ESP32 - Dotstar LED Strip
In this tutorial, we are going to learn how to use ESP32 to control DotStar RGB LED strip. In detail, we will learn:
- How to connect ESP32 to the DotStar LED Strip
- How to program ESP32 control the color and brightness of each individual LED on the led strip
- How to program ESP32 to create the comet effect for the DotStar LED strip
Hardware Used In This Tutorial
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to DotStar RGB LED Strip
Pinout
the DotStar RGB LED Strip has three pins:
- GND pin: needs to be connected to GND (0V)
- CI pin: Clock pin that receives the clock signal. It should be connected to an ESP32 pin.
- DI pin: Data pin that receives the control signal. It should be connected to an ESP32 pin.
- 5V pin: needs to be connected to 5V of external power supply
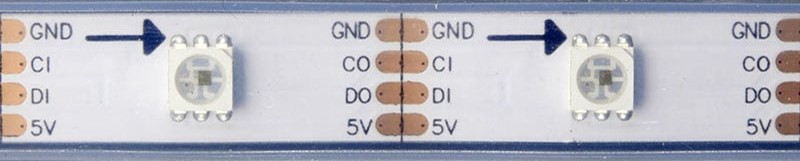
Wiring Diagram
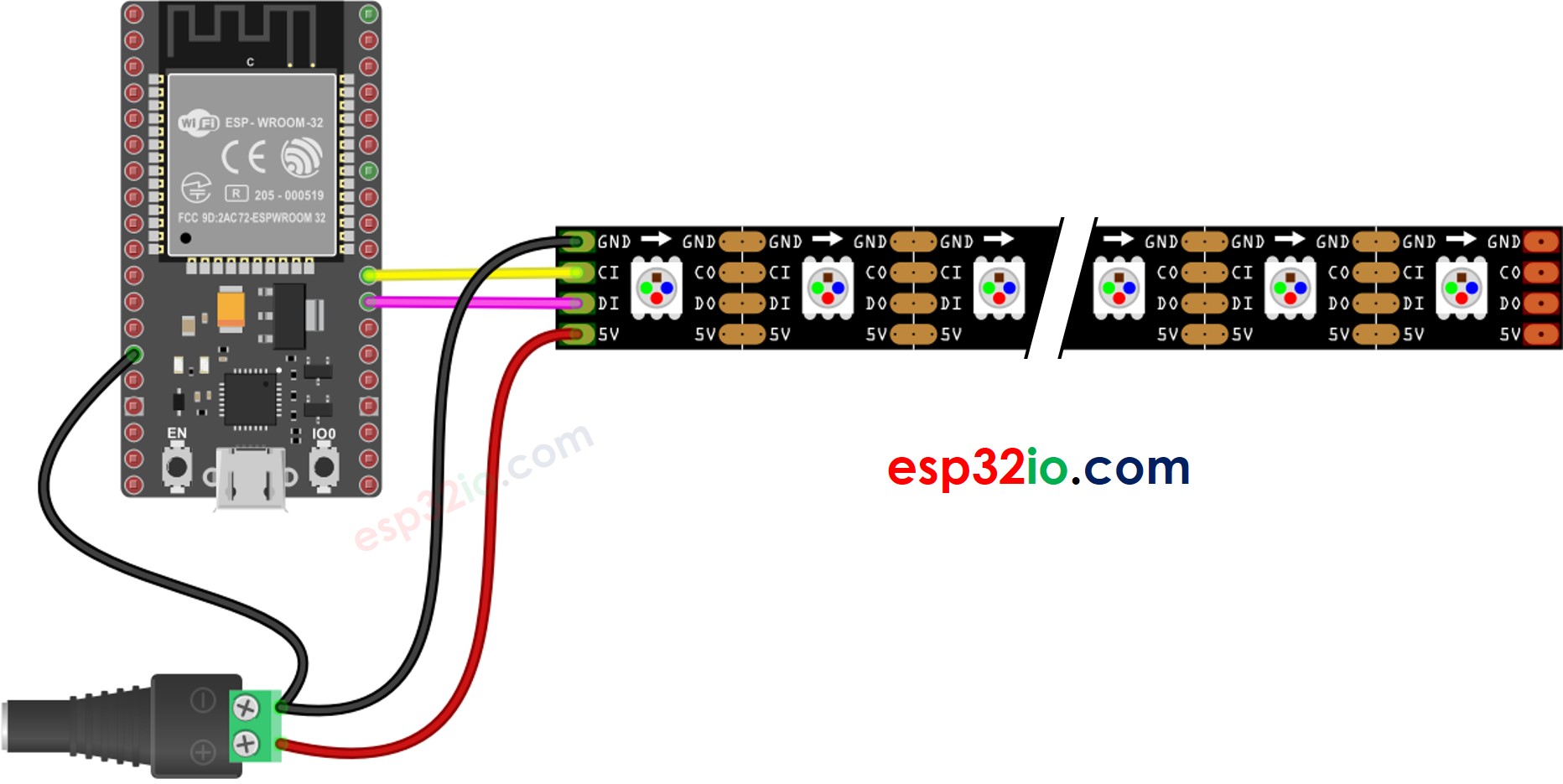
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
How To Program For DotStar RGB LED Strip
- Include a DotStar library
- Declare a DotStar object
- Initializes the DotStar
- Set color (r. g, b) of each individual LED (called pixel) .
- Set brightness of all strip.
※ NOTE THAT:
- DotStar.setBrightness() is used for all pixel on LED strip. To set the brightness for each individual pixel, we can scale the color value.
- The values set by DotStar.setBrightness() and DotStar.setPixelColor() only take effect when DotStar.show() is called.
ESP32 Code
The below code turns pixels to red one by one with a delay between each pixel
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search “Adafruit DotStar”, then find the DotStar library by Adafruit
- Click Install button to install DotStar library.
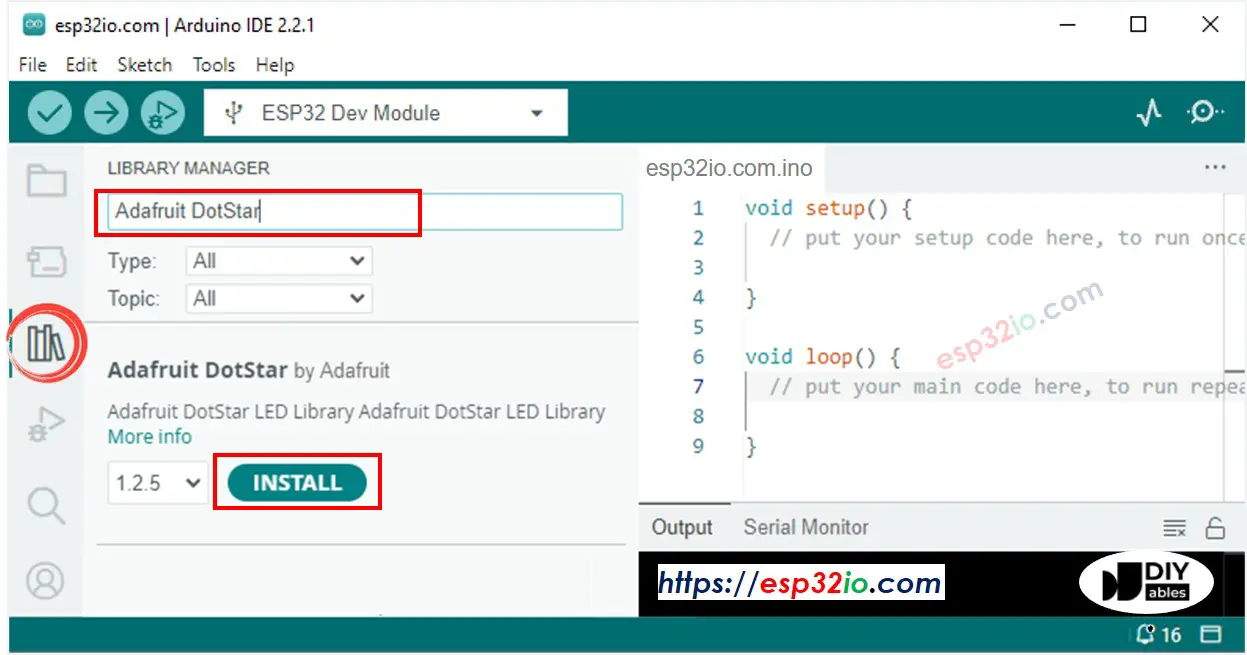
- You will be asked to install the dependency. Click Install All button.
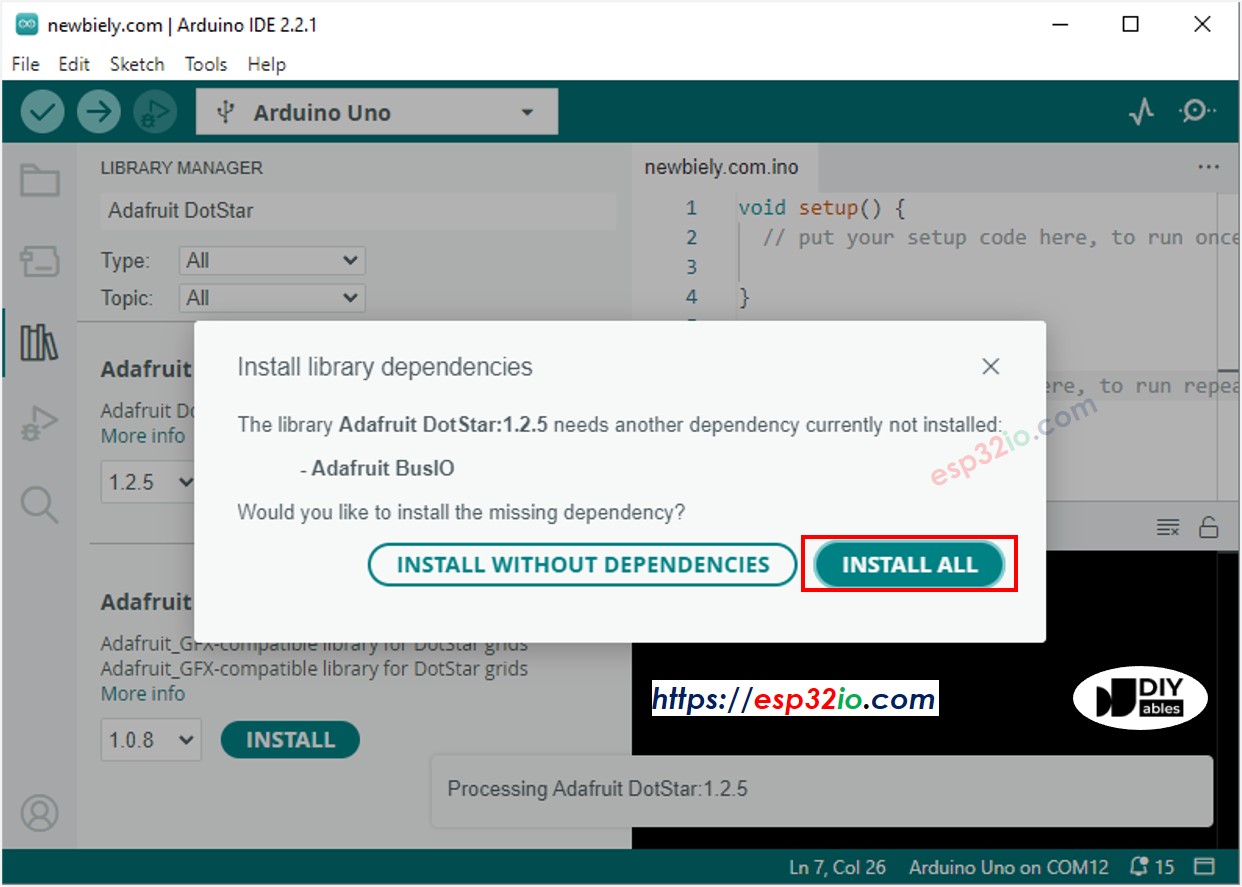
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
- See the LED effect
ESP32 Code - LED Strip Comet Effect
The below code provides the comet effect for the DotStar LED strip
※ NOTE THAT:
For any other LED effects, we offer the paid programming service
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.