ESP32 - Gas Sensor
This tutorial will guide you through the process of utilizing ESP32 and the MQ2 gas sensor to assess air quality by examining the levels of various flammable gases such as LPG, smoke, alcohol, propane, hydrogen, methane, and carbon monoxide. We will cover the following aspects in detail:
- Establishing the connection between the gas sensor and ESP32
- Programming ESP32 to retrieve readings from the gas sensor
This tutorial shows how to program the ESP32 using the Arduino language (C/C++) via the Arduino IDE. If you’d like to learn how to program the ESP32 with MicroPython, visit this ESP32 MicroPython - Gas Sensor tutorial.
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to MQ2 Gas Sensor
The MQ2 gas sensor can detect the presence of various gases like LPG, smoke, alcohol, propane, hydrogen, methane, and carbon monoxide in the surrounding environment. It offers two output options: a digital output pin and an analog output pin.
It's important to note that the MQ2 gas sensor doesn't provide specific information about each gas individually. Instead, it informs us about the combination of gases or the presence of gases as a whole.
By utilizing the MQ2 sensor, we can identify if there is a gas leak or if the air quality is poor. This information enables us to take appropriate actions to ensure our safety, such as activating an alarm or turning on ventilation systems.
Pinout
The MQ2 gas sensor consists of four pins with specific functions:
- VCC pin: This pin needs to be connected to the VCC (5V).
- GND pin: This pin needs to be connected to the GND (0V).
- DO pin: It is a digital output pin that indicates the presence of flammable gases. When flammable gas concentration is detected, the pin outputs a LOW signal; otherwise, it outputs a HIGH signal. The threshold value for detecting gas concentration can be adjusted using a built-in potentiometer.
- AO pin: It is an analog output pin that generates an analog voltage proportional to the gas concentration. When the gas concentration increases, the voltage output also increases, and when the gas concentration decreases, the voltage output decreases correspondingly.
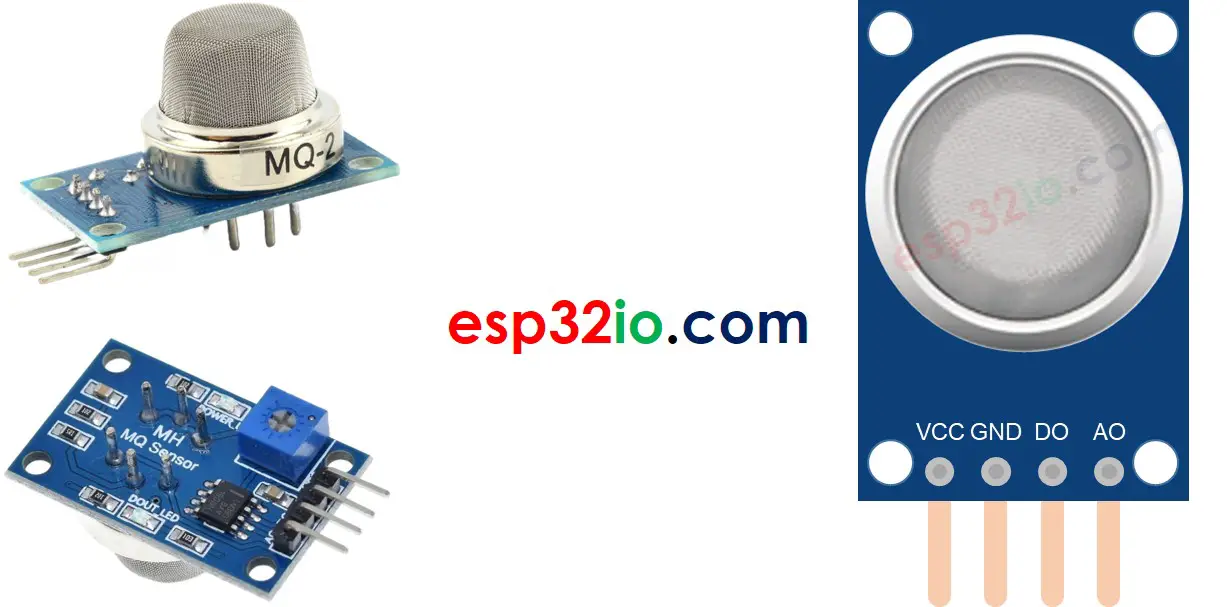
Additionally, the MQ2 gas sensor is equipped with two LED indicators:
- PWR-LED indicator: This LED serves as a power indicator, indicating that the sensor is receiving power. It is turned on when the sensor is powered and functioning.
- DO-LED indicator: This LED is linked to the DO pin of the sensor. It provides a visual representation of the gas concentration based on the value received from the DO pin. When the gas concentration is present and the DO pin is set to LOW, the DO-LED indicator turns on. Conversely, if no gas concentration is detected and the DO pin is set to HIGH, the DO-LED indicator turns off.
How It Works
Regarding the DO pin:
- The MQ2 module features a built-in potentiometer that allows you to adjust the sensitivity or threshold for gas concentration.
- If the gas concentration in the surrounding environment exceeds the set threshold, the output pin of the sensor is set to LOW, and the DO-LED turns on.
- Conversely, if the gas concentration falls below the set threshold, the output pin of the sensor is set to HIGH, and the DO-LED turns off.
Regarding the AO pin:
- As the gas concentration increases, the voltage on the AO pin also increases proportionally.
- Conversely, when the gas concentration decreases, the voltage on the AO pin decreases accordingly.
It's important to note that adjusting the potentiometer does not affect the value on the AO pin.
The MQ2 Sensor Warm-up
The MQ2 gas sensor requires a warm-up period before it can be used effectively. Here are the details:
- When using the sensor for the first time after a long period of storage (around a month or more), it is necessary to warm it up for 24-48 hours. This extended warm-up time ensures accurate operation.
- If the sensor has been used recently, the warm-up time is significantly shorter. It typically takes only 5-10 minutes for the sensor to fully warm up. During this warm-up period, the sensor may initially provide high readings, but these readings will gradually decrease until the sensor stabilizes.
To warm up the MQ2 sensor, simply connect its VCC and GND pins to a power supply or connect them to the VCC and GND pins of an ESP32. Allow the sensor to remain in this state for the required warm-up period.
Wiring Diagram
Since the MQ2 gas sensor module has two outputs, you can choose to use one or both of them, depending on what you need.
- How to connect ESP32 and gas sensor using breadboard (powered via USB cable)
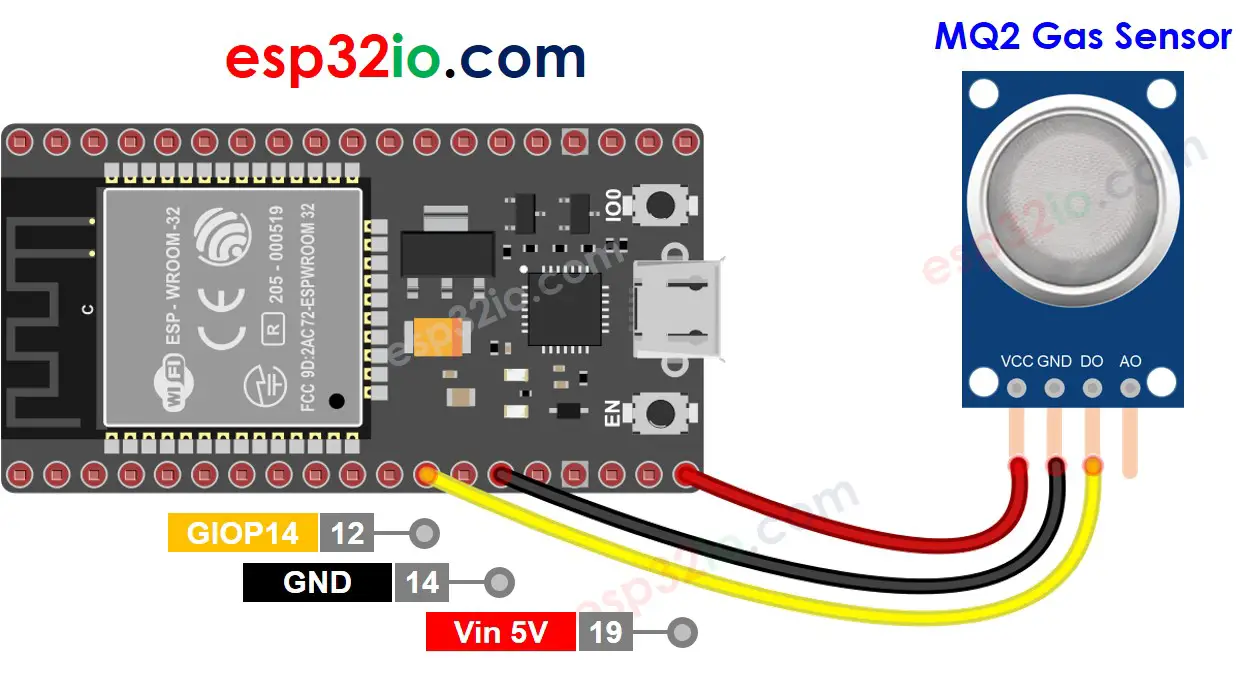
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and gas sensor using breadboard (powered via Vin pin)
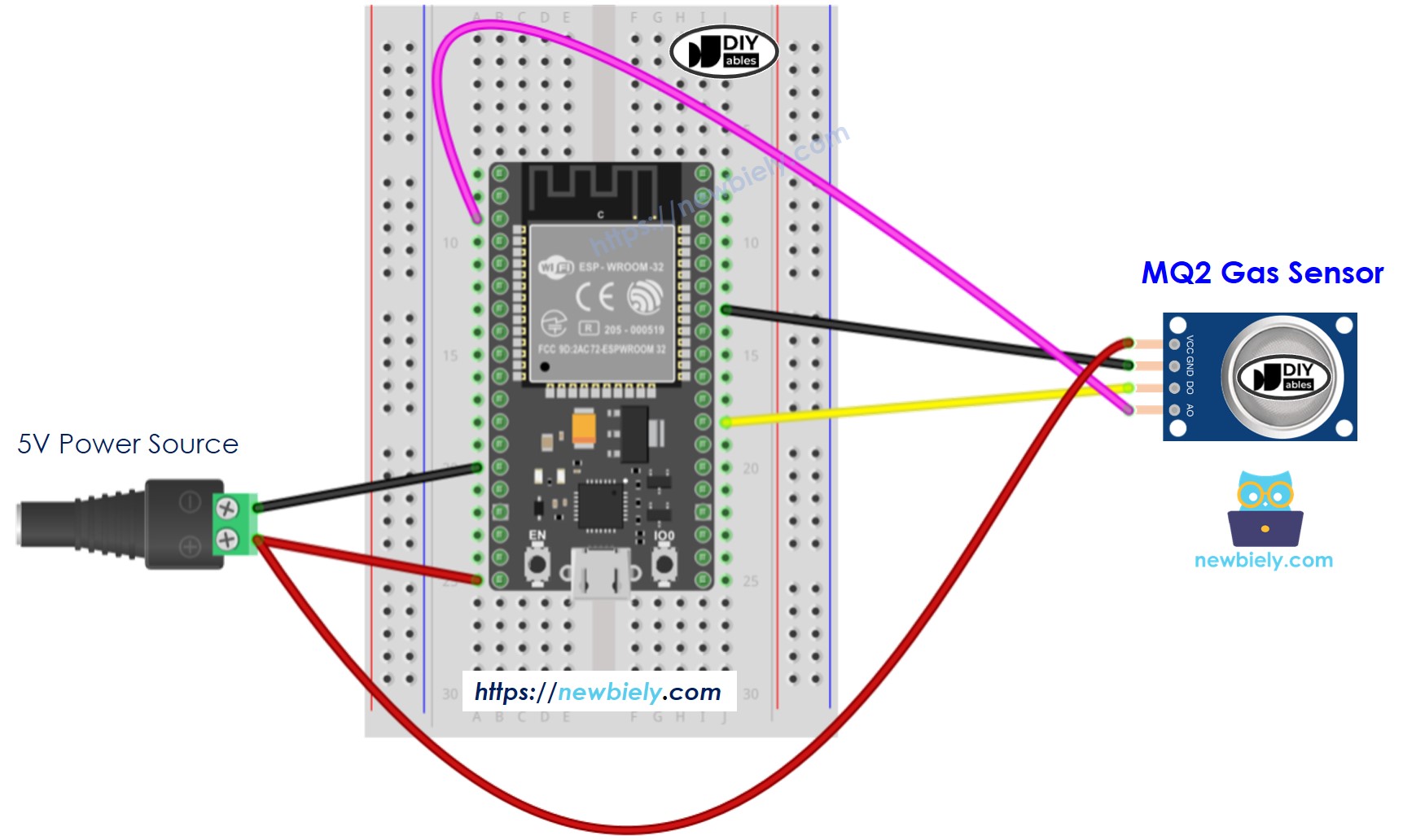
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and gas sensor using screw terminal block breakout board (powered via USB cable)
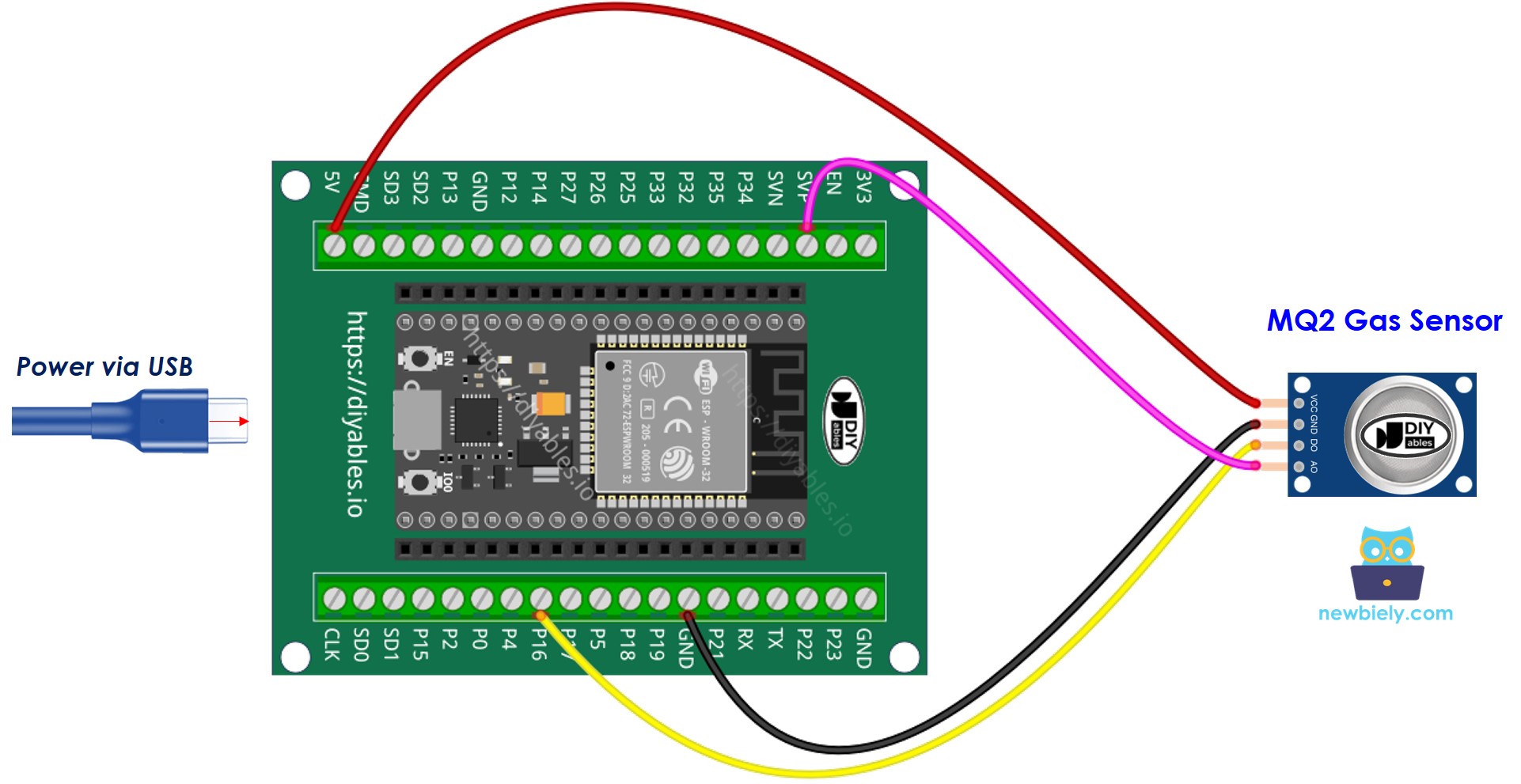
- How to connect ESP32 and gas sensor using screw terminal block breakout board (powered via Vin pin)
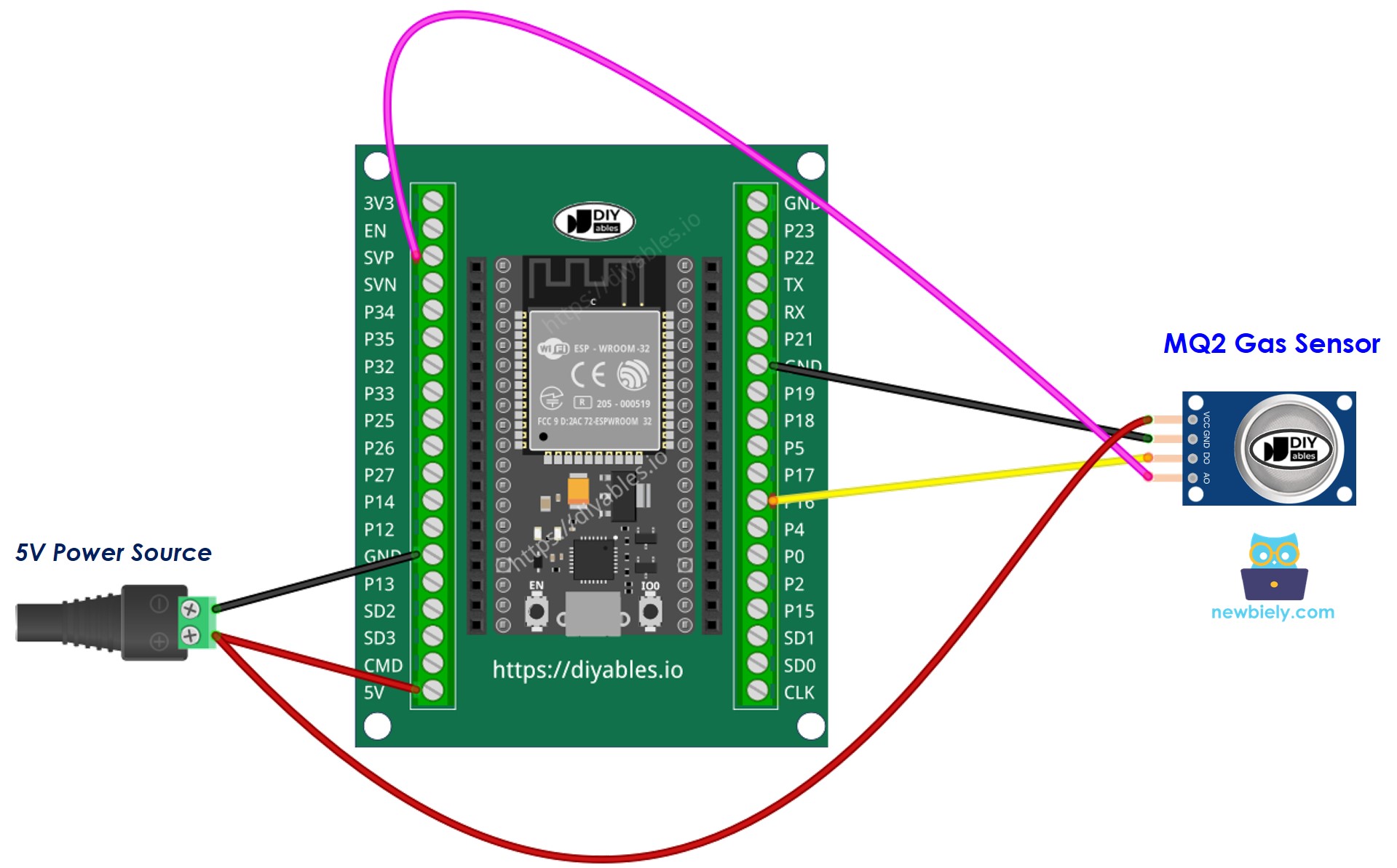
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
ESP32 Code - Read value from DO pin
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
- Place the MQ2 gas sensor near the smoke/gas you want to detect
- See the result on Serial Monitor.
Please keep in mind that if you notice the LED status remaining on constantly or off, you can adjust the potentiometer to fine-tune the sensitivity of the sensor.
ESP32 Code - Read value from AO pin
Quick Instructions
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
- Place the MQ2 gas sensor near the smoke/gas you want to detect
- See the result on Serial Monitor.
From values read from DO or AO, you can infer the air quality based on your standard, or trigger an alarm or turn on ventilation systems.
※ NOTE THAT:
This tutorial uses the analogRead() function to read values from an ADC (Analog-to-Digital Converter) connected to a gas sensor. The ESP32 ADC is good for projects that do NOT need high accuracy. However, for projects that need precise measurements, please note:
- The ESP32 ADC is not perfectly accurate and might need calibration for correct results. Each ESP32 board can be a bit different, so you need to calibrate the ADC for each individual board.
- Calibration can be difficult, especially for beginners, and might not always give the exact results you want.
For projects that need high precision, consider using an external ADC (e.g ADS1115) with the ESP32 or using an Arduino, which has a more reliable ADC. If you still want to calibrate the ESP32 ADC, refer to ESP32 ADC Calibration Driver
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.