ESP32 - Multiple Button
This tutorial guides you on programming an ESP32 to utilize multiple buttons simultaneously without relying on the delay() function. The tutorial offers code in two methods:
We will demonstrate with four buttons. However, you can readily adjust the code for two buttons, three buttons, five buttons, or even more.
Or you can buy the following sensor kits:
Disclosure: some of these links are affiliate links. We may earn a commission on your purchase at no extra cost to you. We appreciate it.
We have specific tutorials about button. Each tutorial contains detailed information and step-by-step instructions about hardware pinout, working principle, wiring connection to ESP32, ESP32 code... Learn more about them at the following links:
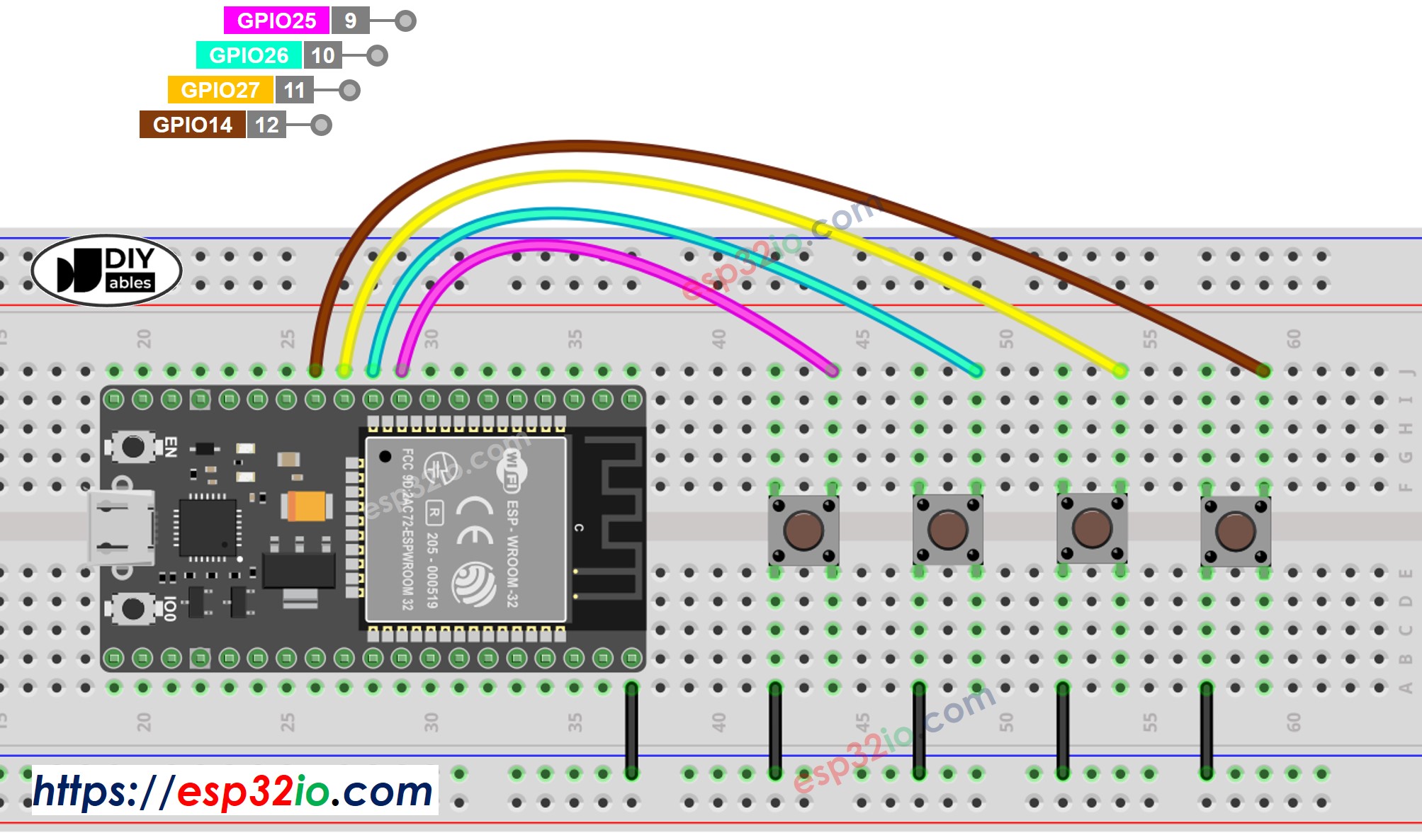
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: How to Power ESP32.
When dealing with multiple buttons, complexity can arise in specific situations:
Fortunately, the ezButton library simplifies this process by handling debounce and button events internally. This spares users from managing timestamps and variables when using the library. Moreover, employing an array of buttons can improve code clarity and brevity.
#include <ezButton.h>
#define BUTTON_PIN_1 25
#define BUTTON_PIN_2 26
#define BUTTON_PIN_3 27
#define BUTTON_PIN_4 14
ezButton button1(BUTTON_PIN_1);
ezButton button2(BUTTON_PIN_2);
ezButton button3(BUTTON_PIN_3);
ezButton button4(BUTTON_PIN_4);
void setup() {
Serial.begin(9600);
button1.setDebounceTime(100);
button2.setDebounceTime(100);
button3.setDebounceTime(100);
button4.setDebounceTime(100);
}
void loop() {
button1.loop();
button2.loop();
button3.loop();
button4.loop();
int button1_state = button1.getState();
int button2_state = button2.getState();
int button3_state = button3.getState();
int button4_state = button4.getState();
if (button1.isPressed())
Serial.println("The button 1 is pressed");
if (button1.isReleased())
Serial.println("The button 1 is released");
if (button2.isPressed())
Serial.println("The button 2 is pressed");
if (button2.isReleased())
Serial.println("The button 2 is released");
if (button3.isPressed())
Serial.println("The button 3 is pressed");
if (button3.isReleased())
Serial.println("The button 3 is released");
if (button4.isPressed())
Serial.println("The button 4 is pressed");
if (button4.isReleased())
Serial.println("The button 4 is released");
}
Do the wiring as above image.
Connect the ESP32 board to your PC via a micro USB cable
Open Arduino IDE on your PC.
Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
Do the wiring as above image.
Connect the ESP32 board to your PC via a USB cable
Open Arduino IDE on your PC.
Select the right ESP32 board (e.g. ESP32) and COM port.
Click to the Libraries icon on the left bar of the Arduino IDE.
Search “ezButton”, then find the button library by ESP32GetStarted
Click Install button to install ezButton library.
The button 1 is pressed
The button 1 is released
The button 2 is pressed
The button 2 is released
The button 3 is pressed
The button 3 is released
The button 4 is pressed
The button 4 is released
We can enhance the provided code by utilizing an array of buttons. The following code demonstrates how this array manages button objects.
#include <ezButton.h>
#define BUTTON_NUM 4
#define BUTTON_PIN_1 25
#define BUTTON_PIN_2 26
#define BUTTON_PIN_3 27
#define BUTTON_PIN_4 14
ezButton buttonArray[] = {
ezButton(BUTTON_PIN_1),
ezButton(BUTTON_PIN_2),
ezButton(BUTTON_PIN_3),
ezButton(BUTTON_PIN_4)
};
void setup() {
Serial.begin(9600);
for (byte i = 0; i < BUTTON_NUM; i++) {
buttonArray[i].setDebounceTime(100);
}
}
void loop() {
for (byte i = 0; i < BUTTON_NUM; i++)
buttonArray[i].loop();
for (byte i = 0; i < BUTTON_NUM; i++) {
int button_state = buttonArray[i].getState();
if (buttonArray[i].isPressed()) {
Serial.print("The button ");
Serial.print(i + 1);
Serial.println(" is pressed");
}
if (buttonArray[i].isReleased()) {
Serial.print("The button ");
Serial.print(i + 1);
Serial.println(" is released");
}
}
}
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.