ESP32 - LED - Fade
This tutorial instructs you how to use:
- ESP32 fades LED by using delay() function
- ESP32 fades LED by using millis() function
- ESP32 fades LED by using ezLED library
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to LED
LED Pinout
LED includes two pins:
- Cathode(-) pin: connect this pin to GND (0V)
- Anode(+) pin: is used to control LED's state
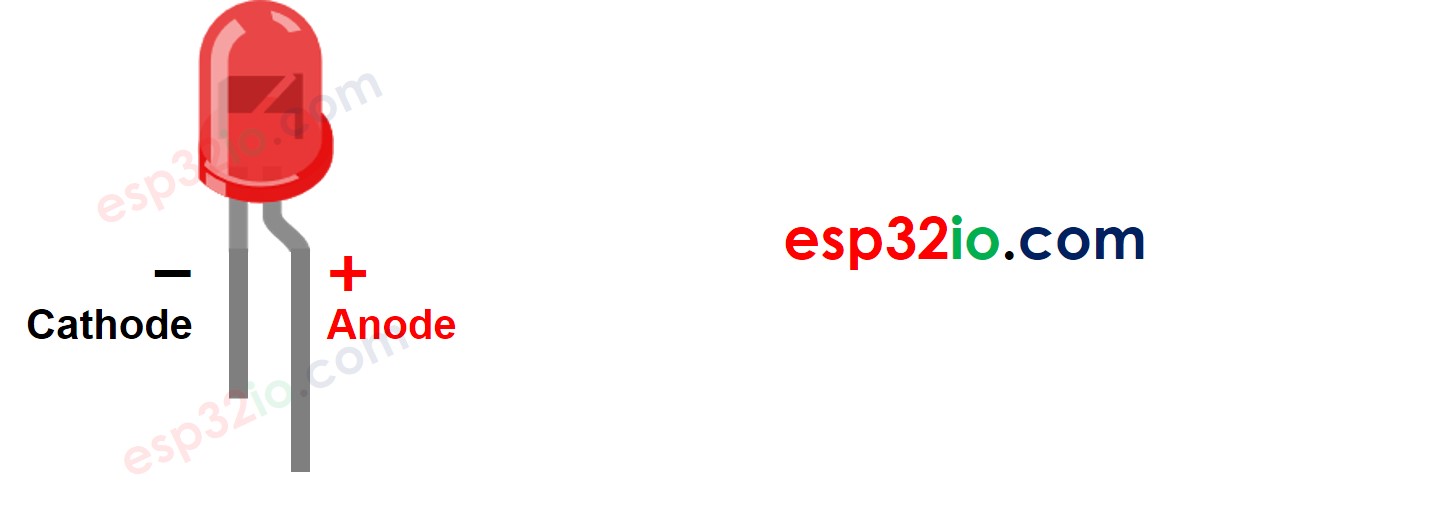
How LED Works
After connecting the cathode(-) to GND:
- If We connect GND to the anode(+), LED is OFF.
- If We connect VCC to the anode(+), LED is ON.
- If We generate a PWM signal to the anode(+) pin, the LED's brightness is in proportion to PWM duty cycle. PWM duty cycle varies from 0 to 255. The bigger the PWM duty cycle is, the brighter the LED is.
- If PWM value is 0, it is equivalent to GND ⇒ LED is OFF
- If PWM value is 255, it is equivalent to VCC ⇒ LED is ON at the highest brightness.
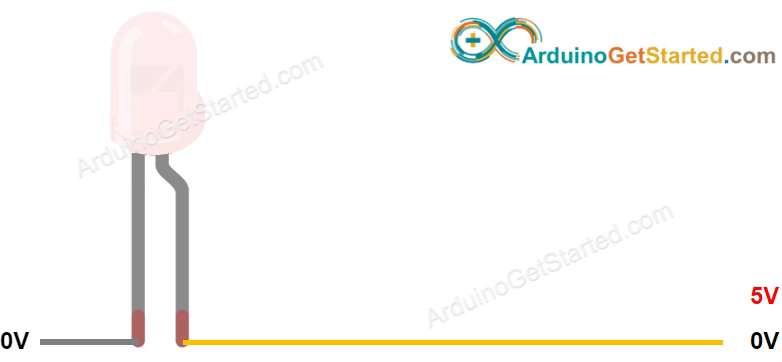
※ NOTE THAT:
Usually, it requires a resistor to protect LED from burning. The resistance's value depends on the LED's specification.
ESP32 - fade LED
The ESP32's digital output pins can be programmed to generate PWM signal. By connecting LED's anode(+) pin to an ESP32's pin, LED's cathode(-) to GND, and then programming ESP32 to change the duty cytle of PWM, we We can fade LED.
Wiring Diagram between LED and ESP32
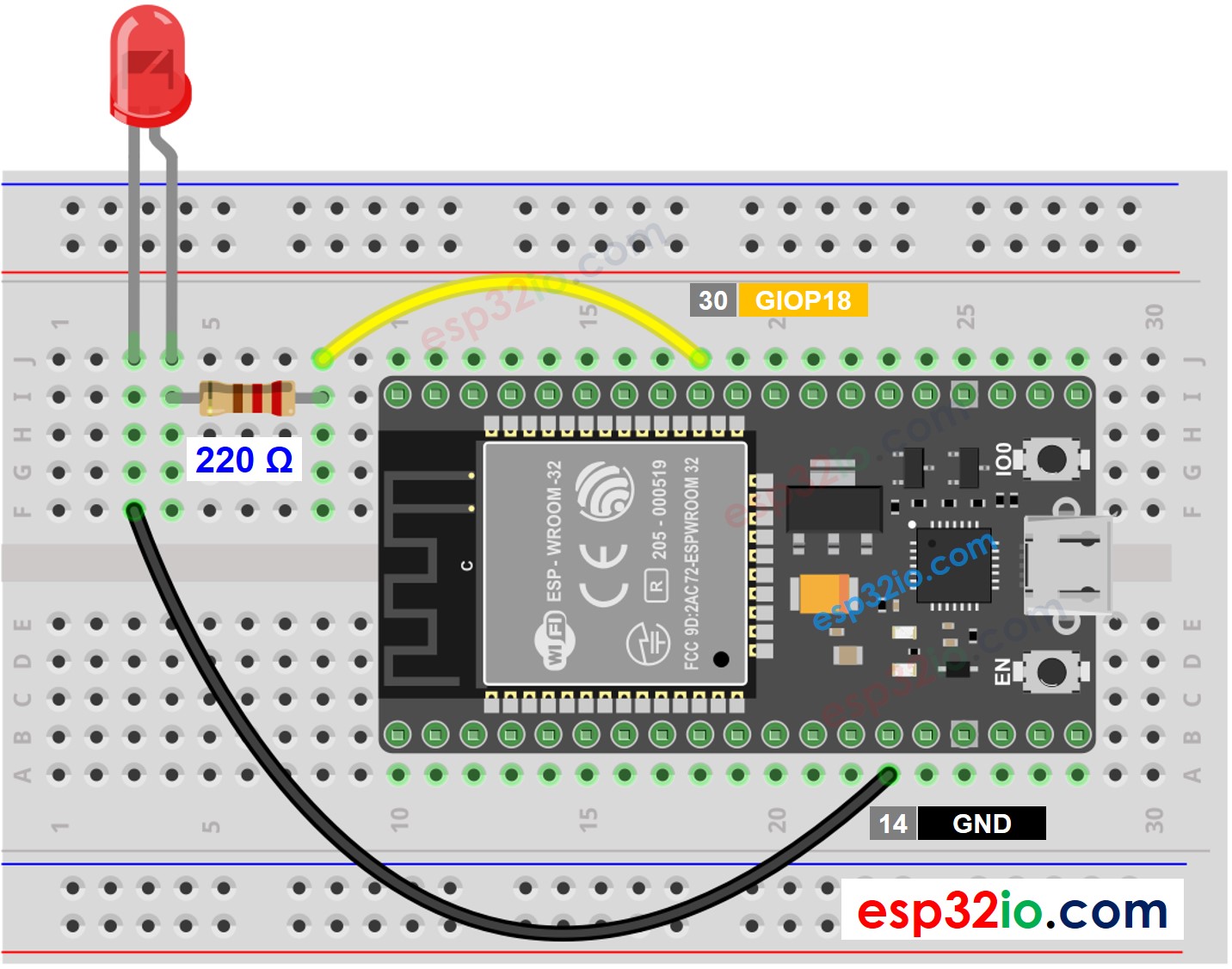
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
How To Program
- Configure an ESP32's pin as a digital output pin by using pinMode() function. For example, pin GPIO18:
- Set brightness of LED by generating PWM signal with corresponding duty cycle by using analogWrite() function:
Where brightness is a value from 0 to 255.
ESP32 Code - Simple Fade Example
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Copy the below code and paste it to Arduino IDE.
- Compile and upload code to ESP32 board by clicking Upload button on Arduino IDE
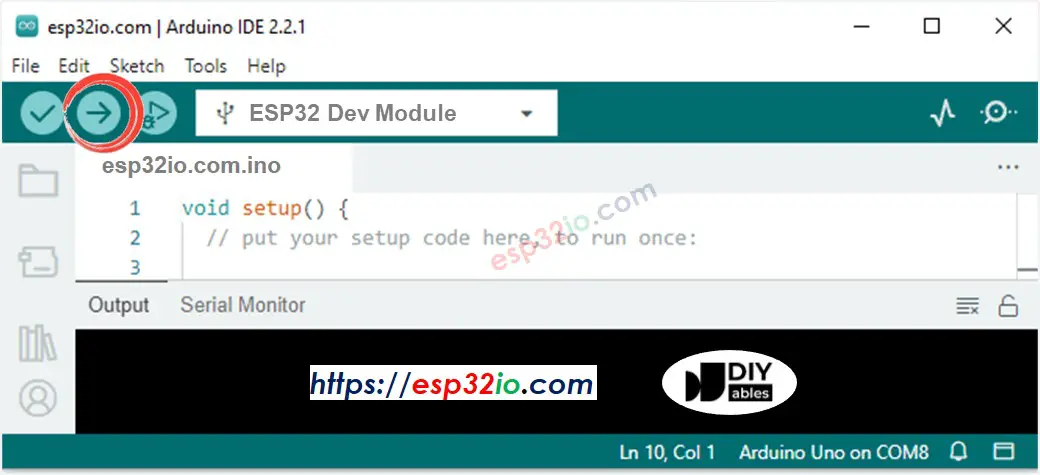
- See the brightness of LED
Line-by-line Code Explanation
The above ESP32 code contains line-by-line explanation. Please read the comments in the code!
How to fade-in LED in a period without using delay()
How to fade-out LED in a period without using delay()
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.