ESP32 - Ultrasonic Sensor
This tutorial instructs you how to use ESP32 with the ultrasonic sensor HC-SR04 to measure the distance to an object.
This tutorial shows how to program the ESP32 using the Arduino language (C/C++) via the Arduino IDE. If you’d like to learn how to program the ESP32 with MicroPython, visit this ESP32 MicroPython - Ultrasonic Sensor tutorial.
Hardware Used In This Tutorial
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to Ultrasonic Sensor
The ultrasonic sensor HC-SR04 is used to measure the distance from the sensor to an object by using ultrasonic waves.
Ultrasonic Sensor Pinout
The ultrasonic sensor HC-SR04 includes four pins:
- VCC pin: connect this pin to VCC (5V)
- GND pin: connect this pin to GND (0V)
- TRIG pin: this pin receives a control pulse from ESP32.
- ECHO pin: this pin generates a pulse corresponding to the measured distance to ESP32.
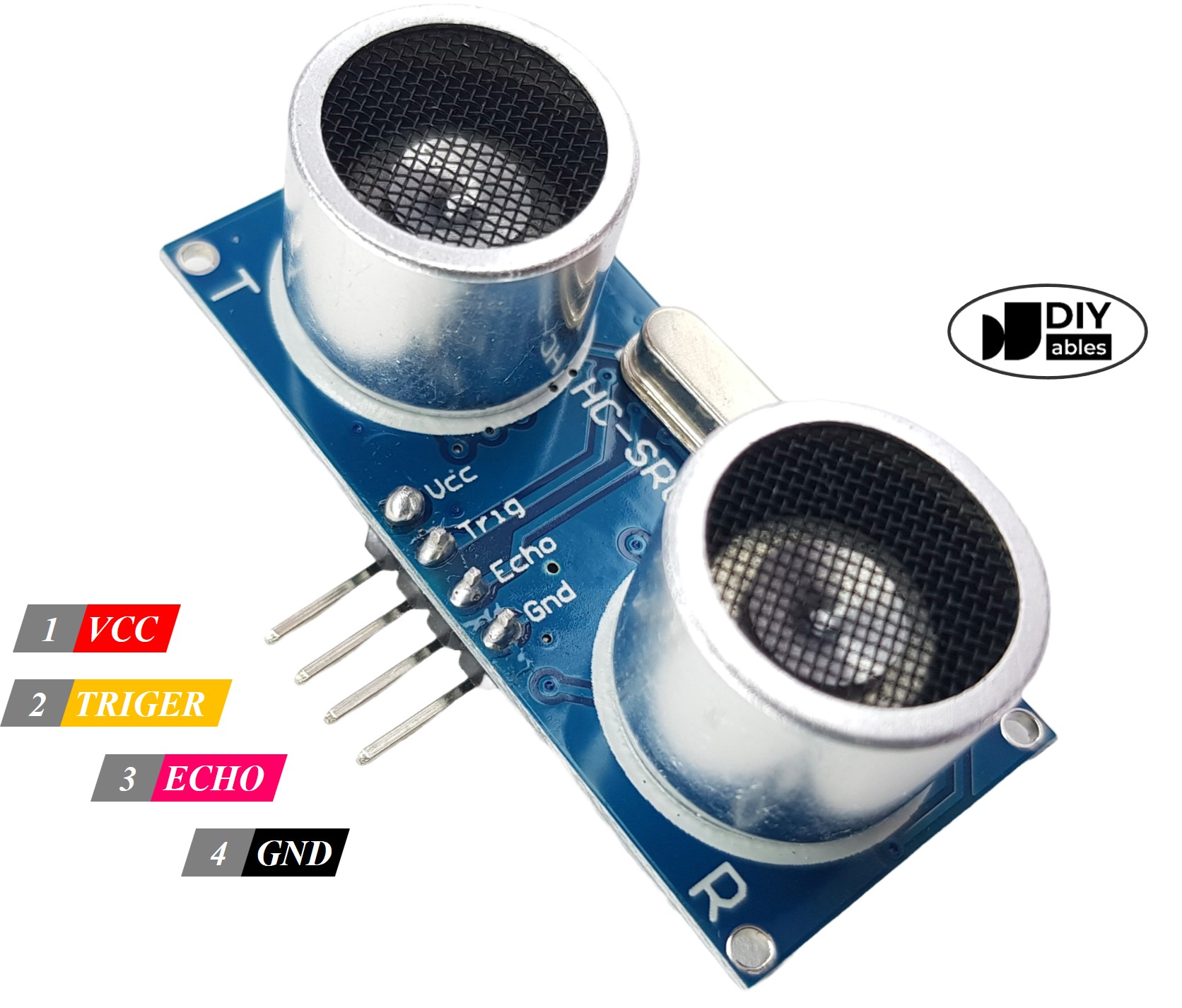
How Ultrasonic Sensor Works
Wiring Diagram between Ultrasonic Sensor and ESP32
- How to connect ESP32 and ultrasonic sensor using breadboard (powered via USB cable)
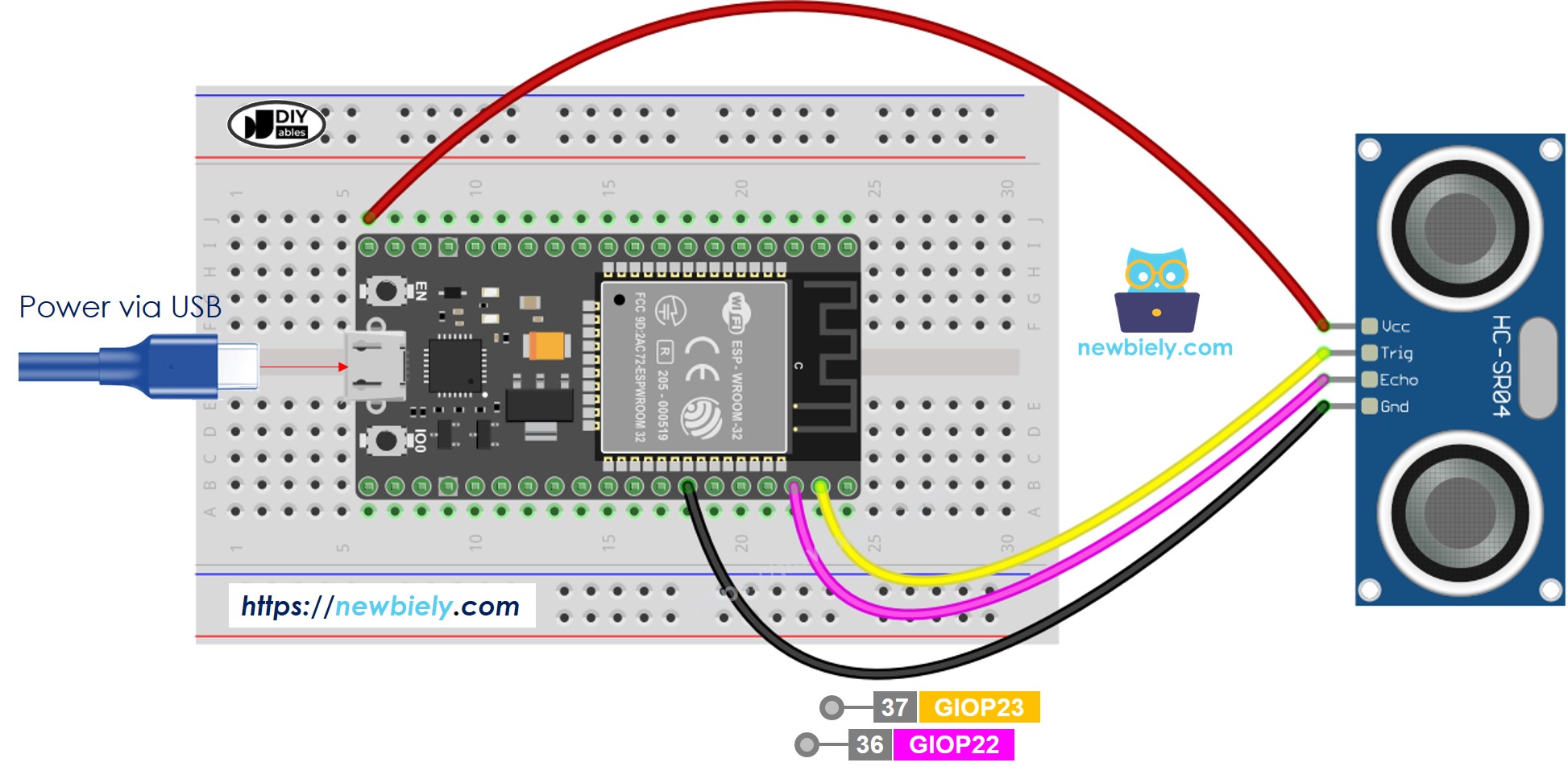
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and ultrasonic sensor using breadboard (powered via Vin pin)
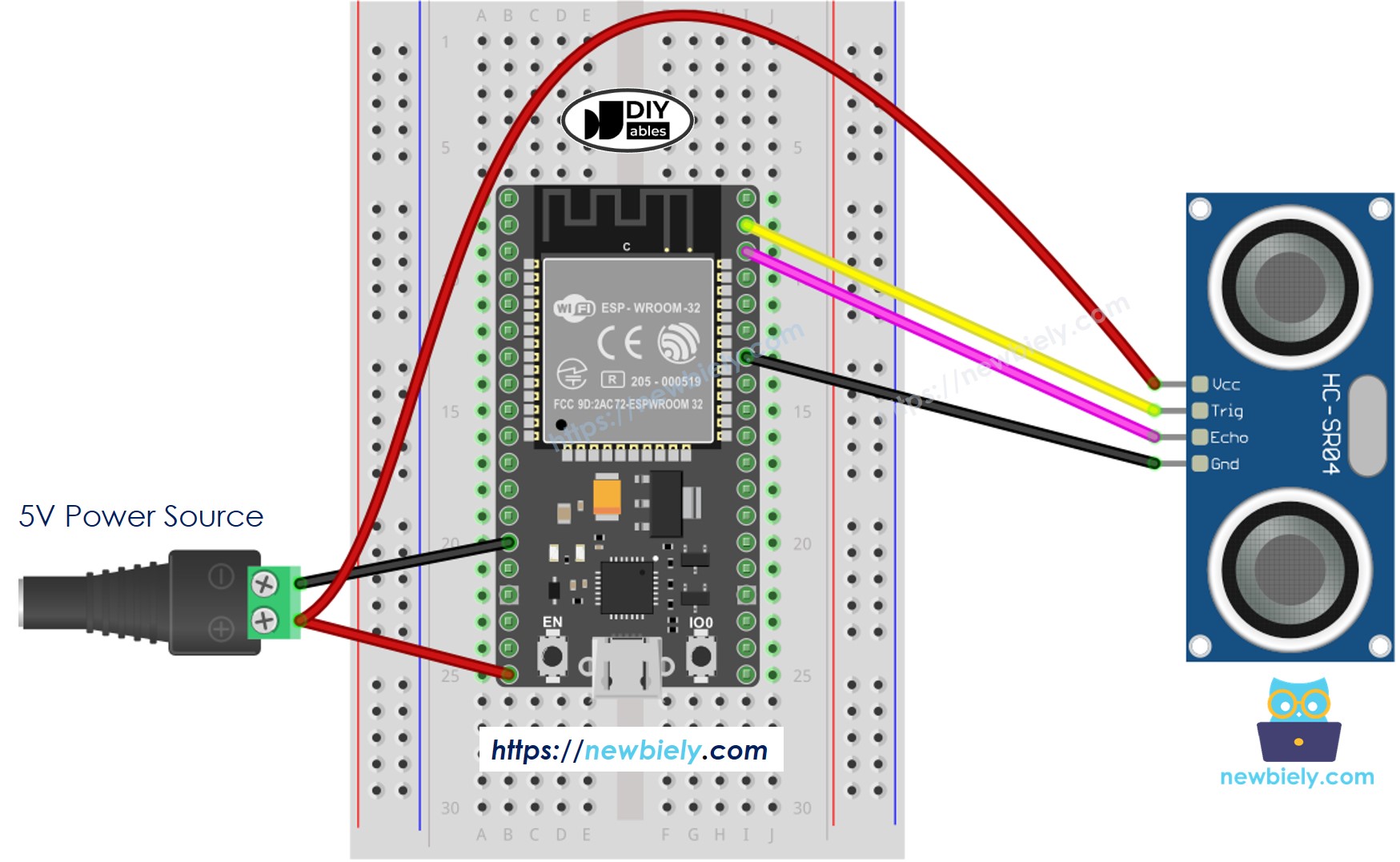
- How to connect ESP32 and ultrasonic sensor using screw terminal block breakout board (powered via USB cable)
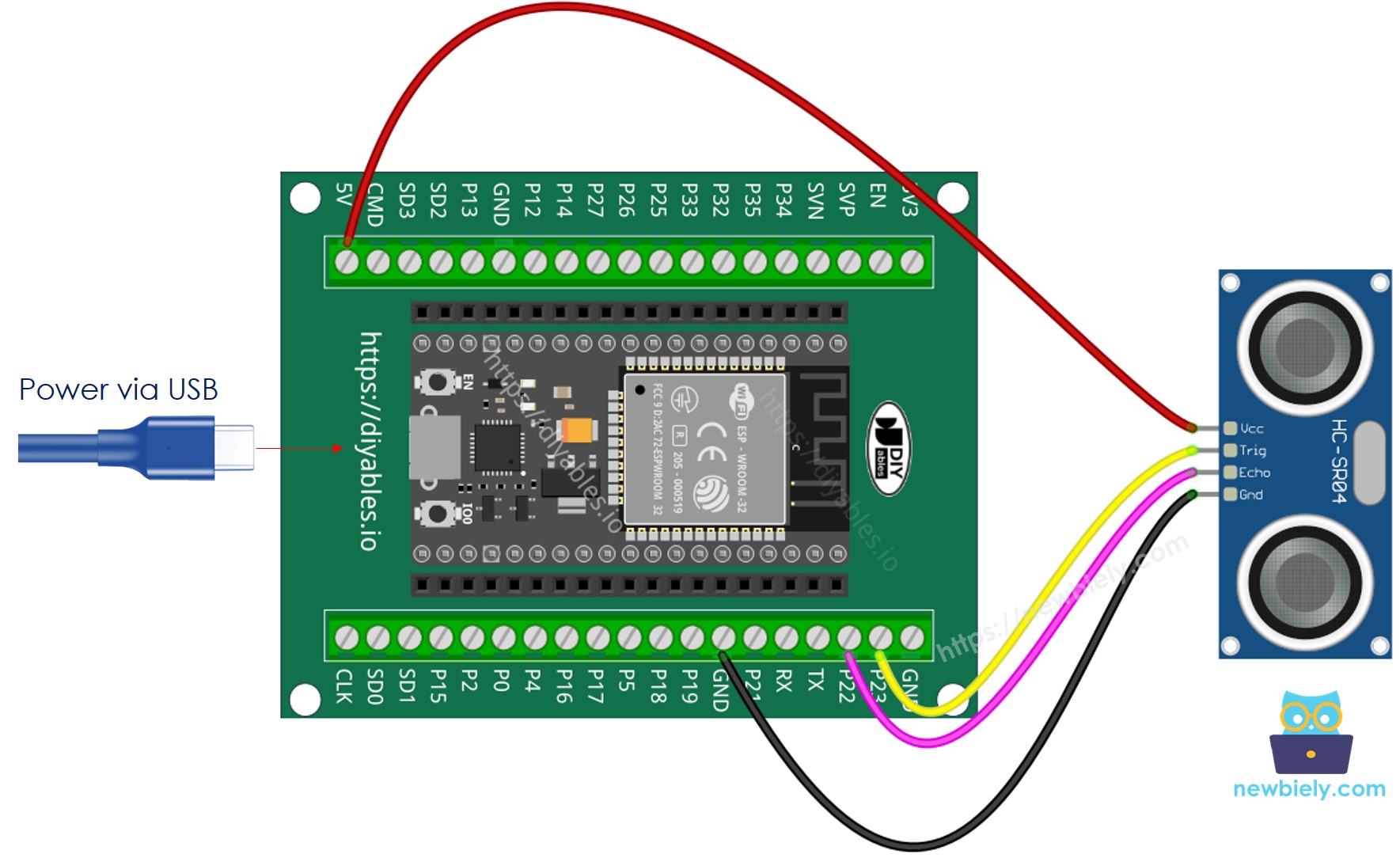
- How to connect ESP32 and ultrasonic sensor using screw terminal block breakout board (powered via Vin pin)
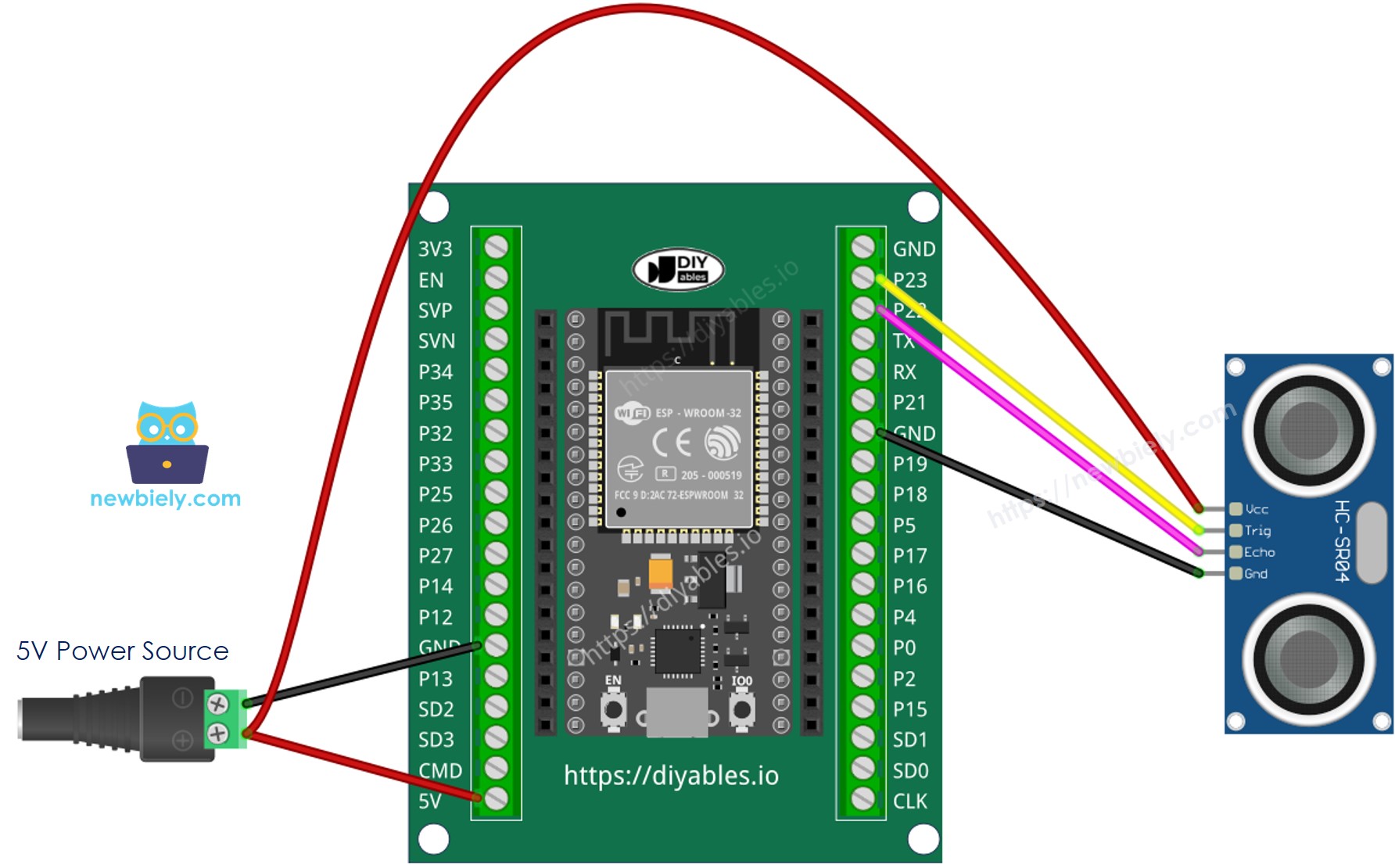
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
How To Program Ultrasonic Sensor
- Generate a 10-microsecond pulse in ESP32's pin by using digitalWrite() and delayMicroseconds() functions. For example, pin GPIO23:
- Measures the pulse duration (µs) in ESP32's pin by using pulseIn() function. For example, pin GPIO22:
- Calculate distance (cm):
ESP32 Code
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Copy the above code and paste it to Arduino IDE.
- Compile and upload code to ESP32 board by clicking Upload button on Arduino IDE
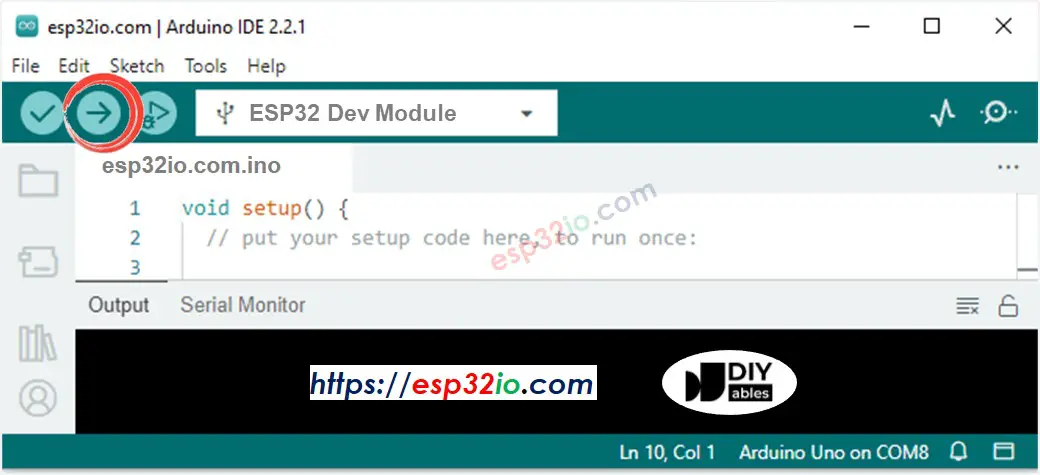
- Open Serial Monitor on Arduino IDE
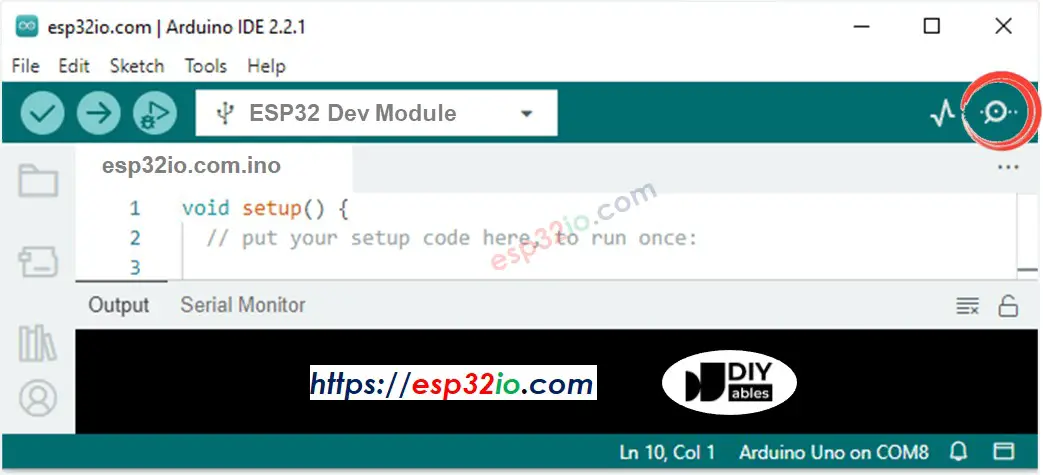
- Move your hand in front of ultrasonic sensor
- See the distance from the sensor to your hand on Serial Monitor
Line-by-line Code Explanation
The above ESP32 code contains line-by-line explanation. Please read the comments in the code!
How to Filter Noise from Distance Measurements of Ultrasonic Sensor
See How to Filter Noise from Distance Measurements of Ultrasonic Sensor
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.