ESP32 - Ultrasonic Sensor - LED
This tutorial instructs you how to use ESP32 and Ultrasonic sensor to control the LED. In detail:
- The ESP32 automatically turns LED on when an object is close to the ultrasonic sensor.
- The ESP32 automatically turns LED off when an object is far from the ultrasonic sensor.
Hardware Used In This Tutorial
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to LED and Ultrasonic Sensor
We have specific tutorials about LED and ultrasonic sensor. Each tutorial contains detailed information and step-by-step instructions about hardware pinout, working principle, wiring connection to ESP32, ESP32 code... Learn more about them at the following links:
Wiring Diagram
The wiring diagram with power supply from USB cable
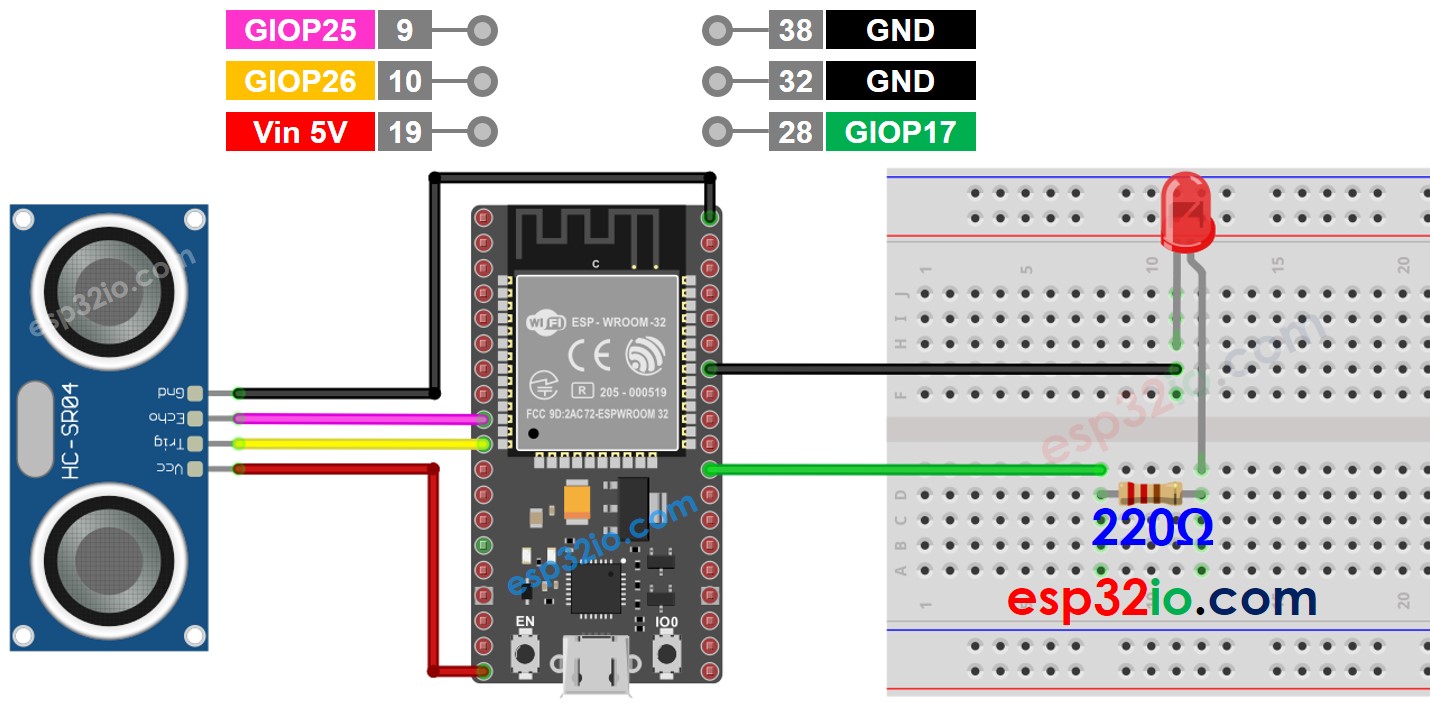
This image is created using Fritzing. Click to enlarge image
The wiring diagram with power supply from 5v adapter
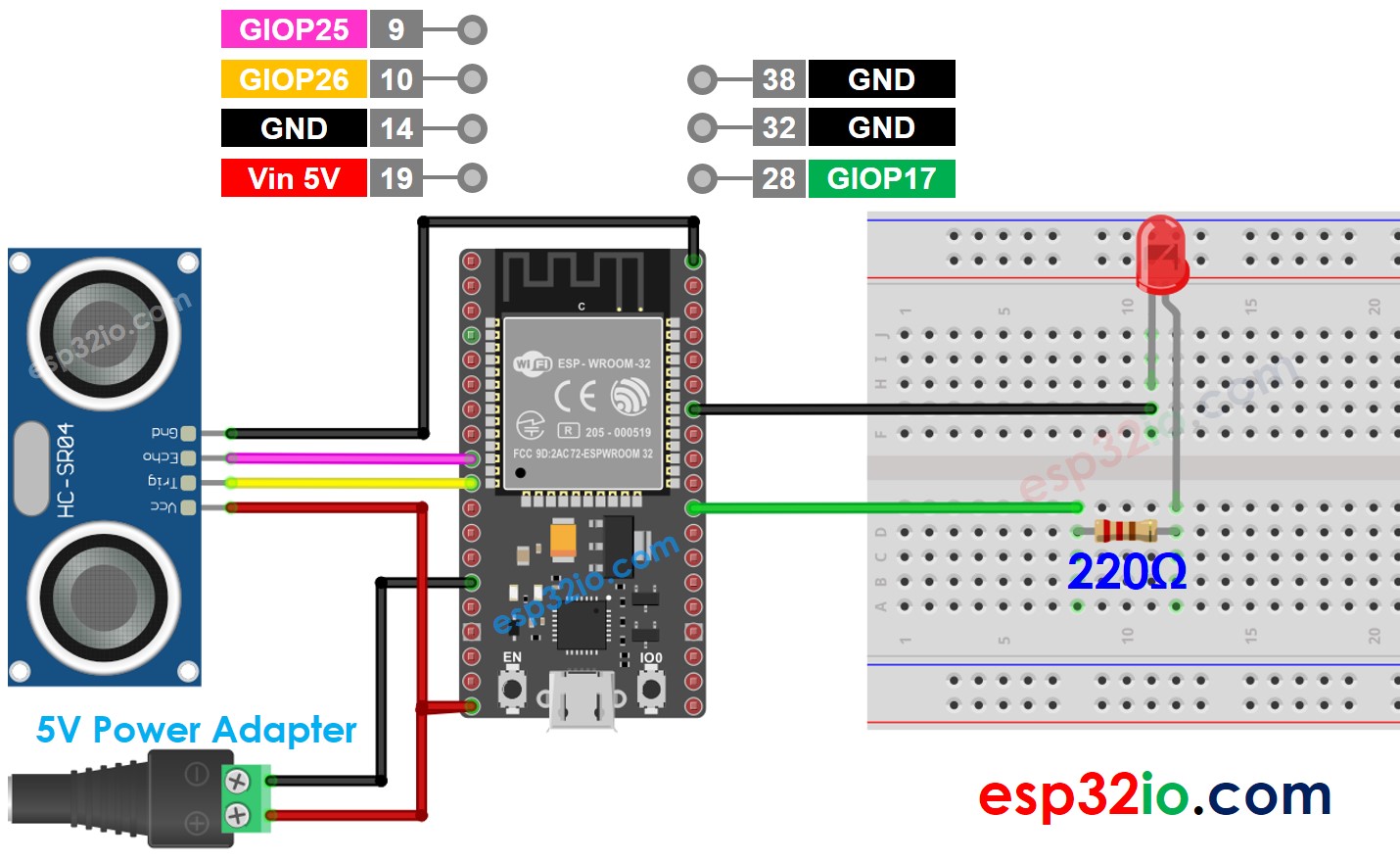
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
ESP32 Code
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Copy the above code and paste it to Arduino IDE.
- Compile and upload code to ESP32 board by clicking Upload button on Arduino IDE
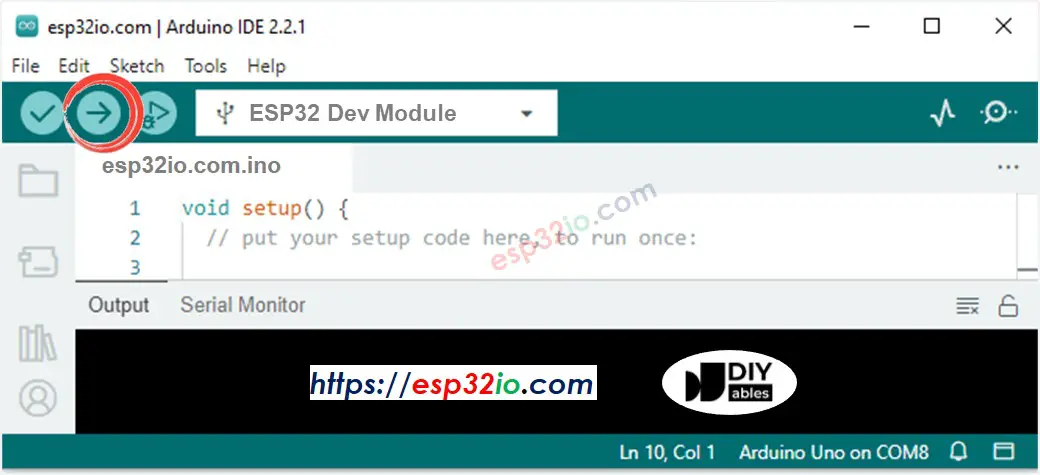
- Move your hand in front of sensor
- See the change of LED's state
Line-by-line Code Explanation
The above ESP32 code contains line-by-line explanation. Please read the comments in the code!
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.