ESP32 - MQTT
In this tutorial, we will explore how to utilize ESP32 for sending and receiving data to and from an MQTT broker using the MQTT protocol. Specifically, we will cover:
- Establishing a connection between ESP32 and an MQTT broker.
- Programming ESP32 to send data to an MQTT broker by publishing data to an MQTT topic.
- Programming ESP32 to receive data by subscribing to an MQTT topic.
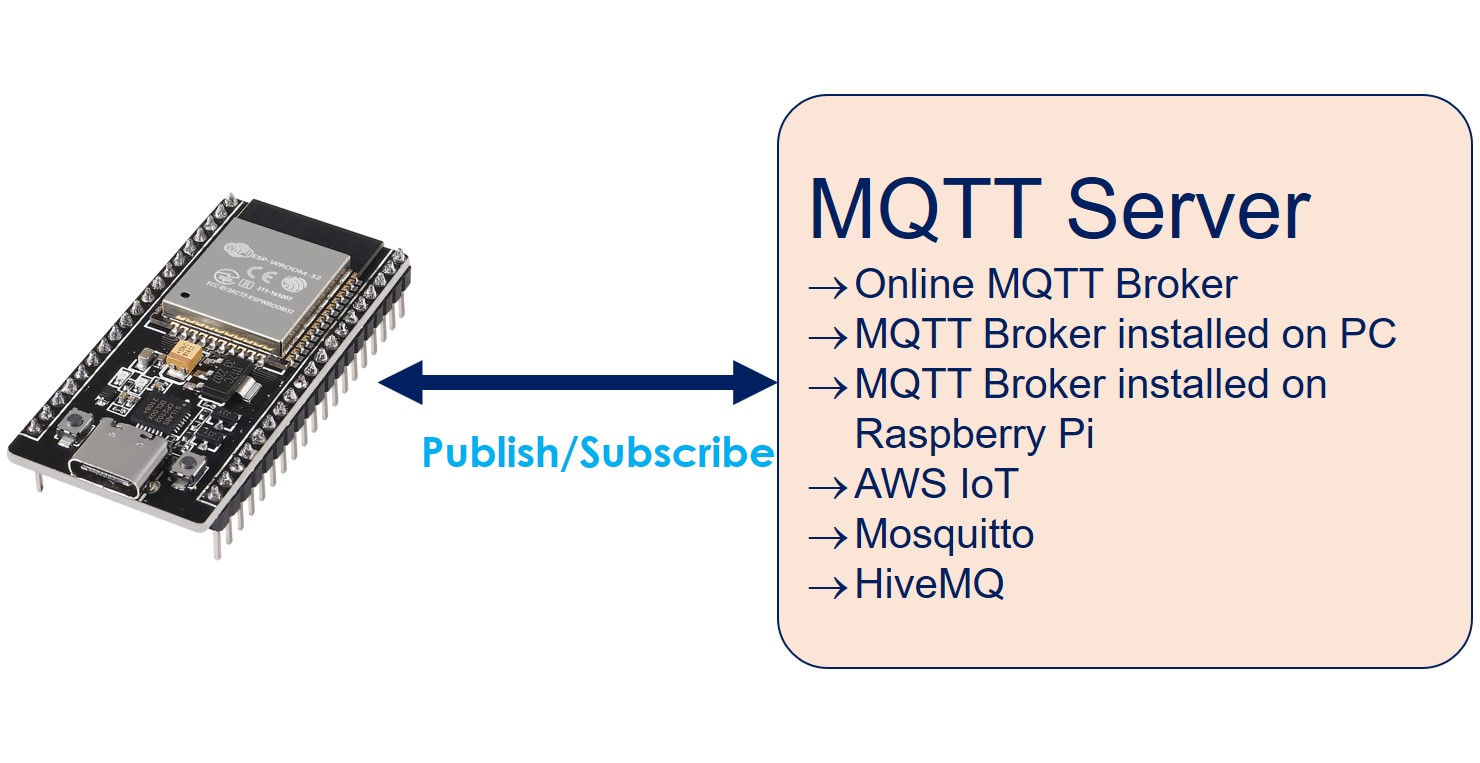
We will delve into two distinct scenarios:
- ESP32 with an online MQTT broker.
- ESP32 with an MQTT broker installed on your PC.
Hardware Used In This Tutorial
1 | × | ESP-WROOM-32 Dev Module | |
1 | × | USB Cable Type-C | |
1 | × | (Recommended) ESP32 Screw Terminal Adapter |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to ESP32 and MQTT
If you're already familiar with the MQTT protocol, great! If not, you can look it up online. This tutorial is all about using ESP32 to send and receive data using MQTT.
Here are some ways ESP32 can work with MQTT:
- Connect ESP32 to an online MQTT broker like Mosquitto or AWS IoT.
- Connect ESP32 to an MQTT broker installed on your computer, such as Mosquitto or HiveMQ.
- Connect ESP32 to an MQTT broker running on your Raspberry Pi, for example, Mosquitto.
- Connect ESP32 to a cloud-based MQTT broker, like Mosquitto or HiveMQ on AWS EC2.
In this tutorial, we'll start by seeing if ESP32 can connect to an online Mosquitto broker. We'll have ESP32 send and receive data through this broker over the internet.
Then, we'll set up the Mosquitto broker on our computer. We'll connect ESP32 to this local broker and continue to send and receive data.
Once you've finished this tutorial, you can explore more by checking out these other tutorials:
These links will provide further guidance on related topics.
Connect ESP32 to an online MQTT broker
In this part, we will learn how to connect ESP32 to test.mosquitto.org, an online MQTT broker created by Mosquitto. Please note that this broker should be used for the testing purpose only.
ESP32 Code
The below ESP32 code does:
- Connect to the MQTT broker
- Subscribe to a topic
- Periodically publish messages to the same topic that it subscribes
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Open Arduino IDE on your PC
- Open the Library Manager by clicking on the Library Manager icon on the left navigation bar of Arduino IDE
- Type MQTT on the search box, then look for the MQTT library by Joel Gaehwiler.
- Click Install button to install MQTT library.
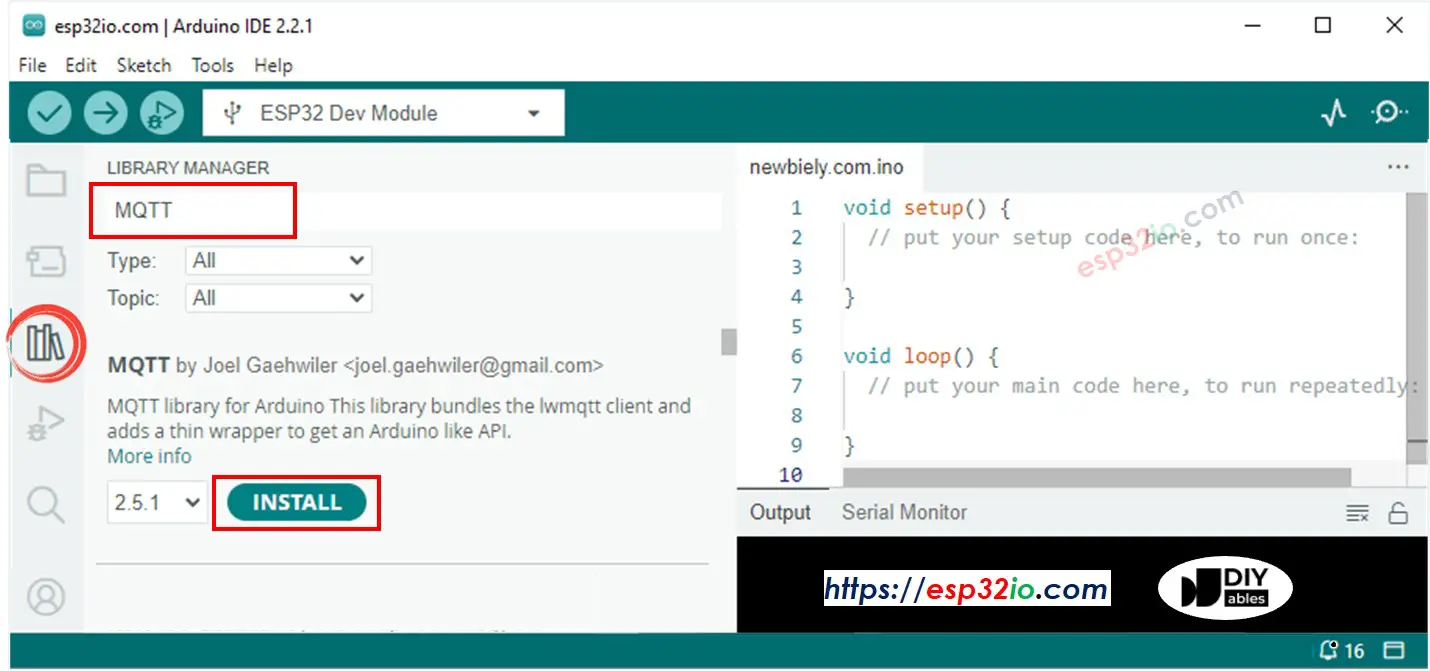
- Type ArduinoJson on the search box, then look for the ArduinoJson library by Benoit Blanchon.
- Click Install button to install ArduinoJson library.
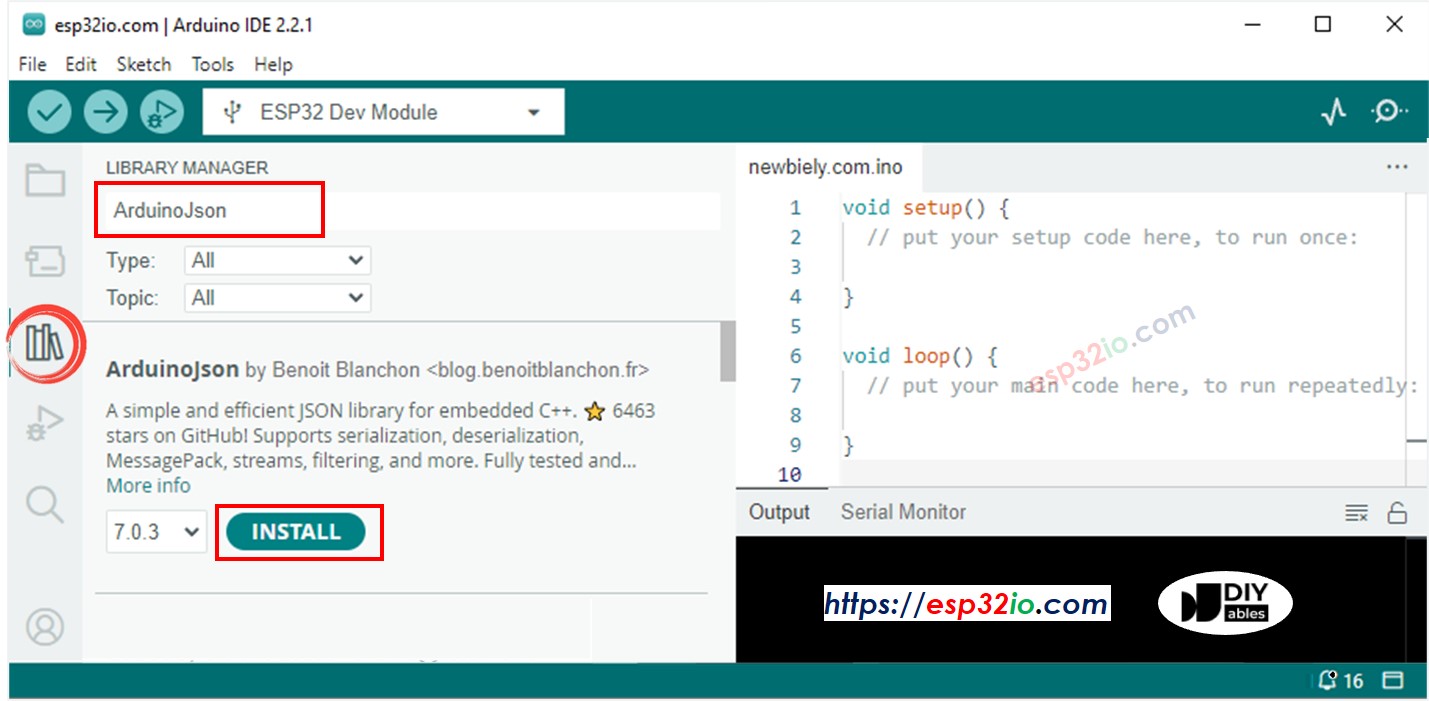
- Copy the above code and open with Arduino IDE
- Replace the WiFi information (SSID and password) in the code with your own.
- In the code, you will see the word 'YOUR-NAME' three times. Replace this word with your name or random characters (alphabet characters only, no spaces). This is necessary because if you do not make the change, there may be multiple people running this code at the same time, which could lead to conflicts because the MQTT client IDs and topics are the same for everyone.
- Click Upload button on Arduino IDE to upload code to ESP32
- Open the Serial Monitor
- See the result on Serial Monitor.
As you can observe, the ESP32 publishes messages to the MQTT broker and then receives the same message back. This occurs because the provided code subscribes to the same topic to which it publishes data. If you prefer the ESP32 not to receive the message it publishes, you can easily achieve this by making the SUBSCRIBE topic different from the PUBLISH topic.
Connect ESP32 to the MQTT broker installed on your PC
Installing Mosquitto MQTT Broker
- Download the Mosquitto MQTT Broker
- Install it on the D: drive instead of the C: drive. Avoid installing the Mosquitto broker on the C: drive to prevent potential issues.
Run Mosquitto MQTT broker
Now, let's check if the MQTT broker is functioning properly by following these steps:
- Go to the directory where Mosquitto was installed. For instance: D:\Draft\mosquitto>
- Make a new file named test.conf, copy the content below, and save it in that directory:
- Run a Command Prompt as Administrator on your PC. Let's call it Broker Window. Do not close it until the end of the tutorial.
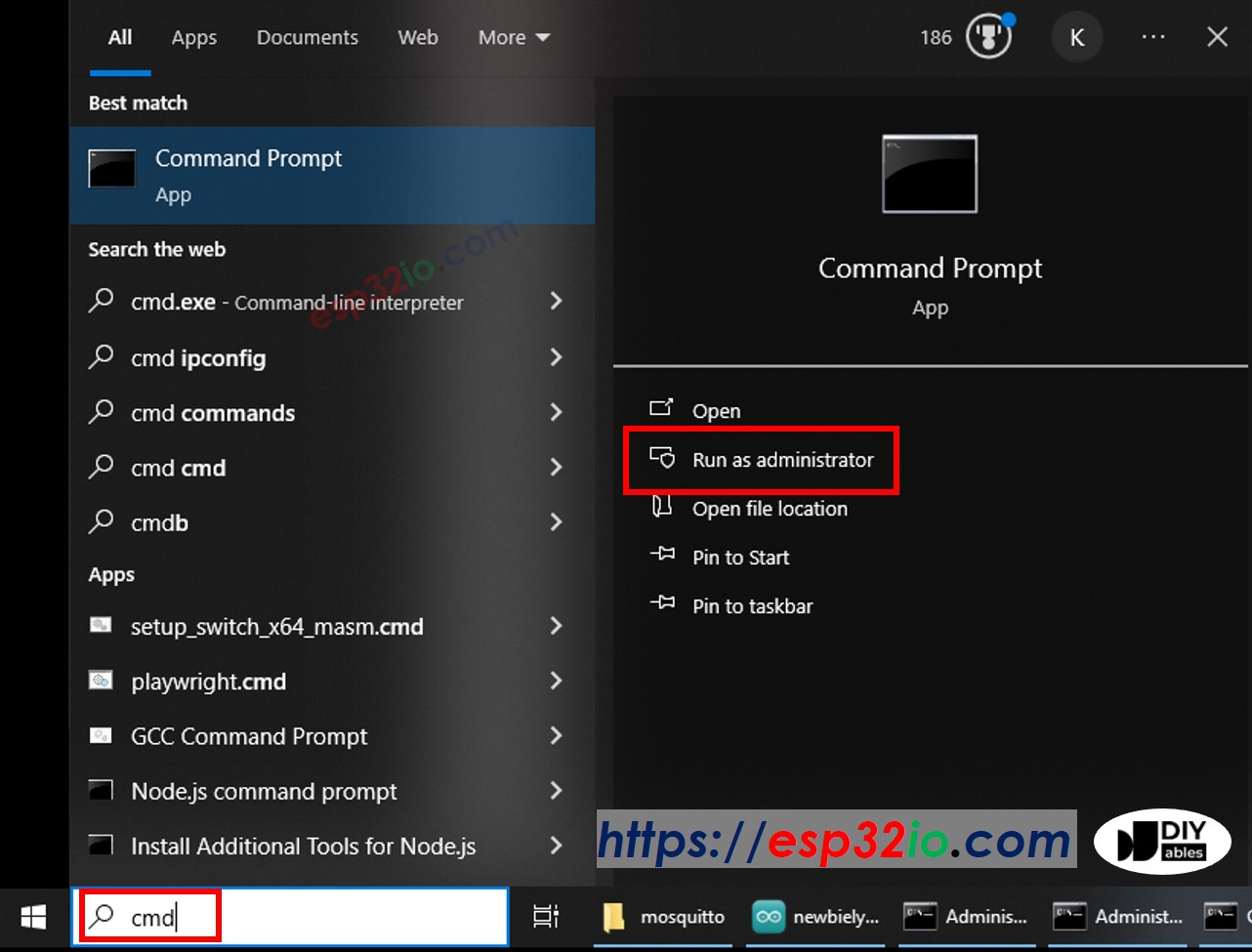
- Run the below commands one by one:
- You will see:
- Open another Command Prompt as Administrator on your PC.
- Find the IP address of your PC by running the below command:
- Write down the IP address for later use. In the above example: 192.168.0.26
Test if the Mosquitto Broker works
- Open another Command Prompt as Administrator on your PC. Let's call it Subscriber Window
- Subscribe to a topic by running the below commands one by one (replace by your IP address):
- Open another Command Prompt as Administrator on your PC. Let's call it Publisher Window
- Publish a message to the same topic by running the below commands one by one (replace by your IP address):
- You will see:
You will see that message is forwarded to the Subscriber Window as follows:
Now, you installed successfully the Mosquitto MQTT broker on your PC. Please do NOT close three windows: Broker Window, Subscriber Window, and Publisher Window. We will use them next.
ESP32 Code
The below ESP32 code does:
- Connect to the MQTT broker
- Subscribe to a topic
- Periodically publish messages to another topic
Quick Instructions
- Copy the above code and open with Arduino IDE
- Replace the WiFi information (SSID and password) in the code with your own.
- Replace the MQTT broker address in the code (domain name or IP address).
- Click Upload button on Arduino IDE to upload code to ESP32
Send message from ESP32 to PC via MQTT
ESP32 codes publishes data to the MQTT topic esp32-001/send, Subscriber Window on PC subscribe that topic to receive the data.
- Open the Serial Monitor, you will see ESP32 periodically publish a message to a topic.
- Check the Subscriber Window, you will see that it receives the message published by ESP32 as below:
Send message from PC to ESP32 via MQTT
ESP32 subscribes to the topic esp32-001/receive, Publisher Window on PC publish a message to that topic to send it to the ESP32.
- Publish a message to the topic that ESP32 subscribed by running the following command on Publisher Window:
- You will see this message is received by ESP32 on Serial Monitor as below:
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.