ESP32 - Temperature Humidity Sensor
This tutorial instructs you how to use ESP32 to read temperature and humidity value from DHT11 or DHT22 sensors, and print it to Serial Monitor.
Hardware Used In This Tutorial
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to DHT11 and DHT22 Temperature and Humidity Sensor
In short, the DHT22 sensor is a more accurate, larger range but more expensive than DHT11. Let's see the common and differences between them
The commons
- The same pinout
- The same wiring to ESP32
- The similar ESP32 code
The differences
The below table show the detaied differences between DHT11 and DHT22:
DHT22 | DHT11 | |
---|---|---|
The price | low cost | ultra low cost |
The humidity range | 0% to 100% | 20% to 80% |
The humidity accuracy | ± 2% to 5% | 5% |
The temperature range | -40°C to 80°C | 0°C to 50°C |
The temperature accuracy | ± 0.5°C | ± 2°C |
The reading rate | 0.5Hz (one time per 2 seconds) | 1Hz (one time per second) |
Dimension | 15.1mm x 25mm x 7.7mm | 15.5mm x 12mm x 5.5mm |
Operating Voltage | 3 to 5V | 3 to 5V |
DHT11 and DHT22 Pinout
DHT11 and DHT22 sensor includes 4 pins:
- GND pin: connect this pin to GND (0V)
- VCC pin: connect this pin to VCC (3.3V or 5V)
- DATA pin: the pin is used to communicate between the sensor and ESP32
- NC pin: Not connected
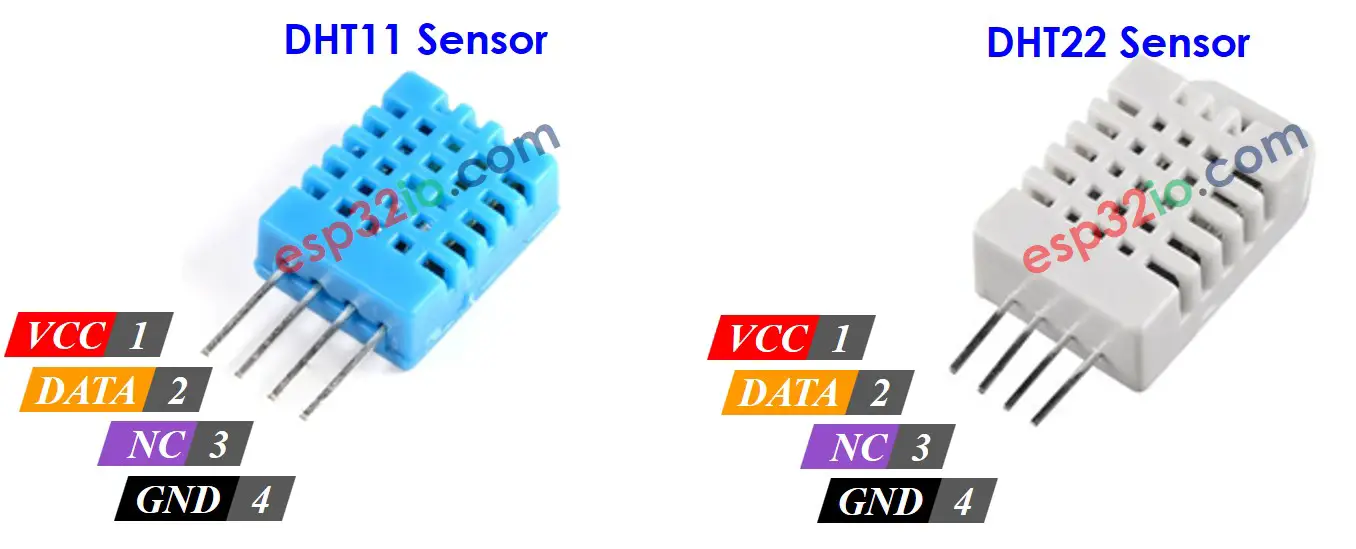
We highily recommend you using DHT11 and DHT22 sensor modules. The modules have a built-in resistor and have only three pin: VCC, GND, and DATA pins (or alternatively: +, -, and OUT pins).
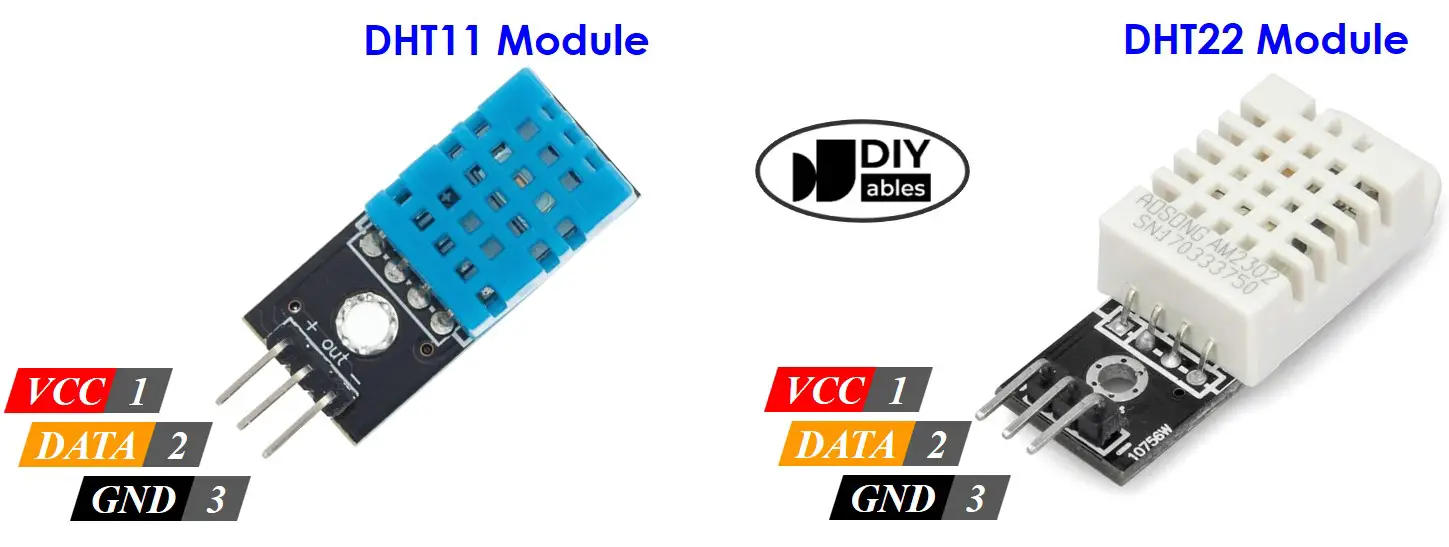
The pins order on the module's can be different between manufacturers. Please check the pin labels printed on the module carefully.
Wiring Diagram between DHT11/DHT22 and ESP32
Wiring to ESP32 is the same for both sensors. In orginal form, A resistor from 5K to 10K Ohms is required to keep the data line high and in order to enable the communication between the sensor and the ESP32
ESP32 - DHT11 Sensor Wiring
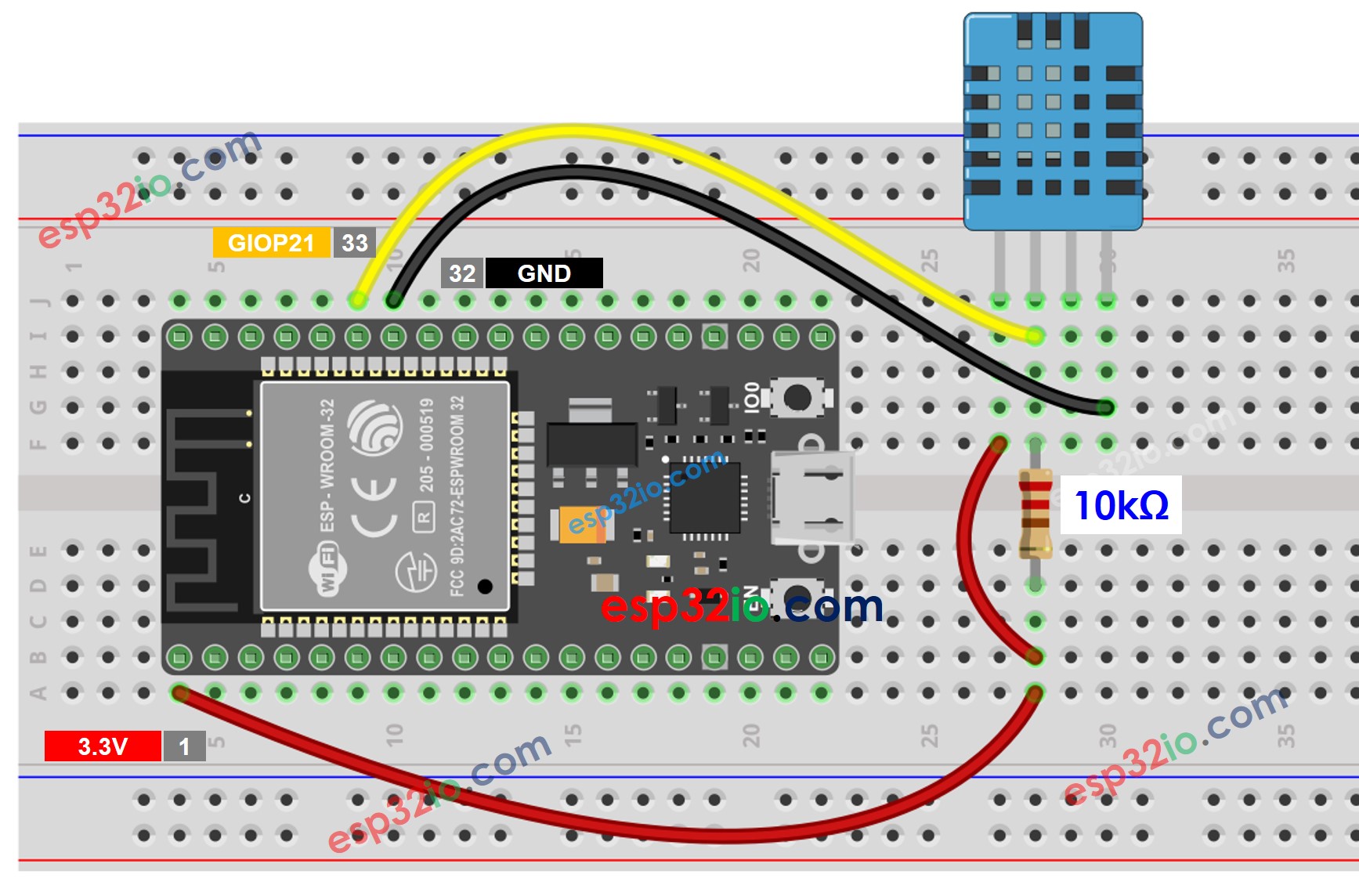
This image is created using Fritzing. Click to enlarge image
ESP32 - DHT22 Sensor Wiring
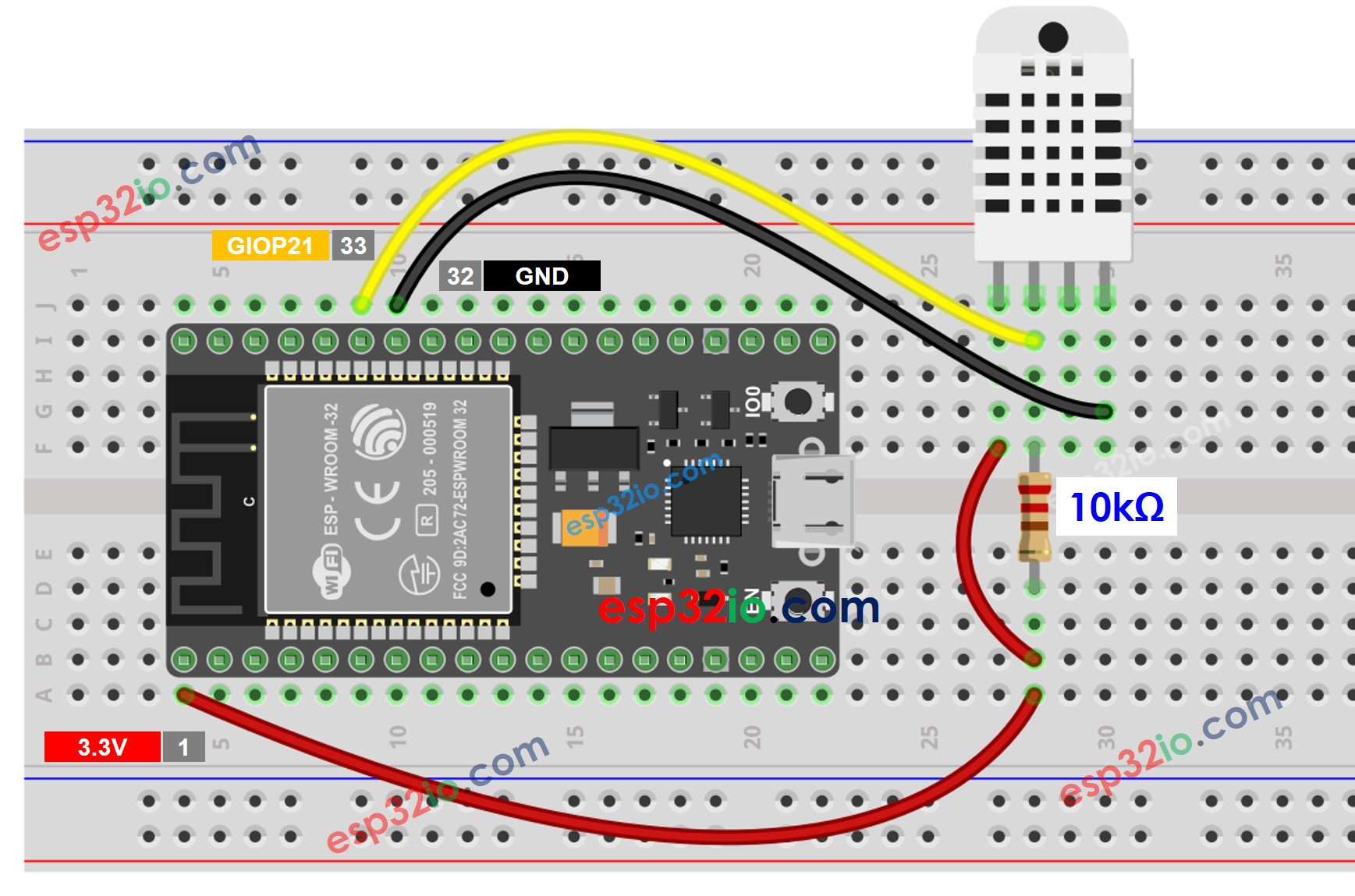
This image is created using Fritzing. Click to enlarge image
ESP32 - DHT11 Module Wiring
Most of DHT22 sensor modules have a built-in resistor, so you don't need to add it. it saves us some wiring or soldering works.
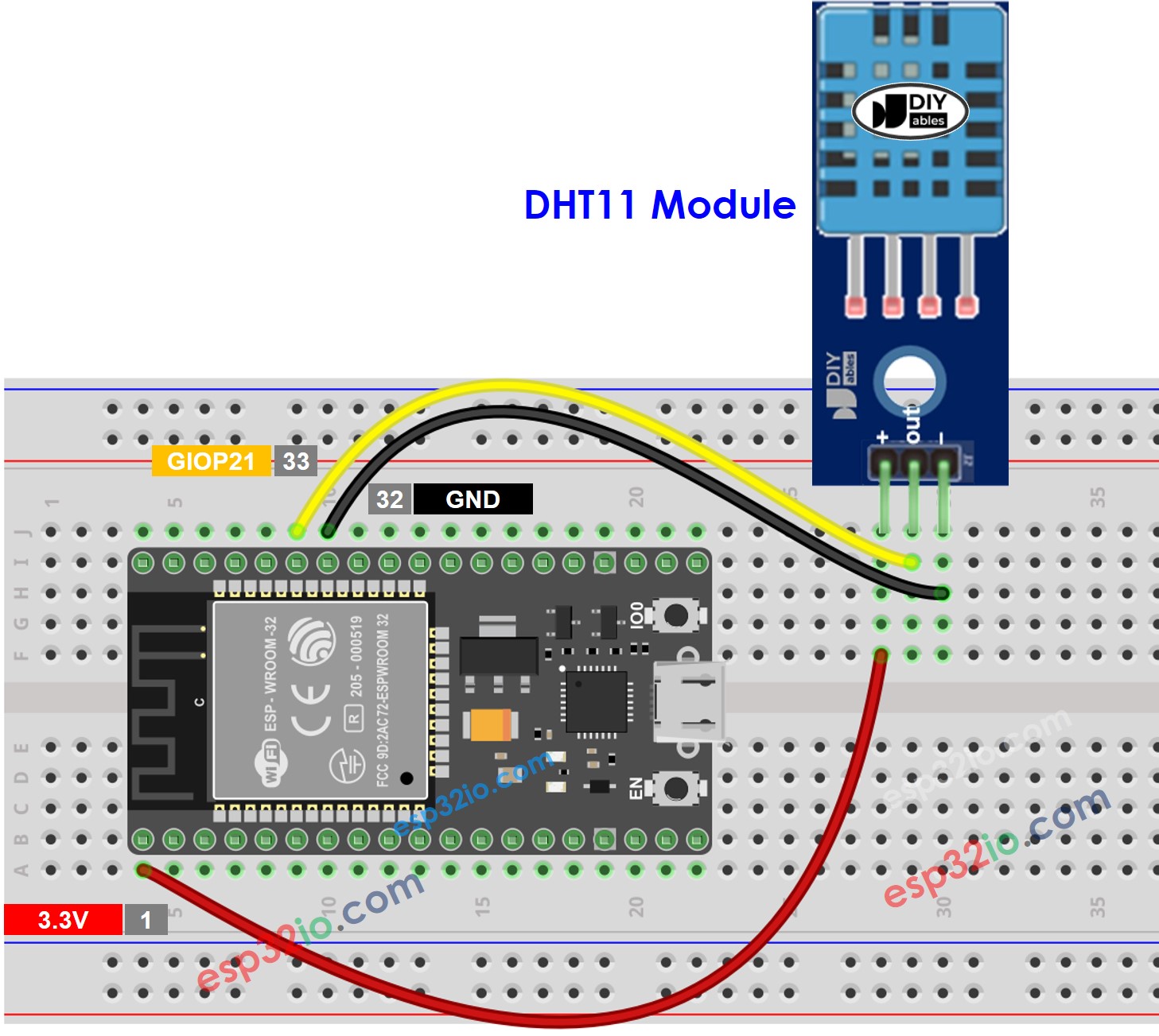
This image is created using Fritzing. Click to enlarge image
ESP32 - DHT22 Module Wiring
Most of DHT22 sensor modules have a built-in resistor, so you don't need to add it. it saves us some wiring or soldering works.
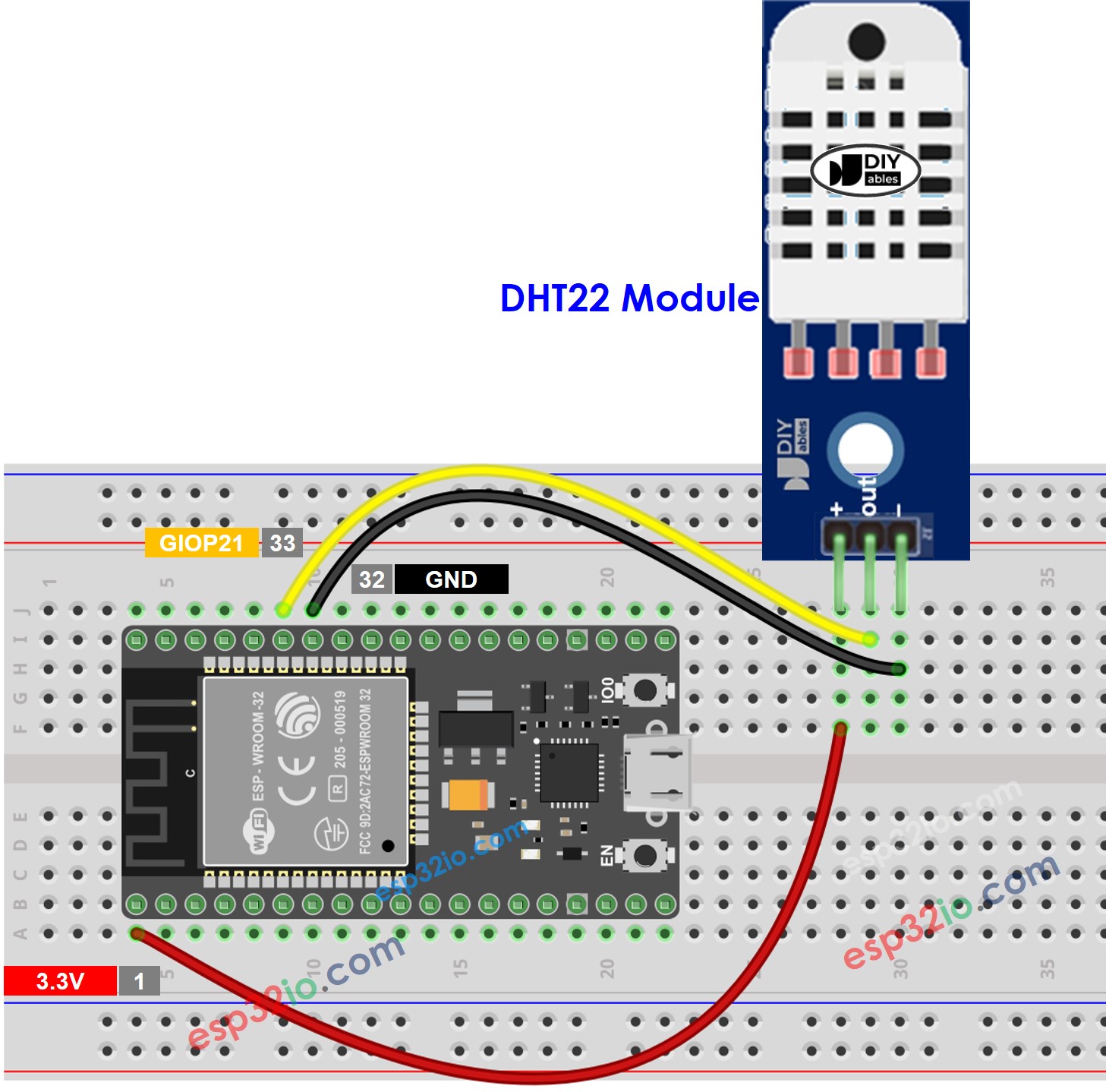
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
ESP32 Code - DHT11
ESP32 Code - DHT22
Two above codes have only one line different.
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Type “DHT” on the search box, then look for the DHT sensor library by Adafruit
- Install the library by clicking on Install button.
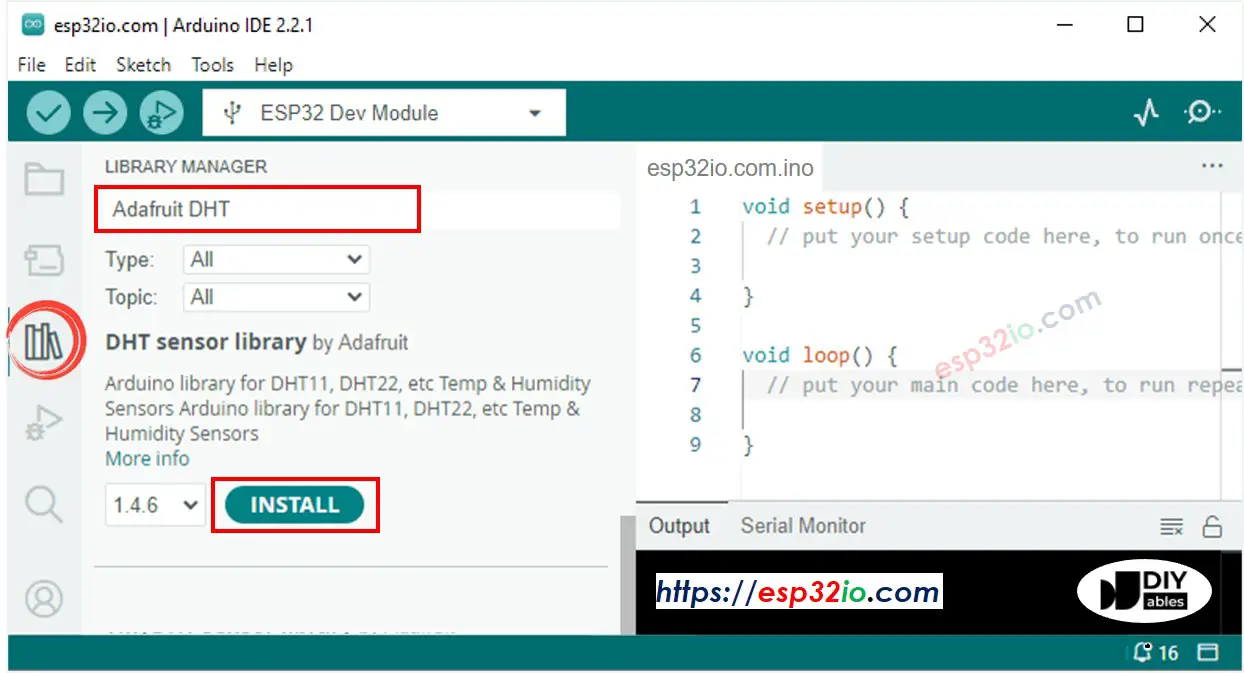
- A windows appears to ask you to install dependencies for the library
- Install all dependencies for the library by clicking on Install All button.
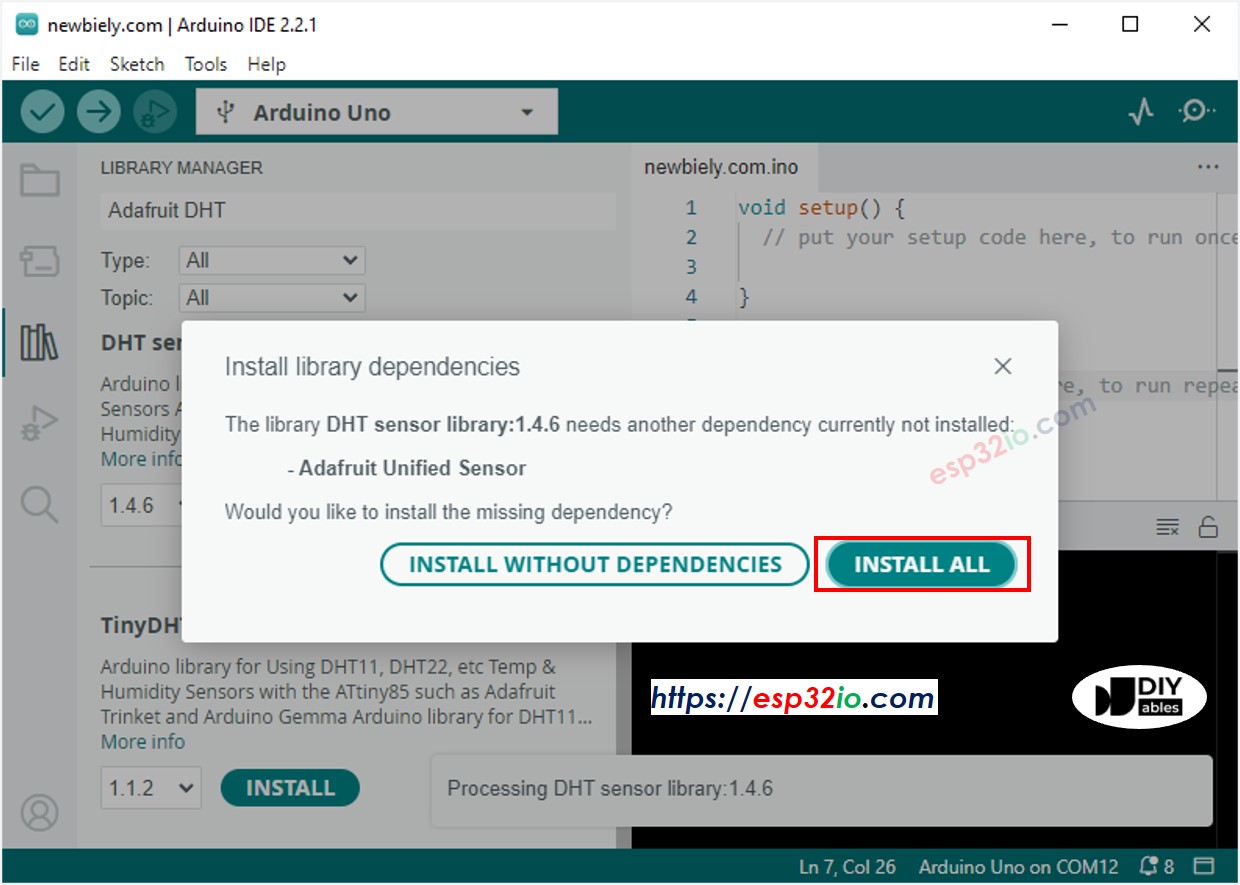
- Copy one of the above code and open with Arduino IDE
- Compile and upload code to ESP32 board by clicking Upload button on Arduino IDE
- Make the sensor colder or hotter. For example, putting the sensor near a hot cup of coffee
- See the result on Serial Monitor. It looks like the below:.
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.