ESP32 - Button Toggle LED
This tutorial instructs you how to use ESP32 to toggle the state of an LED between ON and OFF when a button is pressed. More specifically:
If the button is pressed, turn the LED on
If the button is pressed again, turn the LED off
The above process is repeated over and over again
The ESP32 codes contains code for a button toggles an LED without and with debouncing
Or you can buy the following kits:
Disclosure: Some of the links in this section are Amazon affiliate links, meaning we may earn a commission at no additional cost to you if you make a purchase through them. Additionally, some links direct you to products from our own brand,
DIYables .
We have specific tutorials about LED and button. Each tutorial contains detailed information and step-by-step instructions about hardware pinout, working principle, wiring connection to ESP32, ESP32 code... Learn more about them at the following links:
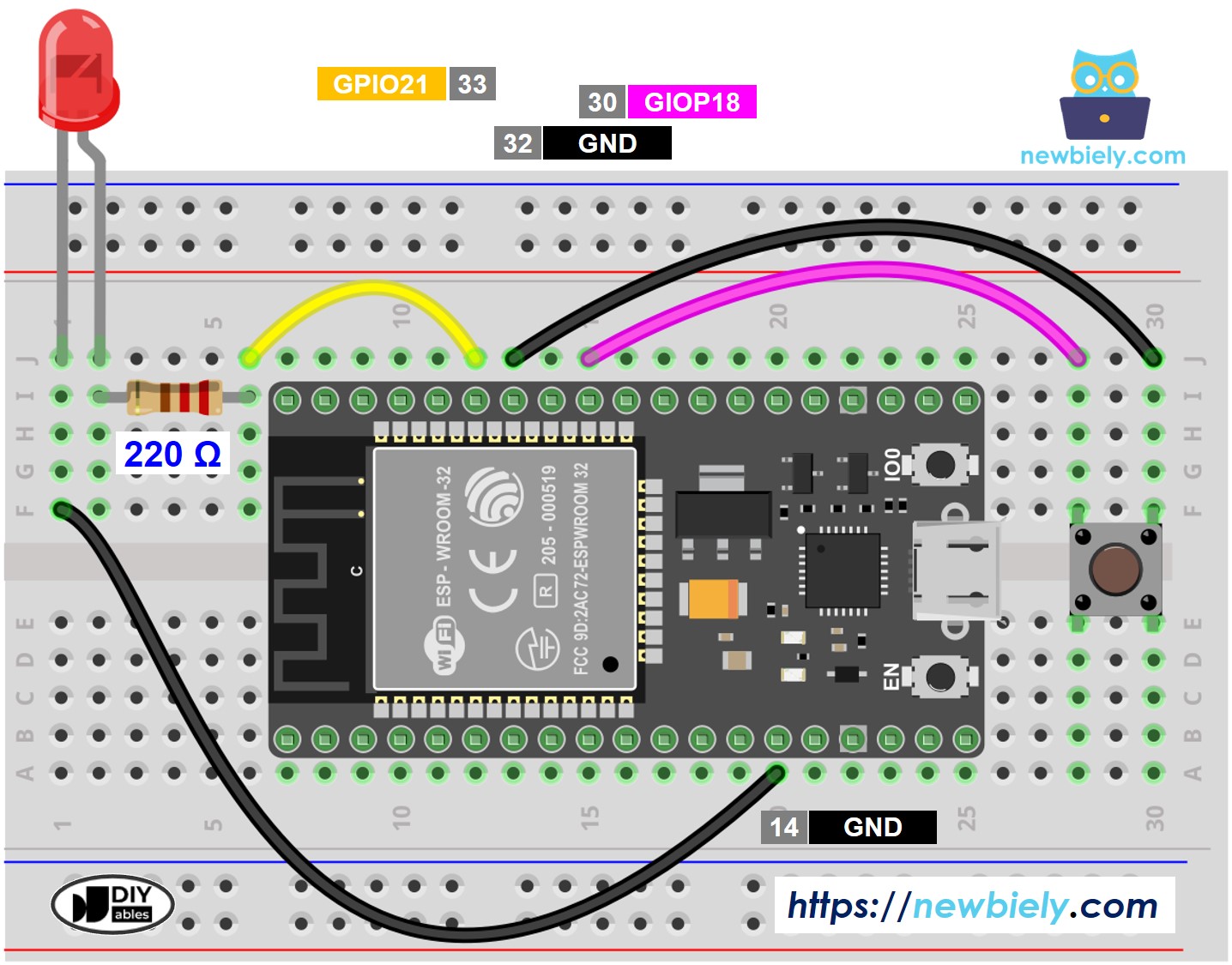
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
#define BUTTON_PIN 18
#define LED_PIN 21
int led_state = LOW;
int button_state;
int last_button_state;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
pinMode(LED_PIN, OUTPUT);
button_state = digitalRead(BUTTON_PIN);
}
void loop() {
last_button_state = button_state;
button_state = digitalRead(BUTTON_PIN);
if (last_button_state == HIGH && button_state == LOW) {
Serial.println("The button is pressed");
led_state = !led_state;
digitalWrite(LED_PIN, led_state);
}
}
Do the wiring as above image.
Connect the ESP32 board to your PC via a micro USB cable
Open Arduino IDE on your PC.
Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
Copy the above code and paste it to Arduino IDE.
Compile and upload code to ESP32 board by clicking Upload button on Arduino IDE
Keep pressing the button several seconds and then release it.
See the change on LED's state
The above ESP32 code contains line-by-line explanation. Please read the comments in the code!
In the code, led_state = !led_state is equivalent to the following code:
if(led_state == LOW)
led_state = HIGH;
else
led_state = LOW;
※ NOTE THAT:
The above code does not have the debounce code for button. Without debouncing for button, the unexpected behaviours can occur. See Why need debounce for button. Debouncing for the button is complicated for beginners. Fortunately, the ezButton library does the button debounce for us.
#include <ezButton.h>
#define BUTTON_PIN 18
#define LED_PIN 21
ezButton button(BUTTON_PIN);
int led_state = LOW;
void setup() {
Serial.begin(9600);
pinMode(LED_PIN, OUTPUT);
button.setDebounceTime(50);
}
void loop() {
button.loop();
if (button.isPressed()) {
Serial.println("The button is pressed");
led_state = !led_state;
digitalWrite(LED_PIN, led_state);
}
}
Install ezButton library. See
How To
Copy the above code and paste it to Arduino IDE.
Compile and upload code to ESP32 board by clicking Upload button on Arduino IDE
Press button several times
See the change on LED's state
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.