ESP32 - Sound Sensor
The sound sensor has the capability to detect the presence of sound in its surroundings. It can be utilized to create trendy sound-responsive projects like lights that activate with a clap or a pet feeder that responds to sound.
In this tutorial, we will learn how to use the ESP32 and a sound sensor to detect sound. We will delve into the following:
- Connecting the sound sensor to the ESP32
- Programming the ESP32 to detect sound using the sound sensor.
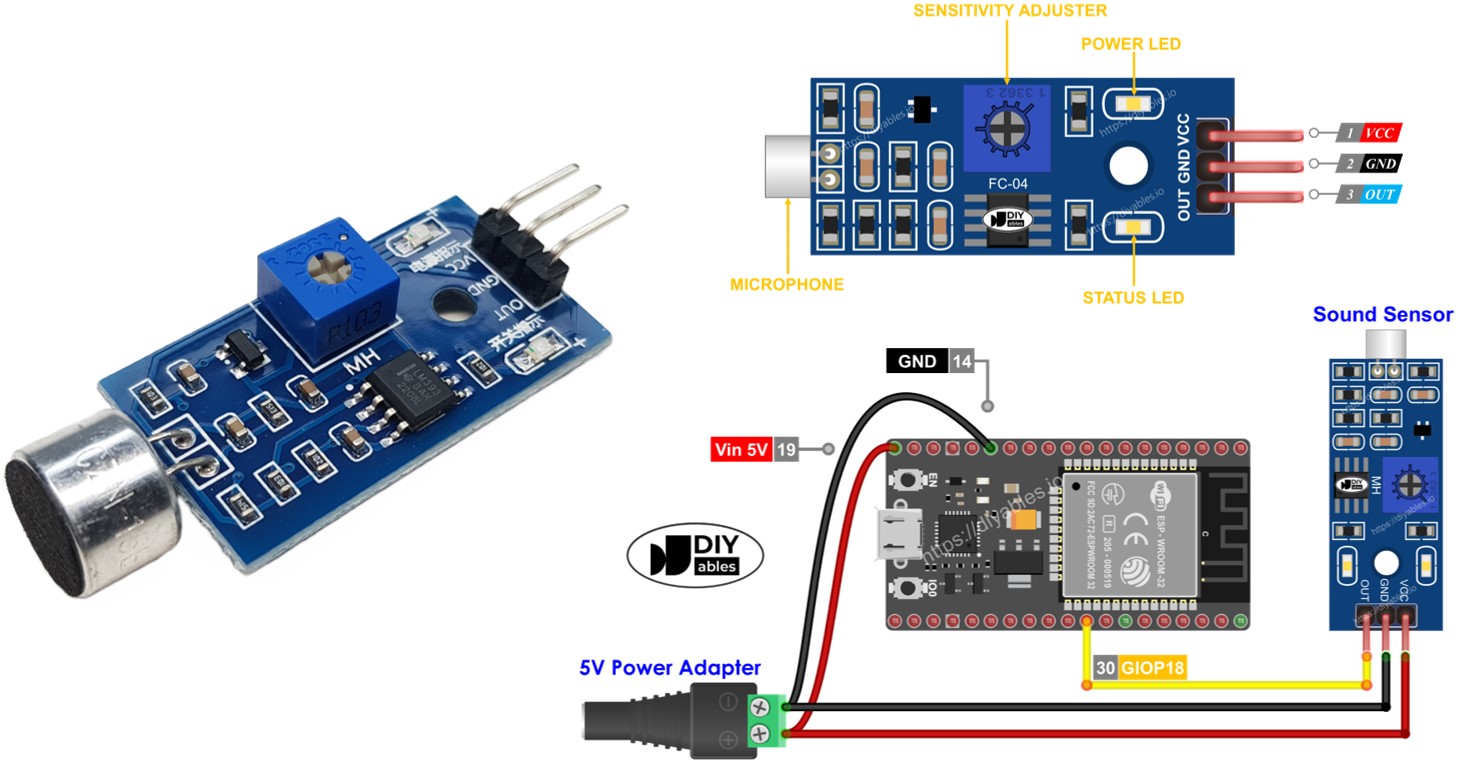
Following that, you have the flexibility to modify the code to trigger an LED or a light (using a relay) upon sound detection, or even enable a servo motor to rotate.
This tutorial shows how to program the ESP32 using the Arduino language (C/C++) via the Arduino IDE. If you’d like to learn how to program the ESP32 with MicroPython, visit this ESP32 MicroPython - Sound Sensor tutorial.
Hardware Used In This Tutorial
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to Sound Sensor
The sound sensor can be used to detect sound in the surrounding environment. There are two types of sound sensor module:
- Digital sound sensor module: outputs the digital signal value (ON/OFF)
- Analog sound sensor module: outputs both analog and digital signal value
The sensitivity of digital output can be addjusted by using a built-in potentiometer.
The Digital Sound Sensor Pinout
The digital sound sensor includes three pins:
- VCC pin: needs to be connected to VCC (3.3V to 5V)
- GND pin: needs to be connected to GND (0V)
- OUT pin: is an output pin: HIGH if quiet and LOW if sound is detected. This pin needs to be connected to ESP32's input pin.
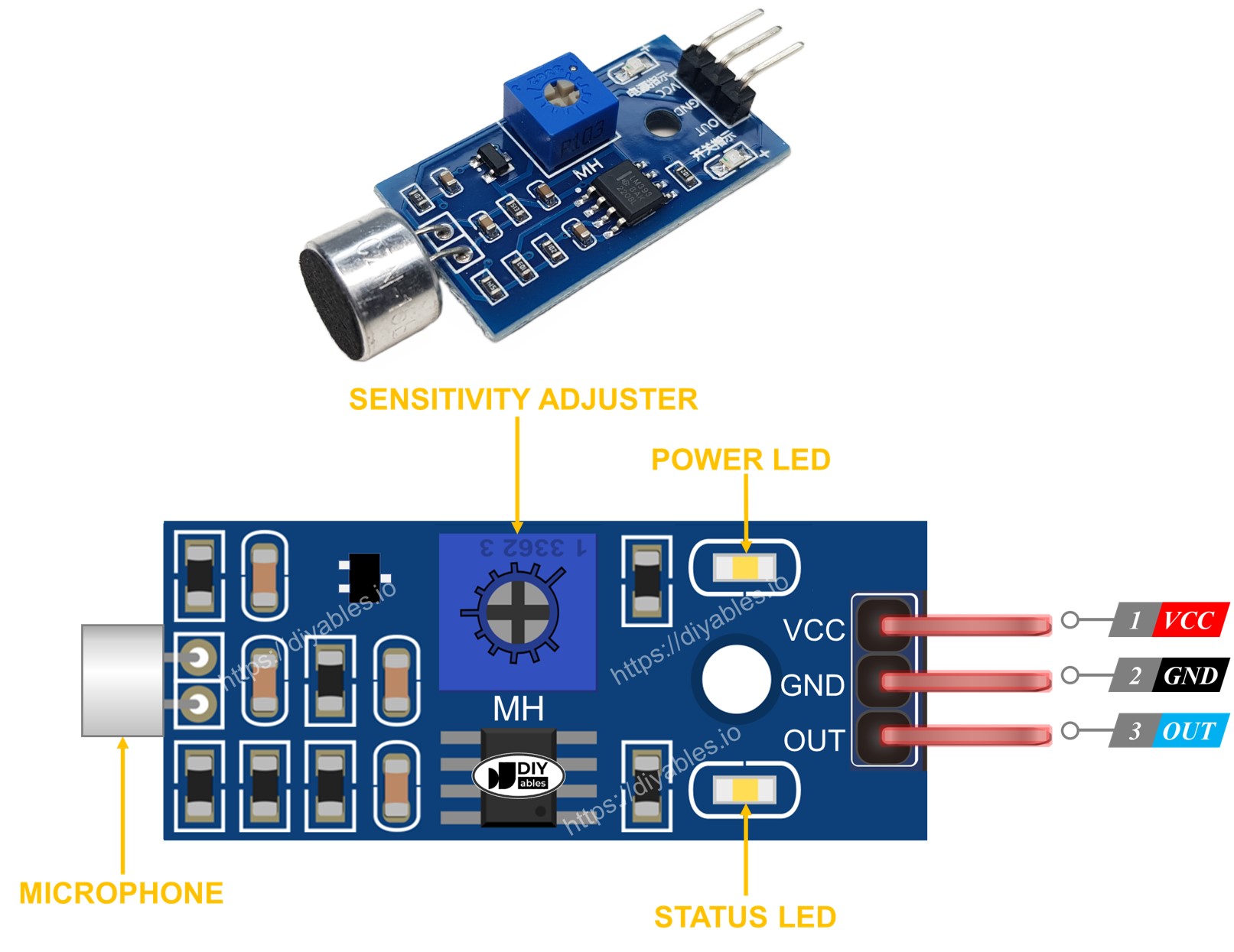
The sound sensor has a handy built-in potentiometer that lets you easily adjust its sensitivity. Additionally, it comes with two LED indicators:
- One LED indicator shows the power status.
- Another LED indicator indicates the sound state, turning on when sound is detected and off when it's quiet.
The Analog Sound Sensor Pinout
The analog sound sensor includes four pins:
- + pin: needs to be connected to 5V
- G pin: needs to be connected to GND (0V)
- DO pin: is a digital output pin: HIGH if quiet and LOW if sound is detected. This pin needs to be connected to ESP32's digital input pin.
- AO pin: is an analog output pin: outputs the analog value indicated sound level. This pin needs to be connected to ESP32's analog input pin.
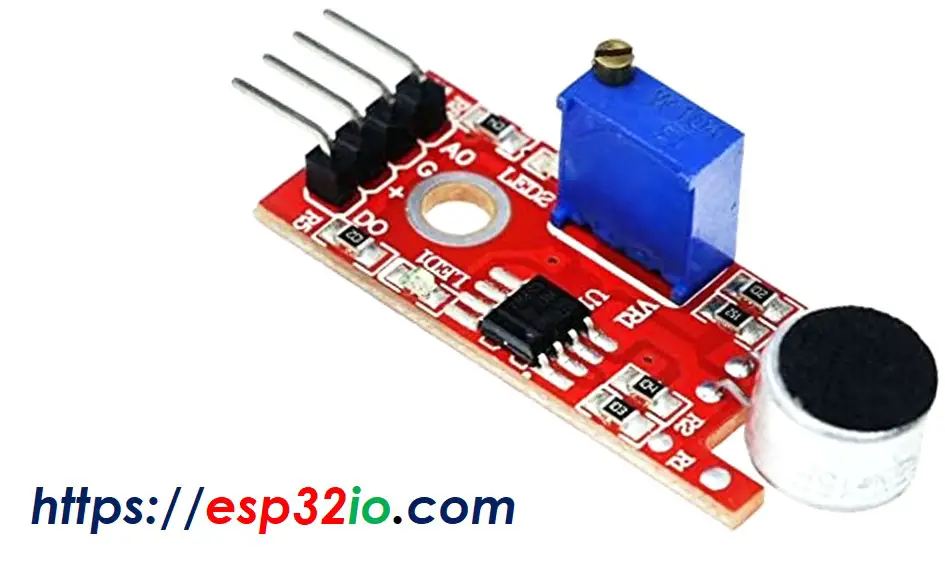
The analog sound sensor has a handy built-in potentiometer that lets you easily adjust its sensitivity for the digital output. Additionally, it comes with two LED indicators:
- One LED indicator shows the power status.
- Another LED indicator indicates the sound state, turning on when sound is detected and off when it's quiet.
How It Works
The module includes a convenient built-in potentiometer that allows you to adjust the sound sensitivity. Here's how the output pin of the sensor behaves:
- When sound is detected, the output pin is set to LOW.
- When sound is not detected, the output pin is set to HIGH.
Wiring Diagram
- How to connect ESP32 and sound sensor using breadboard
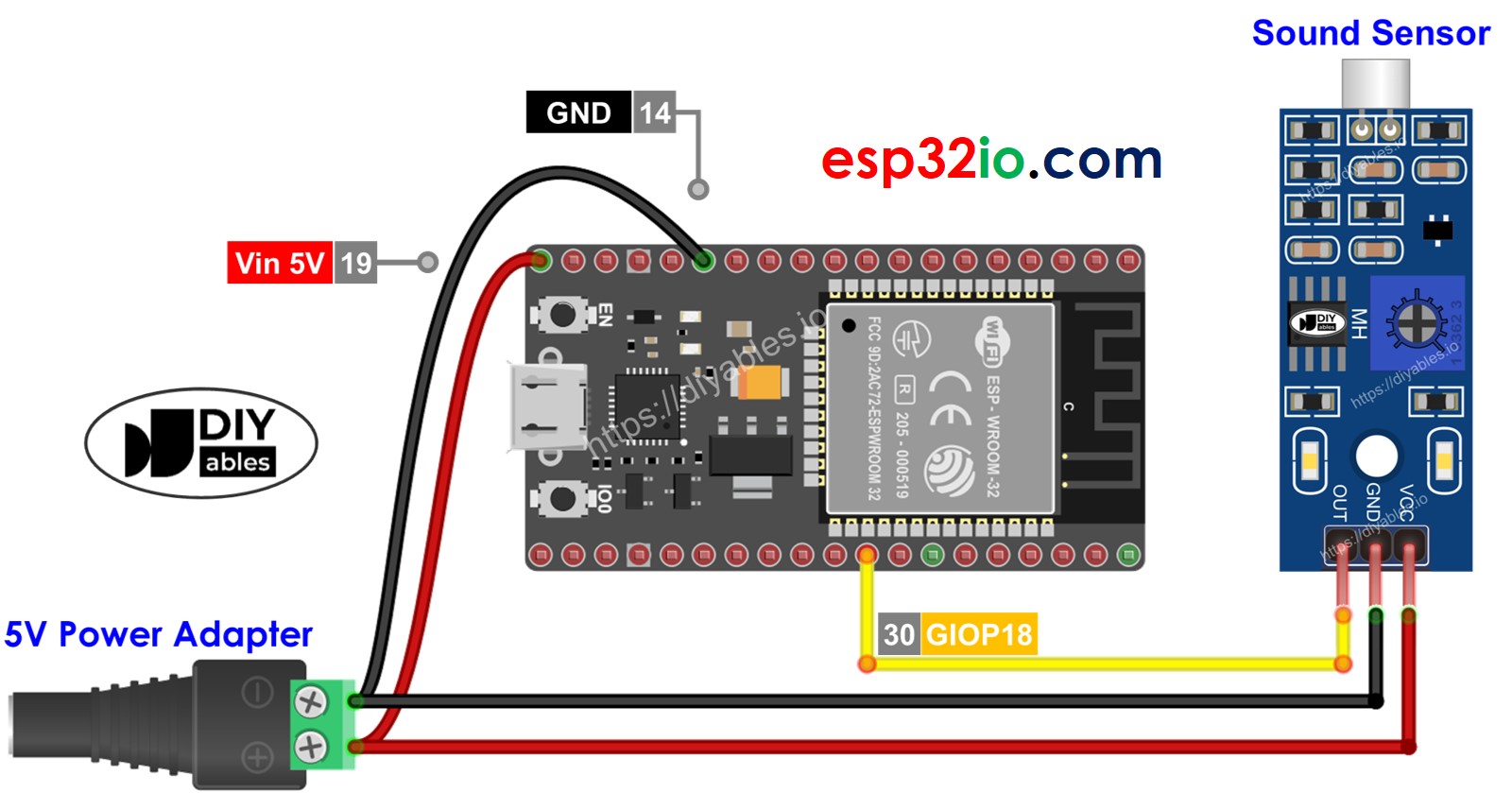
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and sound sensor using screw terminal block breakout board
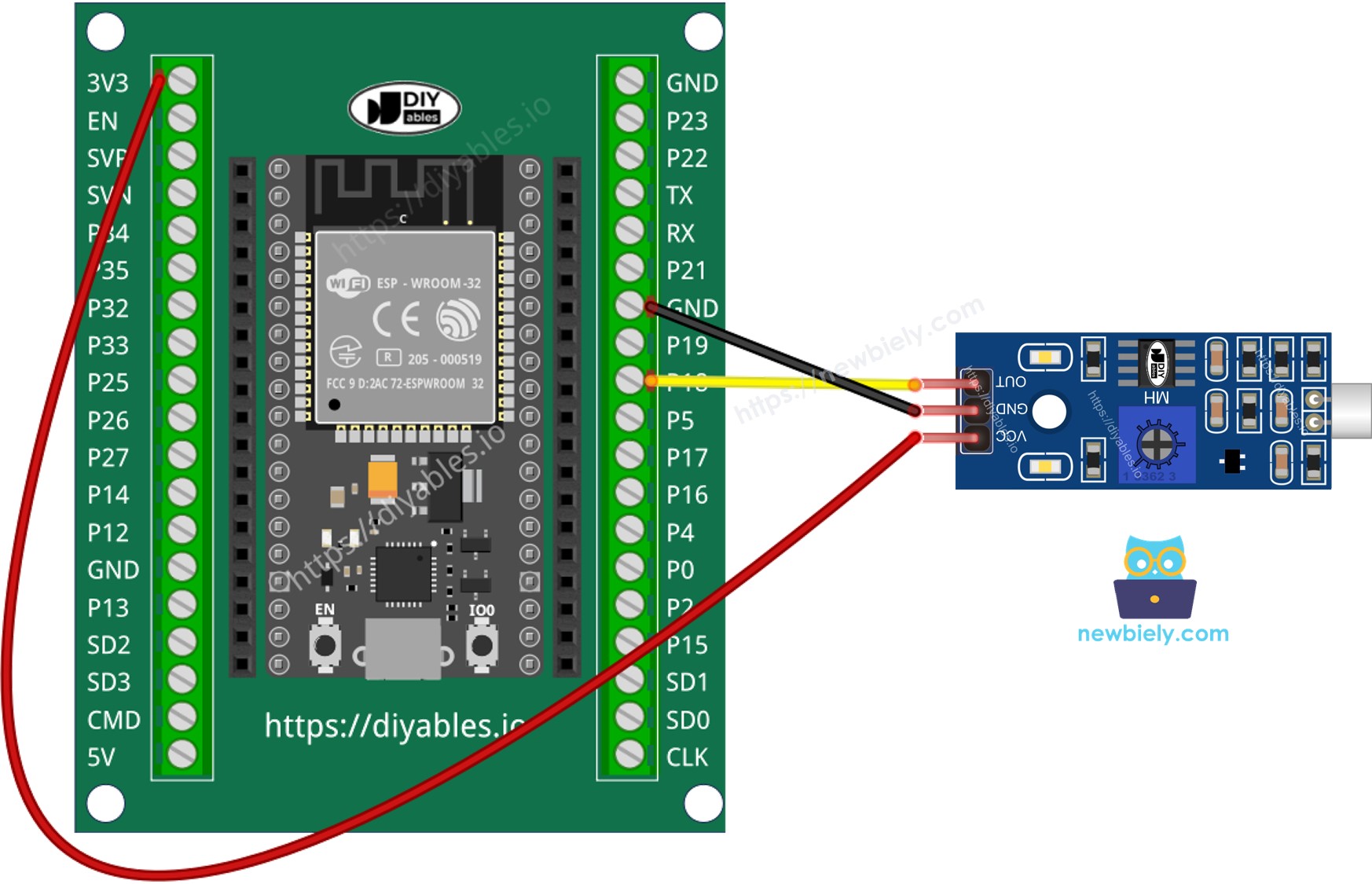
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
How To Program For Sound Sensor
- Initializes the ESP32 pin to the digital input mode by using pinMode() function. For example, pin GPIO18
- Reads the state of the ESP32 pin by using digitalRead() function.
ESP32 Code - Detecting the sound
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
- Clap your hand in front of the sound sensor
- See the result on Serial Monitor.
Please keep in mind that if you notice the LED status remaining constantly on or off, even when there is sound present, you may need to adjust the potentiometer to fine-tune the sound sensitivity of the sensor.
Now, we have the freedom to personalize the code and make it trigger an LED or a light when sound is detected. We can even make a servo motor rotate according to the sound input. For more detailed guidance and step-by-step instructions, you can refer to the tutorials provided at the end of this tutorial.
Troubleshooting
If you encounter any issues with the sound sensor's functionality, please consider the following troubleshooting steps:
- Reduce vibrations: Mechanical vibrations and wind noise can affect the sound sensor's performance. To minimize these disturbances, try mounting the sound sensor on a stable surface.
- Consider the sensing range: Keep in mind that this particular sound sensor has a limited sensing range of approximately 10 inches. For accurate readings, try producing sound closer to the sensor.
- Check the power supply: Ensure that the power supply is clean and free from noise, as the sound sensor is sensitive to power supply interference due to its analog nature.
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.