ESP32 - Temperature Humidity Sensor - LCD
This tutorial instructs you how to use ESP32 to read humidity and temperature value from DHT11/DHT22 sensors, and display on LCD I2C.
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to DHT11, DHT22 and LCD
We have specific tutorials about DHT11, DHT22 temperature sensor and LCD. Each tutorial contains detailed information and step-by-step instructions about hardware pinout, working principle, wiring connection to ESP32, ESP32 code... Learn more about them at the following links:
Wiring Diagram
In some cases, ESP32 may not provide enough power for the LCD display. If LCD does not display anything, power LCD by external power source. The below are wiring diagram for some use cases. Note that the DHT22 sensor can works with 5V or 3.3V, so you can connect the VCC pin of DHT22 to 3.3V pin or 5V pin
ESP32 - DHT11 Module LCD I2C Wiring
- Wiring diagram with breadboard, powering ESP32 via USB port, powering LCD via ESP32 board
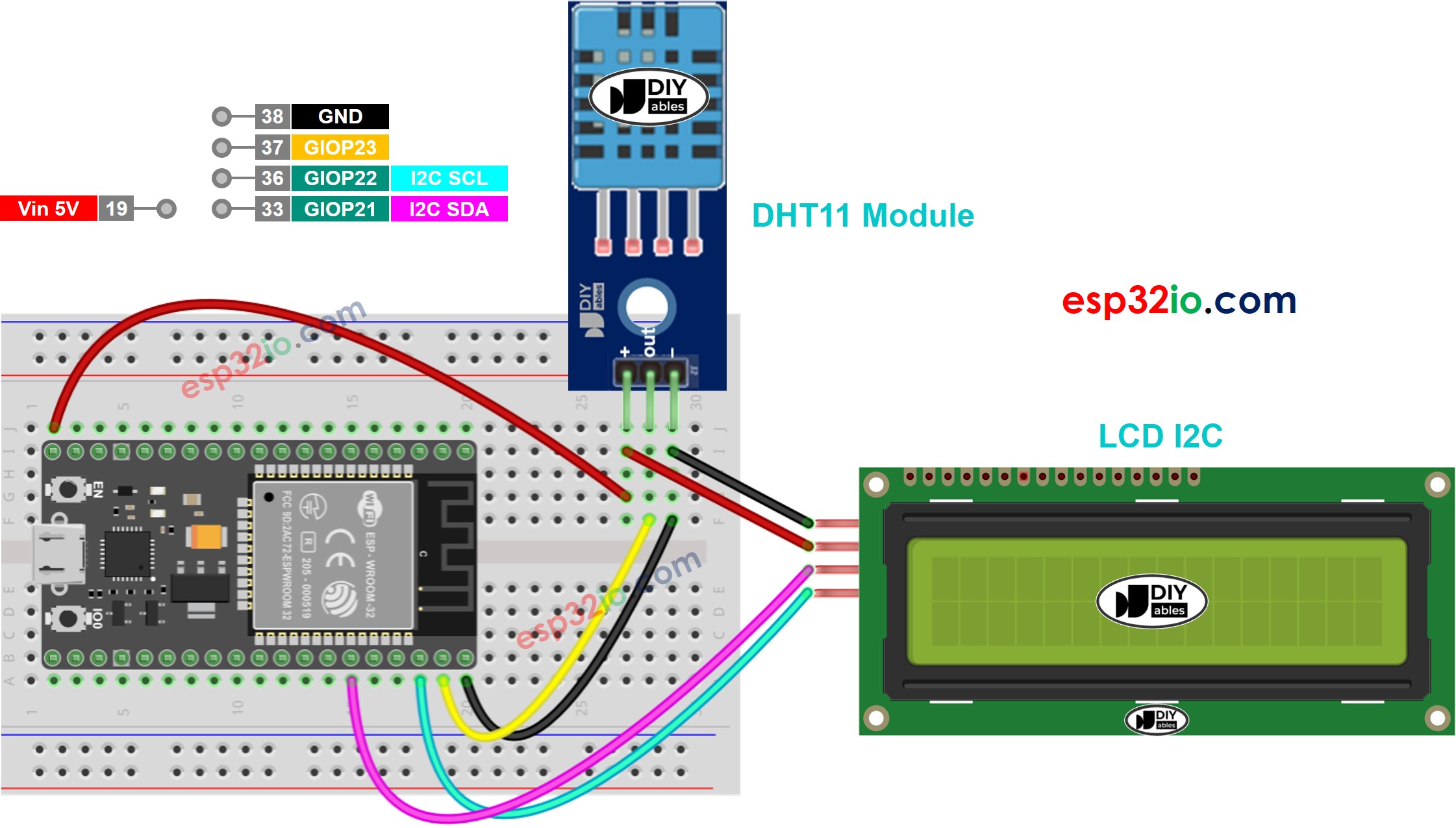
This image is created using Fritzing. Click to enlarge image
- If powering ESP32 via Vin pin and LCD with external power source
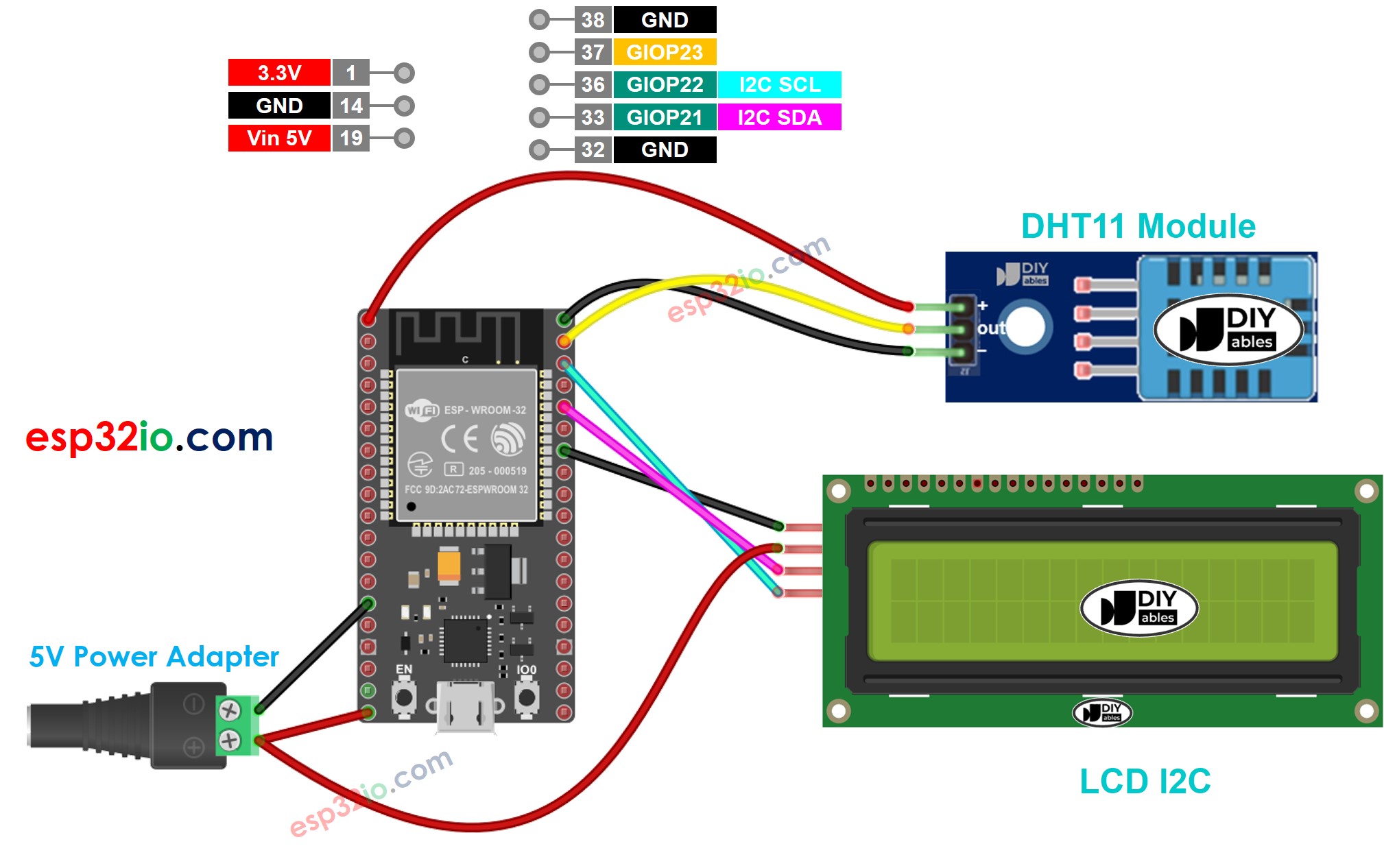
This image is created using Fritzing. Click to enlarge image
- If powering ESP32 via USB port and powering LCD with external power source
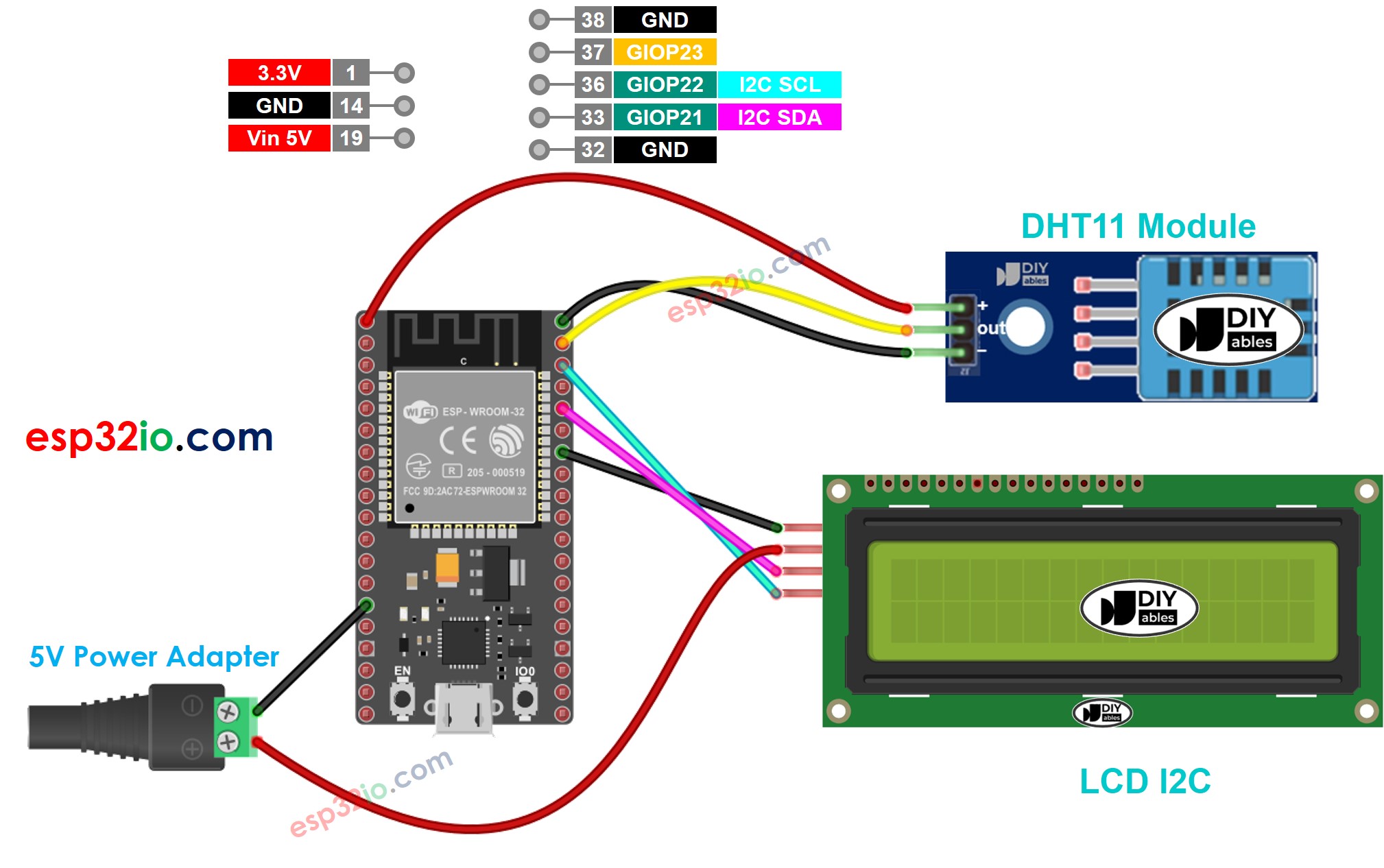
This image is created using Fritzing. Click to enlarge image
ESP32 - DHT22 Module LCD I2C Wiring
- Wiring diagram with breadboard, powering ESP32 via USB port, powering LCD via ESP32 board
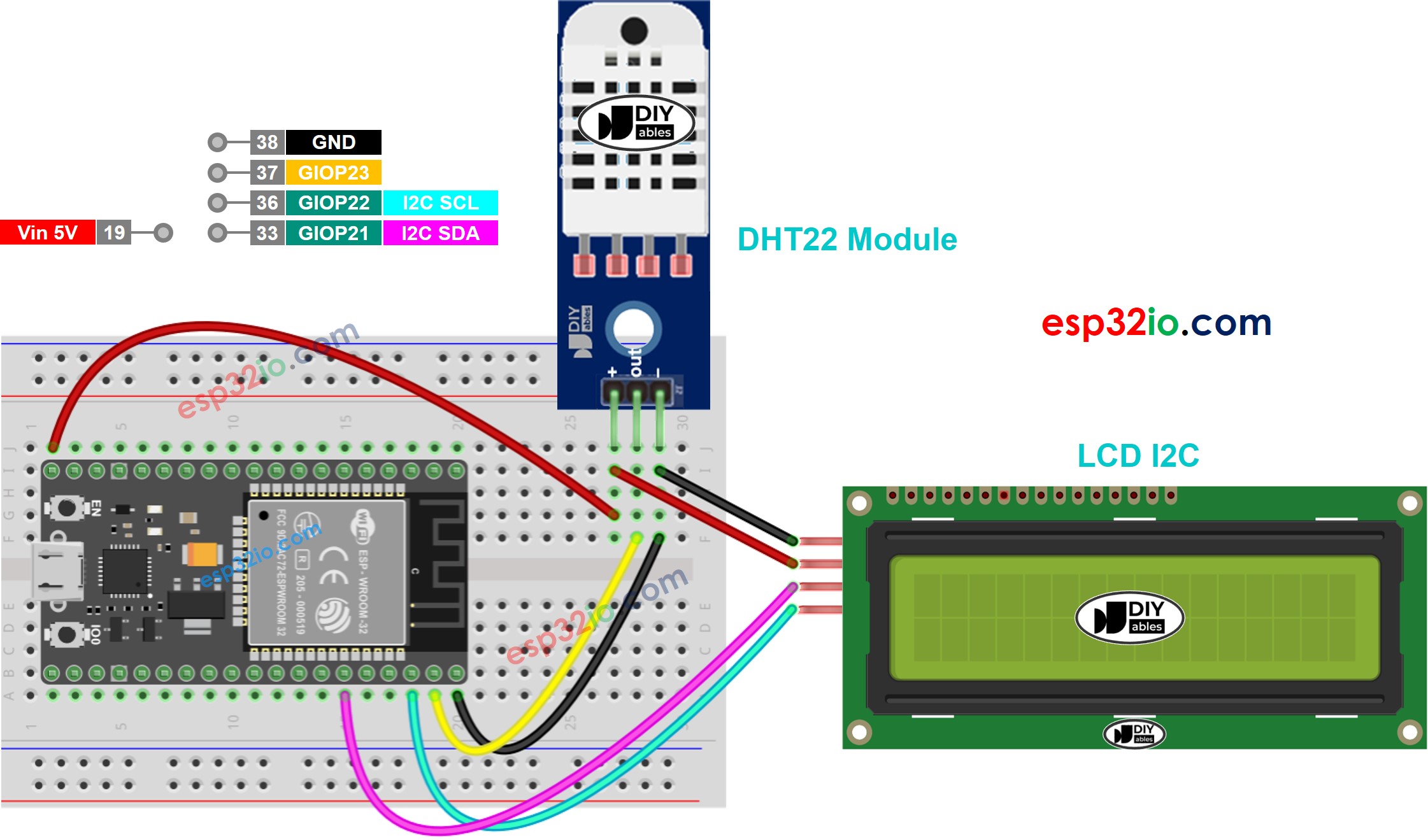
This image is created using Fritzing. Click to enlarge image
- If powering ESP32 via Vin pin and LCD with external power source
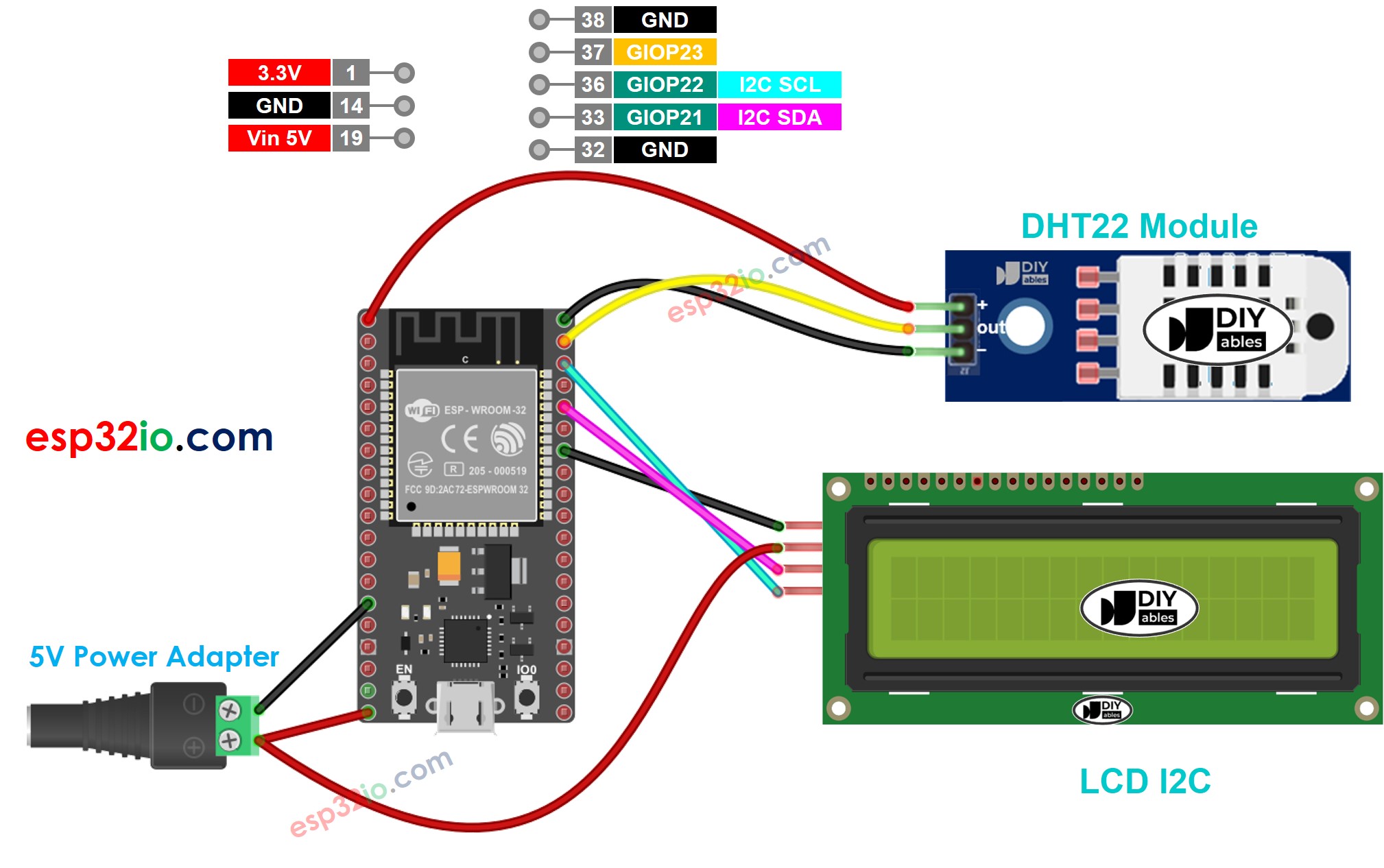
This image is created using Fritzing. Click to enlarge image
- If powering ESP32 via USB port and powering LCD with external power source
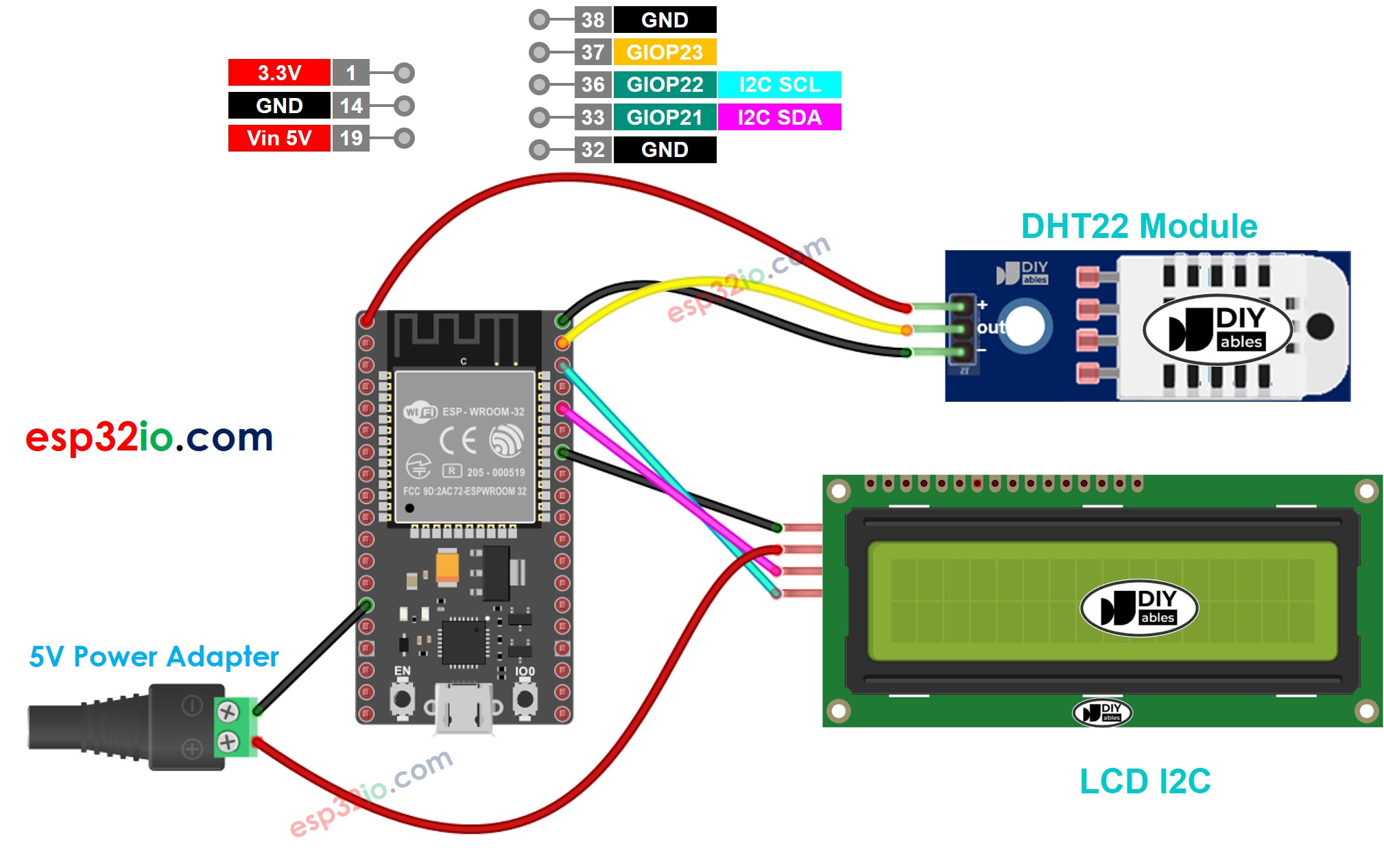
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
ESP32 Code - DHT11 Sensor - LCD I2C
※ NOTE THAT:
The LCD I2C address can be different from each manufacturer. In the code, we used address of 0x27 that is specified by DIYables manufacturer
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Type “DHT” on the search box, then look for the DHT sensor library by Adafruit
- Install the library by clicking on Install button.
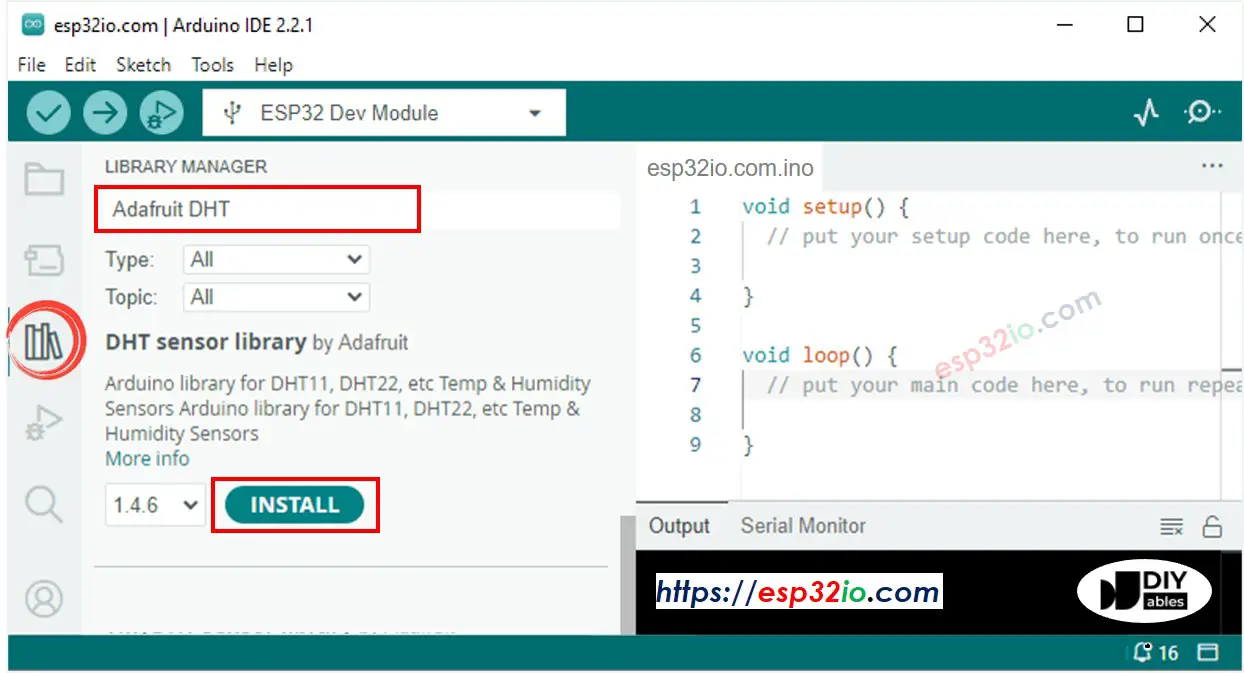
- A windows appears to ask you to install dependencies for the library
- Install all dependencies for the library by clicking on Install All button.
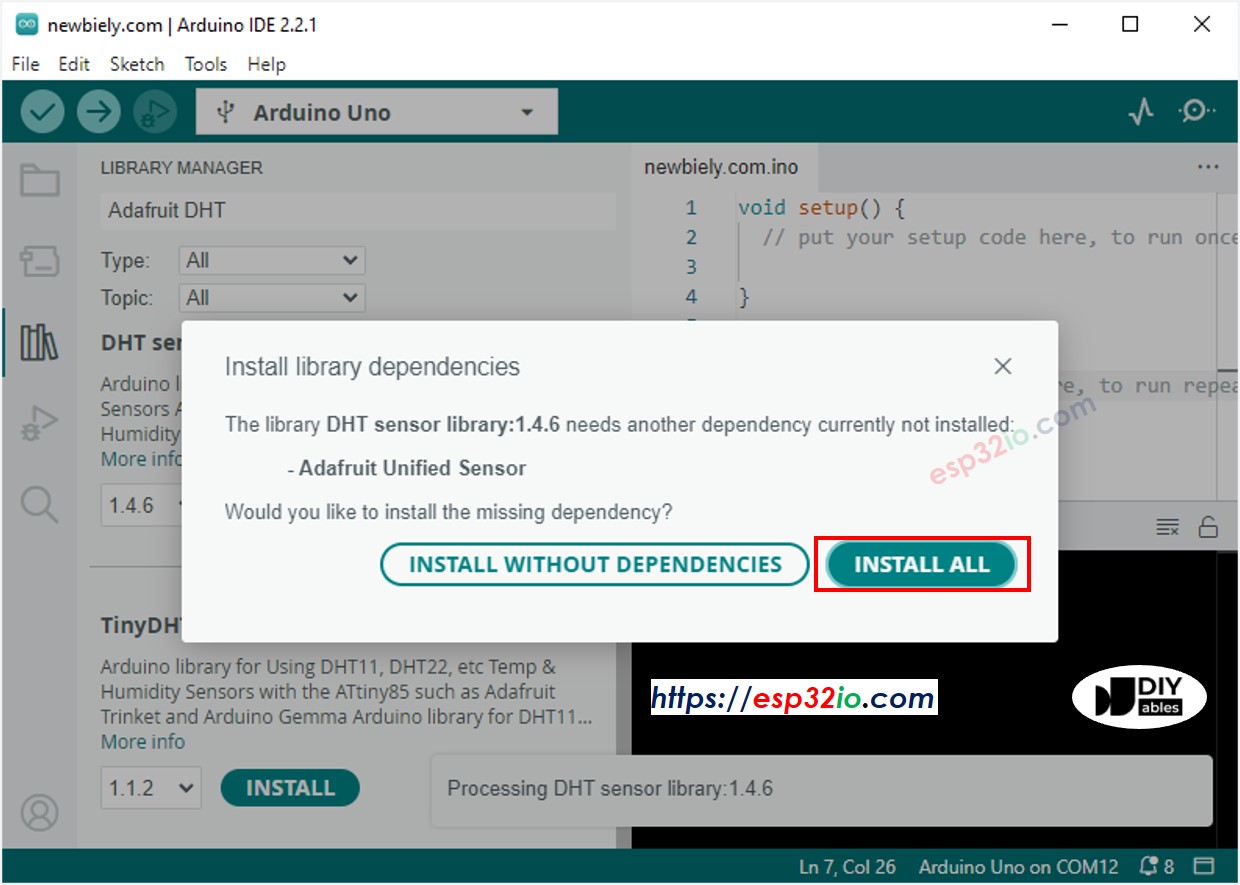
- Type “LiquidCrystal I2C” on the search box, then look for the LiquidCrystal_I2C library by Frank de Brabander
- Click Install button to install LiquidCrystal_I2C library.
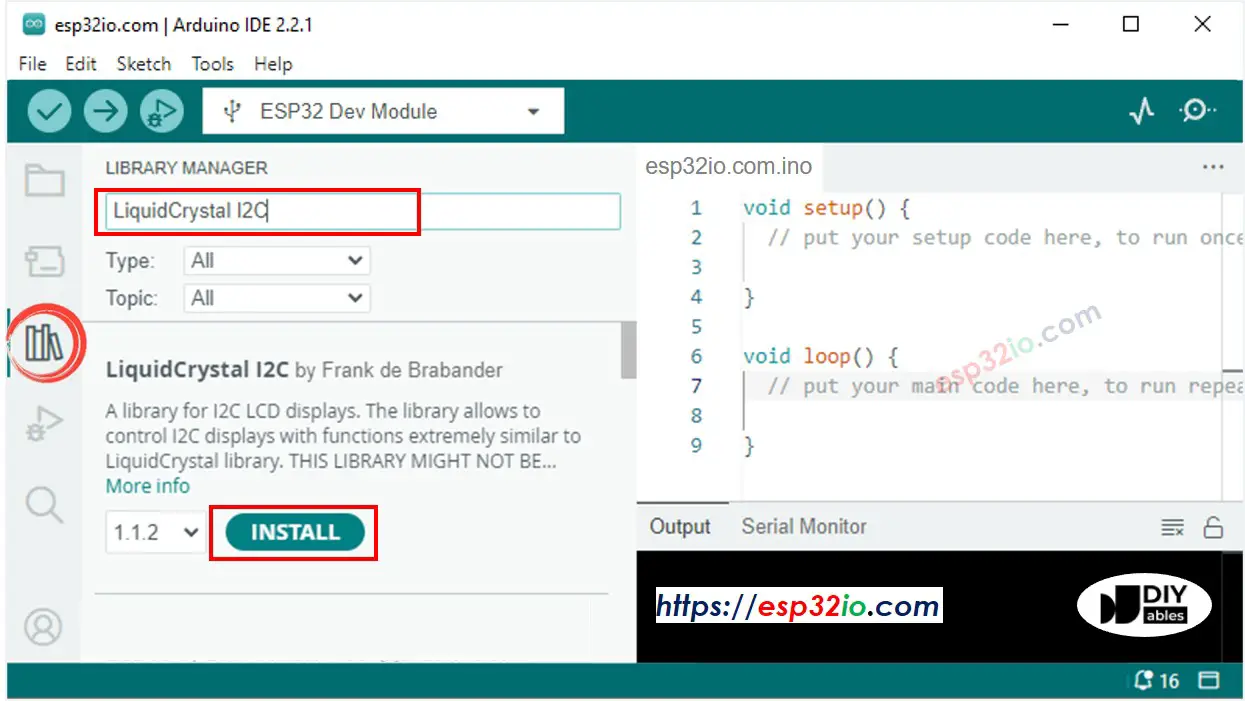
- Copy the above code and paste it to Arduino IDE.
- Compile and upload code to ESP32 board by clicking Upload button on Arduino IDE
- Make the sensor colder or hotter. For example, putting the sensor near a hot cup of coffee
- See the result in LCD
If LCD displays nothing, see Troubleshooting on LCD I2C
ESP32 Code - DHT22 Sensor - LCD I2C
Two above code has only one line different.
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.