ESP32 - HTTP Request
This tutorial instructs you how to use ESP32 to make HTTP request to web server, API, or Web service. In detail, You will learn:
- How to use ESP32 to make HTTP request (GET and POST)
- How to include the sensor's data into HTTP request
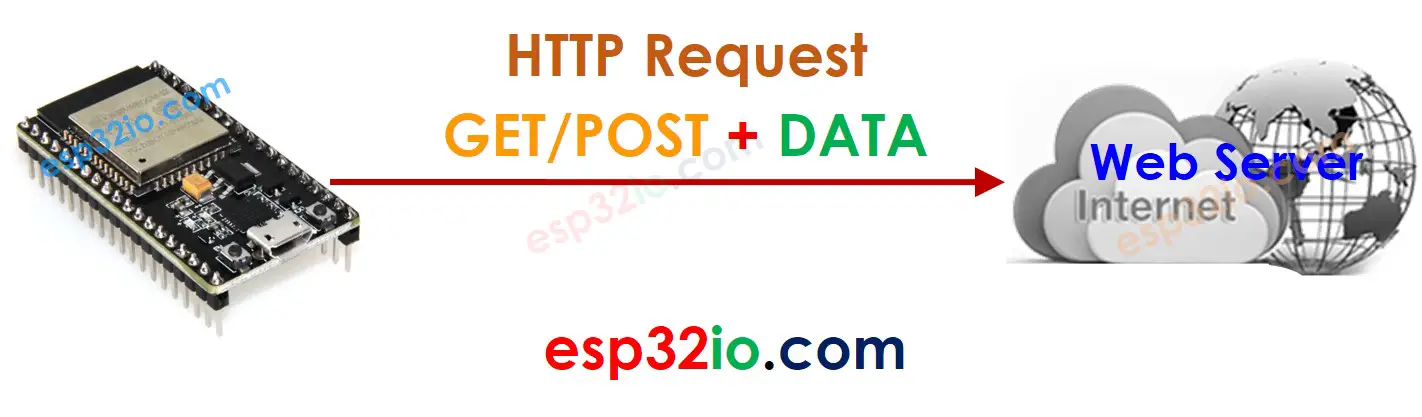
Hardware Used In This Tutorial
1 | × | ESP-WROOM-32 Dev Module | |
1 | × | USB Cable Type-C | |
1 | × | (Optional) DC Power Jack | |
1 | × | Breadboard | |
1 | × | Jumper Wires | |
1 | × | (Recommended) Screw Terminal Expansion Board for ESP32 | |
1 | × | (Recommended) Power Splitter For ESP32 |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some of the links in this section are Amazon affiliate links, meaning we may earn a commission at no additional cost to you if you make a purchase through them. Additionally, some links direct you to products from our own brand, DIYables.
Basic Concepts of Web Client and Web Server
There are some basic concepts of web such as: web address (URL), hostname, pathname, query string, HTTP Request... You can learn detailed about them in HTTP tutorial
How to Make an HTTP Request
- Include libraries
#include <WiFi.h>
#include <HTTPClient.h>
- Declare WiFi SSID and password
const char WIFI_SSID[] = "YOUR_WIFI_SSID"; // CHANGE IT
const char WIFI_PASSWORD[] = "YOUR_WIFI_PASSWORD"; // CHANGE IT
- Declare hostname, pathname, query string
String HOST_NAME = "http://YOUR_DOMAIN.com"; // CHANGE IT
String PATH_NAME = "/products/arduino"; // CHANGE IT
//String PATH_NAME = "/products/arduino.php"; // CHANGE IT
String queryString = "temperature=26&humidity=70"; // OPTIONAL
- Declare a HTTP client object
HTTPClient http;
- If connected to server, and send HTTP request. For example, HTTP GET
http.begin(HOST_NAME + PATH_NAME); //HTTP
int httpCode = http.GET();
- Read the response data from web server
// httpCode will be negative on error
if (httpCode > 0) {
// file found at server
if (httpCode == HTTP_CODE_OK) {
String payload = http.getString();
Serial.println(payload);
} else {
// HTTP header has been send and Server response header has been handled
Serial.printf("[HTTP] GET... code: %d\n", httpCode);
}
} else {
Serial.printf("[HTTP] GET... failed, error: %s\n", http.errorToString(httpCode).c_str());
}
http.end();
How to include data into HTTP request
We can send data to the web server by including data into HTTP request. The data format depends on HTTP request method:
- For HTTP GET request
- Data can be only sent in query string on the pathname.
- HTTP POST request
- Data can be sent NOT ONLY in query string format BUT ALSO any other format such as Json, XML, image ...
- Data is put in HTTP request body.
- Create a query string
- HTTP GET: add query string to pathname
- HTTP POST: put query string in HTTP body
- For both GET and POST, read the response data from web server
Let's learn how to send data in query string format for both HTTP GET and POST
int temp = // from sensor
int humi = // from sensor
String queryString = String("temperature=") + String(temp) + String("&humidity=") + String(humi);
http.begin(HOST_NAME + PATH_NAME + "?" + queryString);
http.addHeader("Content-Type", "application/x-www-form-urlencoded");
int httpCode = http.GET();
http.begin(HOST_NAME + PATH_NAME);
http.addHeader("Content-Type", "application/x-www-form-urlencoded");
int httpCode = http.POST(queryString);
// httpCode will be negative on error
if (httpCode > 0) {
// file found at server
if (httpCode == HTTP_CODE_OK) {
String payload = http.getString();
Serial.println(payload);
} else {
// HTTP header has been send and Server response header has been handled
Serial.printf("[HTTP] GET/POST... code: %d\n", httpCode);
}
} else {
Serial.printf("[HTTP] GET/POST... failed, error: %s\n", http.errorToString(httpCode).c_str());
}
http.end();
Complete ESP32 Code for Making HTTP Request
The blow is the complete ESP32 code for making HTTP GET/POST request
/*
* This ESP32 code is created by esp32io.com
*
* This ESP32 code is released in the public domain
*
* For more detail (instruction and wiring diagram), visit https://esp32io.com/tutorials/esp32-http-request
*/
#include <WiFi.h>
#include <HTTPClient.h>
const char WIFI_SSID[] = "YOUR_WIFI_SSID"; // CHANGE IT // CHANGE IT
const char WIFI_PASSWORD[] = "YOUR_WIFI_PASSWORD"; // CHANGE IT
String HOST_NAME = "http://YOUR_DOMAIN.com"; // CHANGE IT
String HOST_NAME = "http://YOUR_DOMAIN.com"; // CHANGE IT
//String PATH_NAME = "/products/arduino.php"; // CHANGE IT
void setup() {
Serial.begin(9600);
WiFi.begin(WIFI_SSID, WIFI_PASSWORD);
Serial.println("Connecting");
while(WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.print("Connected to WiFi network with IP Address: ");
Serial.println(WiFi.localIP());
HTTPClient http;
http.begin(HOST_NAME + PATH_NAME); //HTTP
int httpCode = http.GET();
// httpCode will be negative on error
if(httpCode > 0) {
// file found at server
if(httpCode == HTTP_CODE_OK) {
String payload = http.getString();
Serial.println(payload);
} else {
// HTTP header has been send and Server response header has been handled
Serial.printf("[HTTP] GET... code: %d\n", httpCode);
}
} else {
Serial.printf("[HTTP] GET... failed, error: %s\n", http.errorToString(httpCode).c_str());
}
http.end();
}
void loop() {
}
Complete ESP32 Code for Making HTTP GET Request with data
/*
* This ESP32 code is created by esp32io.com
*
* This ESP32 code is released in the public domain
*
* For more detail (instruction and wiring diagram), visit https://esp32io.com/tutorials/esp32-http-request
*/
#include <WiFi.h>
#include <HTTPClient.h>
const char WIFI_SSID[] = "YOUR_WIFI_SSID"; // CHANGE IT
const char WIFI_PASSWORD[] = "YOUR_WIFI_PASSWORD"; // CHANGE IT
String HOST_NAME = "http://YOUR_DOMAIN.com"; // CHANGE IT
String PATH_NAME = "/products/arduino"; // CHANGE IT
//String PATH_NAME = "/products/arduino.php"; // CHANGE IT
String queryString = "temperature=26&humidity=70";
void setup() {
Serial.begin(9600);
WiFi.begin(WIFI_SSID, WIFI_PASSWORD);
Serial.println("Connecting");
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.print("Connected to WiFi network with IP Address: ");
Serial.println(WiFi.localIP());
HTTPClient http;
http.begin(HOST_NAME + PATH_NAME + "?" + queryString);
http.addHeader("Content-Type", "application/x-www-form-urlencoded");
int httpCode = http.GET();
// httpCode will be negative on error
if (httpCode > 0) {
// file found at server
if (httpCode == HTTP_CODE_OK) {
String payload = http.getString();
Serial.println(payload);
} else {
// HTTP header has been send and Server response header has been handled
Serial.printf("[HTTP] GET... code: %d\n", httpCode);
}
} else {
Serial.printf("[HTTP] GET... failed, error: %s\n", http.errorToString(httpCode).c_str());
}
http.end();
}
void loop() {
}
Complete ESP32 Code for Making HTTP POST Request with data
/*
* This ESP32 code is created by esp32io.com
*
* This ESP32 code is released in the public domain
*
* For more detail (instruction and wiring diagram), visit https://esp32io.com/tutorials/esp32-http-request
*/
#include <WiFi.h>
#include <HTTPClient.h>
const char WIFI_SSID[] = "YOUR_WIFI_SSID"; // CHANGE IT
const char WIFI_PASSWORD[] = "YOUR_WIFI_PASSWORD"; // CHANGE IT
String HOST_NAME = "http://YOUR_DOMAIN.com"; // CHANGE IT
String PATH_NAME = "/products/arduino"; // CHANGE IT
//String PATH_NAME = "/products/arduino.php"; // CHANGE IT
String queryString = "temperature=26&humidity=70";
void setup() {
Serial.begin(9600);
WiFi.begin(WIFI_SSID, WIFI_PASSWORD);
Serial.println("Connecting");
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.print("Connected to WiFi network with IP Address: ");
Serial.println(WiFi.localIP());
HTTPClient http;
http.begin(HOST_NAME + PATH_NAME);
http.addHeader("Content-Type", "application/x-www-form-urlencoded");
int httpCode = http.POST(queryString);
// httpCode will be negative on error
if (httpCode > 0) {
// file found at server
if (httpCode == HTTP_CODE_OK) {
String payload = http.getString();
Serial.println(payload);
} else {
// HTTP header has been send and Server response header has been handled
Serial.printf("[HTTP] POST... code: %d\n", httpCode);
}
} else {
Serial.printf("[HTTP] POST... failed, error: %s\n", http.errorToString(httpCode).c_str());
}
http.end();
}
void loop() {
}