ESP32 - Temperature via Web
In this guide, we'll explore the process of programming the ESP32 to function as a web server, allowing you to access temperature data via a web interface. Using an attached DS18B20 temperature sensor, you can easily check the current temperature by using your smartphone or PC to visit the web page served by the ESP32. Here's a brief overview of how it works:
- ESP32 is programmed as a web server.
- You type the IP address of ESP32 in a web browser on your smartphone or PC.
- ESP32 responds to the request from the web browser with a web page that contains the temperature read from the DS18B20 sensor.
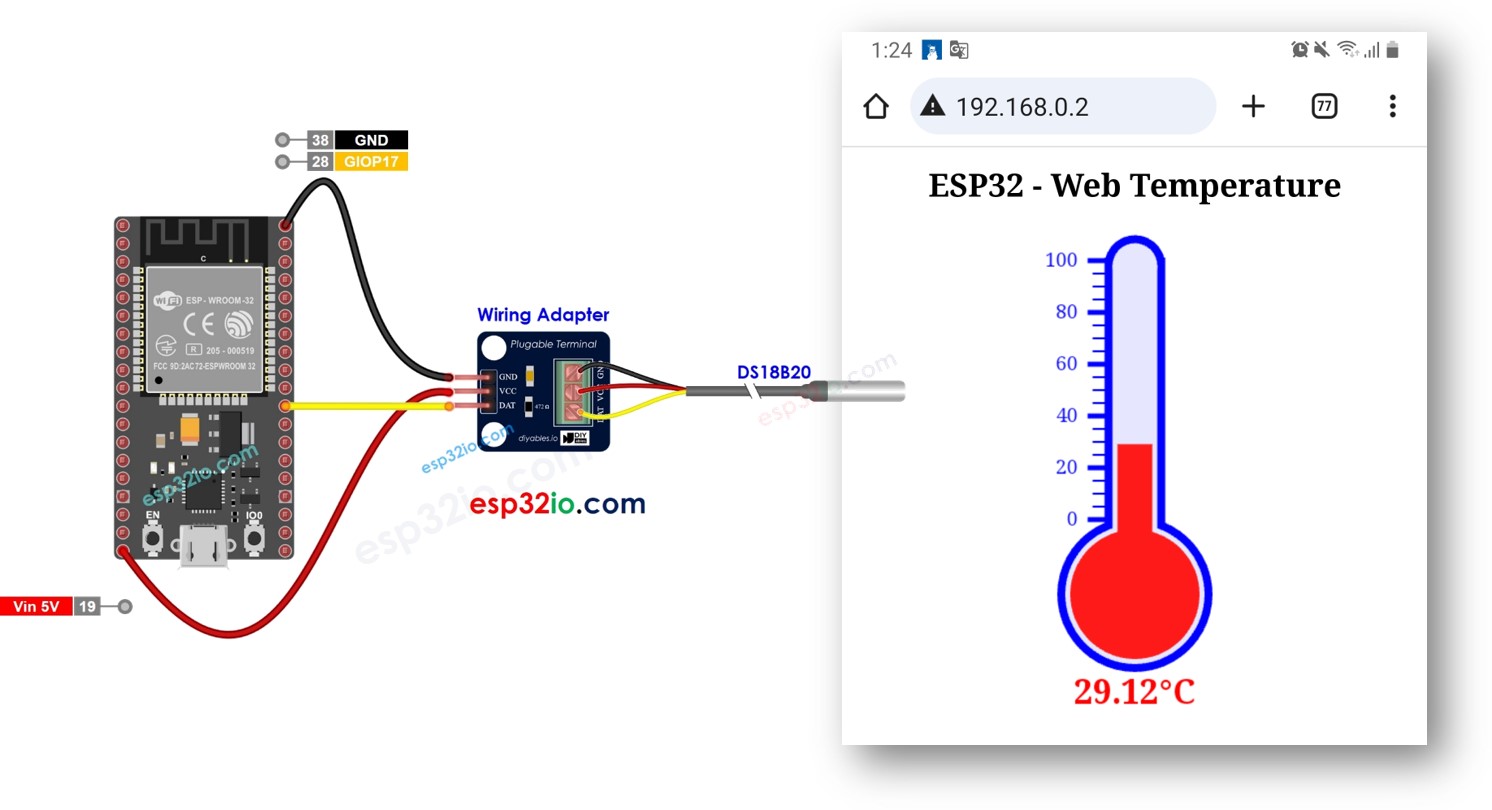
We will go through two example codes:
- ESP32 code that provides a very simple web page that shows the temperature from the DS18B20 sensor. This makes it easy for you to understand how it works. HTML content is embedded in ESP32 code
- ESP32 code that provides a graphic web page that shows the temperature from the DS18B20 sensor, HTML content is separated from ESP32 code.
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Buy Note: Numerous DS18B20 sensors available in the market are of poor quality. We strongly advise purchasing the sensor from the DIYables brand via the link above; we conducted tests, and it performed reliably.
Introduction to ESP32 Web Server and DS18B20 Temperature Sensor
If you do not know about ESP32 Web Server and DS18B20 temperature sensor (pinout, how it works, how to program ...), learn about them in the following tutorials:
Wiring Diagram
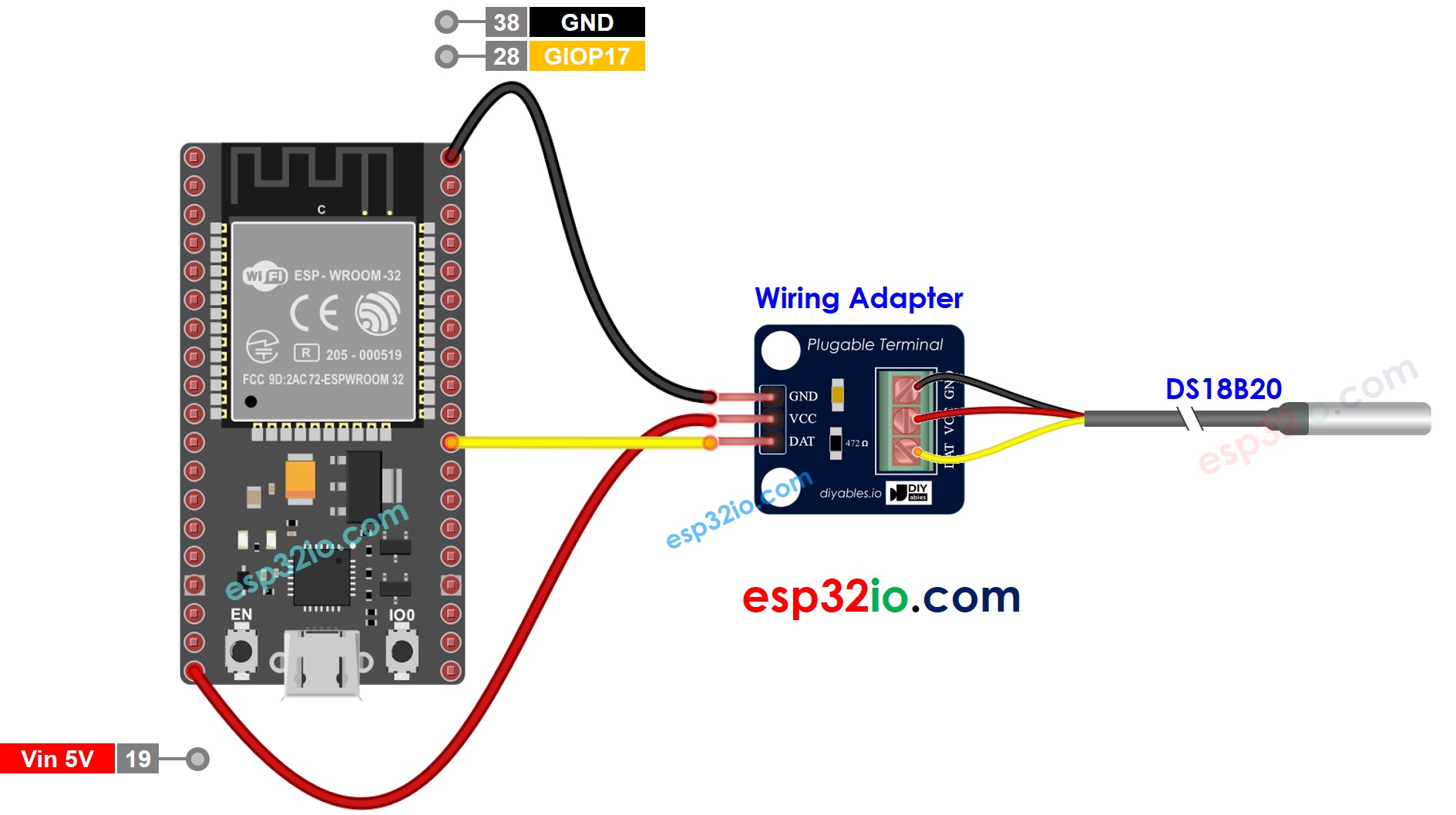
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
ESP32 Code - Simple Web Page
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Open the Library Manager by clicking on the Library Manager icon on the left navigation bar of Arduino IDE.
- Search “ESPAsyncWebServer”, then find the ESPAsyncWebServer created by lacamera.
- Click Install button to install ESPAsyncWebServer library.
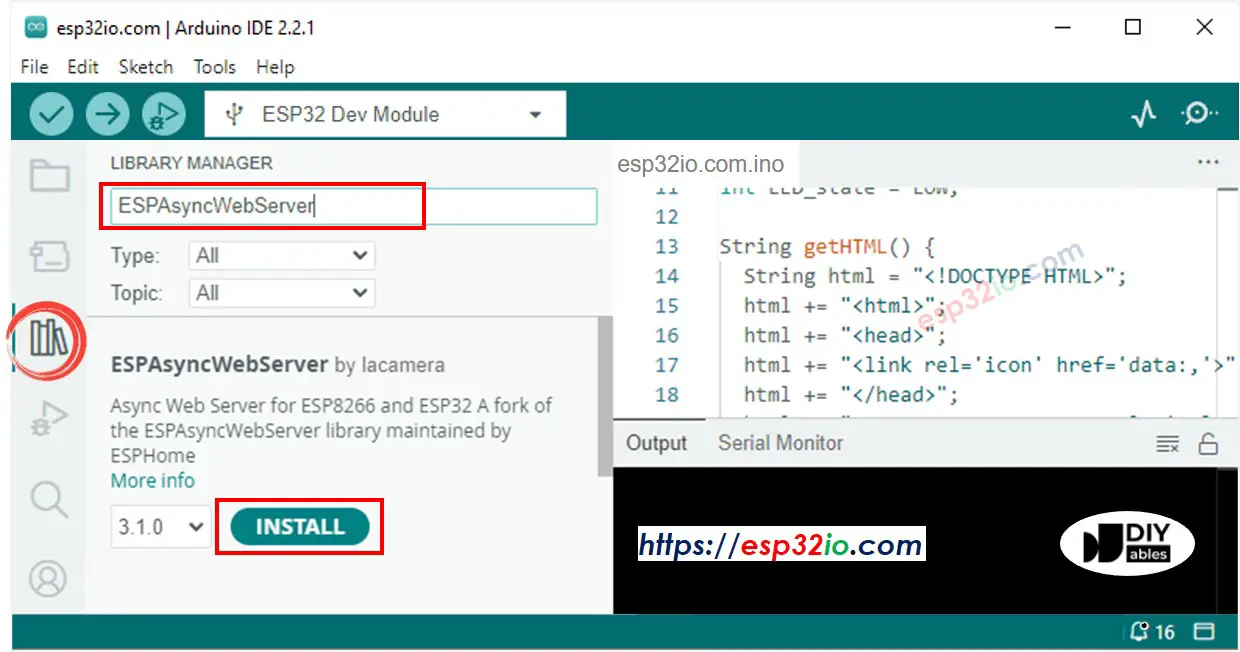
- You will be asked to install the dependency. Click Install All button.
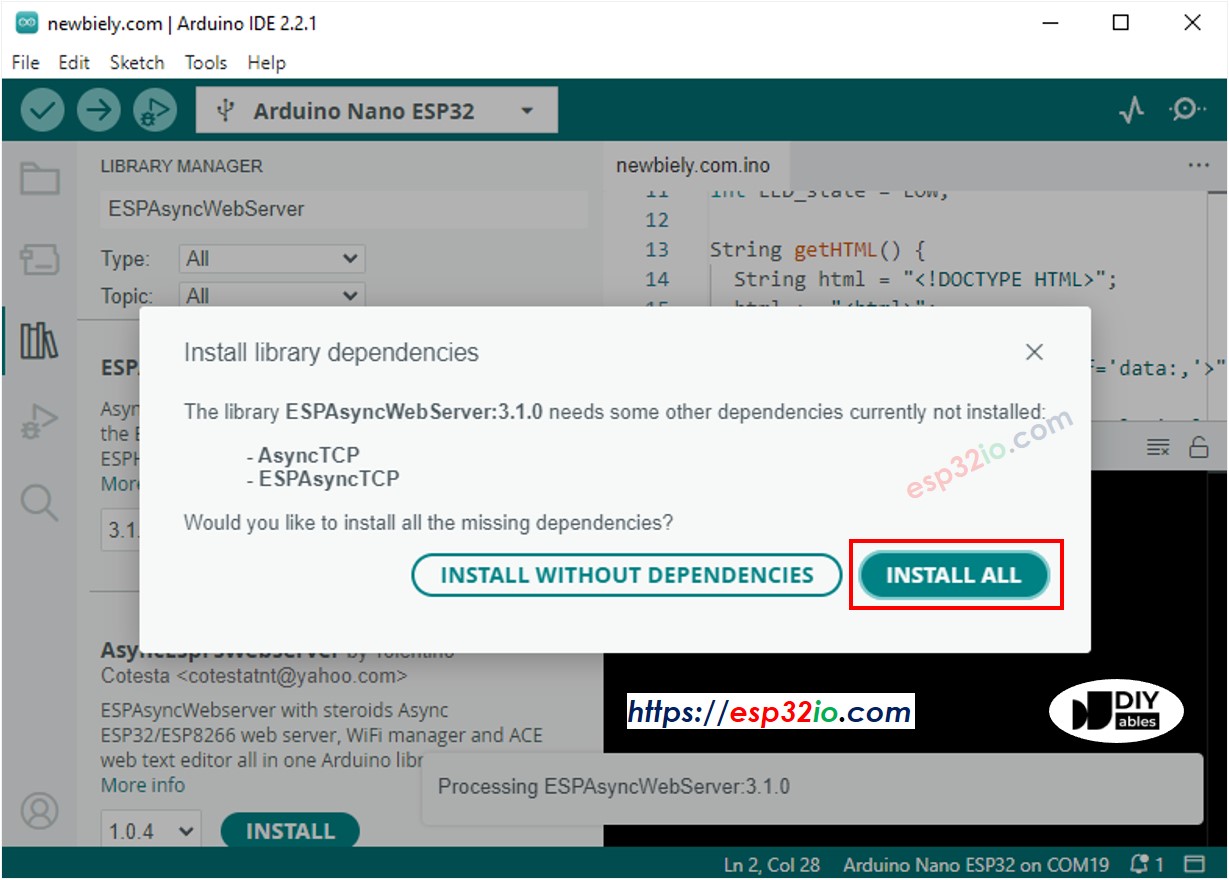
- Search “DallasTemperature” on the search box, then look for the DallasTemperature library by Miles Burton.
- Click Install button to install DallasTemperature library.
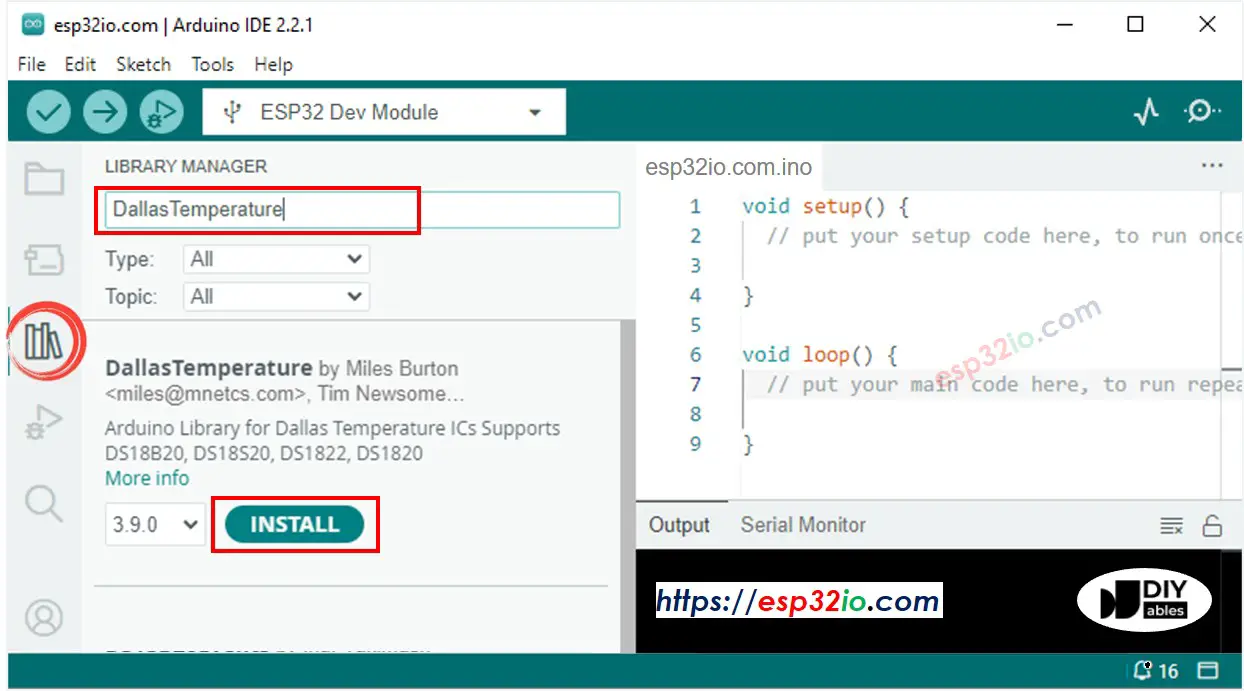
- You will be asked to install the dependency. Click Install All button to install OneWire library.
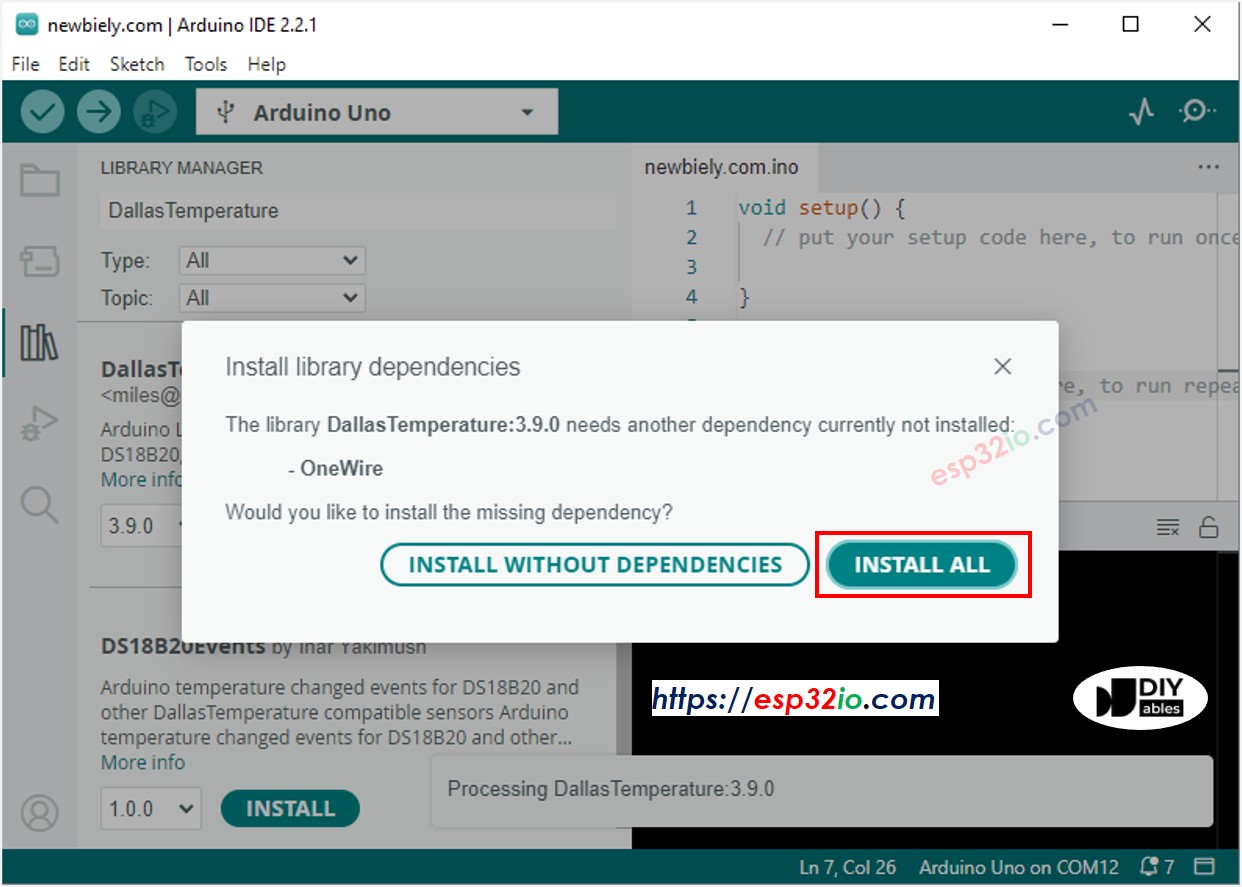
- Copy the above code and open with Arduino IDE
- Change the wifi information (SSID and password) in the code to yours
- Click Upload button on Arduino IDE to upload code to ESP32
- Open the Serial Monitor
- Check out the result on Serial Monitor.
- You will find an IP address. Type this IP address into the address bar of a web browser on your smartphone or PC.
- You will see the following output on the Serial Monitor.
- You will see a very simple web page of ESP32 board on the web browser as below:
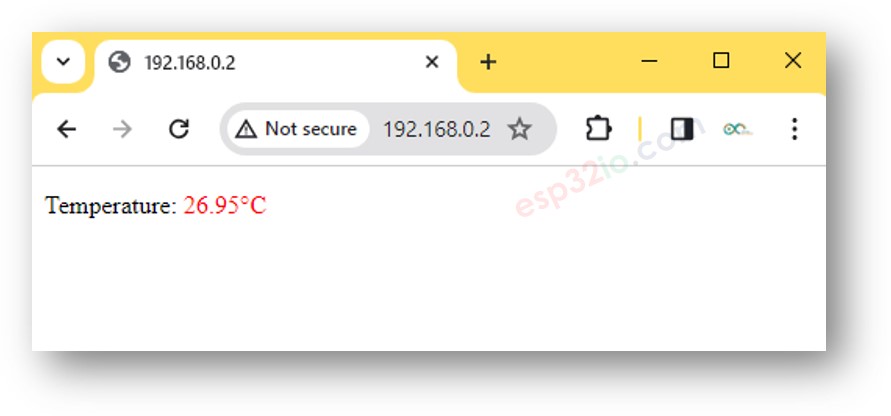
※ NOTE THAT:
With the code provided above, to get the termperature update, you have to reload the page on the web browser. In a next part, we will learn how to make web page update the temperature value on backround without reloading the webpage.
ESP32 Code - Graphic Web Page
As a graphic web page contains a large amount of HTML content, embedding it into the ESP32 code as before becomes inconvenient. To address this, we need to separate the ESP32 code and the HTML code into different files:
- The ESP32 code will be placed in a .ino file.
- The HTML code (including HTML, CSS, and Javascript) will be placed in a .h file.
For detail of how to separate the HTML code from ESP32 code, please refer to ESP32 - Web Server tutorial.
Quick Instructions
- Open Arduino IDE and create new sketch, Give it a name, for example, esp32io.com.ino
- Copy the below code and open with Arduino IDE
- Change the WiFi information (SSID and password) in the code to yours
- Create the index.h file On Arduino IDE by:
- Either click on the button just below the serial monitor icon and choose New Tab, or use Ctrl+Shift+N keys.
- Give the file's name index.h and click OK button
- Copy the below code and paste it to the index.h.
- Now you have the code in two files: esp32io.com.ino and index.h
- Click Upload button on Arduino IDE to upload code to ESP32
- Access the web page of ESP32 board via web browser on your PC or smartphone as before. You will see it as below:
- If you modify the HTML content in the index.h and does not touch anything in esp32io.com.ino file, when you compile and upload code to ESP32, Arduino IDE will not update the HTML content.
- To make Arduino IDE update the HTML content in this case, make a change in the esp32io.com.ino file (e.g. adding empty line, add a comment....)
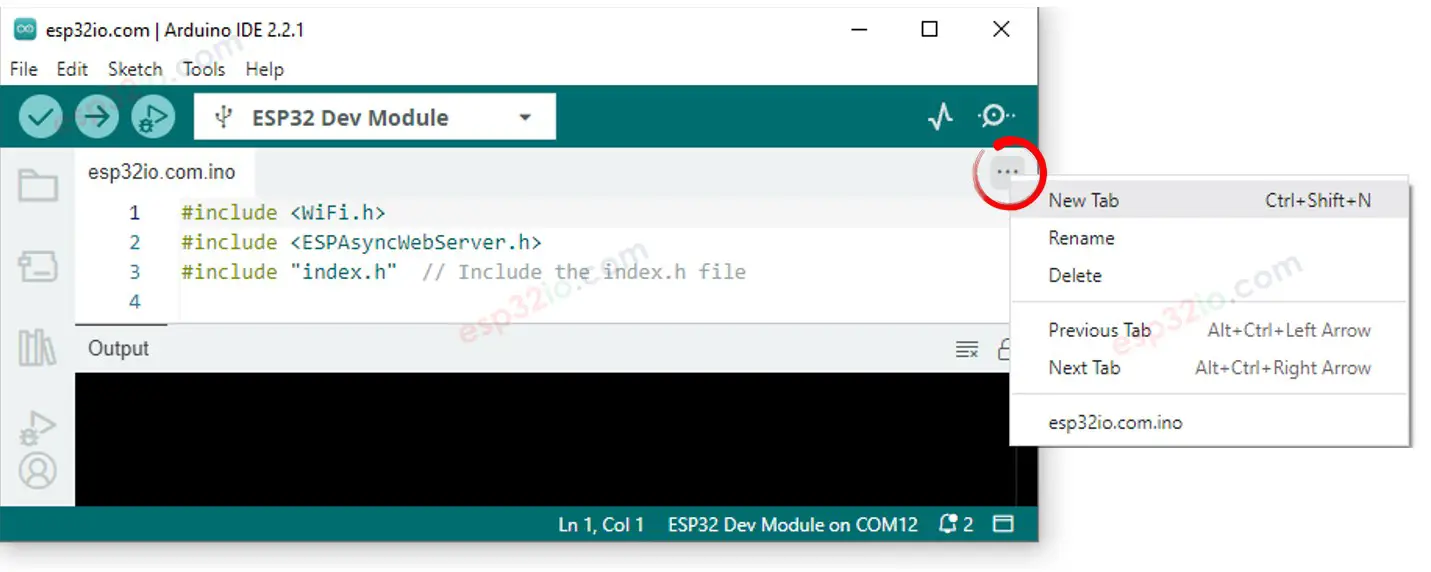
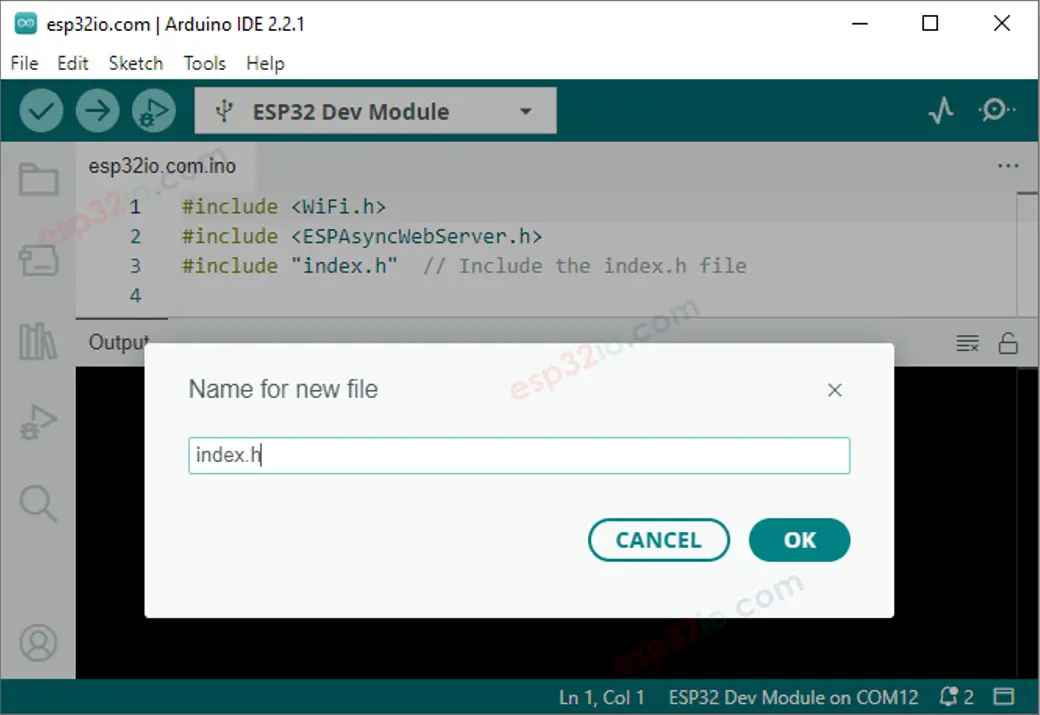
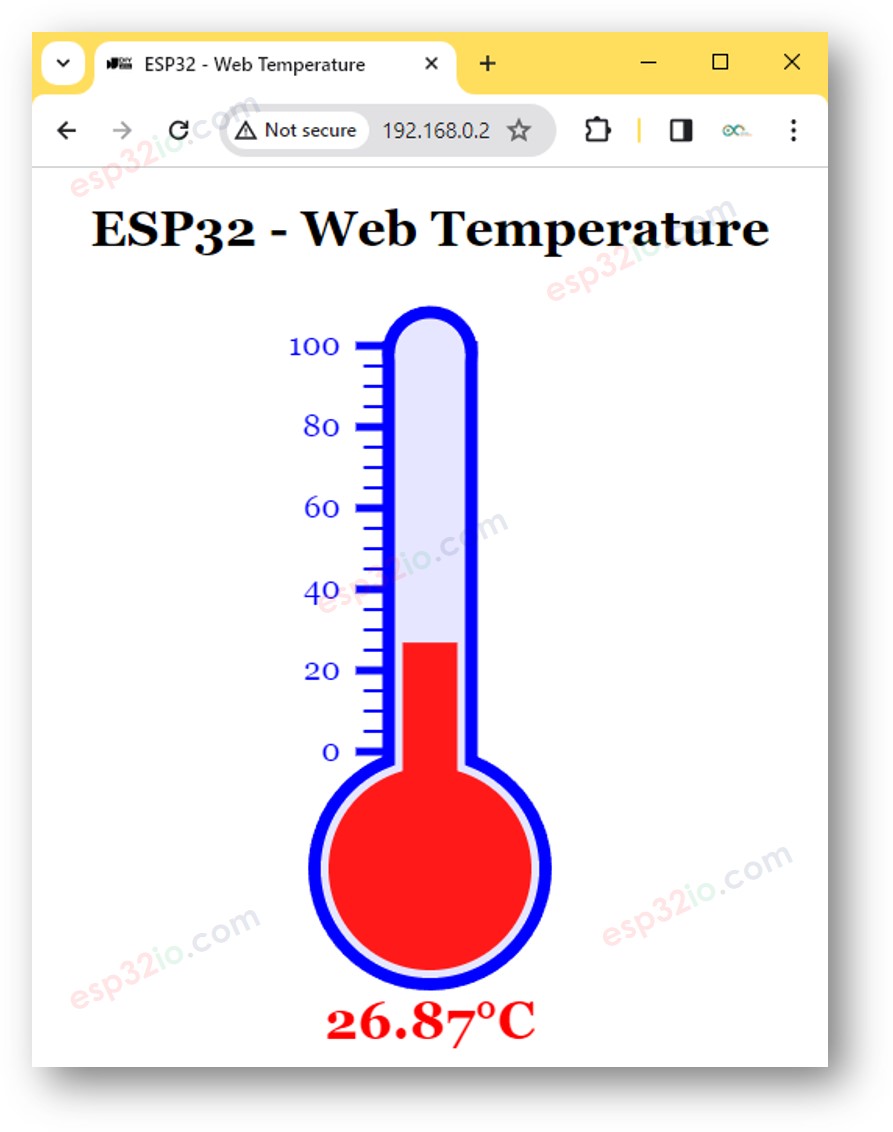
※ NOTE THAT: