ESP32 - RFID/NFC Door Lock System
This tutorial instructs you how to use ESP32 to make an RFID/NFC door lock system.
※ NOTE THAT:
You can combine this door lock with the door lock system using keypad.
Hardware Used In This Tutorial
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to RFID/NFC RC522 Module and Electromagnetic Lock
We have specific tutorials about RFID/NFC RC522 module and Electromagnetic Lock. Each tutorial contains detailed information and step-by-step instructions about hardware pinout, working principle, wiring connection to ESP32, ESP32 code... Learn more about them at the following links:
System Components
A door lock system includes two main parts:
- Door Lock: ESP32, RFID/NFC reader, solenoid lock or electromagnetic lock
- Door Key: RFID/NFC tags
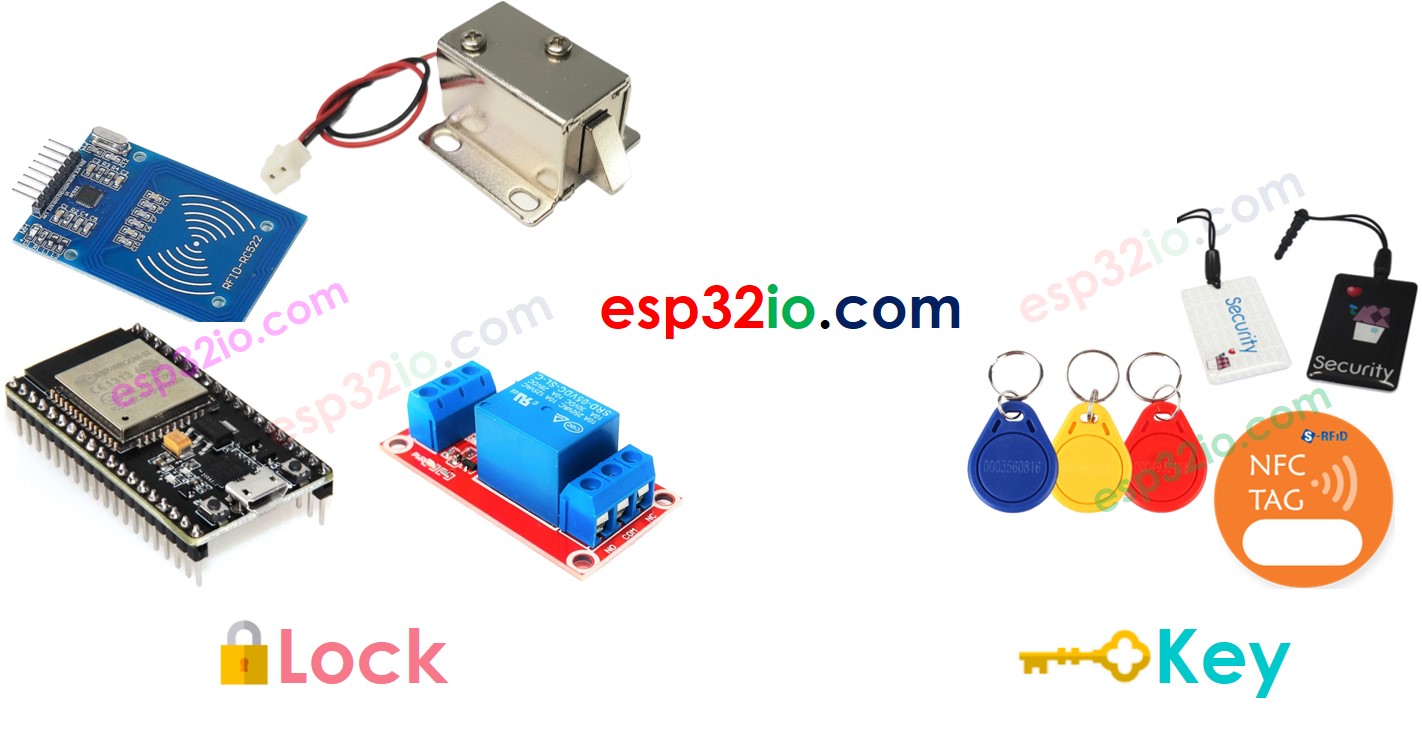
How RFID/NFC Door Lock Works
- The UIDs of authorized tags (key) are predefined in ESP32 code
- When an RFID/NFC tag is tapped on RFID/NFC reader
- ESP32 reads the UID from the reader
- ESP32 compares the UID with the predefined UIDs
- If one UID is matched, ESP32 deactivates the electromagnetic lock to unlock the door.
Wiring Diagram
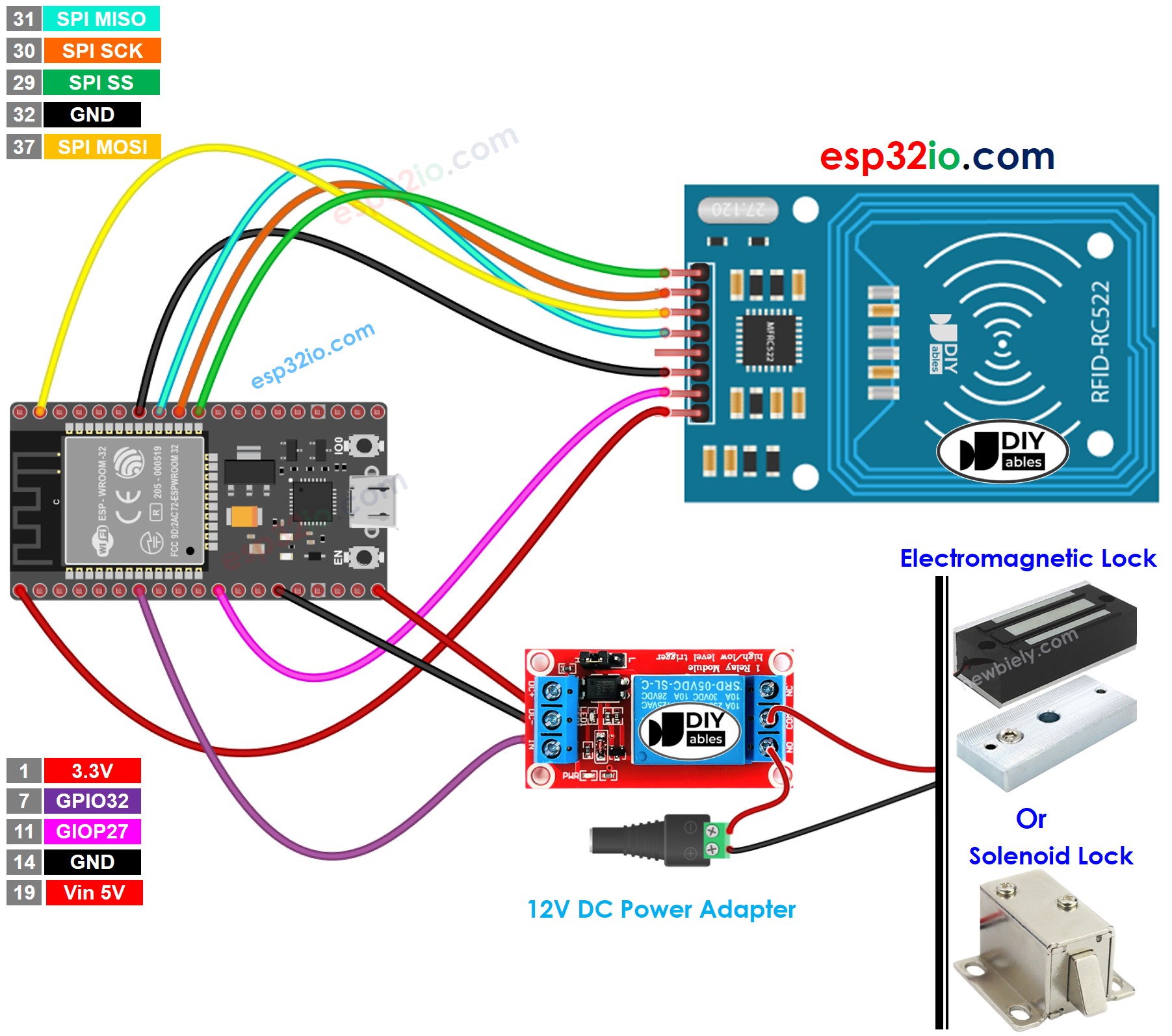
This image is created using Fritzing. Click to enlarge image
※ NOTE THAT:
The pins order can vary according to manufacturers. ALWAYS use the labels printed on the module. The above image shows the pinout of the modules from DIYables manufacturer.
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
ESP32 Code - Single Key
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Type “MFRC522” on the search box, then look for the library by GithubCommunity
- Install the library by clicking on Install button.
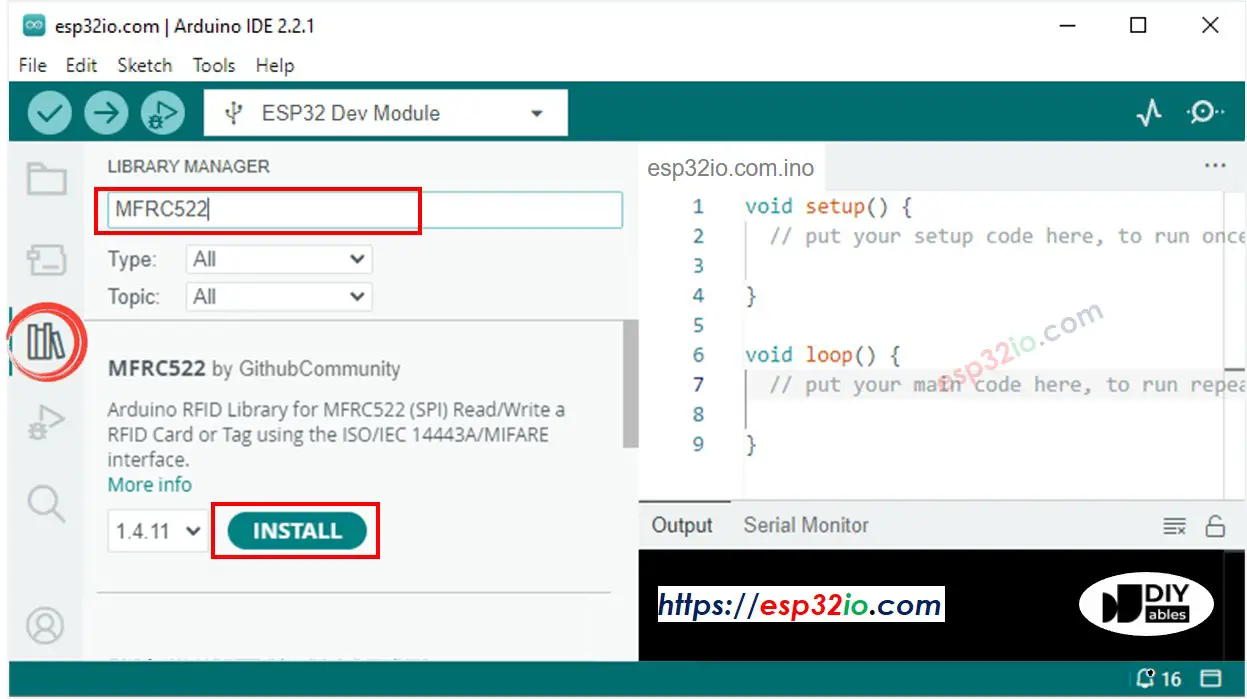
- Find out the tag's UID by doing the following steps:
- Copy the above code and paste it to Arduino IDE.
- Compile and upload code to ESP32 board by clicking Upload button on Arduino IDE
- Open Serial Monitor on Arduino IDE
- Tap an RFID/NFC tag that you want to give authorization on RFID-RC522 reader
- Write down the UID printed on Serial Monitor
- Update UID in the line 18 of the above code. For example, change byte keytagUID[4] = {0xFF, 0xFF, 0xFF, 0xFF}; TO byte keytagUID[4] = {0x2B, 0xB8, 0x59, 0xB1};
- Upload the code to ESP32 again
- Tap an RFID/NFC tag on RFID-RC522 module
- See the result on Serial Monitor
- Check the electromagnetic lock, it should be deactivated
- Tap another RFID/NFC tag on RFID-RC522 module
- See the result on Serial Monitor
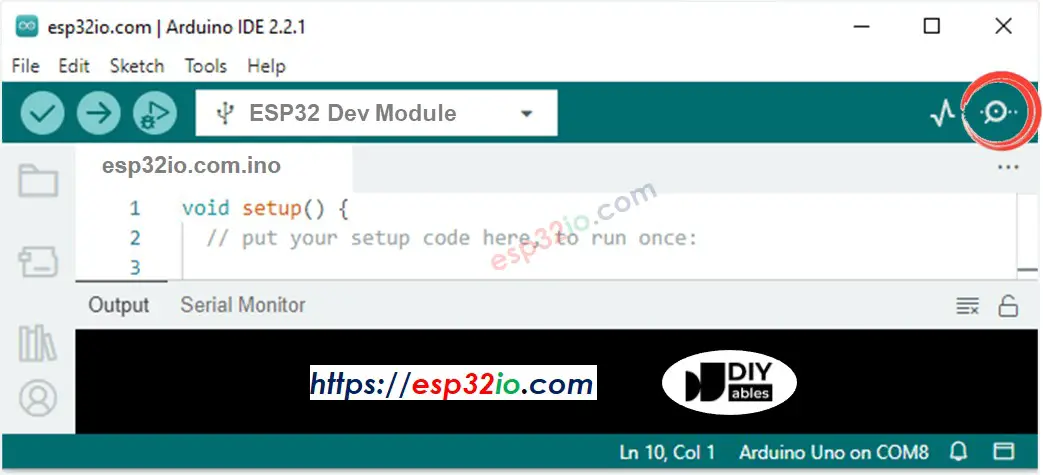
ESP32 Code - Multiple Keys
Let's make a lock that accepts two key: the manager key and secretary key to unlock the door.
Quick Instructions
- Updated two authorized RFID/NFC tag's UUID to the code
- Upload the code to ESP32
- Tap the authorized tags one-by-one on RFID-RC522 module.
- The result on Serial Monitor:
You can modify the code to add three, four, or more tags.
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.