ESP32 - DHT11 - Relay
In this tutorial, we are going to learn how to use ESP32 to control the relay based on the temperature read from DHT11 sensor.
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to Relay and DHT11 Sensor
If you do not know about DHT11 temperature sensor and relay (pinout, how it works, how to program ...), learn about them in the following tutorials:
Wiring Diagram
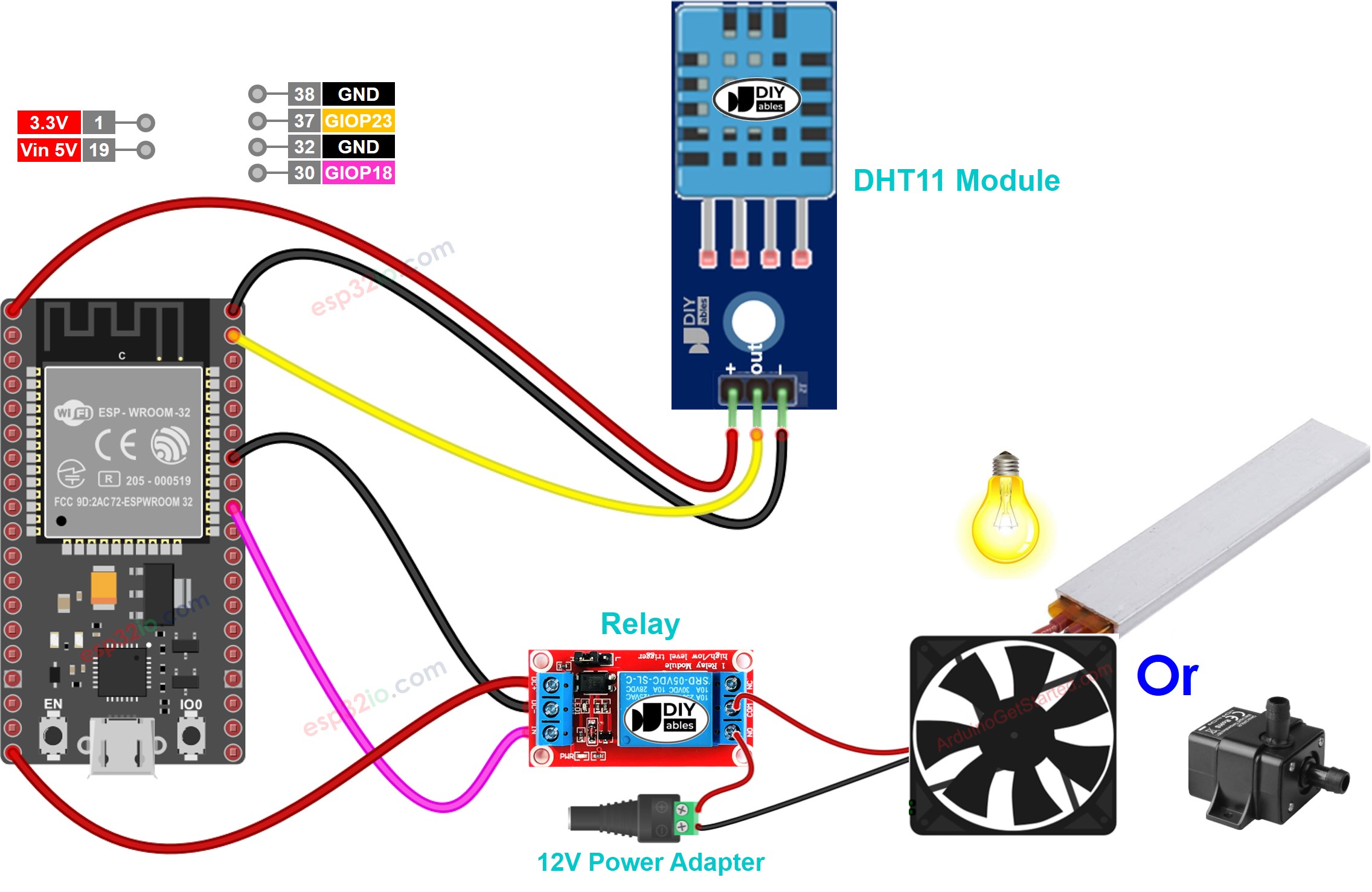
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
How System Works
- ESP32 reads the temperature from the DHT11 sensor
- If the temperature exceeds an upper threshold, ESP32 turn on the relay
- If the temperature falls below a lower threshold, ESP32 turn off the relay
The above process is repeated infinitely in the loop.
If you want to turn on and turn off the relay when the temperature is above and below a specific value respectively, you just need to set the upper threshold and lower threshold to the same value.
ESP32 Code
In the above codes, the ESP32 turn on the relay when the temperature exceeds 25°C, and keep the relay on until the temperature is below 20°C
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search “DHT”, then find the DHT sensor library by Adafruit
- Click Install button to install the library.
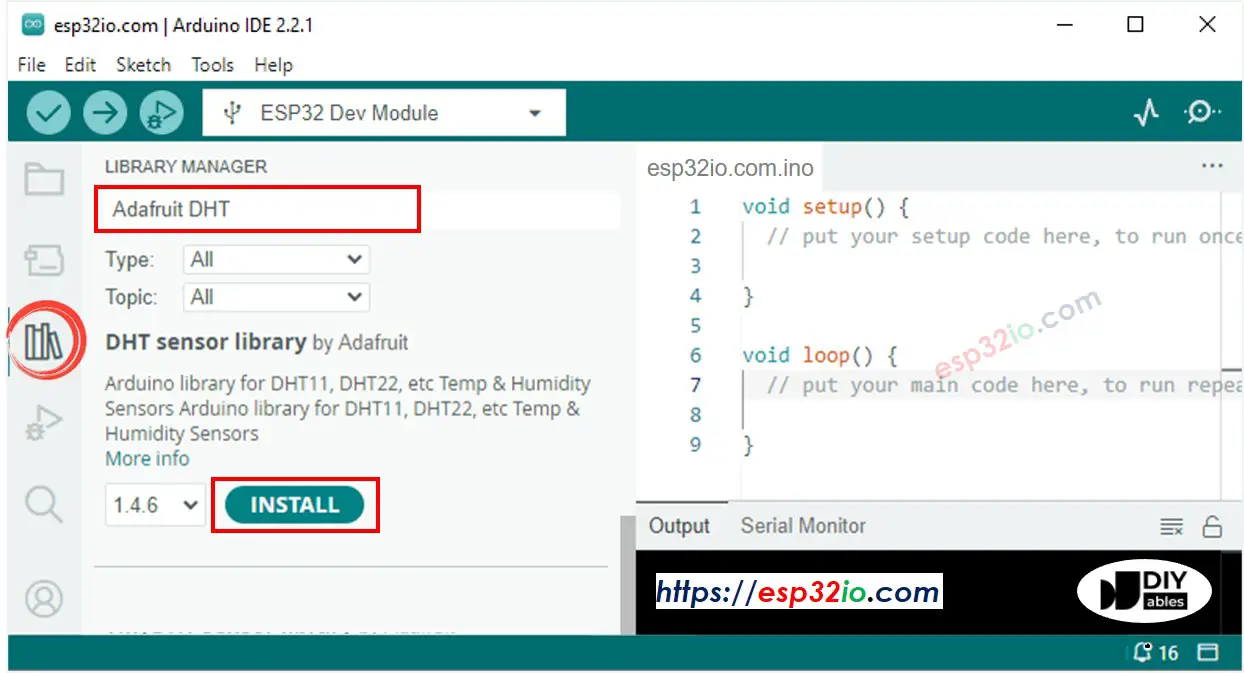
- You will be ased for intall some other library dependancies
- Click Install All button all library dependancies.
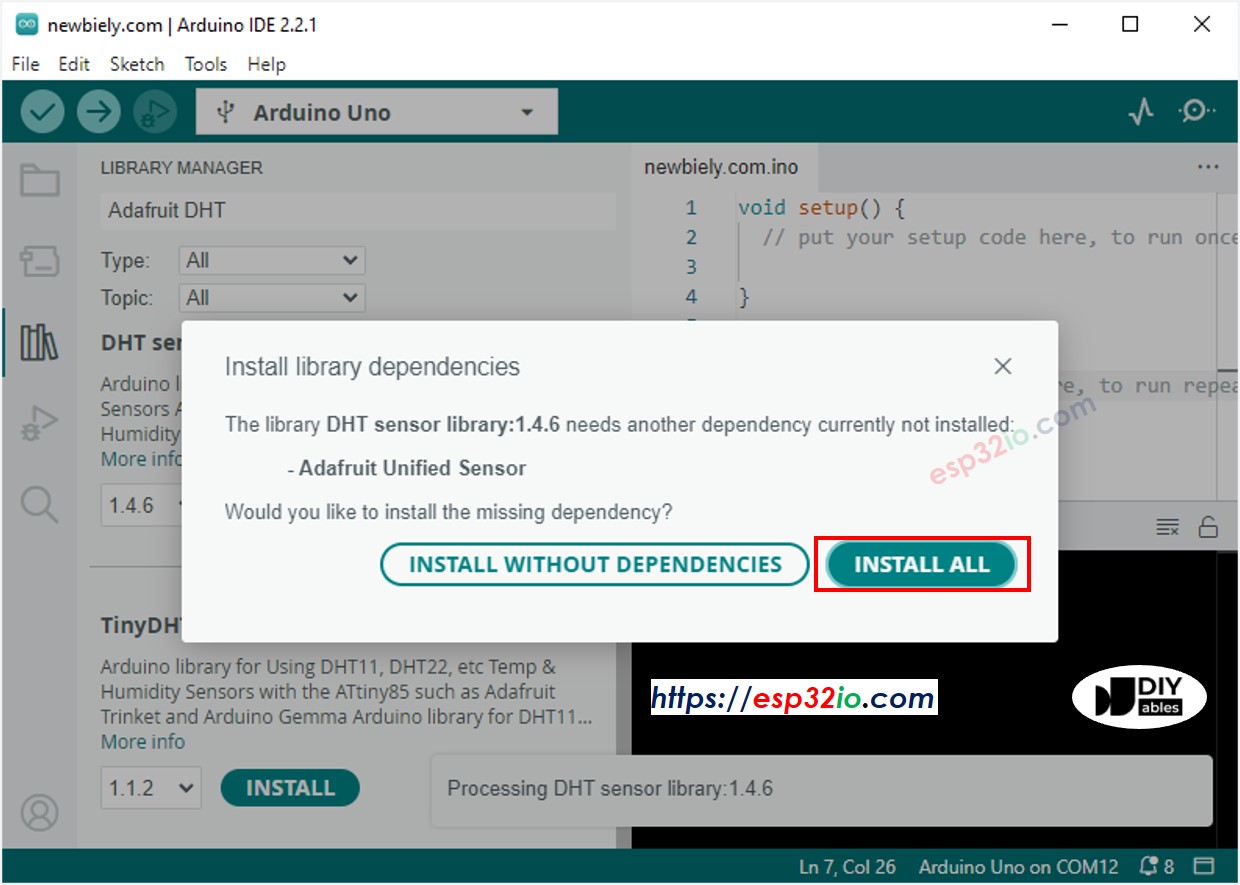
- Copy the above code corresponding to the sensor you have and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
- Make enviroment around sensor hotter or colder
- See the state of the relay
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.