ESP32 - Log Data with Timestamp to SD Card
In this guide, we'll explore the process of logging data with timestamps to a Micro SD Card using ESP32. Specifically, we'll cover the following topics:
- Logging data with timestamps to a single file on a Micro SD Card using ESP32.
- Logging data with timestamps to multiple files on a Micro SD Card, with one file dedicated to each day.
The time information is extracted from an RTC module and is then recorded onto the Micro SD Card alongside the collected data.
The data logged onto the Micro SD Card can encompass a variety of information, such as:
- Data readings from any sensor.
- A log of access to a door lock.
For simplicity, this tutorial will demonstrate the process by reading values from two analog pins, serving as an example dataset. The provided code can be easily adapted to accommodate different types of data as needed.
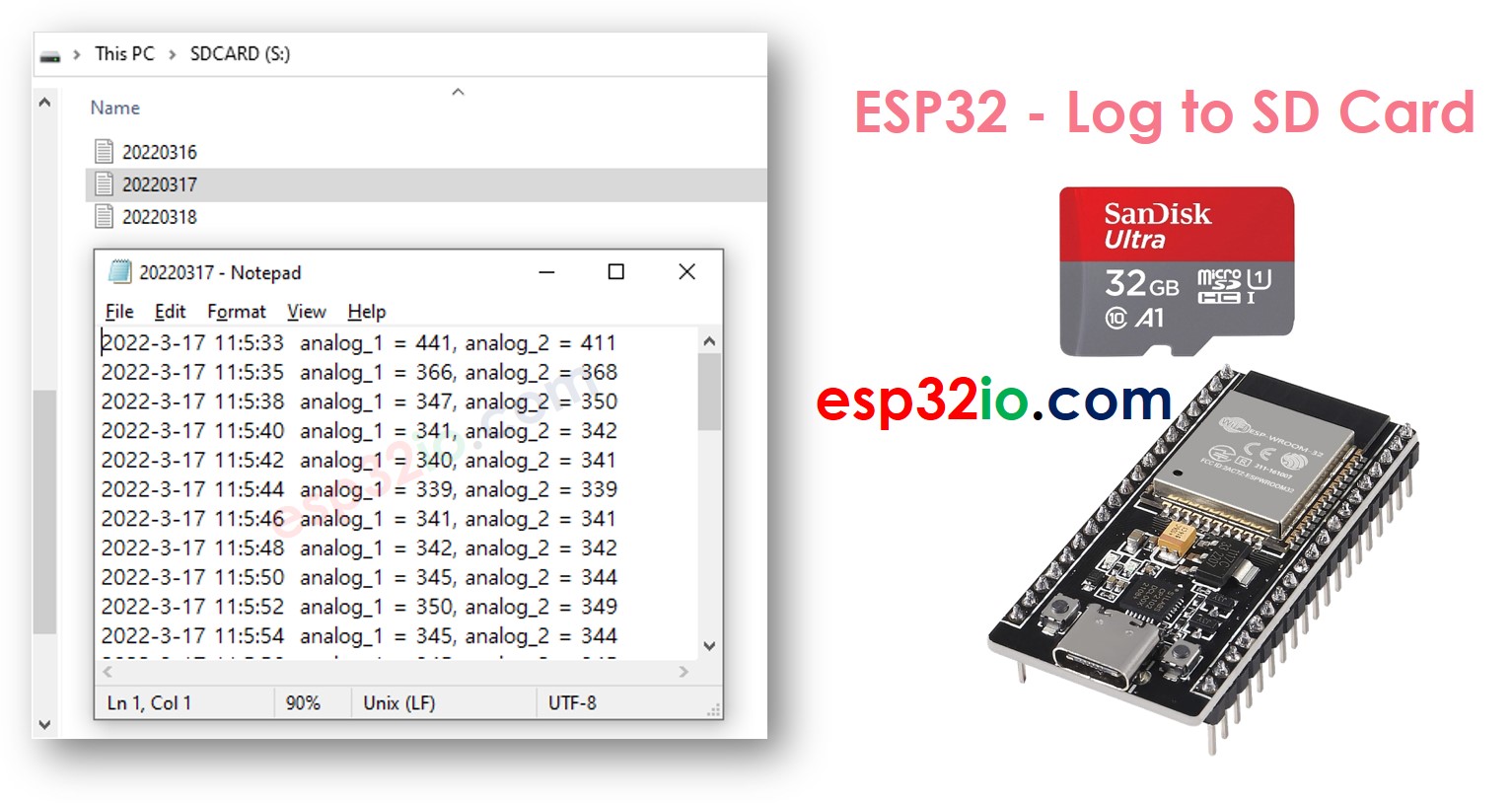
Hardware Used In This Tutorial
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to Micro SD Card Module and RTC Module
Unfamiliar with Micro SD Card Module and RTC module, including their pinouts, functionality, and programming? Explore comprehensive tutorials on these topics below:
- ESP32 - Micro SD Card tutorial.
- ESP32 - Write Variable to SD Card tutorial.
- ESP32 - RTC module tutorial.
Wiring Diagram
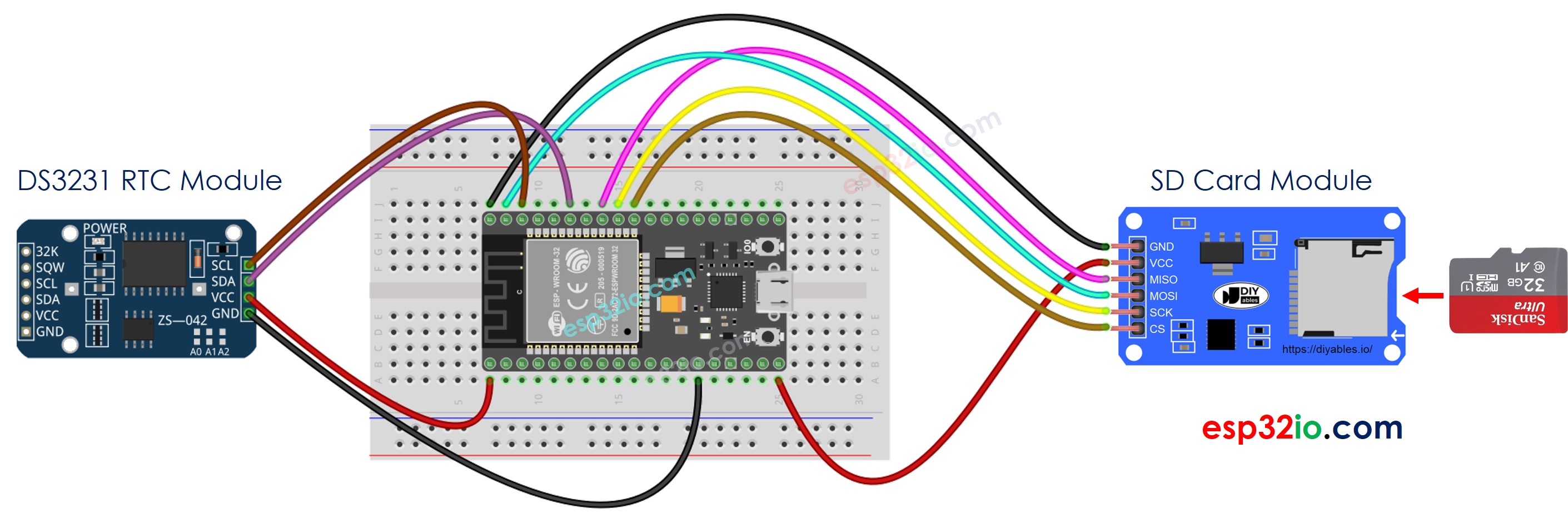
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: How to Power ESP32.
※ NOTE THAT:
If you use an Ethernet shield or any shield that has a Micro SD Card Holder, you do not need to use the Micro SD Card Module. You just need to insert the Micro SD Card to the Micro SD Card Holder on the shield.
ESP32 - Log Data with Timestamp to Micro SD Card
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Make sure that the Micro SD Card is formatted FAT16 or FAT32 (Google for it)
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
- See the result on Serial Monitor.
- Detach the Micro SD Card from the Micro SD Card module
- Insert the Micro SD Card to an USB SD Card reader
- Connect the USB SD Card reader to the PC
- Open the log.txt file on your PC, it looks like below
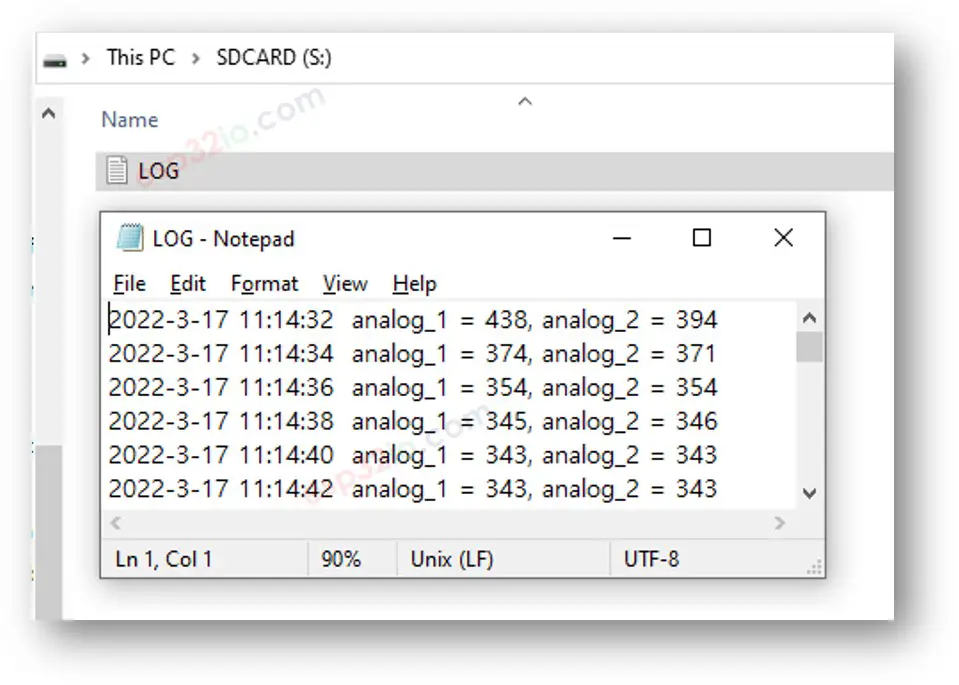
If you do not have an USB SD Card reader, you can check the content of log file by running the below ESP32 Code.
ESP32 - Log Data in multiple files
Writing log to a single file results in a big file size overtime and makes it difficult to check. The below code will write log file in multiple:
- One file per day
- The filename is the date informationL YYYYMMDD.txt
After a long run, If you:
- Detach the Micro SD Card from the Micro SD Card module
- Insert the Micro SD Card to an USB SD Card reader
- Connect the USB SD Card reader to the PC
- You will see the files as follows:
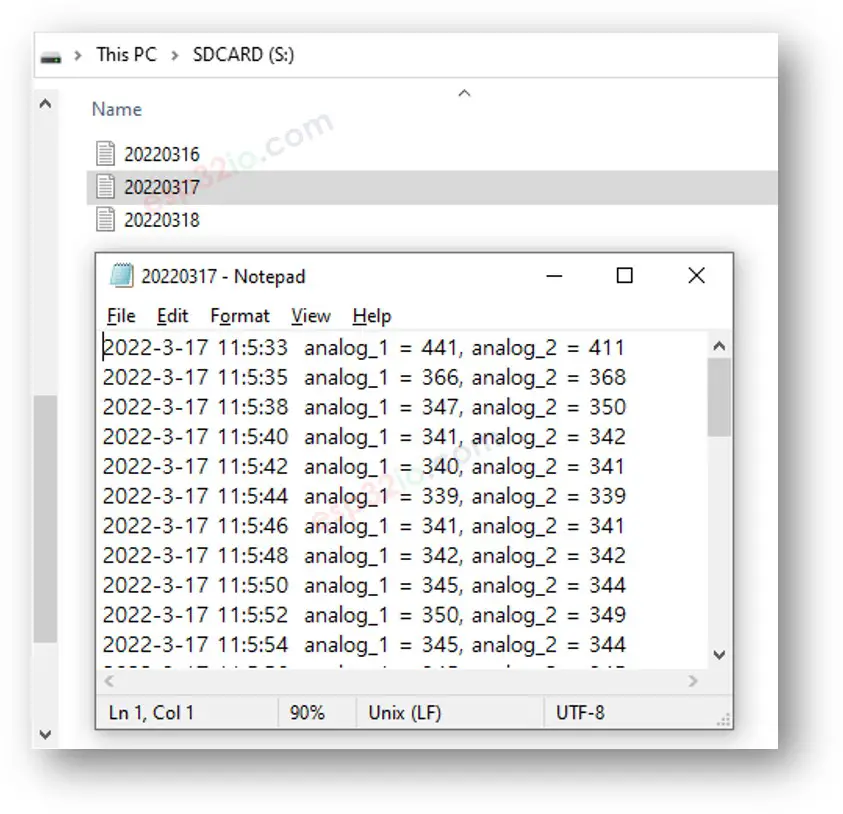
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.