ESP32 - Door Lock System using Password
This tutorial instructs you how to use ESP32, Keypad and electromagnetic lock to make door lock system. Users will be ask for password via keypad. It the password is valid, the ESP32 controls electromagnetic lock to unlock the door. The tutorials also optionally adds an LCD for more convenience. The ESP32 code aslo supports multiple passwords.
Hardware Used In This Tutorial
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to Keypad and Electromagnetic Lock and LCD
We have specific tutorials about kypad, electromagnetic lock and LCD. Each tutorial contains detailed information and step-by-step instructions about hardware pinout, working principle, wiring connection to ESP32, ESP32 code... Learn more about them at the following links:
How check the password
- A special key * is used to reset the password
- A special key # is used to terminate the password input.
- Every inputed key, except for * and # is appended in to a string.
- When # key is pressed, the password input is complete. The ESP32 compares the inputed string with predefined passwords.
- If the inputed password is vaild, unlock the door.
Wiring Diagram
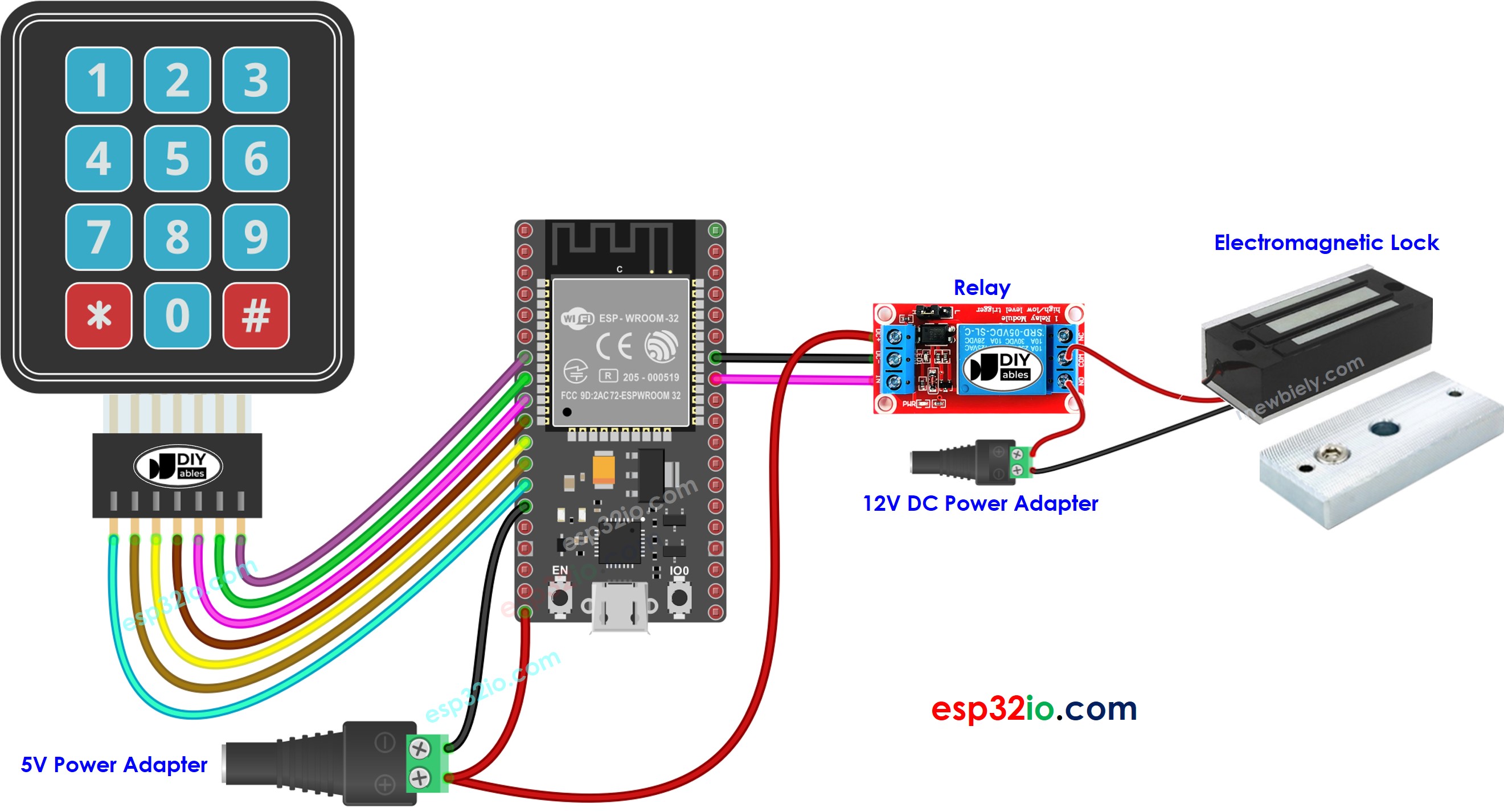
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: How to Power ESP32.
ESP32 Code - Door lock system with password using keypad, electromagnetic lock
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Type “keypad” on the search box, then look for the keypad library by Mark Stanley, Alexander Brevig
- Click Install button to install keypad library.
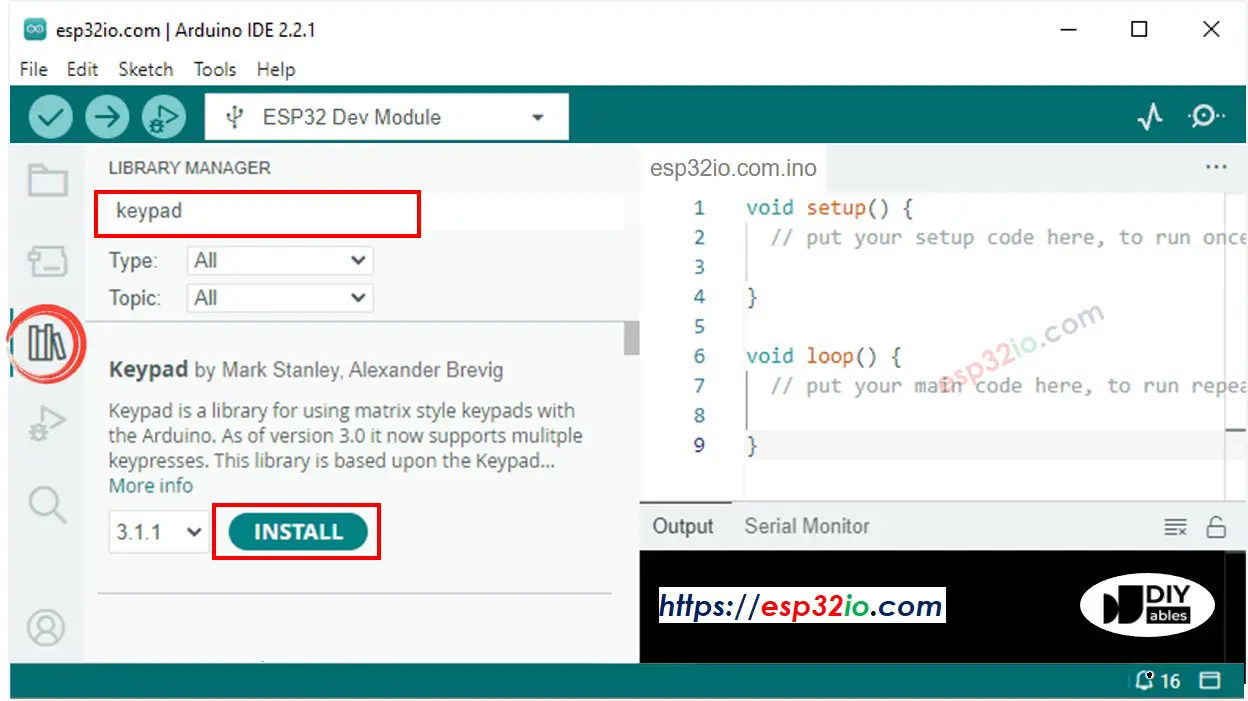
- Copy the above code and paste it to Arduino IDE.
- Compile and upload code to ESP32 board by clicking Upload button on Arduino IDE
- To simulate the electromagnet lock installed on the door, place the electromagnet near the armature plate.
- Press 1111 keys and press #
- Check the attraction force between electromagnet and armature plate.
- Press 1234 keys and press #
- Check the attraction force between electromagnet and armature plate.
- See the result on Serial Monitor
ESP32 Code - Door lock system with password using keypad, electromagnetic lock and LCD
The below code added LCD I2C to display the access status. For wiring with LCD, you can see in this tutorial Esp32 - LCD I2C tutorial
※ NOTE THAT:
The LCD I2C address can be different from each manufacturer. In the code, we used address of 0x27 that is specified by DIYables manufacturer
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.