ESP32 - Light Sensor
This tutorial instructs you how to use ESP32 with the light sensor. In detail, we will learn:
- How light sensor works.
- How to connect light sensor to ESP32.
- How to program for ESP32 to read value from a light sensor.
This tutorial shows how to program the ESP32 using the Arduino language (C/C++) via the Arduino IDE. If you’d like to learn how to program the ESP32 with MicroPython, visit this ESP32 MicroPython - Light Sensor tutorial.
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
The LDR light sensor is very affordable, but it requires a resistor for wiring, which can make the setup more complex. To simplify the wiring, you can use an LDR light sensor module as an alternative.
Introduction to Light Sensor
The most wide-used light sensor is a photoresistor (also known as photocell, or light-dependent resistor, LDR).
It can be used to detect the presence ofthe light. It can also be used to measure the illuminance/brightness level of the light.
Light Sensor Pinout
A light sensor has two pins. Just like a normal resistor, We do NOT need to distinguish these pins.
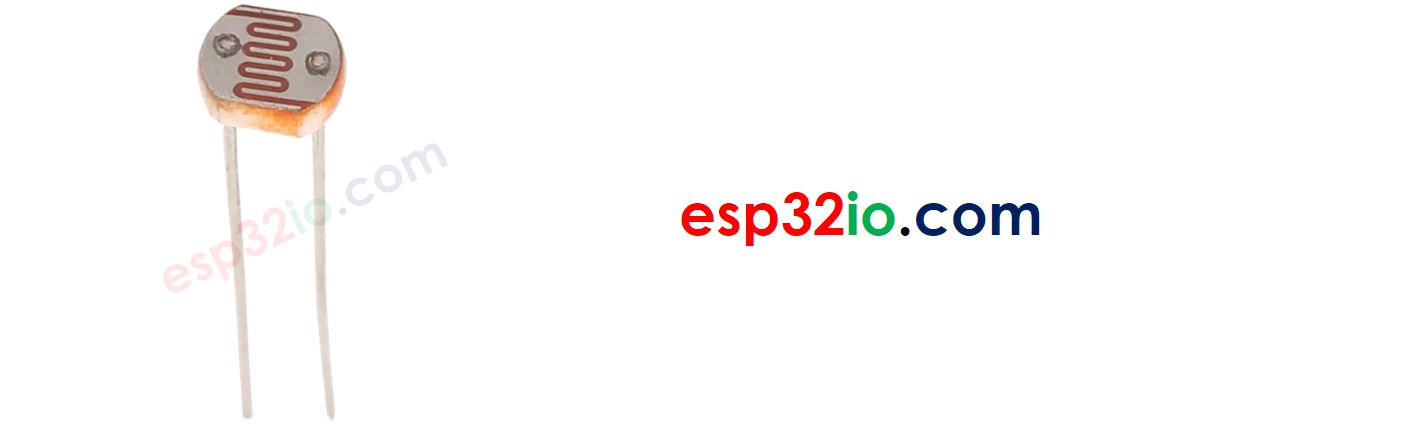
How Light Sensor Works
The photoresistor's resistance is in inverse proportion to the intensity of the light. The less light the photoresistor's face is exposed, the more the photoresistor's resistance is. Therefore, we can infer how bright the ambient light is by measuring the photoresistor's resistance.
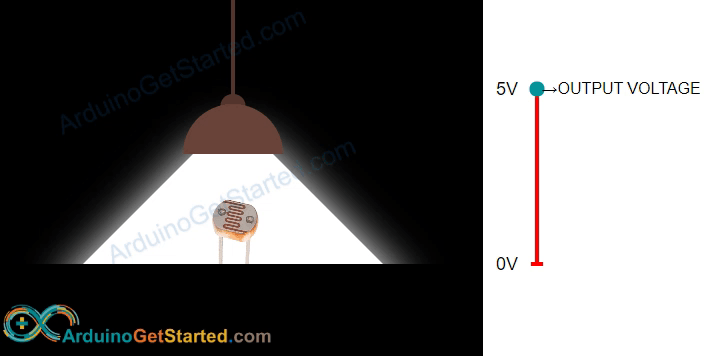
WARNING
The value measured by photoresistor reflects the approximated tendency of the light's intensity, it does NOT represent exactly the luminous flux. Therefore, the photoresistor should not be used in an application that requires high accuracy. calibration is also required for some kind application.
ESP32 - Light Sensor
The ESP32's analog input pin converts the voltage (between 0v and ADC_VREF - default is 3.3V) into integer values (between 0 and 4095), called analog value or ADC value. By connecting an analog input pin of ESP32 to the photoresistor, we can read the analog value by using analogRead() function.
Wiring Diagram between Light Sensor and ESP32
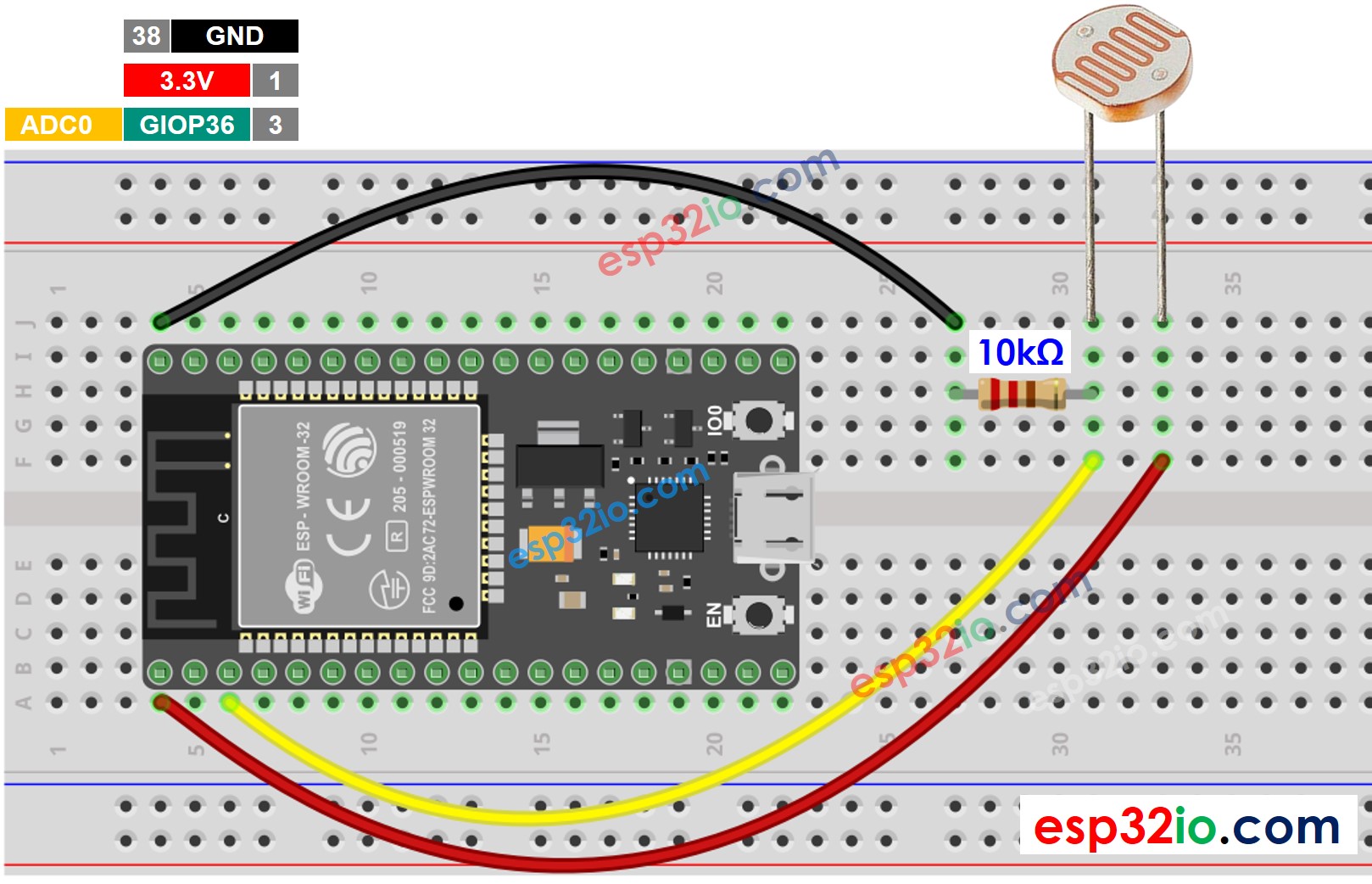
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
ESP32 Code
The below ESP32 code reads the value from a light sensor and infers the light level
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Copy the above code and paste it to Arduino IDE.
- Compile and upload code to ESP32 board by clicking Upload button on Arduino IDE
- Open Serial Monitor on Arduino IDE
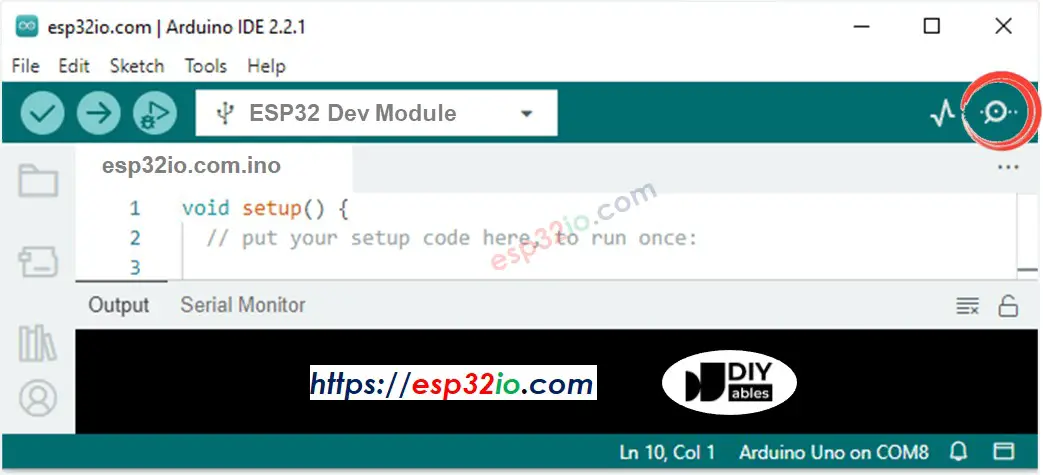
- Radiates light to sensor
- See the result on Serial Monitor. It looks like the below::
Light Sensor and LED
Wiring Diagram
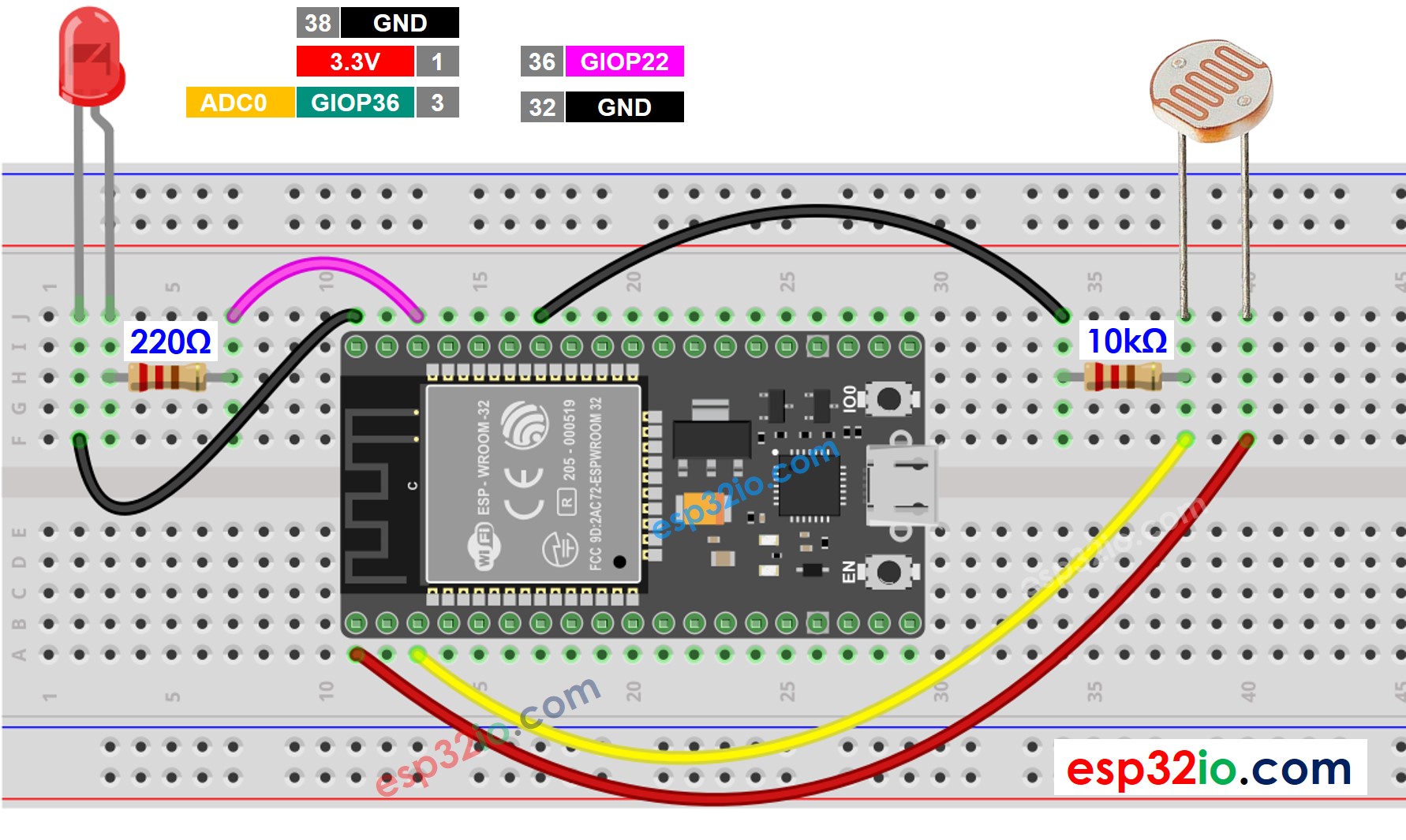
This image is created using Fritzing. Click to enlarge image
ESP32 Code
The below code turns ON the LED if it is dark, otherwise turns OFF the LED
※ NOTE THAT:
This tutorial uses the analogRead() function to read values from an ADC (Analog-to-Digital Converter) connected to a light sensor. The ESP32 ADC is good for projects that do NOT need high accuracy. However, for projects that need precise measurements, please note:
- The ESP32 ADC is not perfectly accurate and might need calibration for correct results. Each ESP32 board can be a bit different, so you need to calibrate the ADC for each individual board.
- Calibration can be difficult, especially for beginners, and might not always give the exact results you want.
For projects that need high precision, consider using an external ADC (e.g ADS1115) with the ESP32 or using an Arduino, which has a more reliable ADC. If you still want to calibrate the ESP32 ADC, refer to ESP32 ADC Calibration Driver
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.