ESP32 - Touch Sensor
This tutorial instructs you how to use ESP32 with the touch sensor (also known as touch switch, or touch button).
This tutorial shows how to program the ESP32 using the Arduino language (C/C++) via the Arduino IDE. If you’d like to learn how to program the ESP32 with MicroPython, visit this ESP32 MicroPython - Touch Sensor tutorial.
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to Touch Sensor
Touch Sensor Pinout
Touch sensor has 3 pins:
- GND pin: connect this pin to GND (0V)
- VCC pin: connect this pin to VCC (5V or 3.3v)
- SIGNAL pin: is an output pin: LOW when NOT touched, HIGH when touched. This pin needs to be connected to ESP32's input pin.
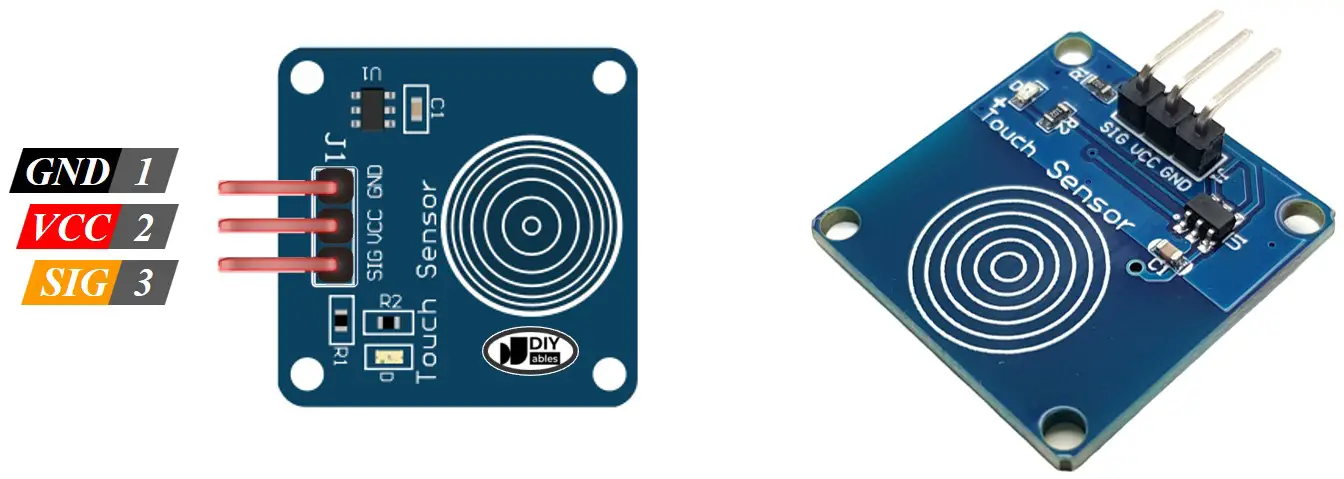
How Touch Sensor Works
- The state of the SIGNAL pin is LOW when the touch sensor is NOT touched
- The state of the SIGNAL pin is HIGH when the touch sensor is touched
ESP32 - Touch Sensor
We can connect the touch sensor's SIGNAL pin to an ESP32's input pin and use the ESP32 code to read the state of touch sensor.
Wiring Diagram between Touch Sensor and ESP32
- How to connect ESP32 and touch sensor using breadboard
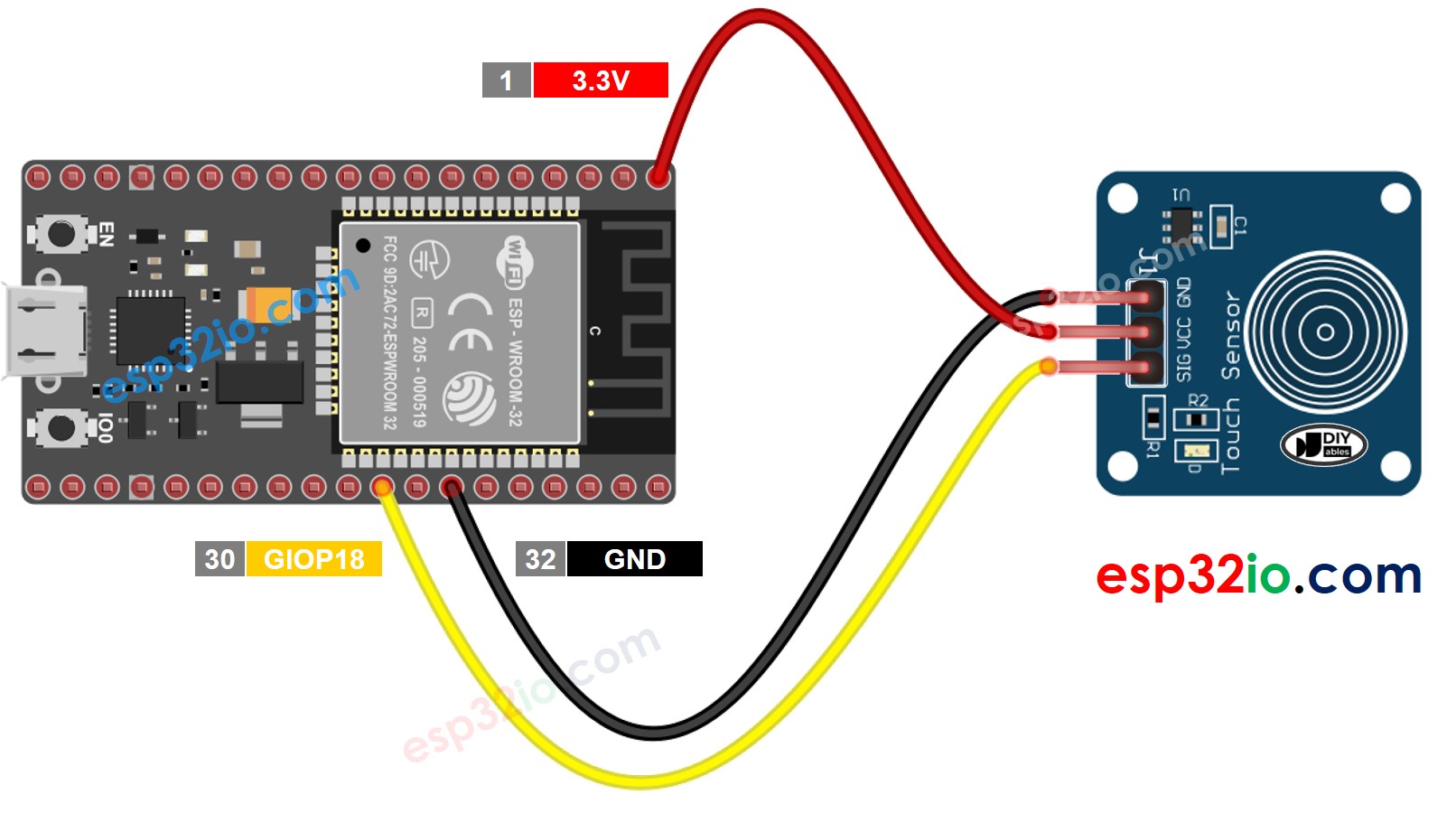
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and touch sensor using screw terminal block breakout board
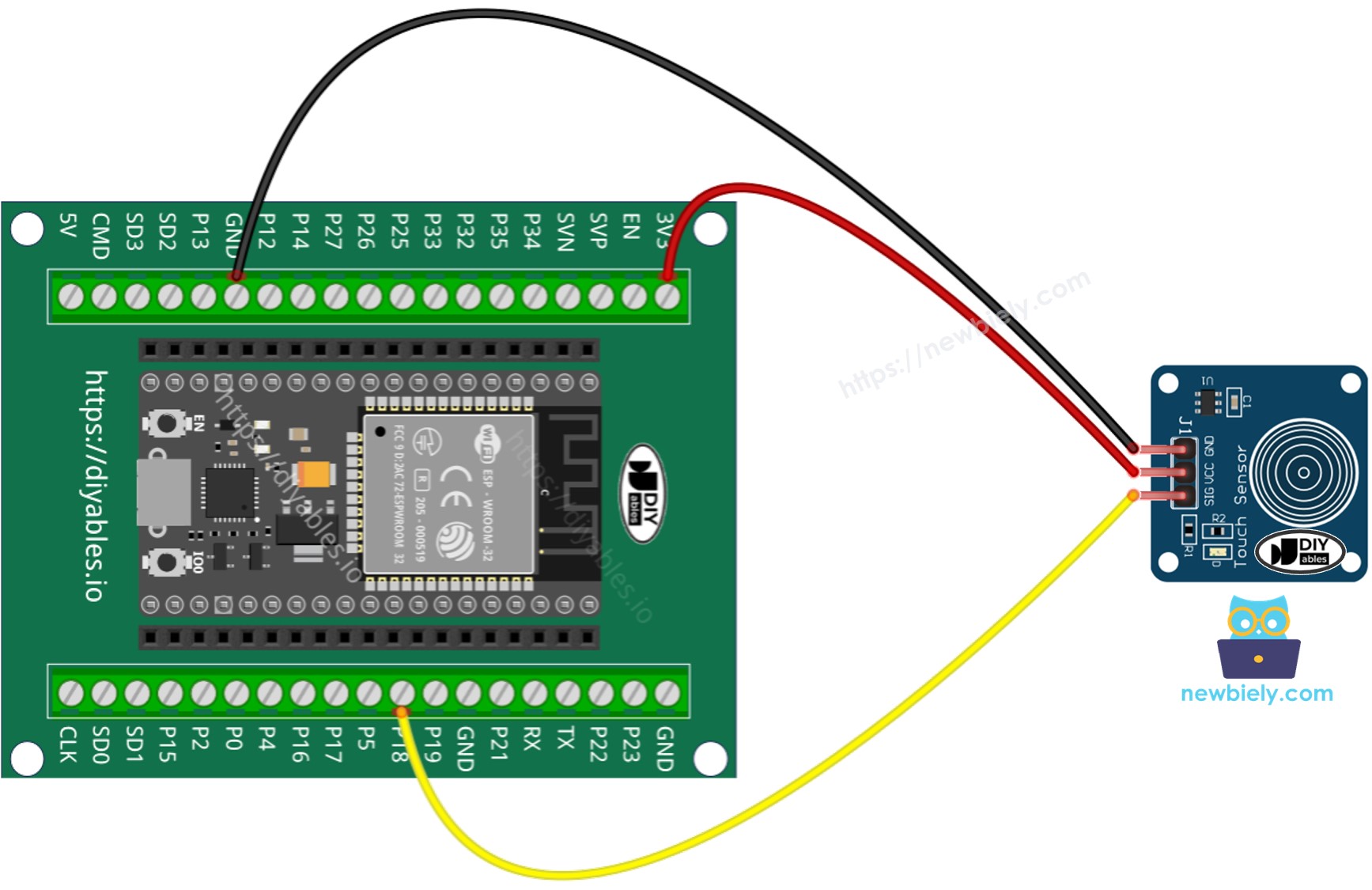
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
How To Program Touch Sensor
- Initializes the ESP32 pin to the digital input mode by using pinMode() function. For example, pin GPIO18
- Reads the state of the ESP32 pin by using digitalRead() function.
Touch Sensor - ESP32 Code
The below code reads the state of the touch sensor and print it to the Serial Monitor.
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Copy the above code and paste it to Arduino IDE.
- Compile and upload code to ESP32 board by clicking Upload button on Arduino IDE
- Touch your finger to the sensor and release.
- See the result on Serial Monitor. It looks like the below:
How to detect the state change from LOW to HIGH
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.