ESP32 - RFID MP3 Player
In this tutorial, we will explore the process of creating an RFID-based MP3 player using ESP32, an RC522 RFID reader, and an MP3 player module. The MP3 player module is equipped with a micro SD card where multiple songs are stored. Each RFID card represents for a song, and the number of RFID cards matches the number of songs.
By swiping an RFID card in front of the RFID reader, the ESP32 plays the corresponding song associated with that specific RFID card.
Hardware Used In This Tutorial
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to RFID/NFC RC522 Module and MP3 Player
Unfamiliar with RFID/NFC RC522 Module and MP3 player, including their pinouts, functionality, and programming? Explore comprehensive tutorials on these topics below:
Wiring Diagram
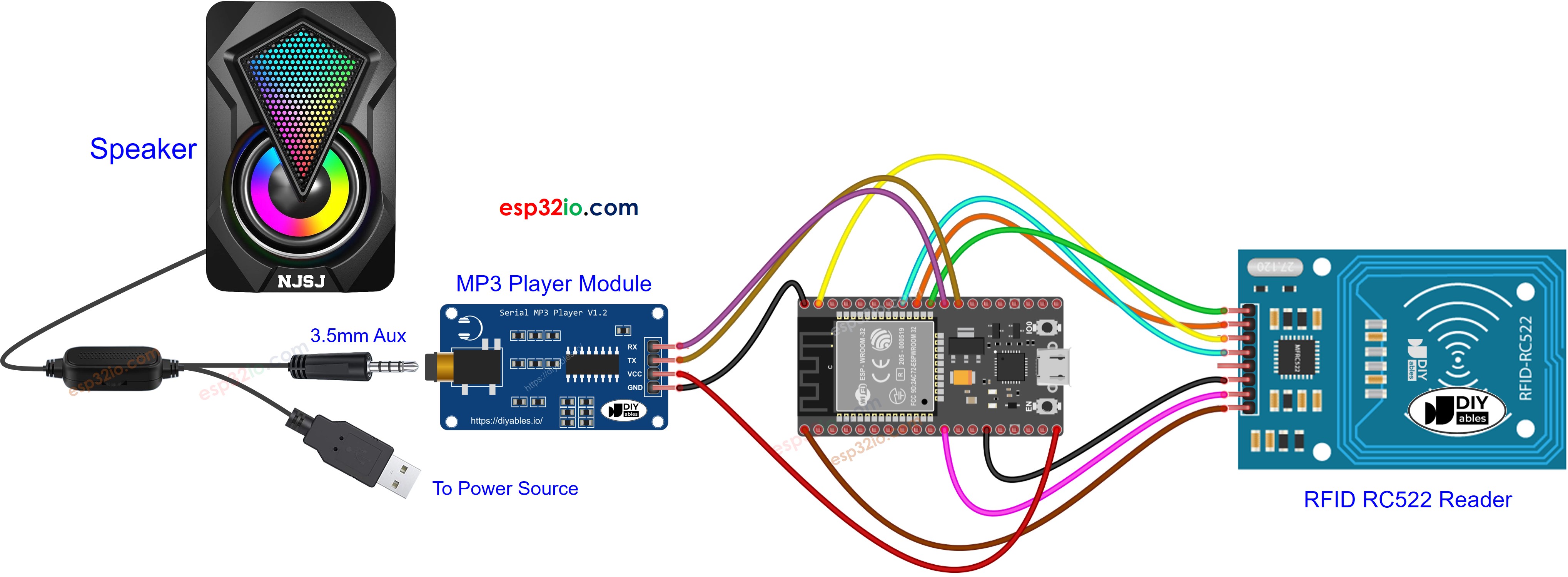
This image is created using Fritzing. Click to enlarge image
※ NOTE THAT:
The order of pins can vary according to manufacturers. ALWAYS use the labels printed on the module. The above image shows the pinout of the modules from DIYables brand.
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
Preparation
- Pre-store a list of songs that you want to play to micro SD Card.
- Insert the micro SD Card to the MP3 player module
- Connect the MP3 player module to ESP32
- Connect the speaker to the MP3 player module to a
- Connect the speaker to a power source.
- Connect the RFID reader to the ESP32.
Because UID is usually not printed on RFID Tag, The first step we need to do is to find out the tags' UID. This can be done by:
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Copy the below code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
- Open Serial Monitor
- Tap RFID card/keyfob one by one on RFID-RC522 module
- Take note of UIDs on Serial Monitor, it looks like below:
We will use these RFID UIDs to update the ESP32 code below
ESP32 Code - RFID Mp3 Player
Quick Instructions
- Copy the above code and open with Arduino IDE
- Update UIDs you obtained in the preperation step to the above code.
- Upload the code to ESP32
- Tap an RFID Tag on RFID-RC522 module one by one
- Check out the sound from MP3 Player
- If the everything run smoothly, each RFID card will be associlated with a song.
- You can mark the name of the song on each RFID card.
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.