ESP32 RS422
This tutorial instructs you how to use RS422 communication with ESP32. We'll learn through the following steps:
- Connecting ESP32 to the TTL to RS422 module.
- Programming ESP32 to receive data from the TTL to RS422 module.
- Programming ESP32 to send data to the TTL to RS422 module.
- Sending data between your PC and ESP32 through RS422 bidirectionally.
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to TTL to RS422 Module
When employing serial communication on the ESP32 using functions such as Serial.print(), Serial.read(), and Serial.write(), data transmission occurs via the TX pin while data reception takes place through the RX pin. These pins function at TTL level, meaning they handle signals with a limited range. Therefore, for serial communication over longer distances, it becomes essential to convert the TTL signal to RS232, RS422, or RS485 standards.
In this guide, we will explore the use of RS422 (also referred to as RS-422) with the ESP32 by incorporating a TTL to RS422 module. This module facilitates the conversion of TTL signals to RS422 signals and vice versa.
Pinout
The RS422 to TTL module features two interfaces:
- TTL Interface (connected to ESP32):
- VCC Pin: This power pin should be connected to VCC (5V or 3.3V).
- GND Pin: This power pin should be connected to GND (0V).
- RXD Pin: This data pin should be connected to a TX pin of the ESP32.
- TXD Pin: This data pin should be connected to an RX pin of the ESP32.
- RS422 Interface:
- A (R+) Pin: This is the RX+ pin of the module. Connect this pin to the TX+ pin (T+ or Y pin) of the other RS422 device.
- B (R-) Pin: This is the RX- pin of the module. Connect this pin to the TX- pin (T- or Z pin) of the other RS422 device.
- Y (T+) Pin: This is the TX+ pin of the module. Connect this pin to the RX+ pin (R+ or A pin) of the other RS422 device.
- Z (T-) Pin: This is the TX- pin of the module. Connect this pin to the RX- pin (R- or B pin) of the other RS422 device.
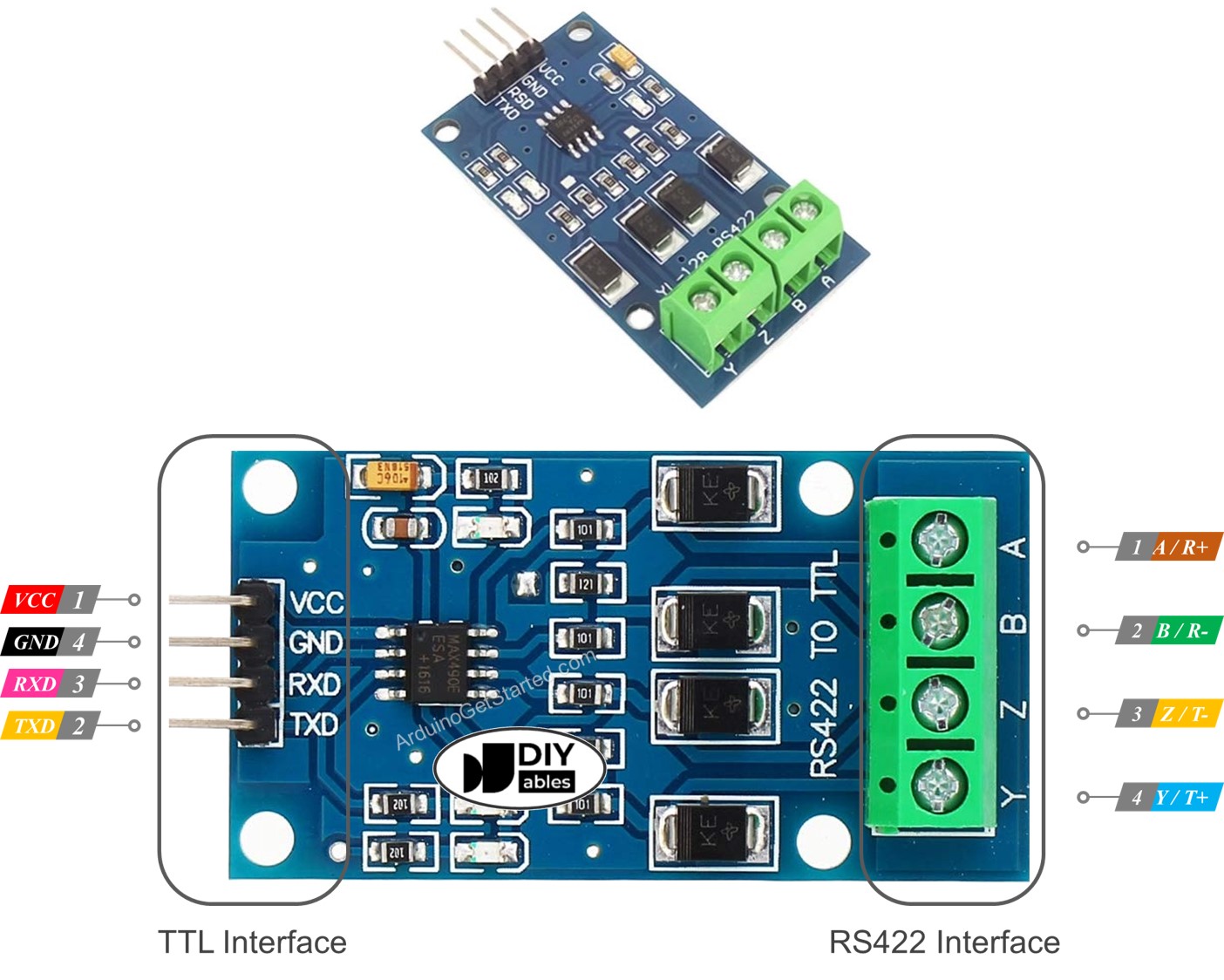
Wiring Diagram
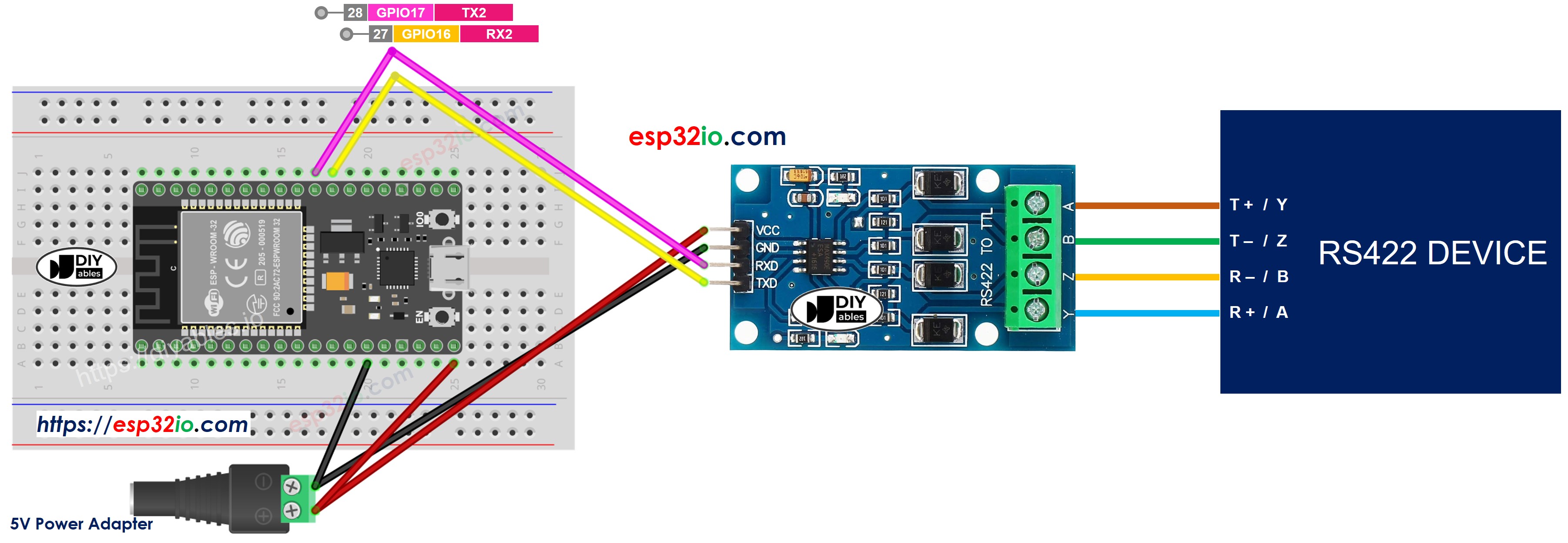
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
How To Program ESP32 to use the RS422 module
- Initializes the Serial interface:
- To read data come from RS422, you can use the following functions:
- To write data to RS422, you can use the following functions:
- And more functions to use with RS422 in Serial reference
ESP32 Code
Testing
You can do a test by sending data from your PC to ESP32 via RS-422 and vice versa. To do it, follow the below steps:
- Connect ESP32 to your PC via RS422-to-USB cable as below:
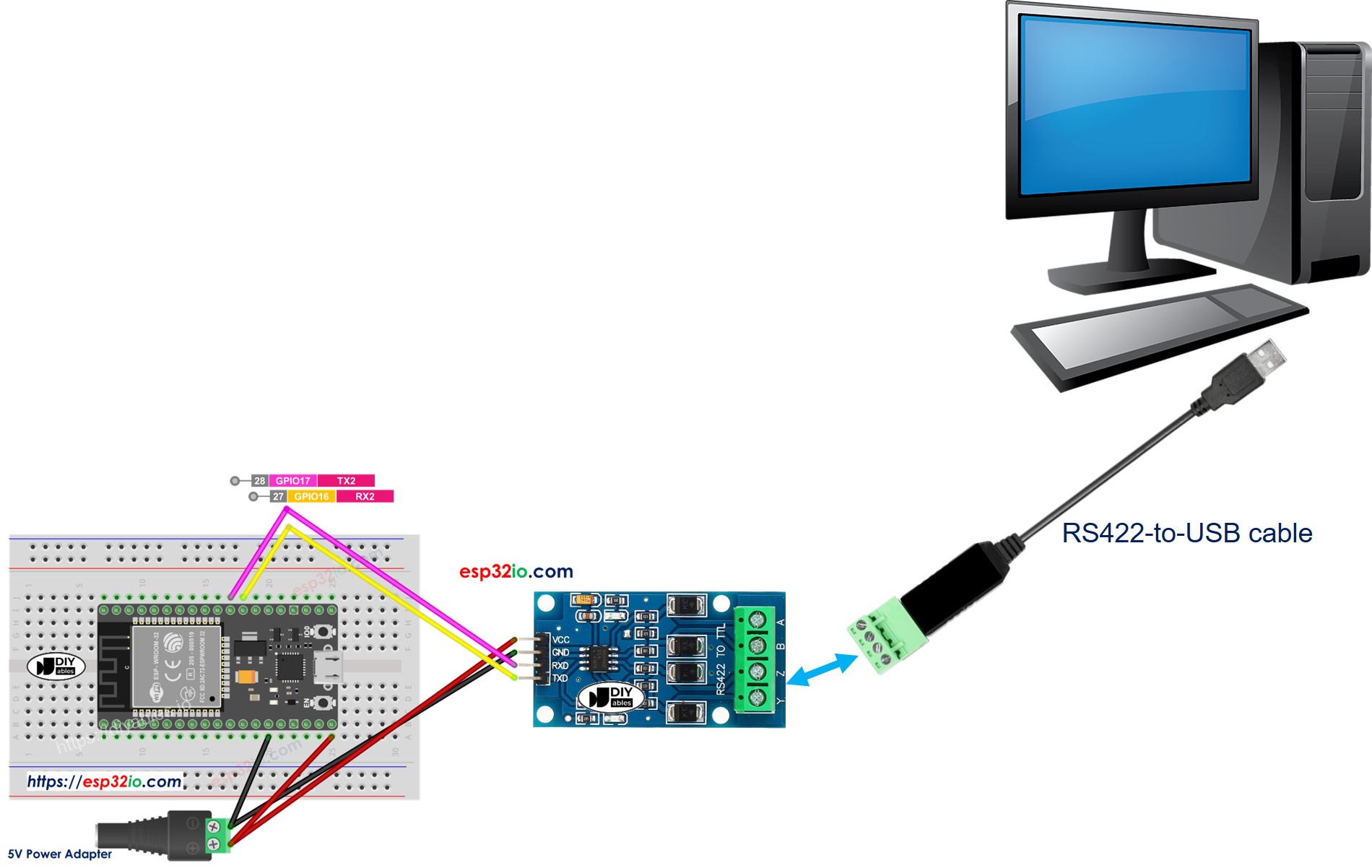
- Open the Serial Terminal Program and configure the Serial parameters (COM port, baurate...)
- Type some data from the Serial Termial to send it to ESP32.
- If successful, you will see the echo data on the Serial Terminal.
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.