ESP32 - DRV8825 Stepper Motor Driver
In this guide, we'll explore the DRV8825 Stepper Motor Driver and discover how to operate it with an ESP32 to manage the stepper motor. We'll cover the following details:
- What is the DRV8825 stepper motor driver module
- How the DRV8825 stepper motor driver module functions
- How to link the DRV8825 stepper motor driver with ESP32 and stepper motor
- How to code the ESP32 to manage the stepper motor using the DRV8825 module
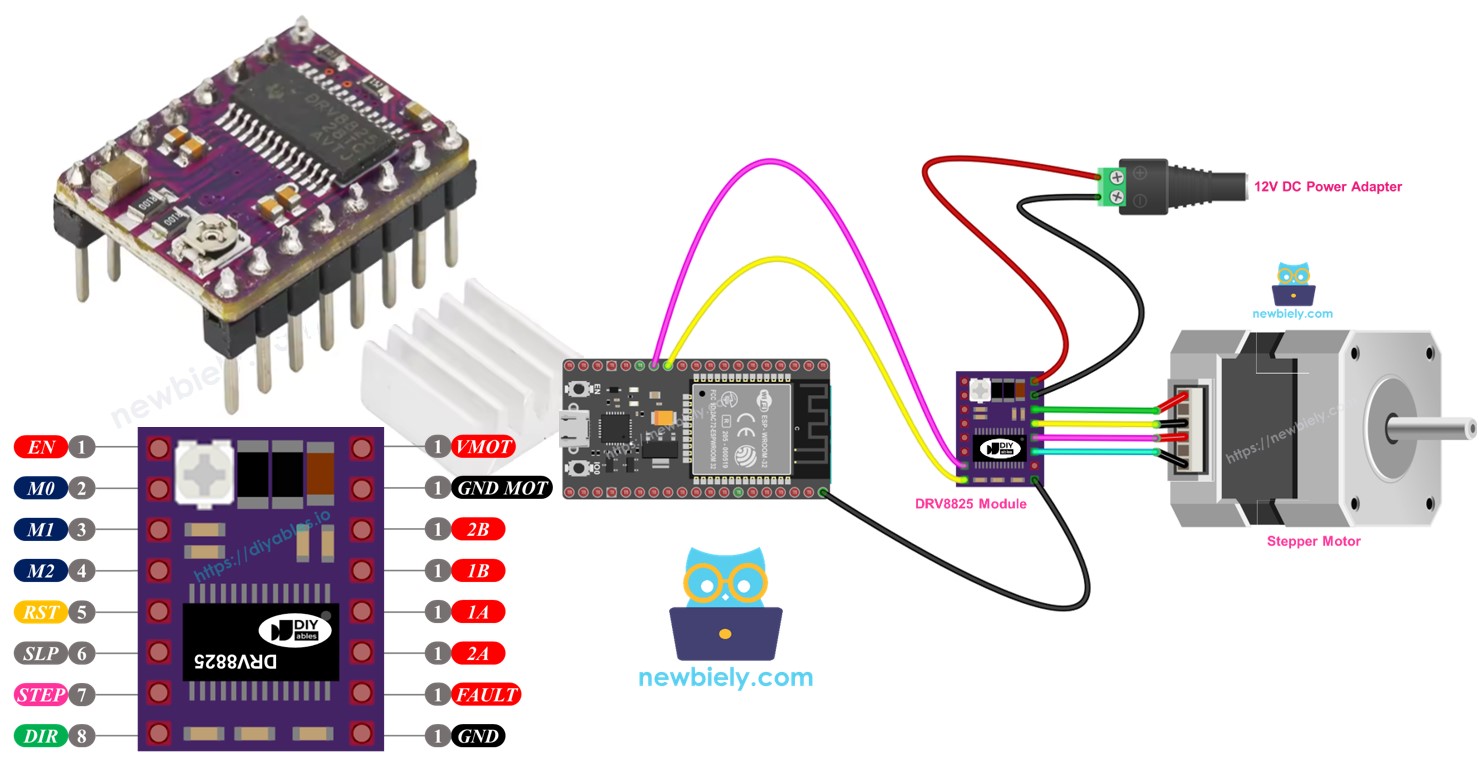
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to DRV8825 Stepper Motor Driver
The DRV8825 is a popular stepper motor driver used to control bipolar stepper motors in devices like CNC machines, 3D printers, and robots. It includes features like an adjustable current limit, protection against overheating, and various microstepping options such as full-step, 1/2, 1/4, 1/8, 1/16, and 1/32. This module can manage up to 2.2A per coil with adequate cooling and works across a broad voltage range from 8.2V to 45V, which makes it versatile for different stepper motors.
To understand concepts about stepper motors such as full-step, microstepping, unipolar stepper, and bipolar stepper, check out the ESP32 - Stepper Motor guide.
It's impressive that managing the speed and direction of a bipolar stepper motor, like the NEMA 17, only needs two ESP32 pins.
DRV8825 Stepper Motor Driver Pinout
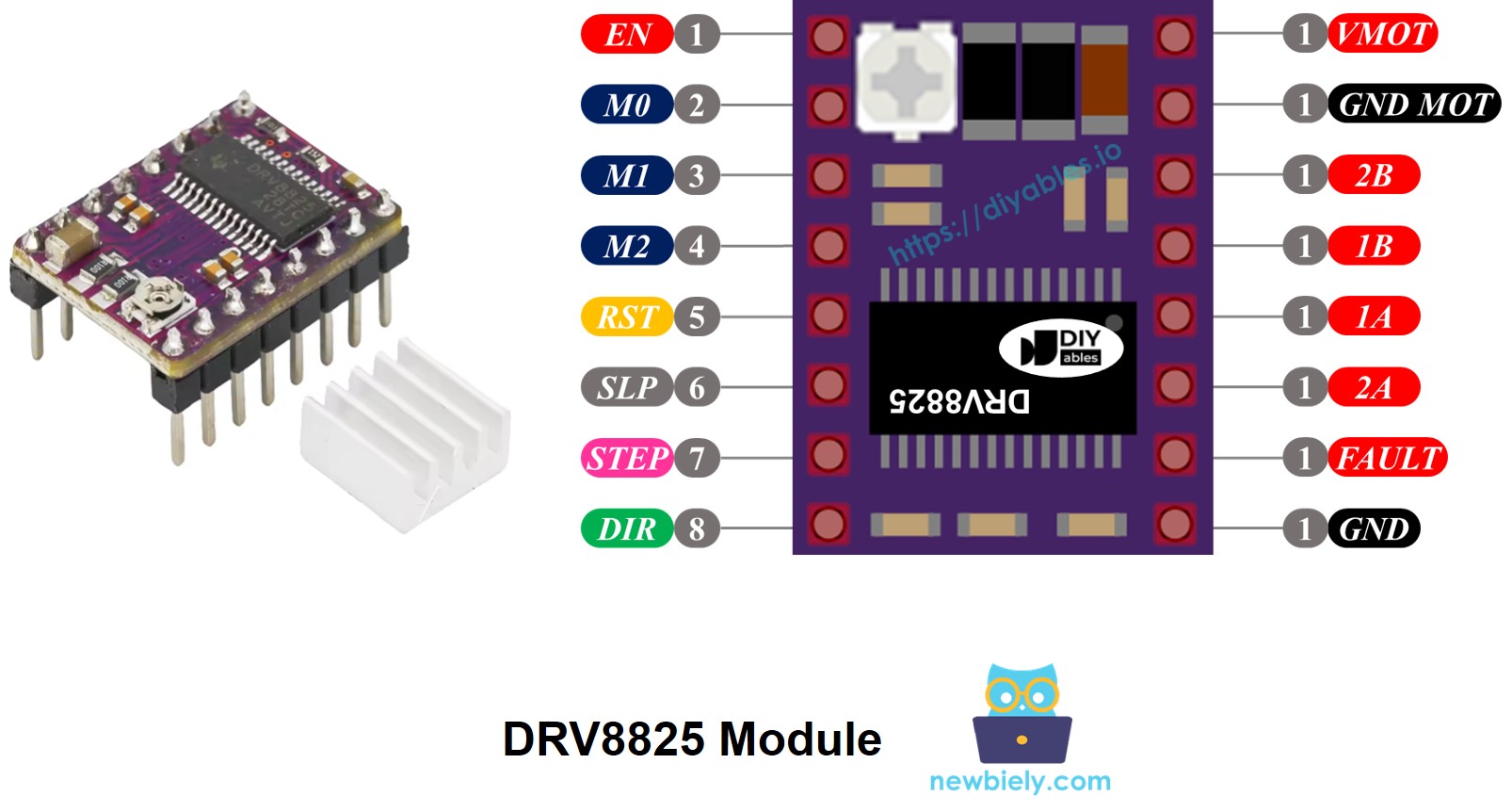
The DRV8825 Stepper motor driver features 16 pins. Here’s a common layout for the DRV8825 stepper motor driver module. Keep in mind that some versions of the module might name the pins differently, but their functions are the same.
Pin Name | Description |
---|---|
VMOT | Motor power supply (8.2 V to 45 V). This powers the stepper motor. |
GND (for Motor) | Ground reference for the motor power supply. Connect this pin to the GND of the motor power supply |
2B, 2A | Outputs to Coil B of the stepper motor. |
1A, 1B | Outputs to Coil A of the stepper motor. |
FAULT | Fault Detection Pin. This is an output pin that drives LOW whenever the H-bridge FETs are disabled as the result of over-current protection or thermal shutdown. |
GND (for Logic) | Ground reference for the logic signals. Connect this pin to the GND of ESP32 |
ENABLE | Active-Low pin to enable/disable the motor outputs. LOW = Enabled, HIGH = Disabled. |
M1, M2, M3 | Microstepping resolution selector pins (see table below). |
RESET | Active-Low reset pin - pulling this pin LOW resets the driver. |
SLEEP | Active-Low sleep pin - pulling this pin LOW puts the driver into low-power sleep mode. |
STEP | Step input - a rising edge on this pin advances the motor by one step (or one microstep, depending on microstepping setting). |
DIR | Direction input - sets the rotation direction of the stepper motor. |
Moreover, there is a tiny built-in knob you can turn to set the current limit, which helps stop the stepper motor and driver from getting too hot.
In short, these 16 pins are grouped into these types based on their use:
- Pins linked to the stepper motor: 1A, 1B, 2A, 2B.
- Pins linked to ESP32 for driver management: ENABLE, M1, M2, M3, RESET, SLEEP.
- Pins linked to ESP32 for controlling motor direction and speed: DIR, STEP.
- Pin for sending feedback to ESP32: FAULT.
- Pins linked to the motor's power source: VMOT, GND (motor power ground).
- Pin linked to the ESP32's ground: GND (logic ground).
The DRV8825 module does not need a logic power source from the ESP32 board because it gets power from the motor power supply through its built-in 3.3V voltage regulator. Nevertheless, it is important to link the ESP32's ground to the DRV8825 module's GND (logic) pin to make sure it works correctly and has a common ground reference.
Microstep Configuration
The DRV8825 driver allows for microstepping by splitting each step into smaller parts. This is done by sending varying levels of current to the motor coils.
For instance, NEMA 17 motor with a 1.8-degree step angle (200 steps per revolution):
- Full-step mode: 200 steps per revolution
- Half-step mode: 400 steps per revolution
- Quarter-step mode: 800 steps per revolution
- Eighth-step mode: 1600 steps per revolution
- Sixteenth-step mode: 3200 steps per revolution
- Thirty-second-step mode: 6400 steps per revolution
As you raise the microstepping level, the motor operates more smoothly and accurately, but it needs more steps for each full turn. If you keep the same step pulse rate (pulses per second), then each full turn will take more time, making the motor slower.
But, if your microcontroller can send pulses fast enough to match the higher step count, you can keep or even boost speed. The real limit depends on how quickly both the driver and your microcontroller can handle these pulses without missing steps.
DRV8825 Microstep Selection Pins
The DRV8825 includes three inputs to select microstep resolution: M0, M1, and M2 pins. By setting these pins to certain logic levels, you can select from six microstepping resolutions:
M0 Pin | M1 Pi | M2 Pi | Microstep Resolution |
---|---|---|---|
Low | Low | Low | Full step |
High | Low | Low | Half step |
Low | High | Low | 1/4 step |
High | High | Low | 1/8 step |
Low | Low | High | 1/16 step |
High | Low | High | 1/32 step |
Low | High | High | 1/32 step |
High | High | High | 1/32 step |
These microstep selection pins come with built-in pull-down resistors that keep them in a LOW state by default. If not connected, the motor will run in full-step mode.
How it Works
To operate a stepper motor with the DRV8825 module, you require two ESP32 pins: one for the DIR pin and another for the STEP pin. The DRV8825 processes these signals from the ESP32 to accurately move the stepper motor.
- STEP Pin: Each signal on the STEP pin moves the motor forward by one tiny step or one full step, based on your setup.
- DIR Pin: Sets which way the motor spins.
The driver uses these signals and its own settings to send control commands to the motor through the 1A, 1B, 2A, and 2B pins.
You can also set up extra pins on the DRV8825 module (ENABLE, M1, M2, M3, RESET, SLEEP) in one of three methods:
- Keep them separate so the driver can use standard settings.
- Connect them directly to GND or VCC for a constant mode.
- Link them to ESP32 pins to manage these functions through your programming.
Wiring Diagram between ESP32, DRV8825 module and Stepper Motor
The diagram below displays the basic connections required between the ESP32, DRV8825 module, and the stepper motor. In this arrangement, the DRV8825 driver functions in its standard mode (full-step).
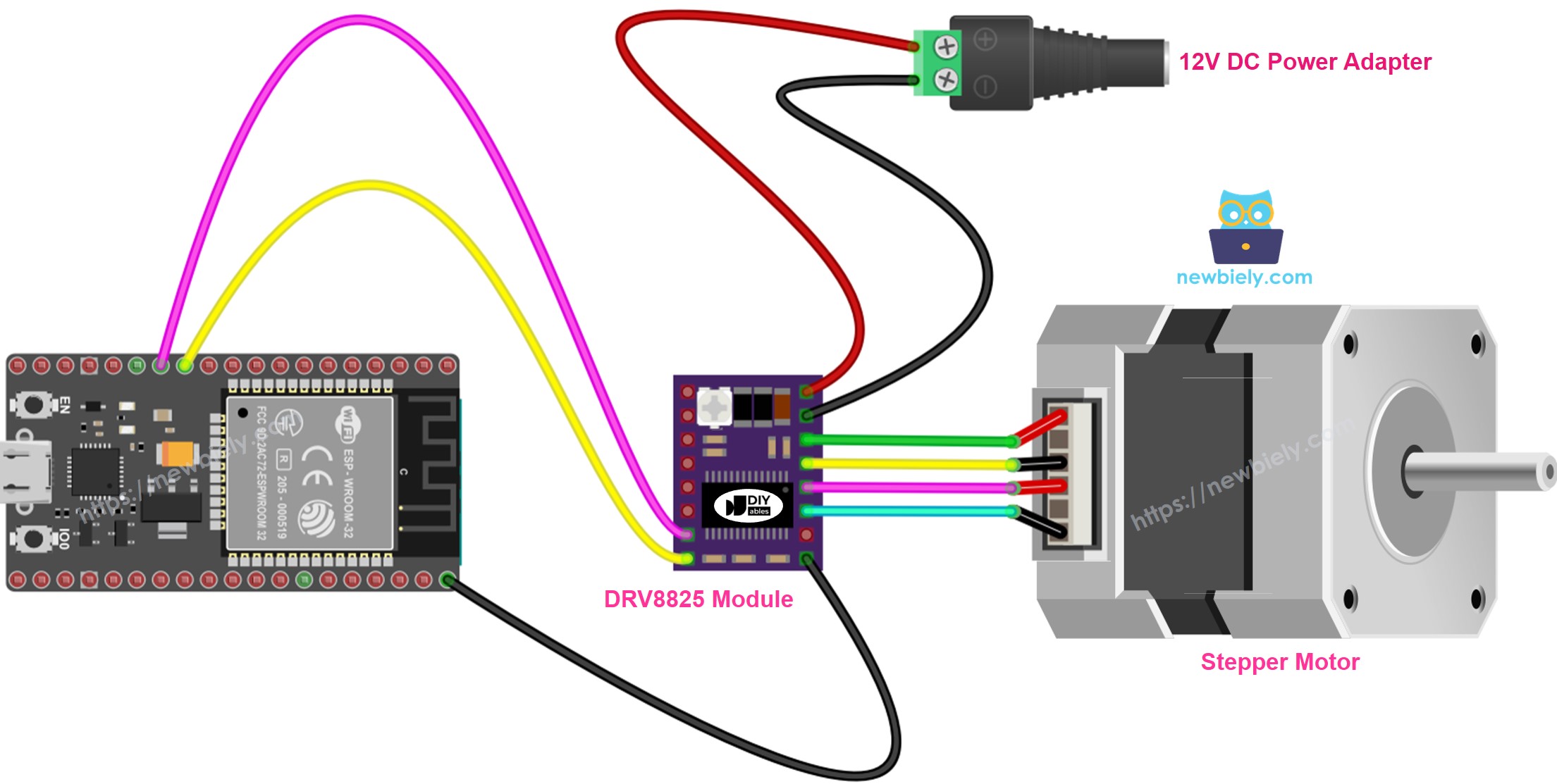
This image is created using Fritzing. Click to enlarge image
In depth:
- VMOT: Connect to the motor's power source (like 12V).
- GND (for Motor): Attach to the motor power supply's ground.
- 1A, 1B, 2A, 2B: Attach these to the coils of the stepper motor.
- STEP: Attach to the digital pin D4 on ESP32.
- DIR: Attach to the digital pin D3 on ESP32.
- GND (for Logic): Connect to the GND pin on ESP32.
- Other pins: Do not connect.
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
ESP32 Code
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Copy the above code and open it in Arduino IDE.
- Go to the Libraries section on the left side of the Arduino IDE.
- Look for "AccelStepper", then locate the AccelStepper library by Mike McCauley.
- Press the Install button to add the AccelStepper library.
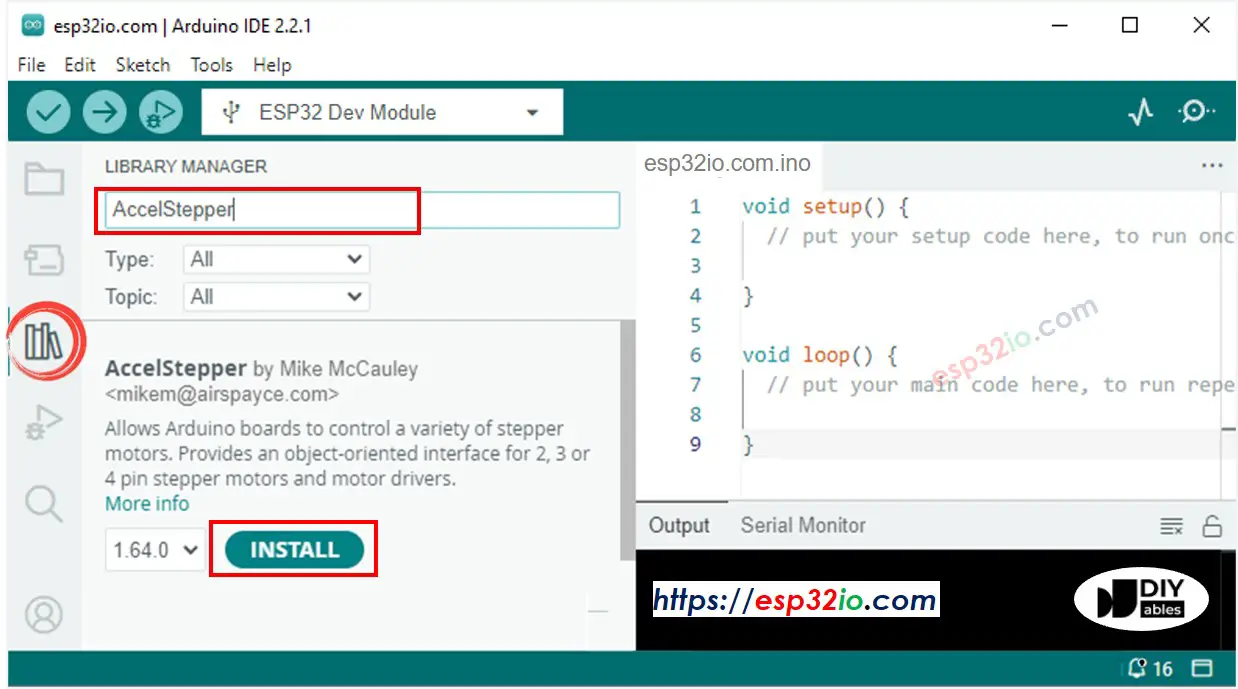
- Copy the code and open it in Arduino IDE
- Click the Upload button in Arduino IDE to send the code to your ESP32
- You will see the motor move back and forth
Tip: When running the motor in full-step mode, its movement might not be very smooth, but this is usual. For a smoother movement, turn on microstepping by setting the M1, M2, and M3 pins.
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.