ESP32 - 74HC595 4-Digit 7-Segment Display
This tutorial guides you on using an ESP32 to control a 74HC595 4-digit 7-segment display module. It covers the following topics:
- Connecting ESP32 to the 74HC595 4-digit 7-segment display module
- Programming ESP32 to display integer numbers on the module
- Programming ESP32 to display float numbers on the module
- Programming ESP32 to display numbers and characters on the module.
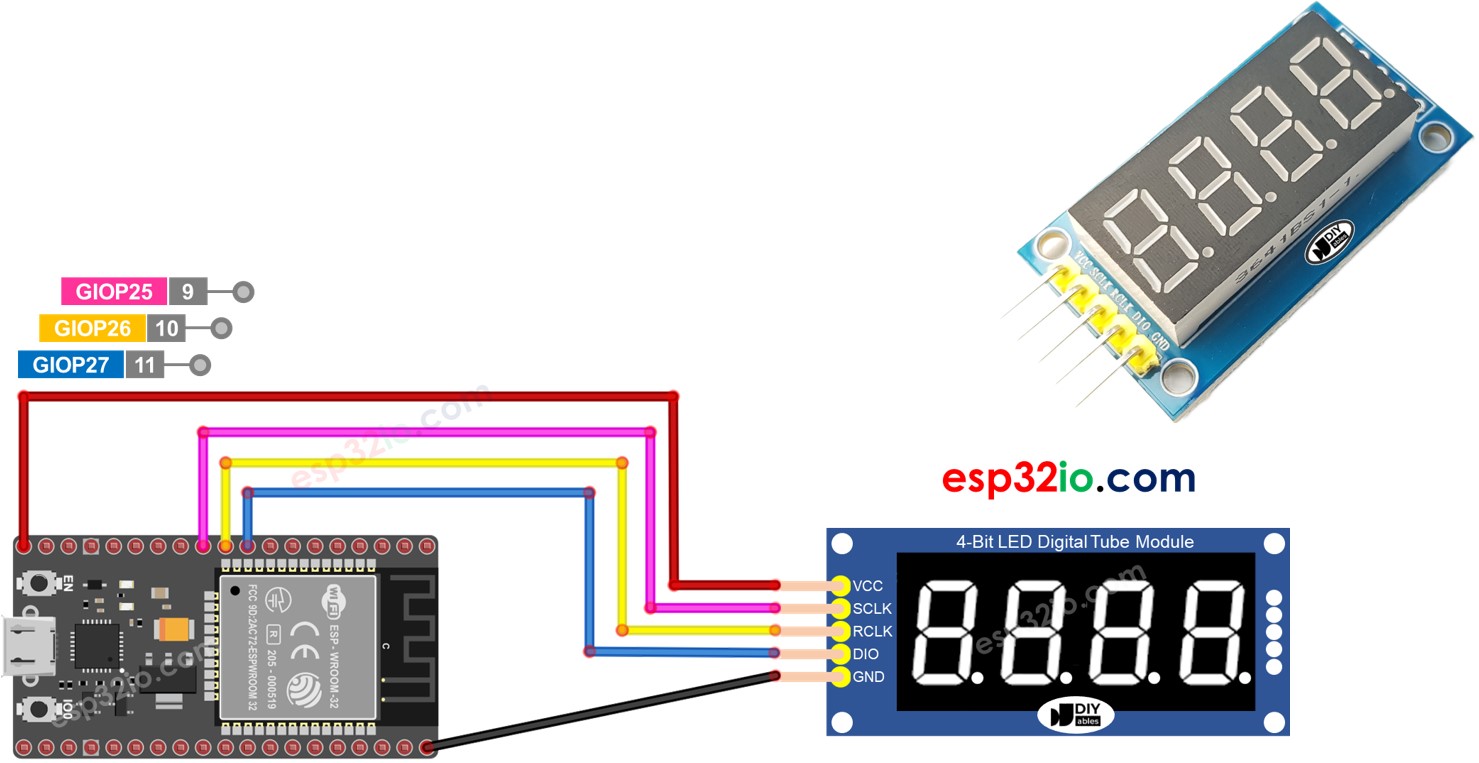
This tutorial will utilize a 4-dot 4-digit 7-segment display module capable of displaying float values. If you need to display a colon separator, please refer to the TM1637 4-digit 7-segment Display Module
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to 74HC595 4-digit 7-segment Display
An ideal module for displaying temperature or any float value is the 74HC595 4-digit 7-segment display. This module typically includes four 7-segment LEDs, four dot-shaped LEDs, and two 74HC595 drivers for each digit.
Pinout
The 74HC595 4-digit 7-segment display module includes 5 pins:
- SCLK pin: is a clock input pin. Connect to any digital pin on ESP32.
- RCLK pin: is a clock input pin. Connect to any digital pin on ESP32.
- DIO pin: is a Data I/O pin. Connect to any digital pin on ESP32.
- VCC pin: supplies power to the module. Connect it to the 3.3V to 5V power supply.
- GND pin: is a ground pin.
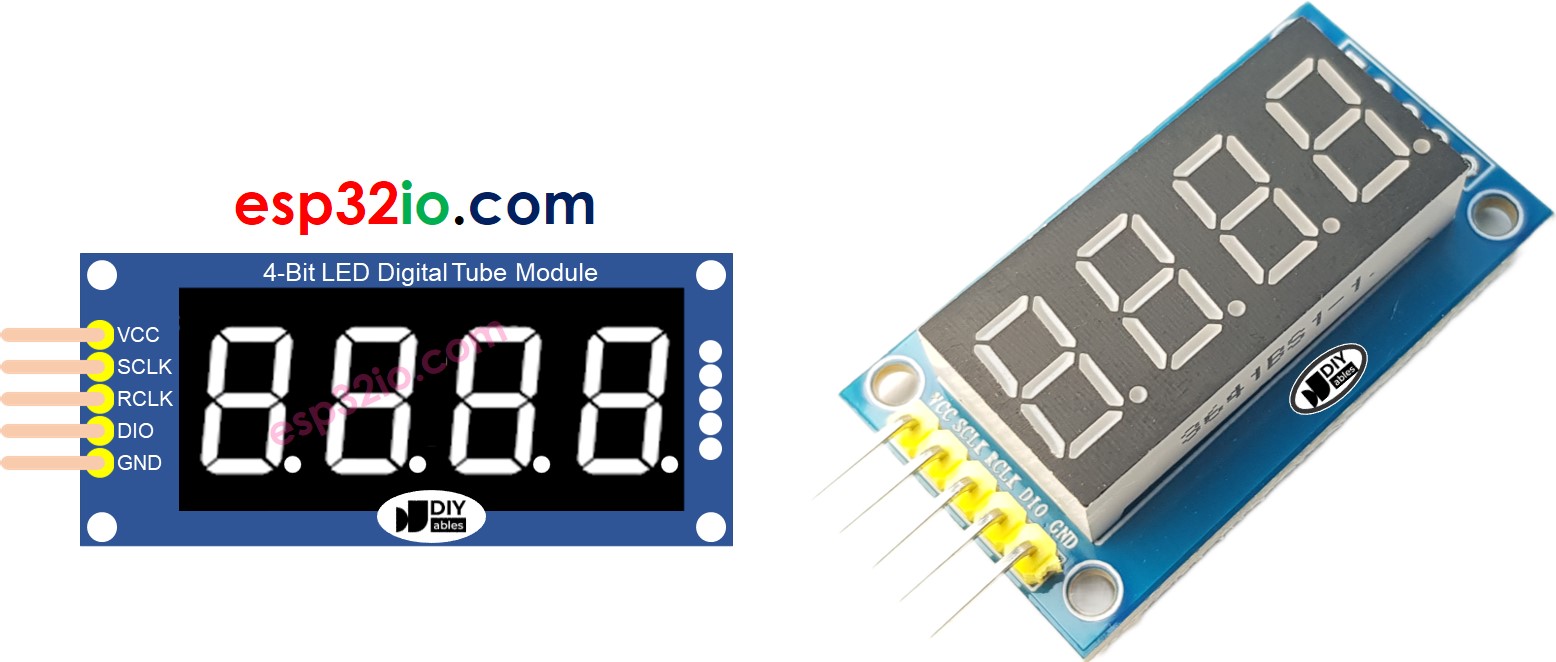
Wiring Diagram
The table below shown the wiring between ESP32 pins and a 74HC595 4-digit 7-segment display pins:
ESP32 | 74HC595 7-segment display |
---|---|
Vin | 5V |
27 | SCLK |
26 | RCLK |
25 | DIO |
If you are using different pins, make sure to modify the pin numbers in the code accordingly.
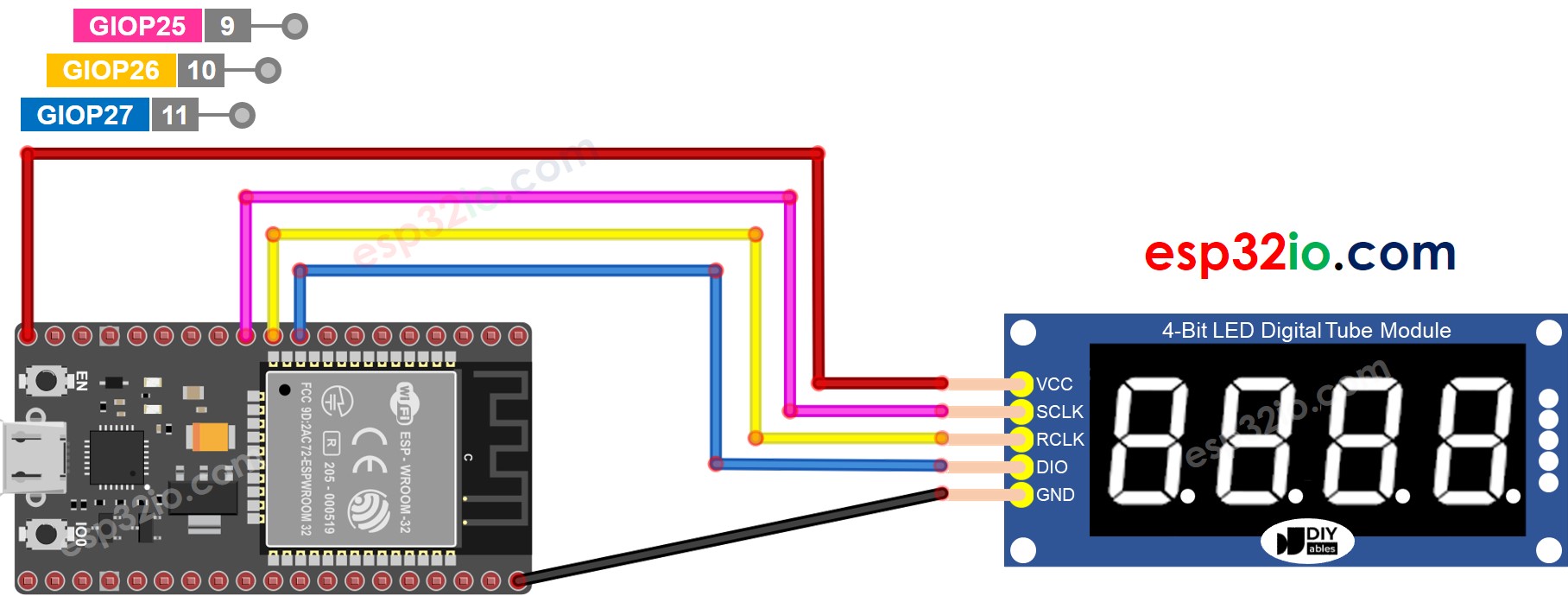
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
Library Installation
To program easily for 74HC595 4-digit 7-segment Display, we need to install DIYables_4Digit7Segment_74HC595 library by DIYables.io. Follow the below steps to install the library:
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search “DIYables_4Digit7Segment_74HC595”, then find the DIYables_4Digit7Segment_74HC595 library by DIYables.io
- Click Install button.
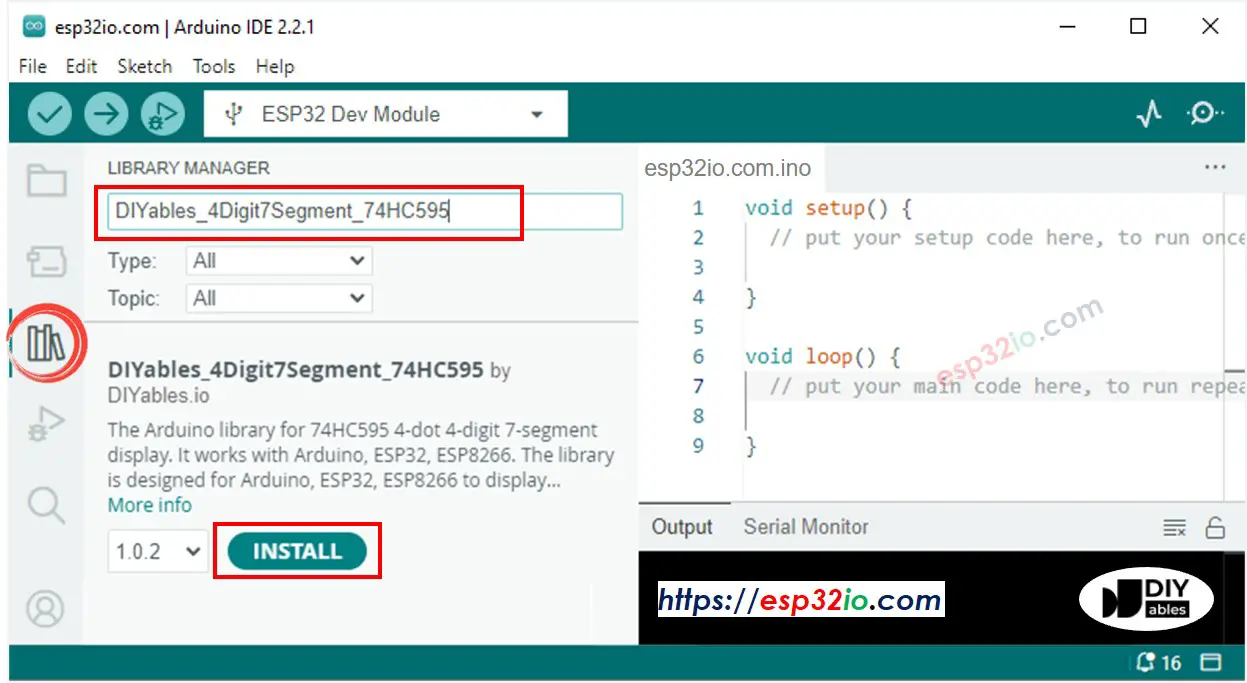
You can also see this library on Github
How To Program For 74HC595 4-digit 7-segment using ESP32
- Include the library
- Define ESP32's pins that connects to SCLK, RCLK and DIO of the display module. For example, pin D7, D6 and D5
- Create a display object of type DIYables_4Digit7Segment_74HC595
- Then you can display the integer numbers with the zero-padding option, supporting the negative number:
- You can display the float numbers with the decimal place, zero-padding options, supporting the negative number:
- You can also display number, decimal point, character digit-by-digit by using lower-level functions:
- Because the 74HC595 4-digit 7-segment module uses the multiplexing technique to control individual segments and LEDs, ESP32 code MUST:
- Call display.show() function in the main loop
- Not use delay() function in the main loop
You can see more detail in the the library reference
ESP32 Code - Display Integer
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Copy the above code and open with ESP32 IDE
- Click Upload button on ESP32 IDE to upload code to ESP32
- See the states of the 7-segment display
ESP32 Code - Display Float
ESP32 Code - Display Temperature
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.