ESP32 - Ultrasonic Sensor - OLED
This tutorial instructs you how to use ESP32 to read the distance from ultrasonic sensor and display it on an OLED.
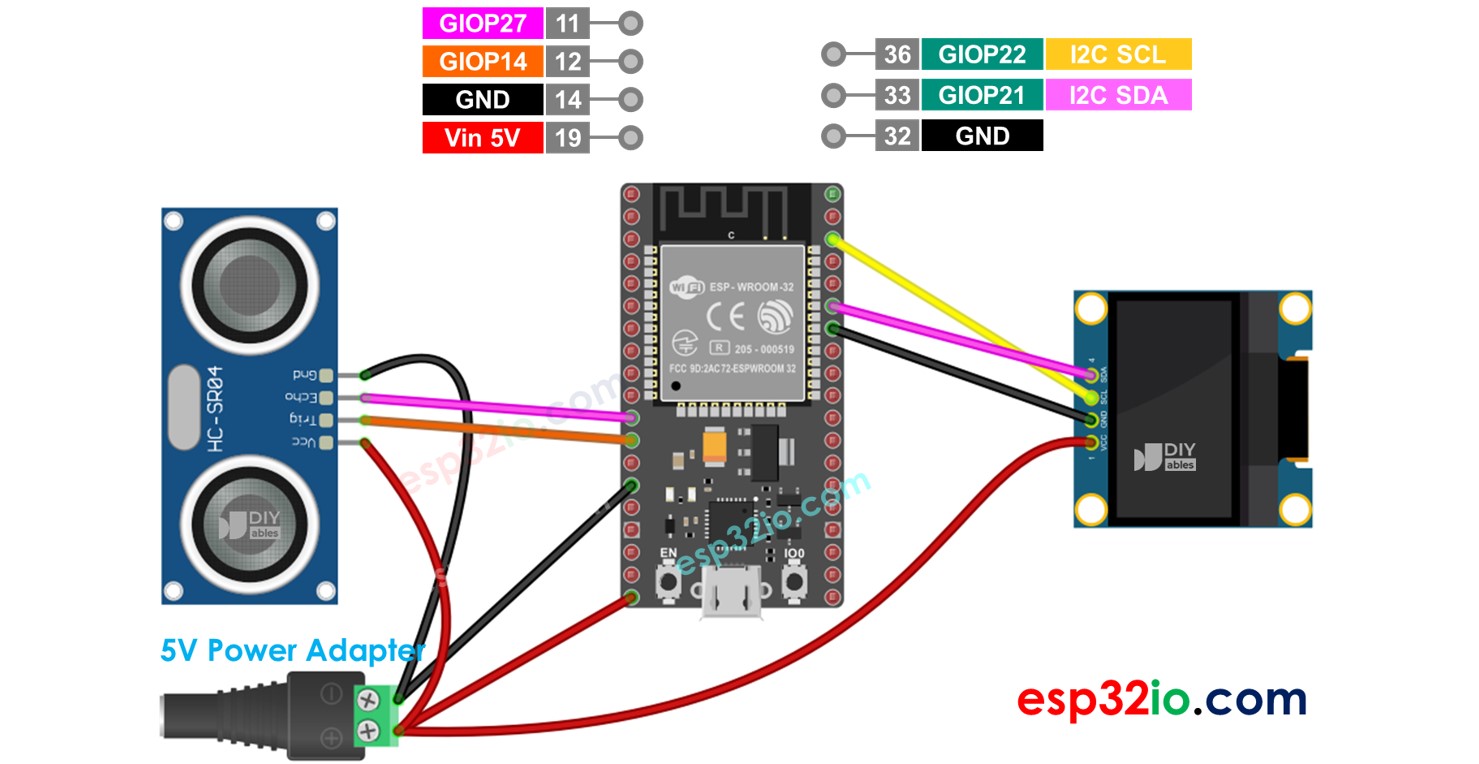
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to OLED and Ultrasonic Sensor
We have specific tutorials about OLED and Ultrasonic Sensor. Each tutorial contains detailed information and step-by-step instructions about hardware pinout, working principle, wiring connection to ESP32, ESP32 code... Learn more about them at the following links:
- ESP32 - OLED tutorial
- ESP32 - Ultrasonic Sensor tutorial
Wiring Diagram
- The wiring diagram with power supply from USB cable
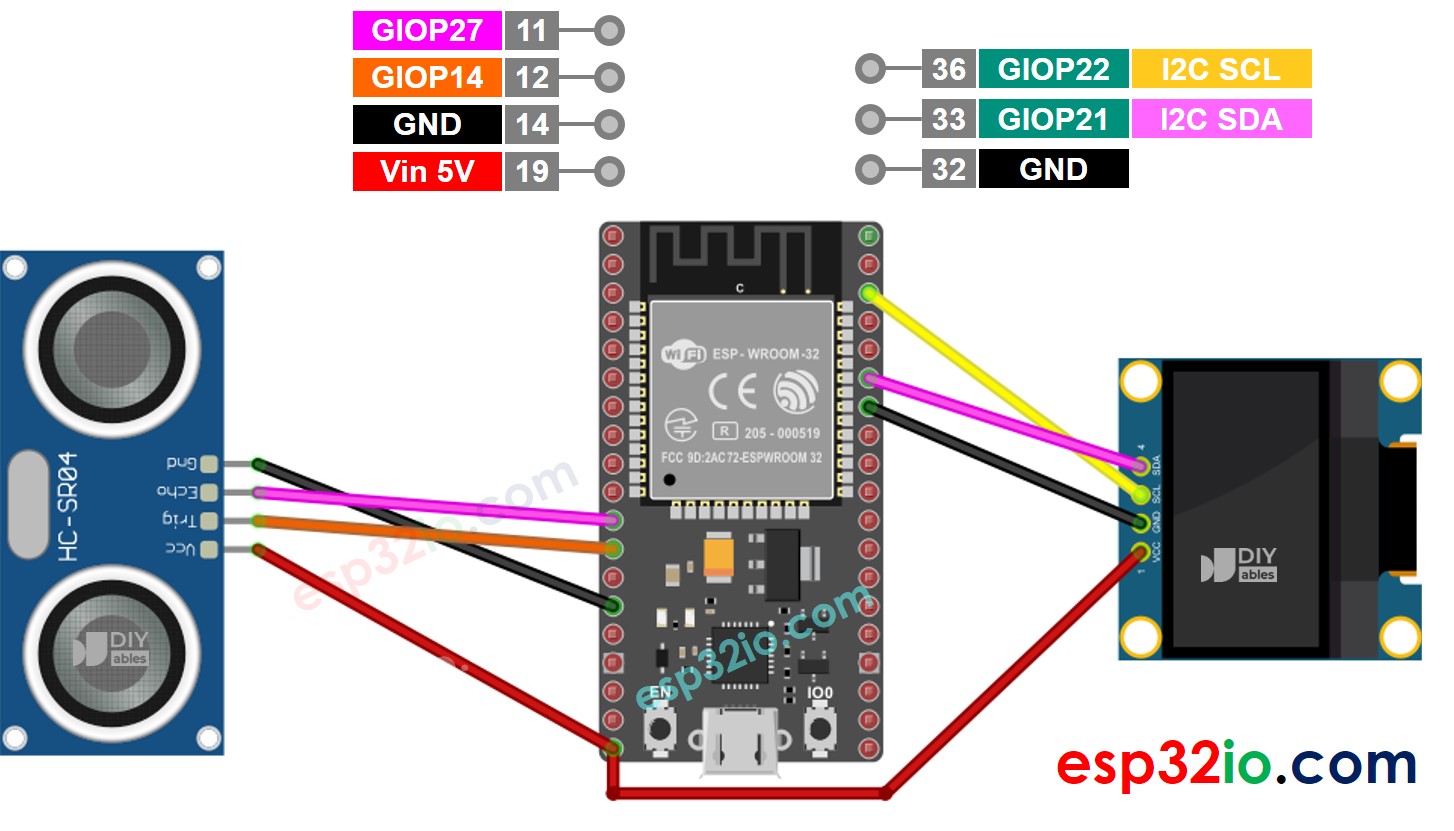
This image is created using Fritzing. Click to enlarge image
- The wiring diagram with power supply from 5v adapter
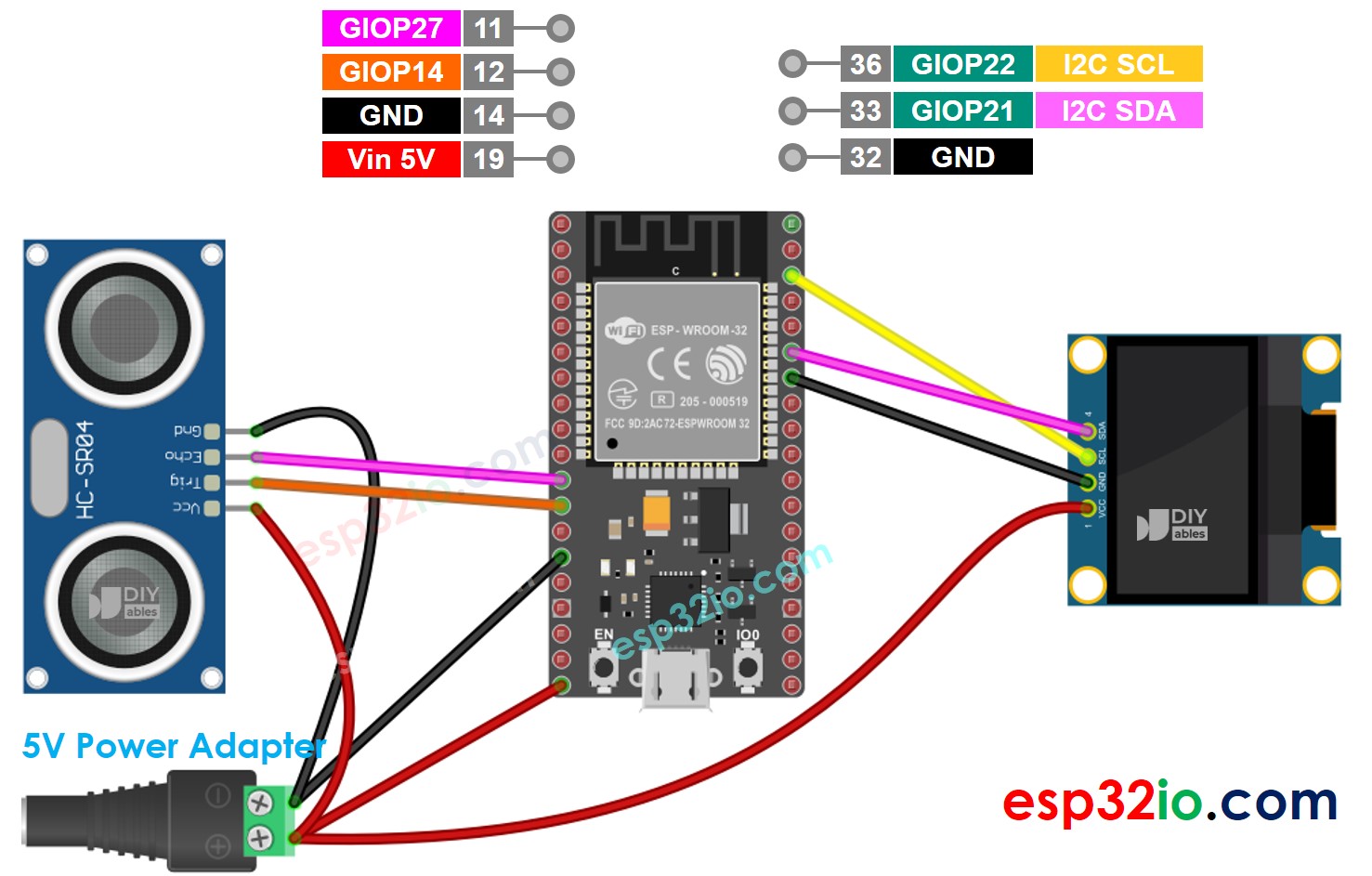
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
ESP32 Code - Ultrasonic Sensor - OLED
Quick Instructions
- Open Arduino IDE.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search “SSD1306”, then find the SSD1306 library by Adafruit
- Click Install button to install the library.
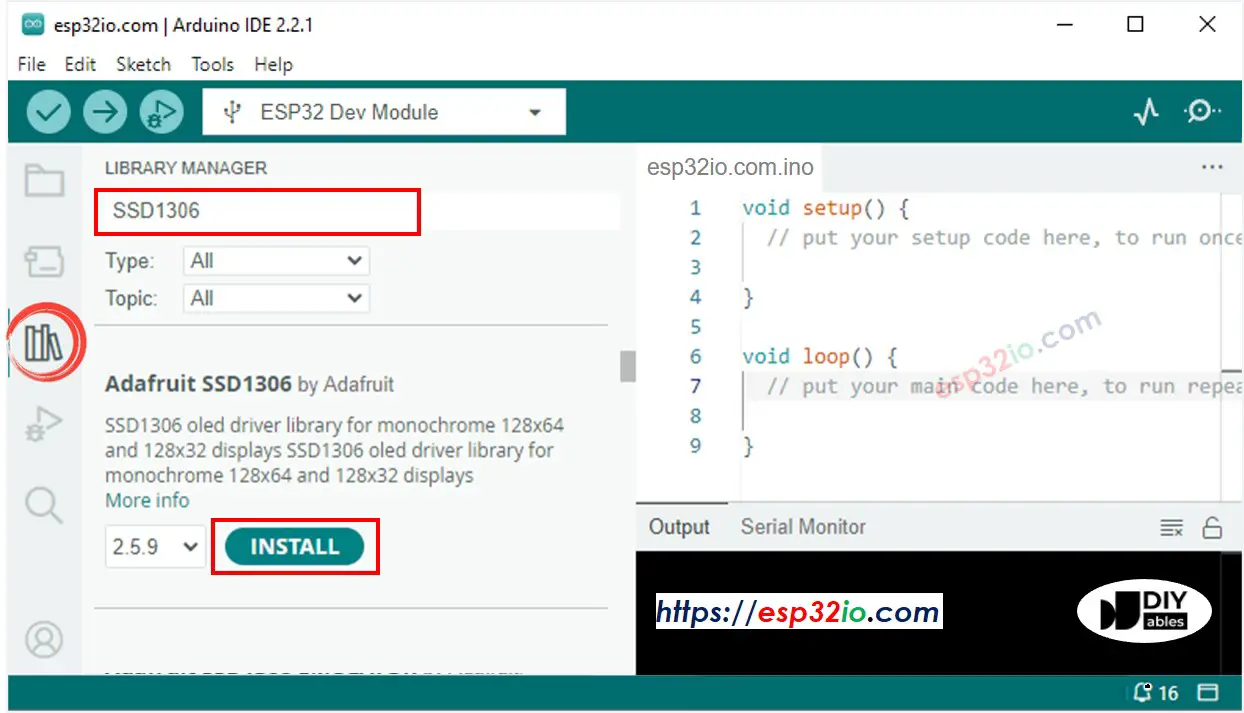
- You will be asked for intalling some other library dependencies
- Click Install All button to install all library dependencies.
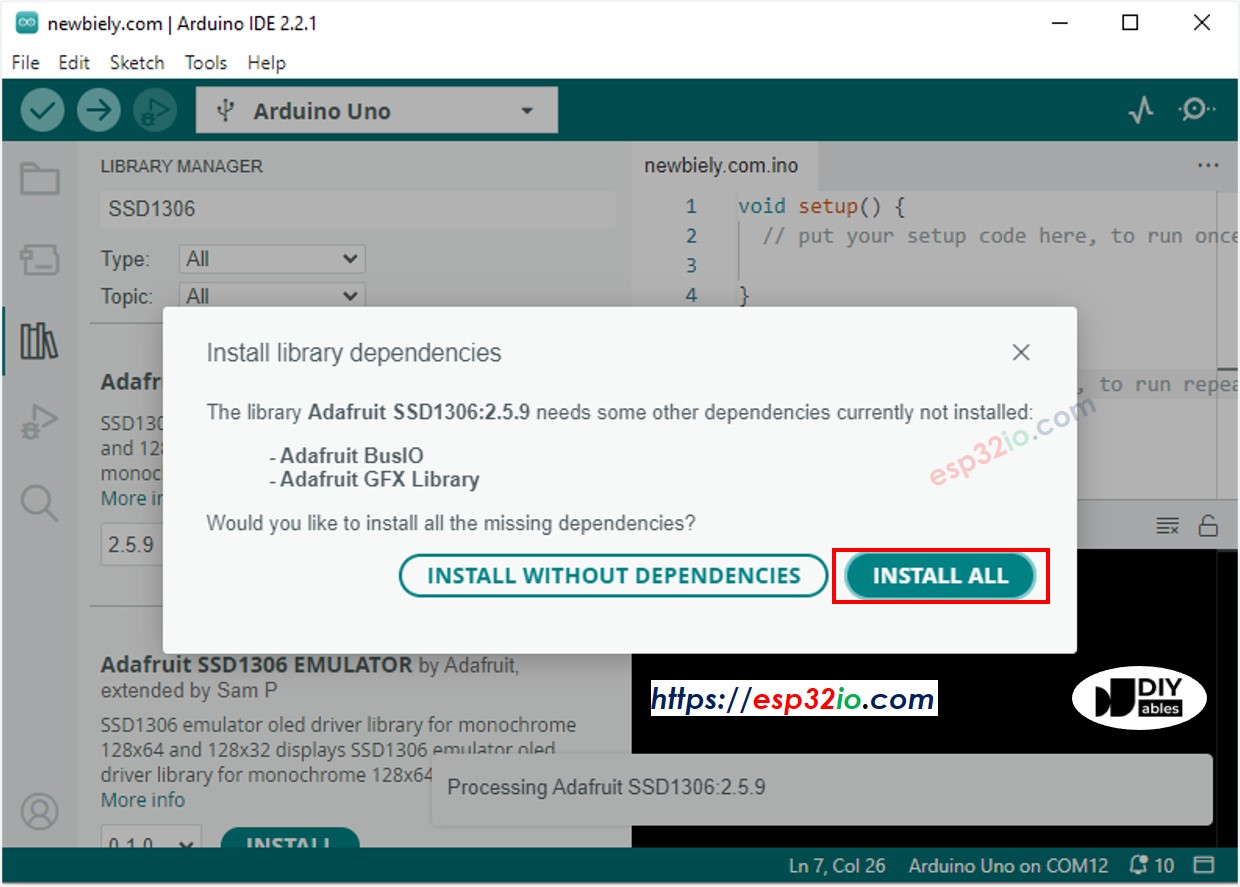
- Copy the above code and paste it to Arduino IDE
- Compile and upload code to ESP32 board by clicking Upload button on Arduino IDE
- Move your hand in front of sensor
- See the result on OLED and Serial Monitor
※ NOTE THAT:
The about code automatically horizontal and vertical center aligns the text on OLED display. See How to vertical/horizontal center on OLED for more detail.
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.