ESP32 - LED Matrix
LED matrix display, also known as LED display, or dot matrix display, are wide-used. In this tutorial, we are going to learn:
- LED matrix
- How to connect ESP32 to 8x8 LED matrix
- How to connect ESP32 to 32x8 LED matrix
- How to connect ESP32 to display text, numbers, and animated effects on the LED matrix.
After that, you can easily adapt the code for other LED matrices such as 16x8 LED matrix, 64x8 LEd matrix ...
This tutorial shows how to program the ESP32 using the Arduino language (C/C++) via the Arduino IDE. If you’d like to learn how to program the ESP32 with MicroPython, visit this ESP32 MicroPython - LED Matrix tutorial.
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to LED Matrix
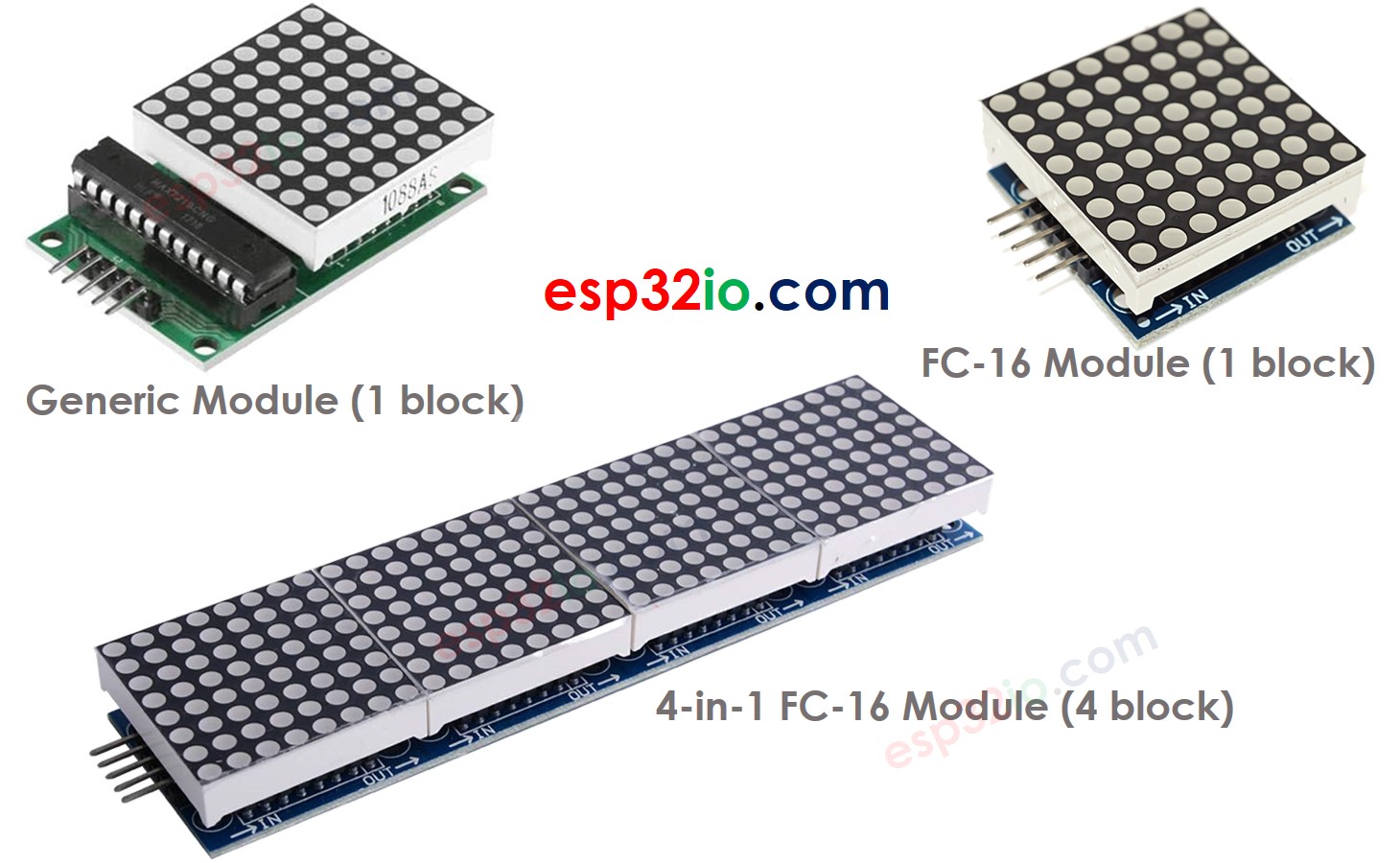
There are many kinds of LED Matrix. With ESP32, the MAX7219-based LED matrix is widely used. MAX7219-based LED matrix has the following features:
- A base unit of an LED matrix is a block
- Each block has an 8x8 LED matrix (64 LED) and a MAX7219 driver.
- There are two popular block forms: the generic module and the FC-16 module.
- A LED matrix can be composed of a single block or multiple blocks in a daisy-chain
- You can buy a pre-built multiple-block LED Matrix (e.g. 4-in-1, 8-in-1)
- You can also buy multiple blocks and wire them to form a LED matrix with the desired size.
- You will declare the size of the LED matrix you use in the ESP32 code.
Pinout
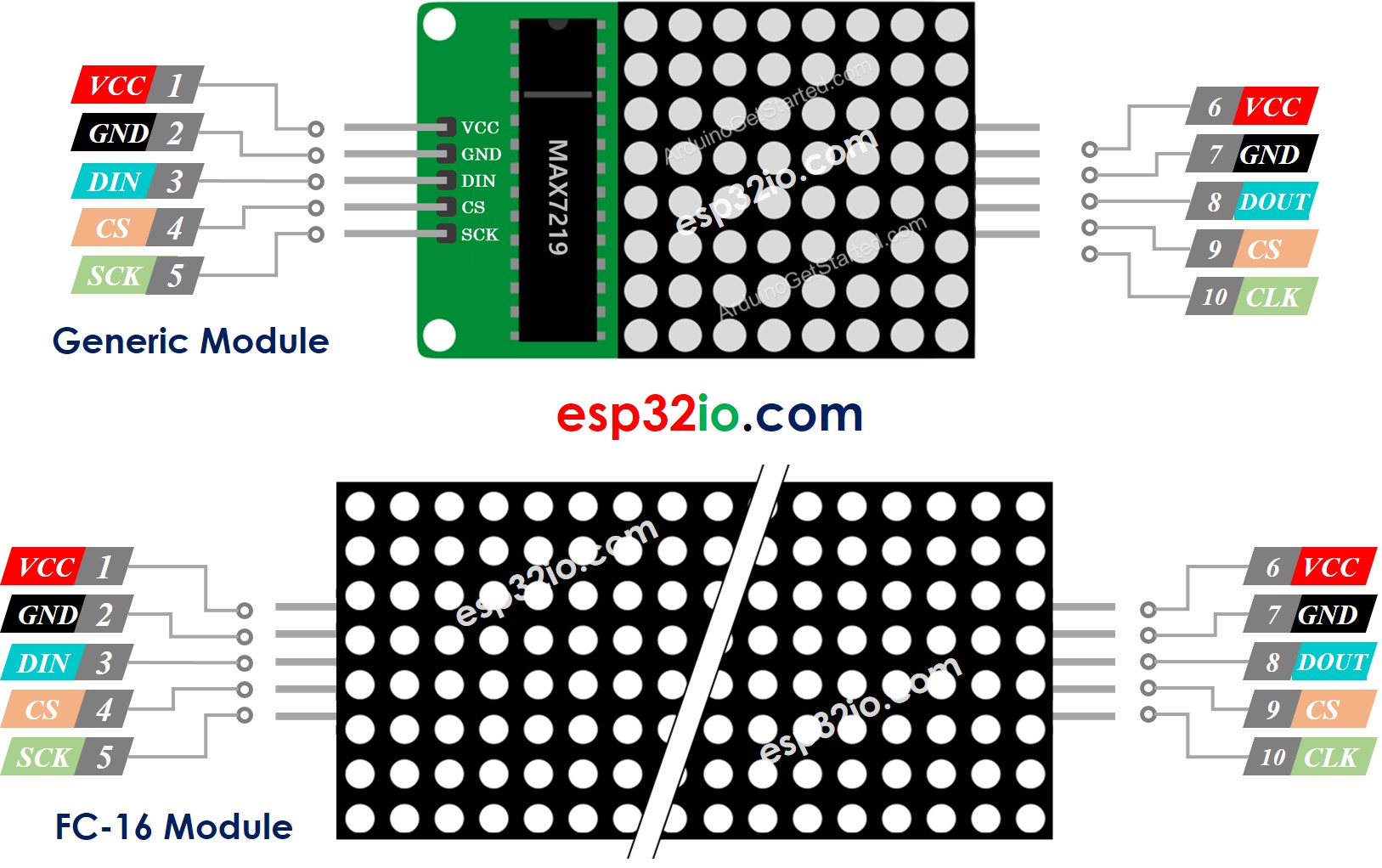
A LED Matrix is formed by a single or multiple blocks. Each block includes two groups of pins:
- Input pins group:
- VCC: connects to 5V.
- GND: connects to GND.
- DIN is the Data pin, Connect it to any digital pin of the ESP32.
- CS: Chip Select, Connect it to any digital pin of the ESP32.
- CLK: Clock pin, Connect it to any digital pin of the ESP32.
- Output pins group:
- VCC: connects to VCC of the input pins group on the next module.
- GND: connects to GND of the input pins group on the next module.
- DOUT: Data Out, connects to the DIN pin of the input pins group of the next module.
- CS: connects to CS of the input pins group on the next module.
- CLK connects to CLK of the input pins group on the next module.
Wiring Diagram
If the LED matrix is made of a single block:
- Connect the input pins groups to ESP32
- Let the output pins group unconnected
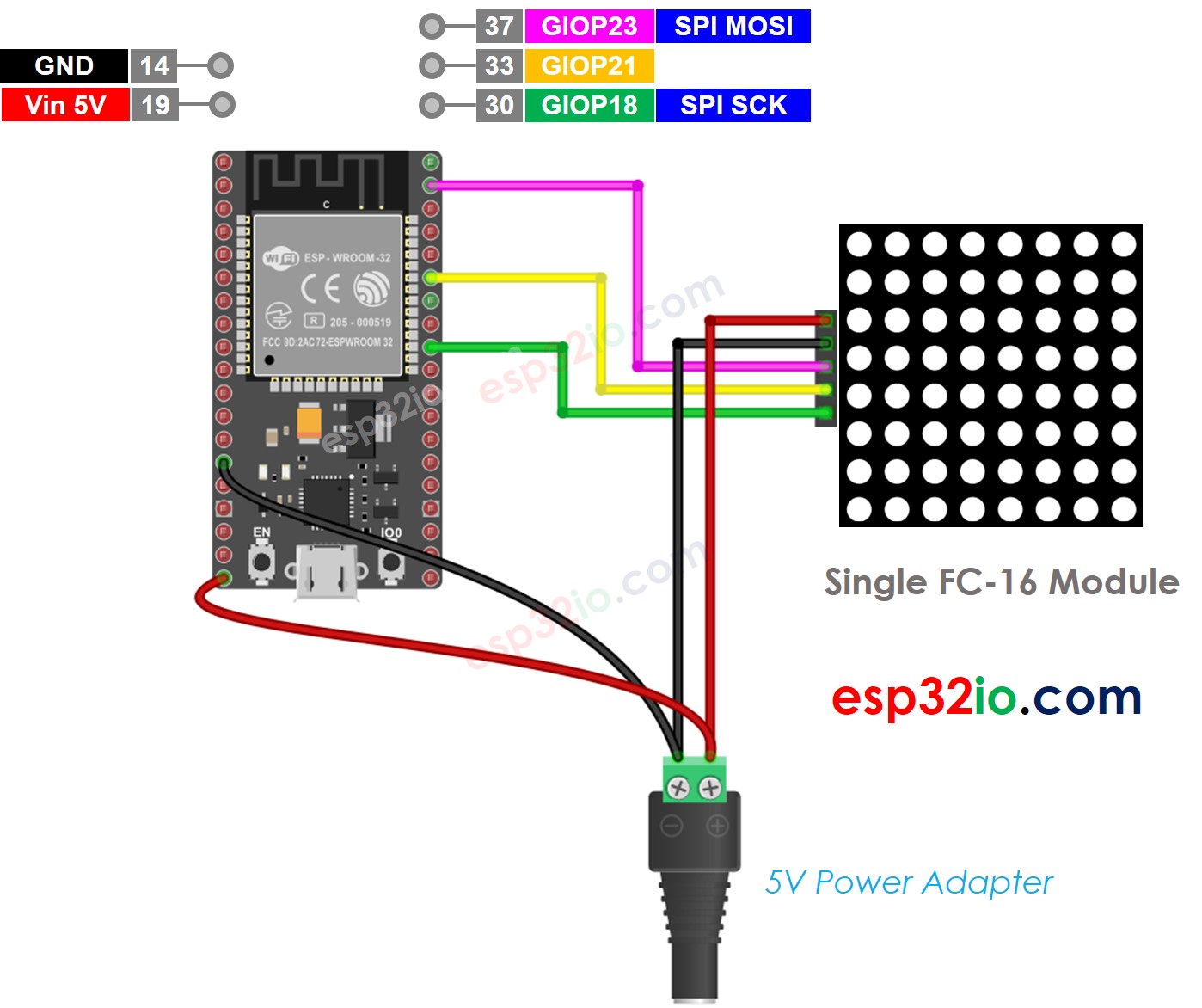
This image is created using Fritzing. Click to enlarge image
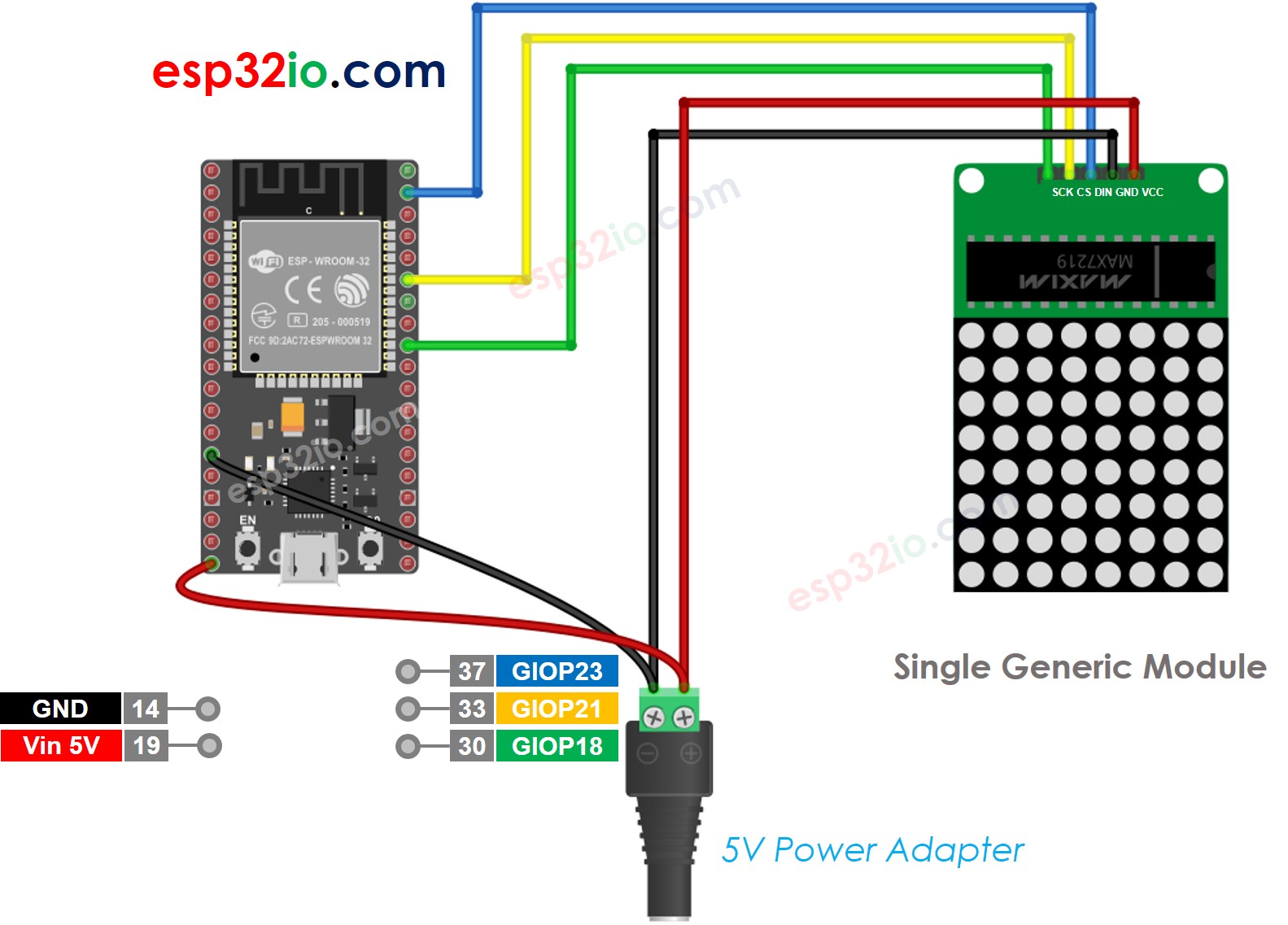
This image is created using Fritzing. Click to enlarge image
If the LED matrix is pre-built multiple blocks:
- Connect the input pins groups to ESP32
- Let the output pins group unconnected
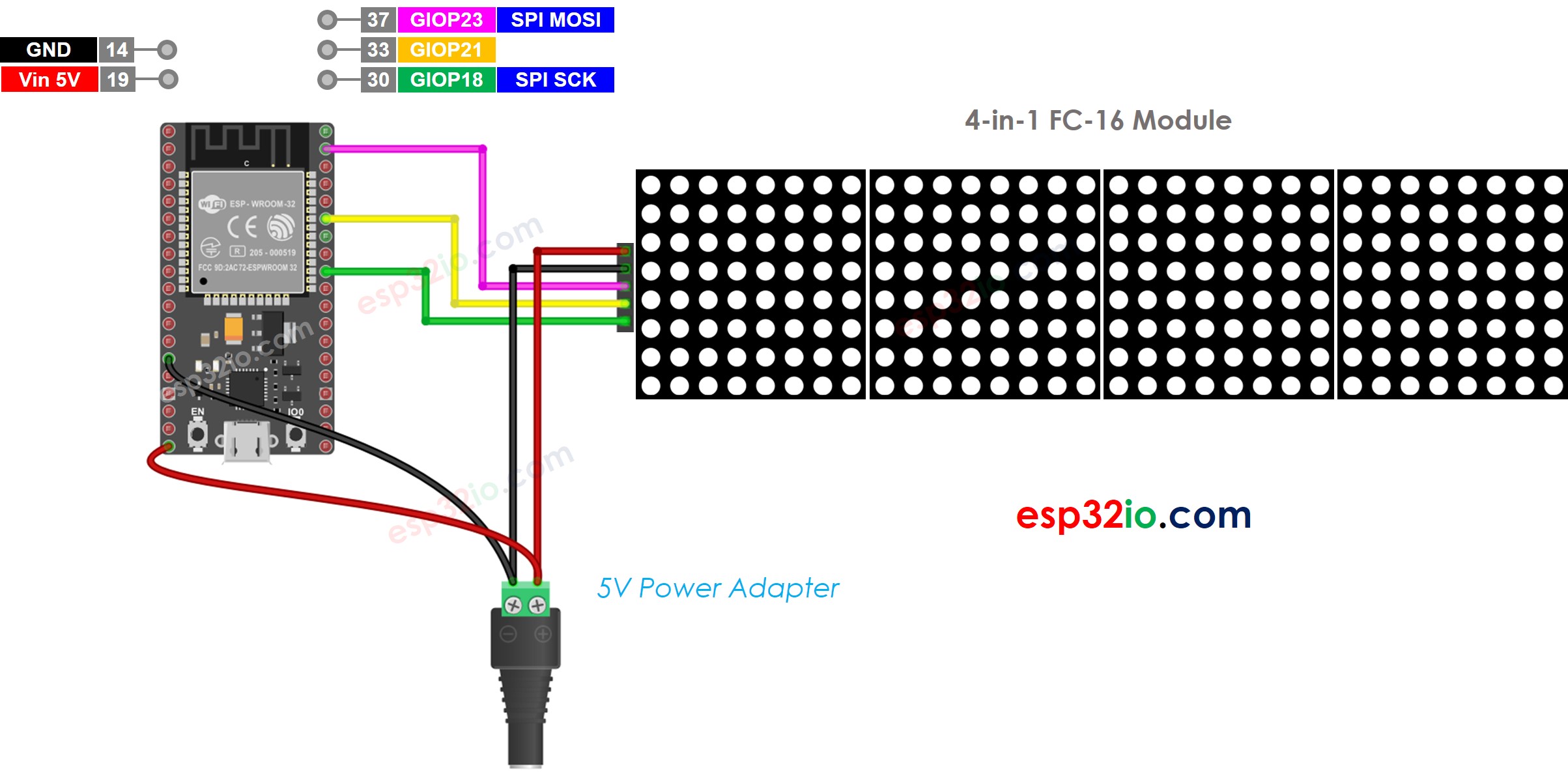
This image is created using Fritzing. Click to enlarge image
If the LED matrix is made of multiple blocks by yourself:
- Connect the input pins groups of the first block to ESP32
- Connect the output pins groups of each block to the input pins groups of the next block
- Let the output pins group of the last block unconnected
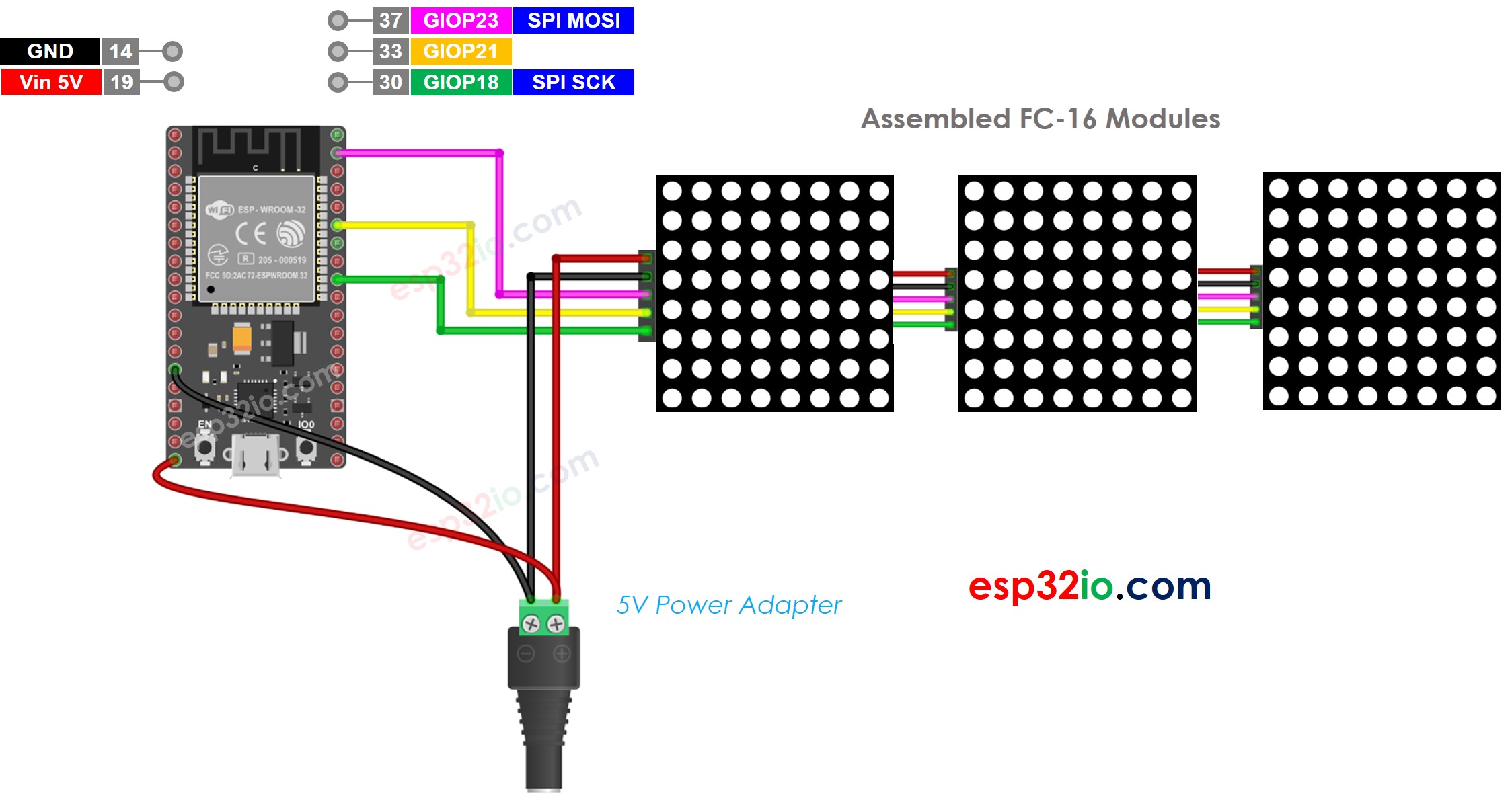
This image is created using Fritzing. Click to enlarge image
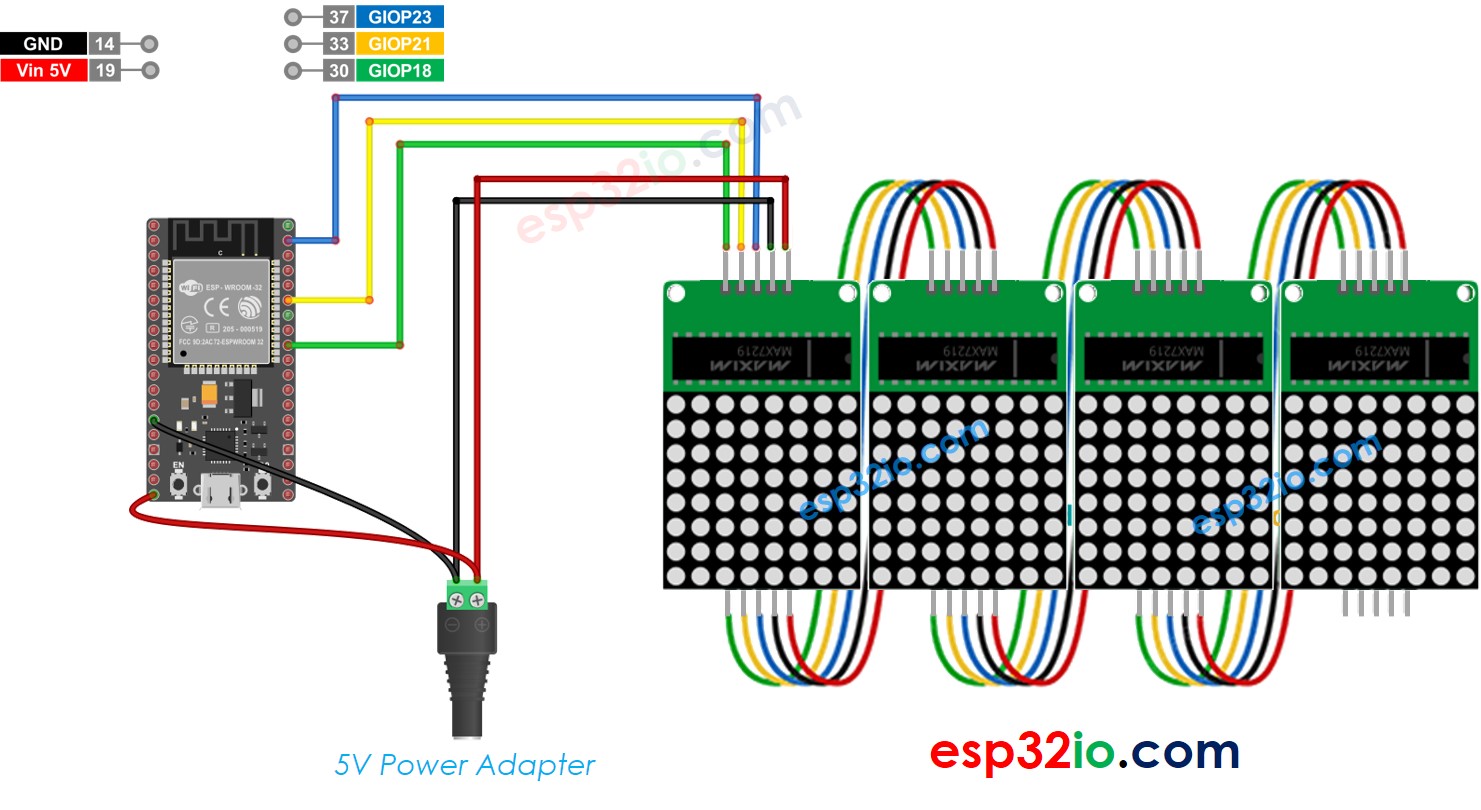
This image is created using Fritzing. Click to enlarge image
Because the display draws a lot of currents (up to 1A at maximum brightness):
- Do not use the power from the 5V pin of ESP32.
- Use an external 5V power supply instead. ESP32 and LED matrix can share power from a 5V power adapter
Because ESP32 connects to LED matrix via SPI pins:
- Pin GPIO18 (SCK) and GPIO23 (MOSI) on ESP32
- Pin GPIO21 (CS) can be changed to any pin
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
How To Program For LED Matrix
It is not easy to control the LED matrix. Fortunately, libraries are available to make it easy. Below is a step-by-step on how to write ESP32 code to control the LED matrix
- Include libraries:
- Specify which hardware is being used: GENERIC_HW or FC16_HW.
- Define how many LED block is used. for example, a 4-in-1 LED matrix has 4 blocks.
- Define the pin that connects to the LED matrix’s CS pin. For example, pin GPIO21
- Create a new instance of the MD_Parola class for the LED matrix display.
- Code in the setup() function:
- Display text, number and show animated effects: see next part
ESP32 - LED Matrix Code
The below code is for 32x8 FC-16 LED matrix display (4 blocks). But you can easily adapt it for 8x8, 16x8, 64x8...
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search “MD_Parola”, then find the MD_Parola library
- Click Install button.
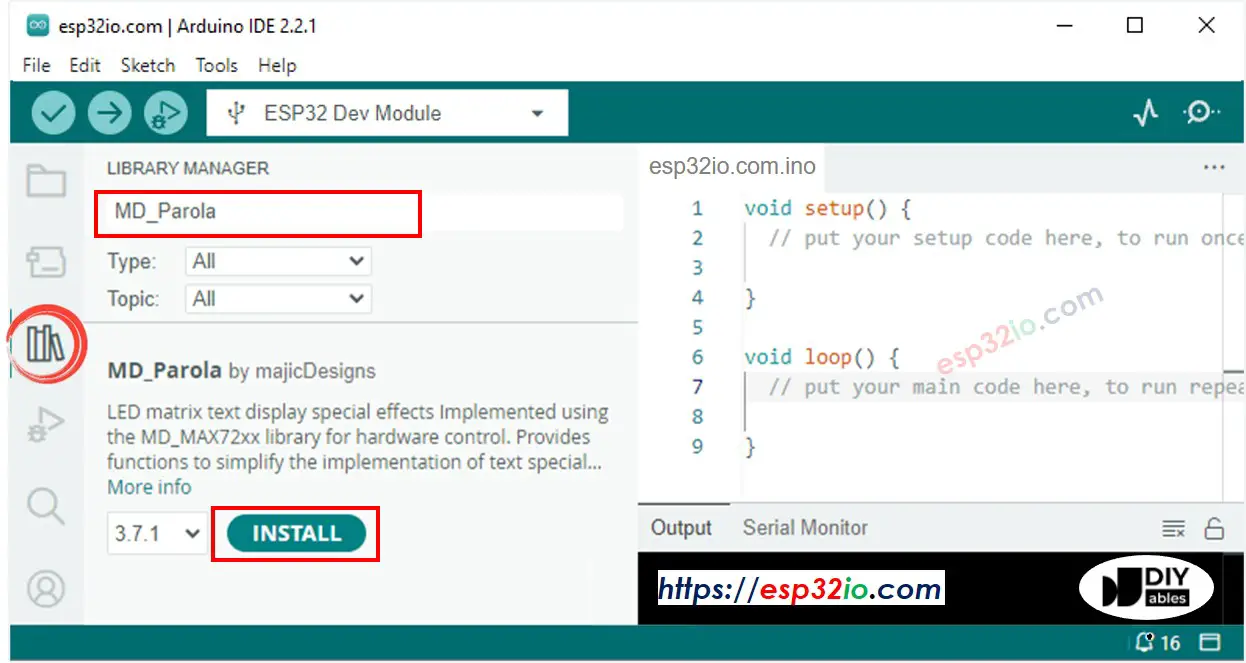
- You will be asked to install the “MD_MAX72XX” library
- Click Install All button to install the dependency.
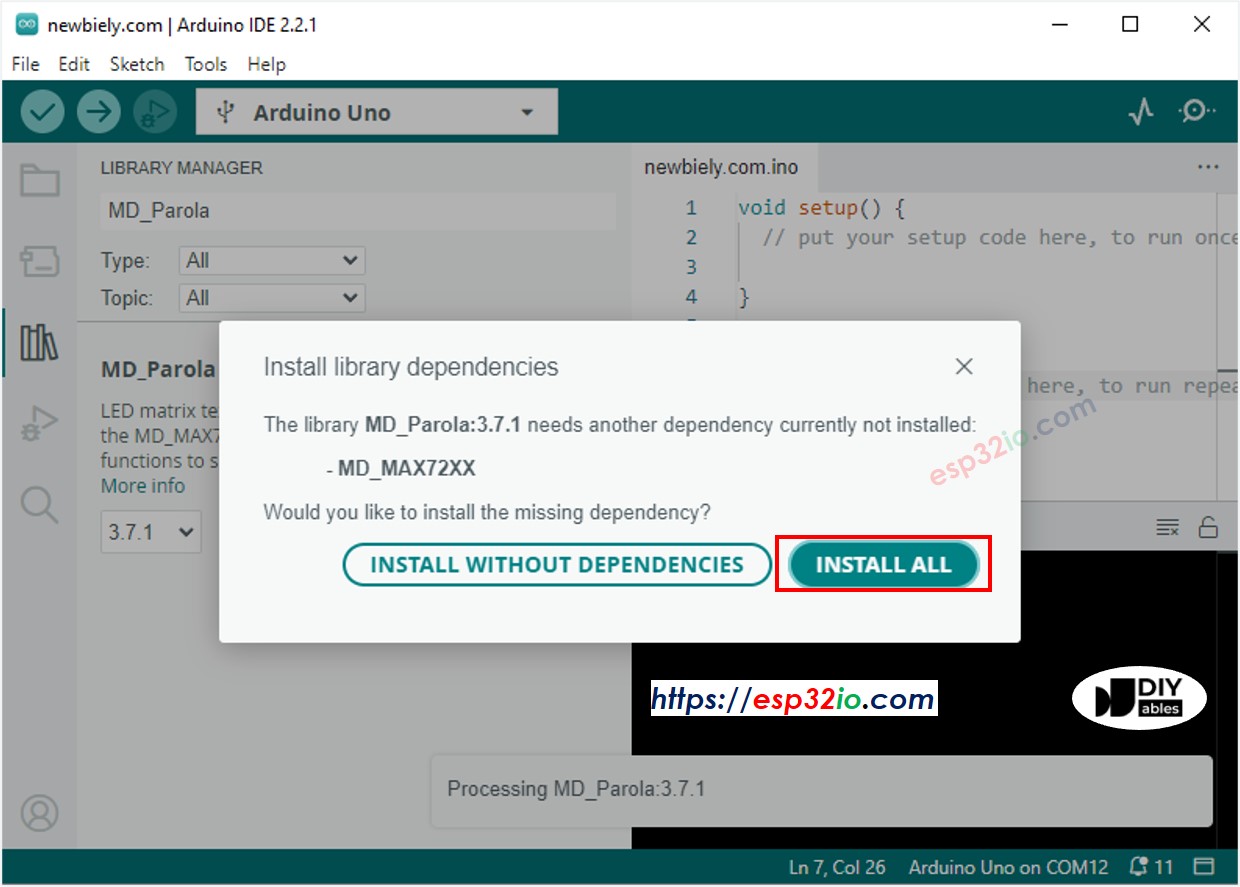
- Copy the above code and open it with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
- See the LED matrix display
ESP32 LED Matrix Code – Scrolling Text
When you want to print a long message that is too long to fit on a LED matrix display, you can use the scroll text effect technique.
The below ESP32 code shows how to scroll a message on the LED matrix display.
For more text effects, please visit MD_Parola Library Reference.
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.