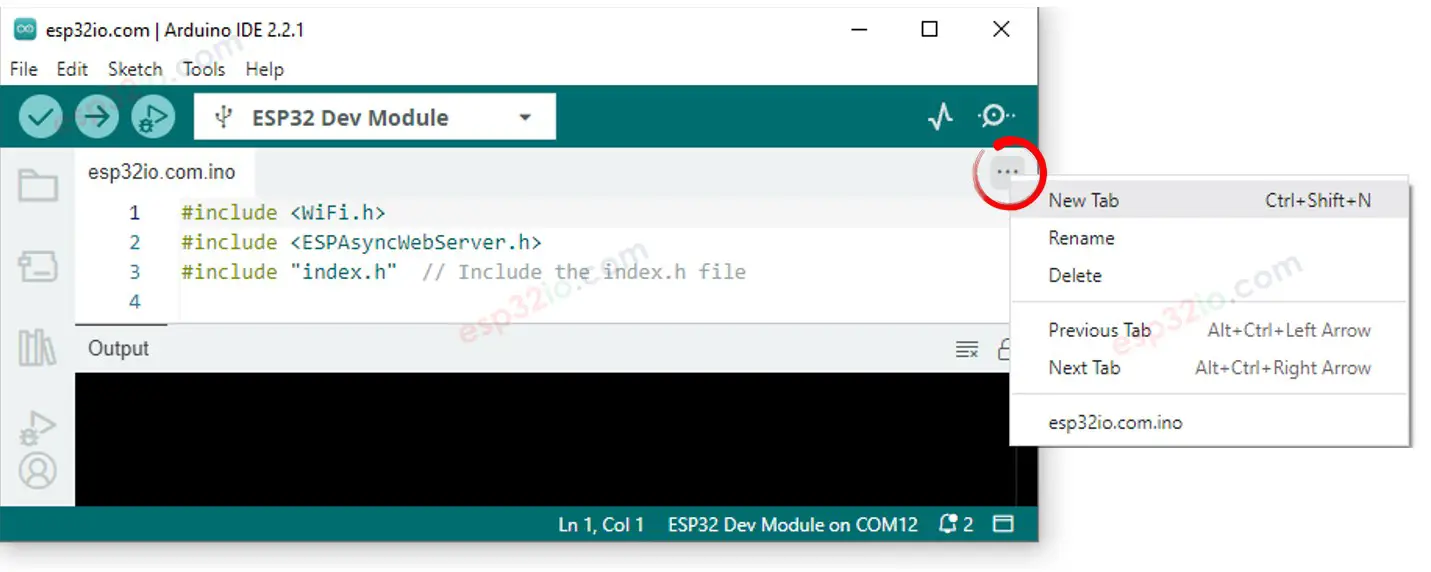
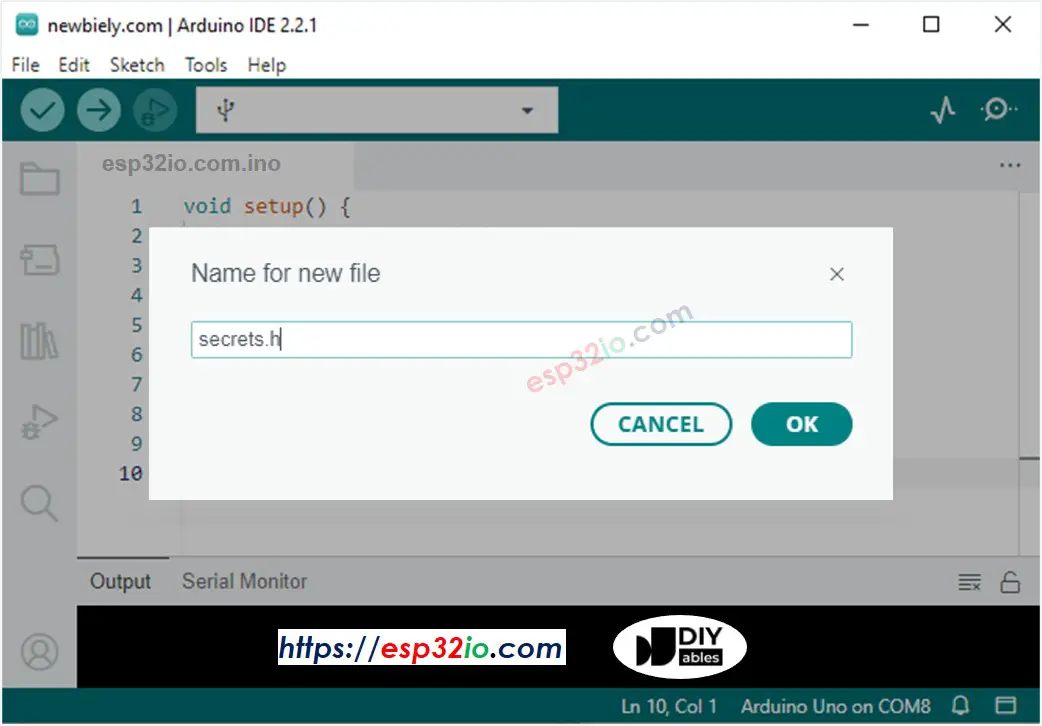
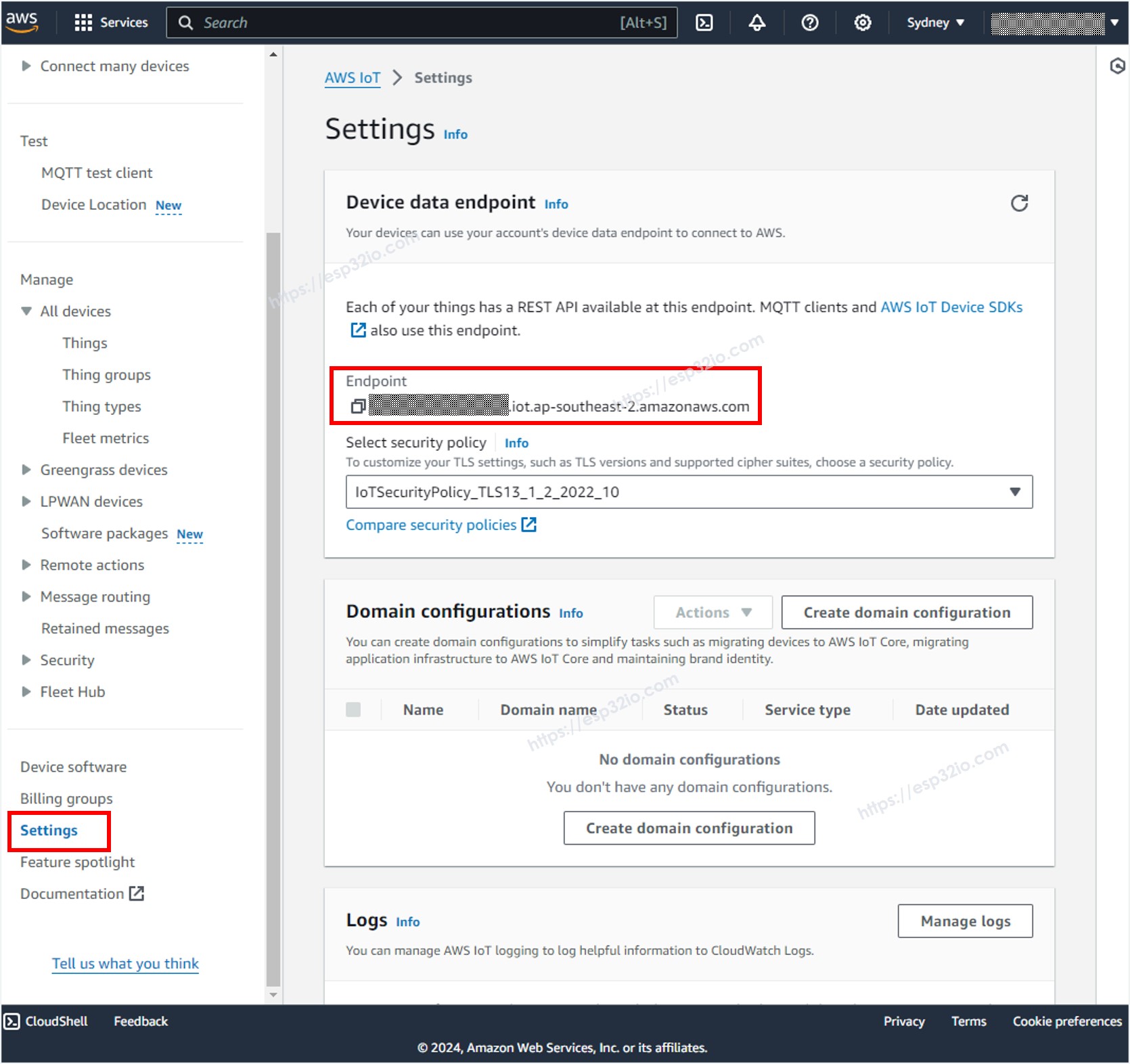
Sending data from ESP32 to AWS IoT
The above ESP32 code periodically read data from an analog pin and send it to AWS IoT every 4 seconds. If opening the Serial Monitor on Arduino IDE, you will see the log like below:
To check if the data are received by AWS IoT or not, do the following steps:
- In AWS IoT Console, navigate to Test MQTT Test Client
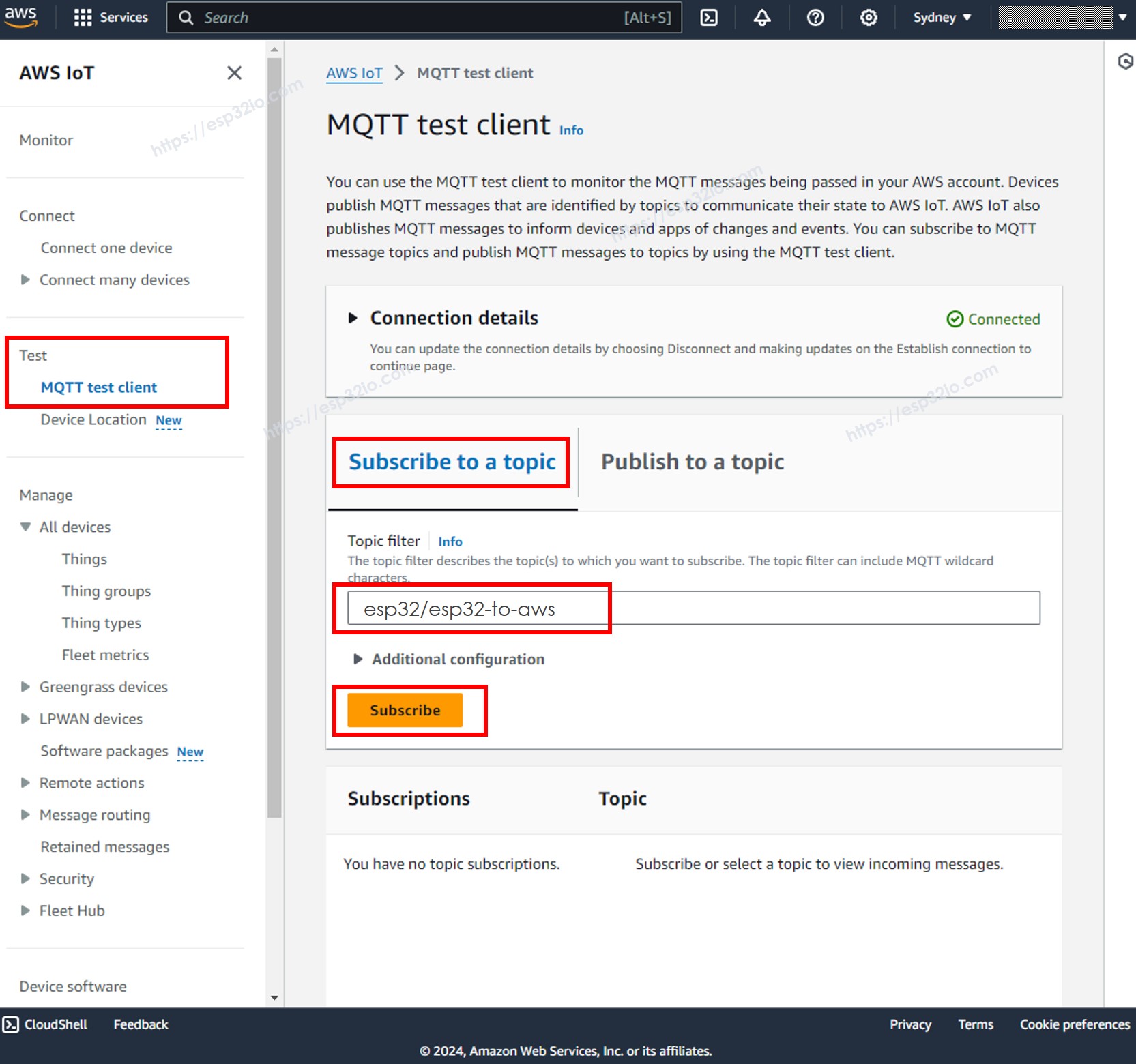
- Click the Subcribe to a topic button.
- Type esp32/esp32-to-aws to the Topic filter. You can change the topic but MUST be matched the topic on the ESP32 code.
- Click the Subcribe button.
- You will be able to see the data sent from ESP32 on the AWS IoT Console.
Sending data from AWS IoT to ESP32
You are able to send the data from AWS IoT Console to the ESP32 by doing the following steps:
- On Arduino IDE, Open the Serial Monitor
- On AWS IoT Console, navigate to Test MQTT Test Client
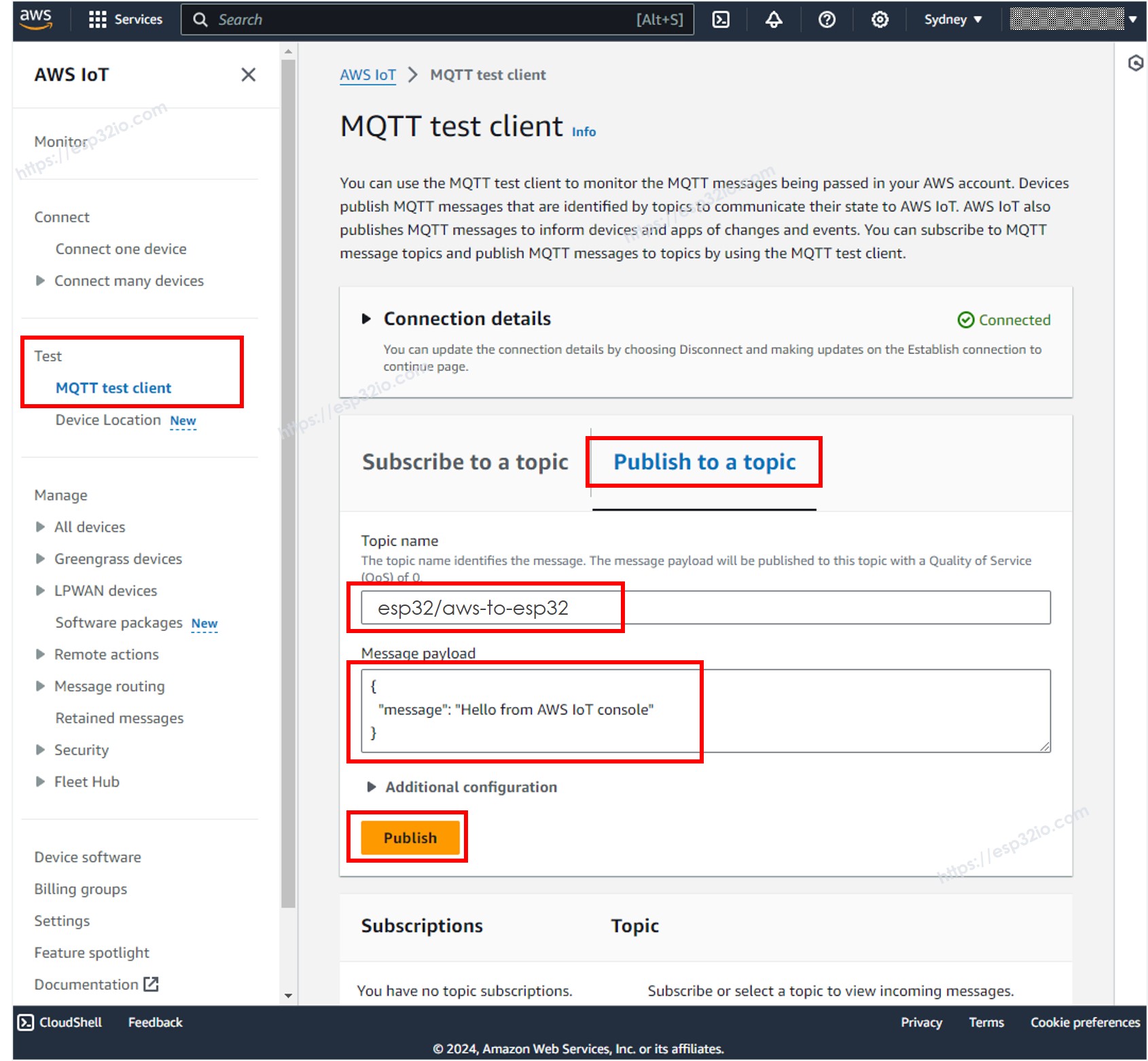
- Click the Publish to a topic button.
- Type esp32/aws-to-esp32 to the Topic name. You can change the topic but MUST be matched the topic on the ESP32 code.
- Optionally, you can change the message payload, or just keep it as default.
- Click the Publish button.
- Check out the Serial Monitor on Arduino IDE, you will see the message sent from AWS IoT Console.
Do more with AWS
Now, you can establish bidirectional communication between the ESP32 and AWS IoT Core. This means you can send data from the ESP32 to AWS IoT Core and receive data from AWS IoT Core on the ESP32. Additionally, you have the capability to configure IoTRules, enabling the ESP32 to seamlessly connect with other AWS services such as Lambda, DynamoDB, Amplify, and RDS. With IoTRules, you can automate actions based on data received from the ESP32, enabling a wide range of IoT applications and integrations.