ESP32 - TM1637 4-Digit 7-Segment Display
This tutorial teaches you how to use the ESP32 with the TM1637 4-digit 7-segment display module. It covers the following topics:
- Connecting the 4-digit 7-segment display to the ESP32
- Programming the ESP32 to display information on the 4-digit 7-segment display.
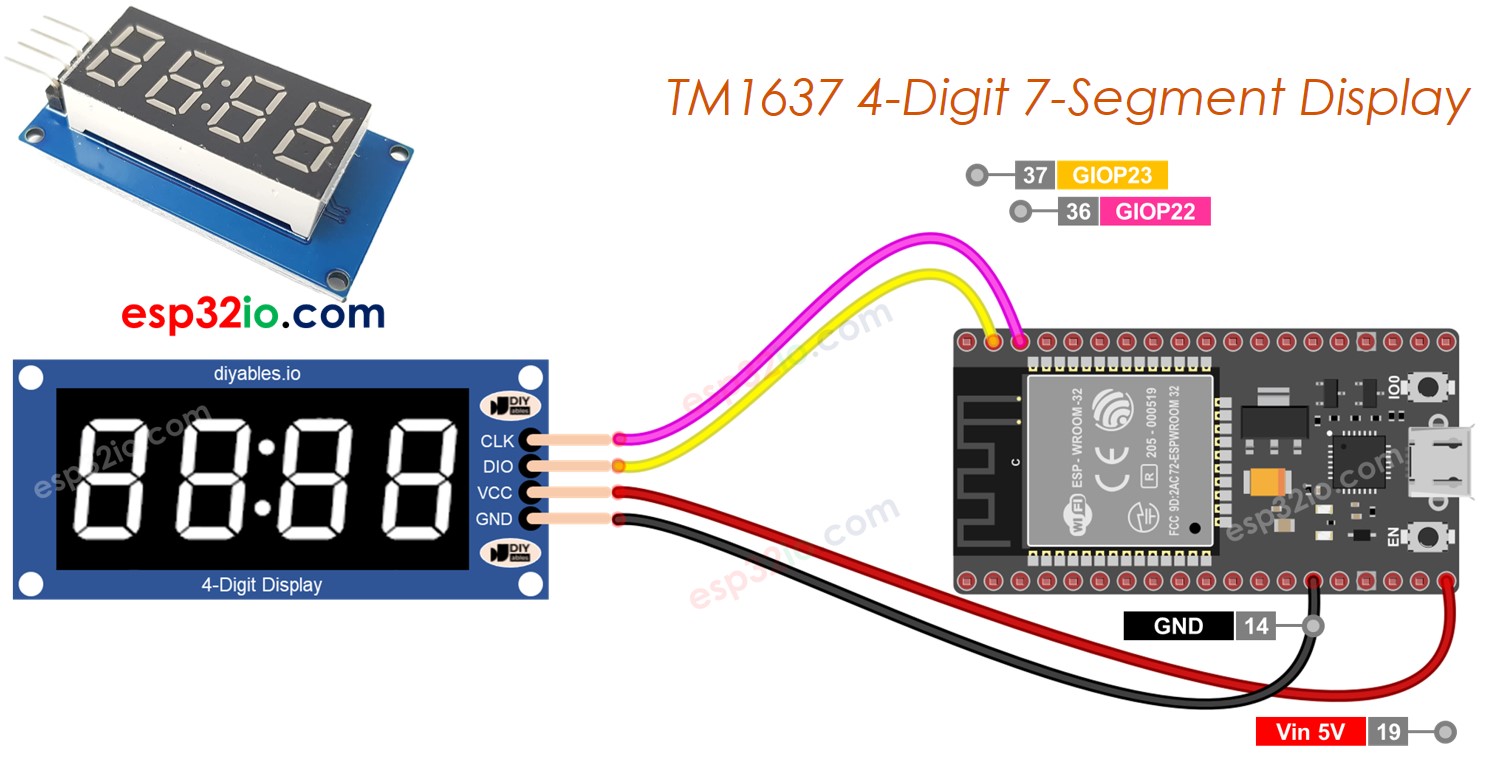
In this tutorial, we will be using a 4-digit 7-segment display module with a colon separator. If you wish to display float numbers, please refer to the 74HC595 4-digit 7-segment Display Module tutorial.
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to TM1637 4-digit 7-segment Display
A 4-digit 7-segment display is commonly used for clock, timer and counter, displaying temperature... However it usually requires 12 connections. The TM1637 module simplifies this by needing only 4 connections: 2 for power and 2 for controlling the segments.
A TM1637 module generally consists of four 7-segment LEDs and one of the following options:
- A colon-shaped LED in the middle: It is ideal for displaying time in hours and minutes, or minutes and seconds, or scores of two teams.
- Four dot-shaped LEDs for each digit: It is ideal for displaying the temperature or any decimal value.
The TM1637 4-Digit 7-Segment Display Pinout
TM1637 4-digit 7-segment display module has four pins:
- CLK pin: is a clock input pin which should be connected to any digital pin on ESP32.
- DIO pin: is a Data I/O pin which should be connected to any digital pin on ESP32.
- VCC pin: is used to supply power to the module and should be connected to the 3.3V to 5V power supply.
- GND pin: is a ground pin which should be connected to the ground of ESP32.
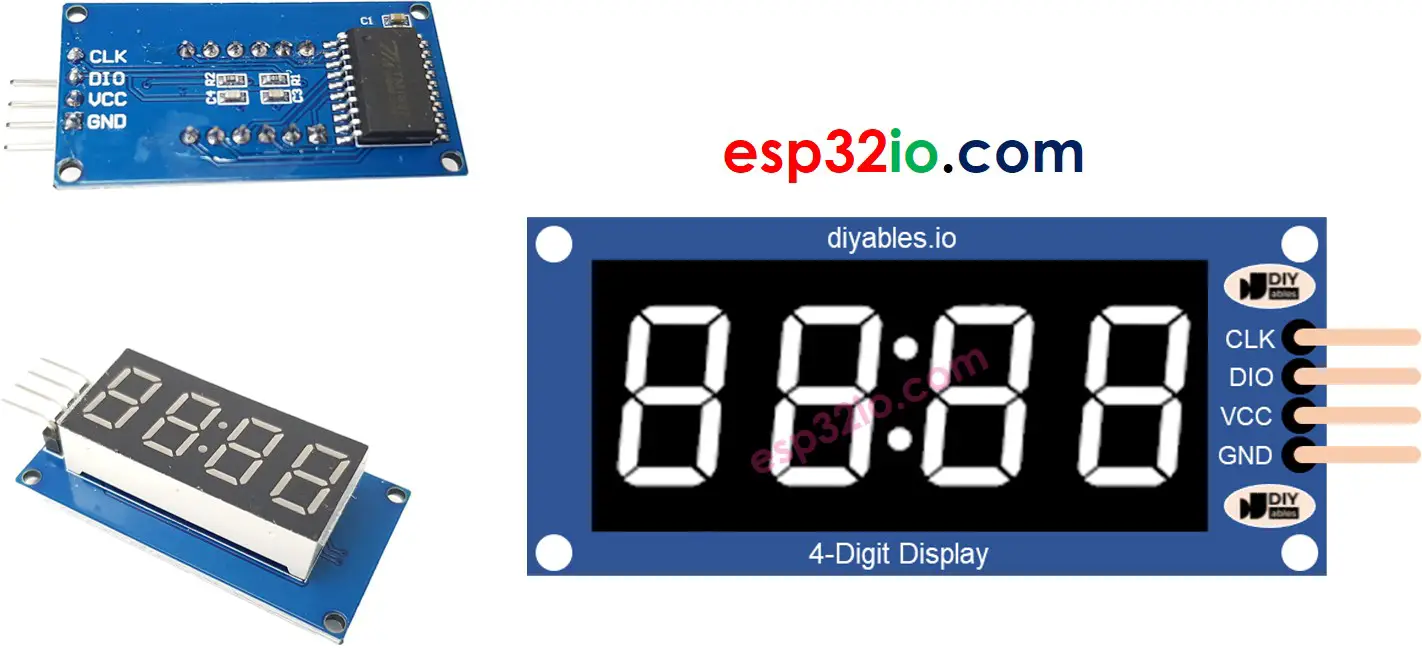
Wiring Diagram
In order to connect a TM1637 to an ESP32, four wires are required: two for power and two for controlling the display. The module can be powered by the 5-volt output of the ESP32. The CLK and DIO pins should be connected to any digital pins of the Arduino; for instance, pins 2 and 3. If different pins are used, the pin numbers in the code must be altered.
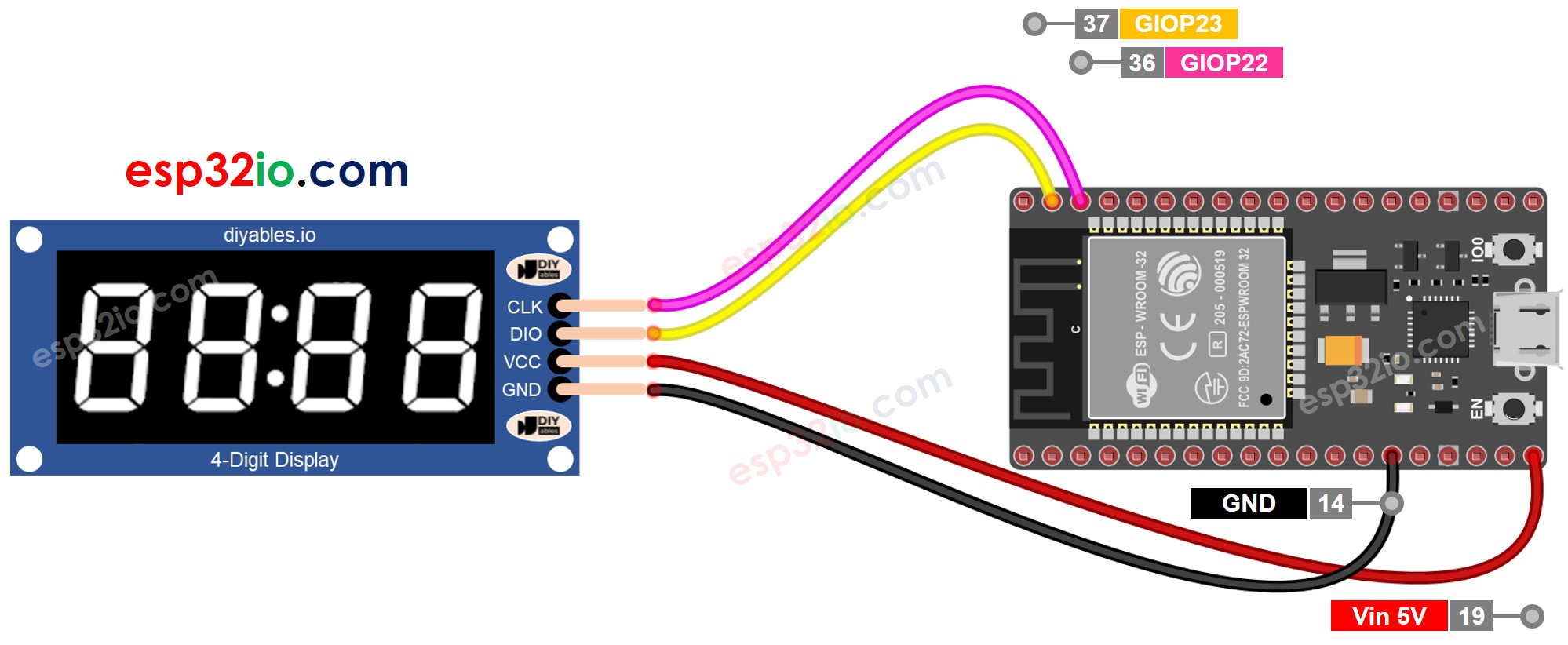
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
Library Installation
To program easily for TM1637 4-digit 7-segment Display, we need to install TM1637Display library by Avishay Orpaz. Follow the below steps to install the library:
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search “TM1637”, then find the TM1637Display library by Avishay Orpaz
- Click Install button.
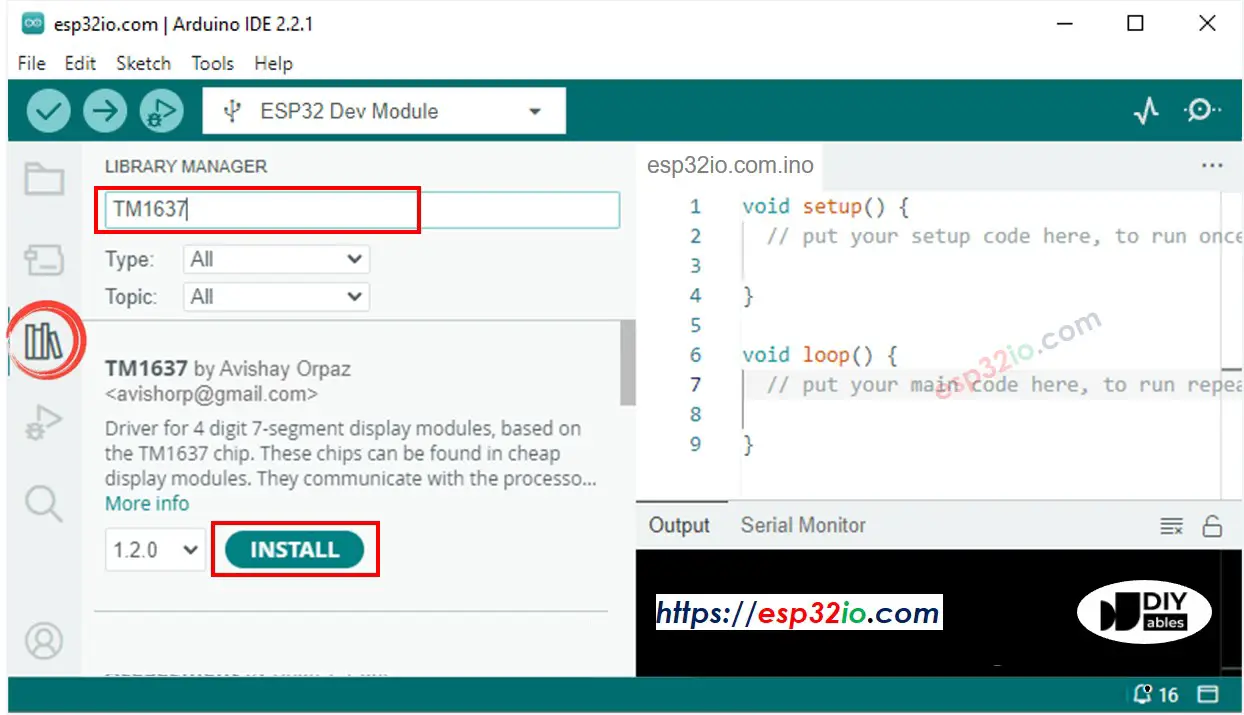
How To Program For TM1637 4-digit 7-segment using ESP32
- Include the library
- Specify the ESP32 pins that are linked to the CLK and DIO of the display module. For instance, D9 and D10.
- Create a TM1637Display object.
- Then you can show numbers, numbers with decimals, numbers with negative signs, or letters. In the case of letters, you need to specify the letter form. Let's look at each one separately.
- Displaying numbers: see the examples below, '_' in the following description stands for a digit that is not displayed in practice:
- Show the number with a colon or dot:
You can find additional information regarding the functions at the end of this tutorial.
ESP32 Code
Quick Instructions
To get started with ESP32 on Arduino IDE, follow these steps:
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Copy the above code and paste it to Arduino IDE.
- Compile and upload code to ESP32 board by clicking Upload button on Arduino IDE
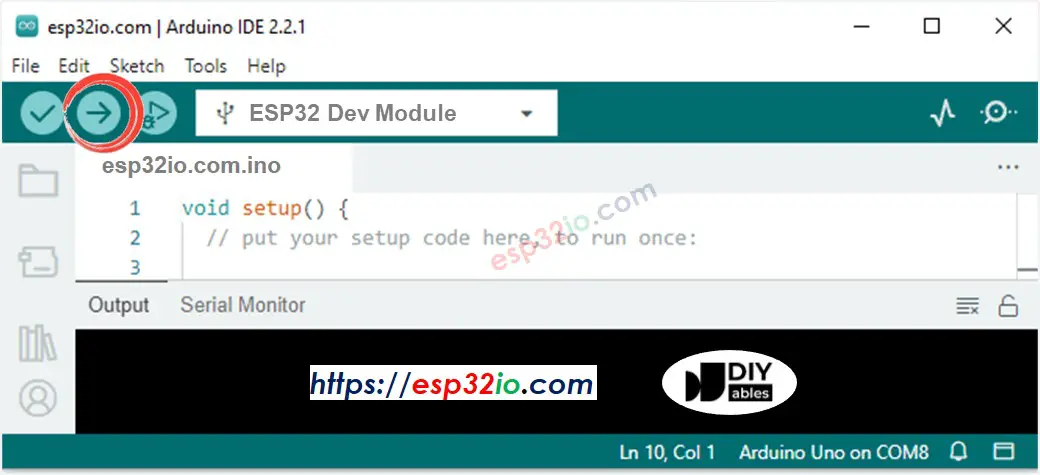
- Observe the states of the 7-segment display.
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.
Function References
Below are the references for:
- display.clear()
- display.showNumberDec()
- display.showNumberDecEx()
- display.setSegments()
- display.setBrightness()
display.clear()
Description
This function clears the display. It turns all LEDs off.
display.showNumberDec()
Description
The 7-segment display is utilized to show a decimal number. This function is used for that purpose.
Syntax
Parameter
- num: is the value to be shown on the 7-segment display, ranging from -9999 to 9999.
- leading_zero: an optional parameter with a default value of false, determines whether leading zeros should be displayed.
- length, another optional parameter with a default value of 4, sets the number of digits to be displayed.
- pos: also an optional parameter with a default value of 0, sets the position of the most significant digit.
Please be aware that the function will not show anything if the number is outside the range or if the length value is more than 4.
showNumberDecEx()
Description
This function is an upgrade of showNumberDec(), providing more control over the display of a decimal number on the 7-segment display. It has the capability to individually control the dot or colon segments of each digit.
Syntax
Parameter
- num1: This is the number to be displayed on the 7-segment display. It should be within the range of -9999 to 9999.
- dots: This parameter is used to specify which segments of the display should be turned on as dots. Each bit of the value corresponds to a digit on the display. Possible values are:
- 0b10000000 for displaying the first dot (0.000)
- 0b01000000 for displaying the second dot (00.00), or the colon (00:00), It depends on the module type.
- 0b00100000 for displaying the third dot (000.0)
- leading_zero: This is an optional parameter with a default value of false. If it is set to true, leading zeros will be shown.
- length: This is an optional parameter with a default value of 4. It determines the number of digits to be displayed on the 7-segment display.
- pos: This is an optional parameter with a default value of 0. It sets the position of the most significant digit of the number.
For example, If you use display.showNumberDecEx(1530, 0b01000000), it will show:
- The number 15:30 on the 7-segment display if the module has a colon-shaped LED.
- The number 15.30 on the 7-segment display if the module has dot-shaped LEDs.
Please be aware that the function will not display anything if the number is outside of the range or if the value of length is more than 4.
setSegments()
Description
The function enables one to directly set the segments of the 7-segment display. It can be used to show letters, special characters, or to switch off all LED segments.
Syntax
Parameter
- segments: This parameter sets the segments of the 7-segment display, which is an array of bytes. Each byte represents the segments of each digit and each segment is represented by a bit in the byte.
- length: This is an optional parameter with a default value of 4. It determines the number of digits to be displayed on the 7-segment display.
- pos: This is an optional parameter with a default value of 0. It specifies the position of the most significant digit of the number.
This function is beneficial when you need to show characters or symbols that are not available on the standard 7-segment display. You can create any pattern you desire by setting the segments directly.
Please be aware that the function will not display anything if the number is out of range or the value of length is greater than 4.
setBrightness()
Description
The 7-segment display's brightness can be adjusted using this function.
Syntax
Parameter
- brightness: This parameter adjusts the luminosity of the 7-segment display. The value should be between 0 and 7, with a higher number producing a brighter display.
- on: This is an optional parameter, with a default value of true. It is used to switch the display on or off. If set to false, the display will be deactivated.