ESP32 - Limit Switch
This tutorial instructs you how to use the Limit Switch with ESP32.
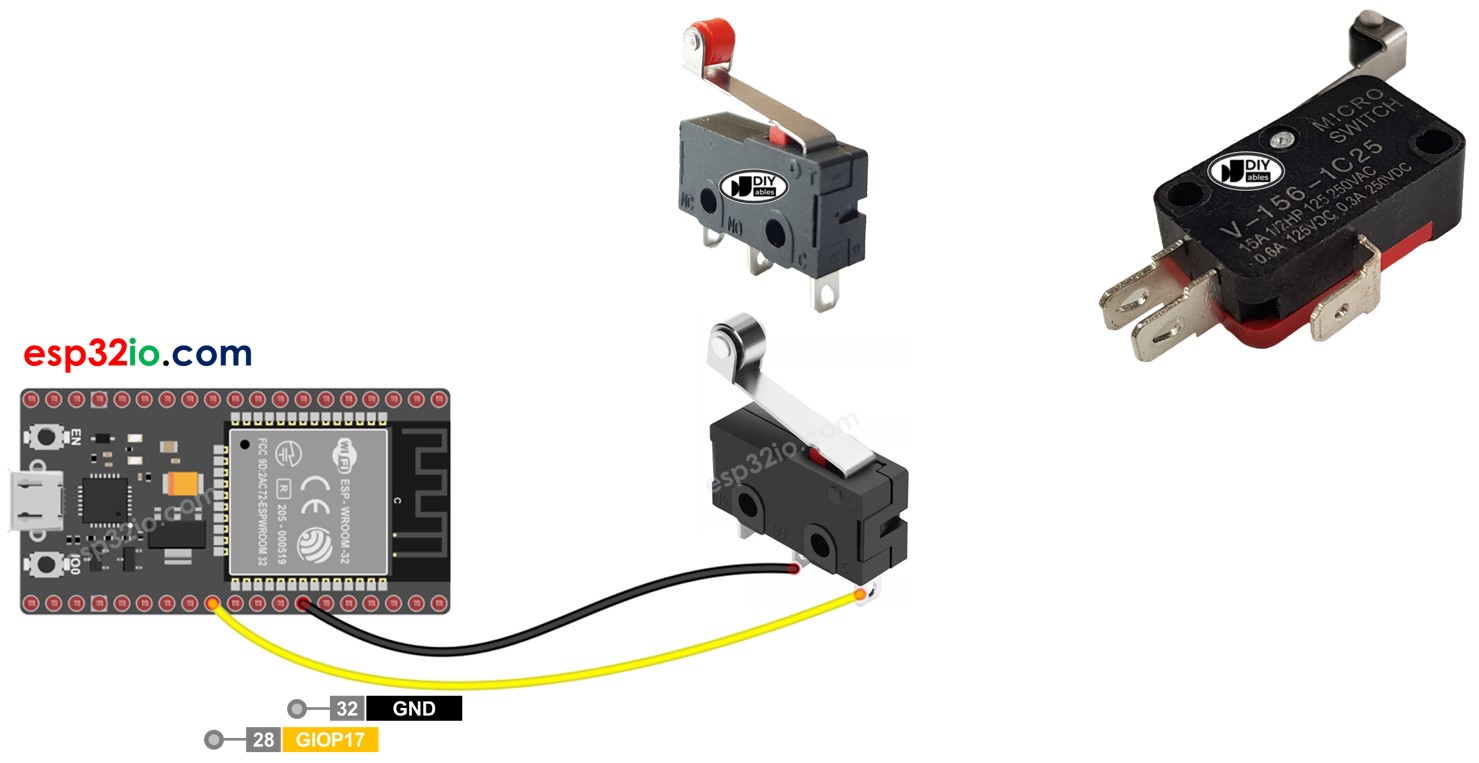
It is important to note that this tutorial is specifically about using a Limit Switch, please do not confuse with the following:
This tutorial shows how to program the ESP32 using the Arduino language (C/C++) via the Arduino IDE. If you’d like to learn how to program the ESP32 with MicroPython, visit this ESP32 MicroPython - Limit Switch tutorial.
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to Limit Switch
It is called Limit Switch because its main functionality is to detect the moving object reaching a limit.
A Limit Switch is so named because its primary purpose is to detect when a moving object has reached a limit.
Pinout
There exist various types of limit switches, but among the most commonly used are the KW12-3 and V-156-1C25. Each of these types features three pins:
- C pin: This is the common pin, it is used in both normally open and normally closed modes.
- NO pin: This is the normally open pin, it is used in the normally open mode.
- NC pin: This is the normally closed pin, it is used in the normally closed mode.
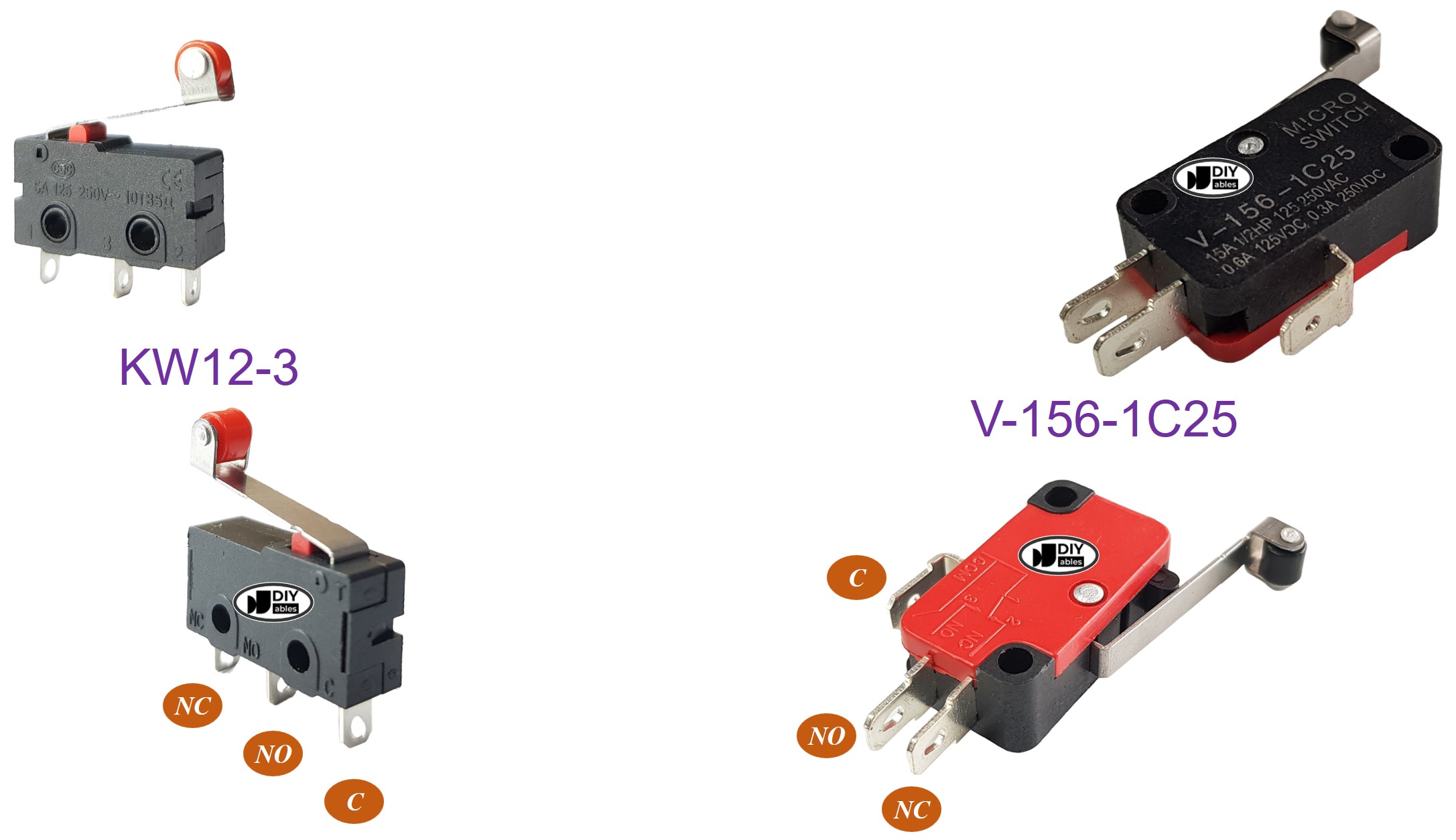
How It Works
A typical Limit Switch application typically uses only two pins, the C pin and one of the remaining two pins. This results in four different ways to use a Limit Switch. The table below illustrates the wiring and reading state on an ESP32 for each of these four methods.
C pin | NO pin | NC pin | ESP32 Input Pin's State | |
---|---|---|---|---|
1 | GND | ESP32 Input Pin (with pull-up) | not connected | HIGH when untouched, LOW when touched |
2 | GND | not connected | ESP32 Input Pin (with pull-up) | LOW when untouched, HIGH when touched |
3 | VCC | ESP32 Input Pin (with pull-down) | not connected | LOW when untouched, HIGH when touched |
4 | VCC | not connected | ESP32 Input Pin (with pull-down) | HIGH when untouched, LOW when touched |
By swapping the GND pin and ESP32 Input Pin for each of the four ways, there are a total of eight different ways to connect an ESP32 to a Limit Switch.
Out of the eight ways, the tutorial will focus on one method, specifically the first method will be used as an example.
Wiring Diagram
- How to connect ESP32 and limit switch using breadboard
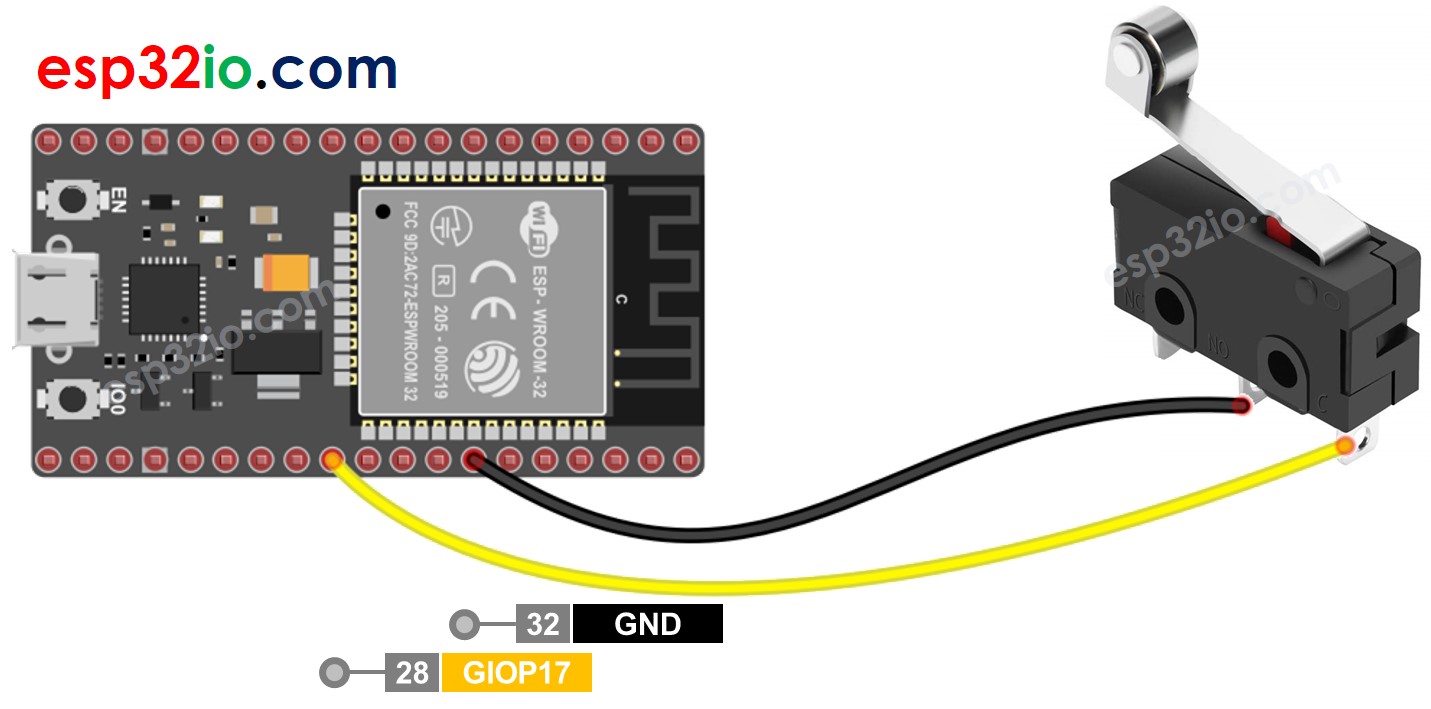
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and limit switch using screw terminal block breakout board
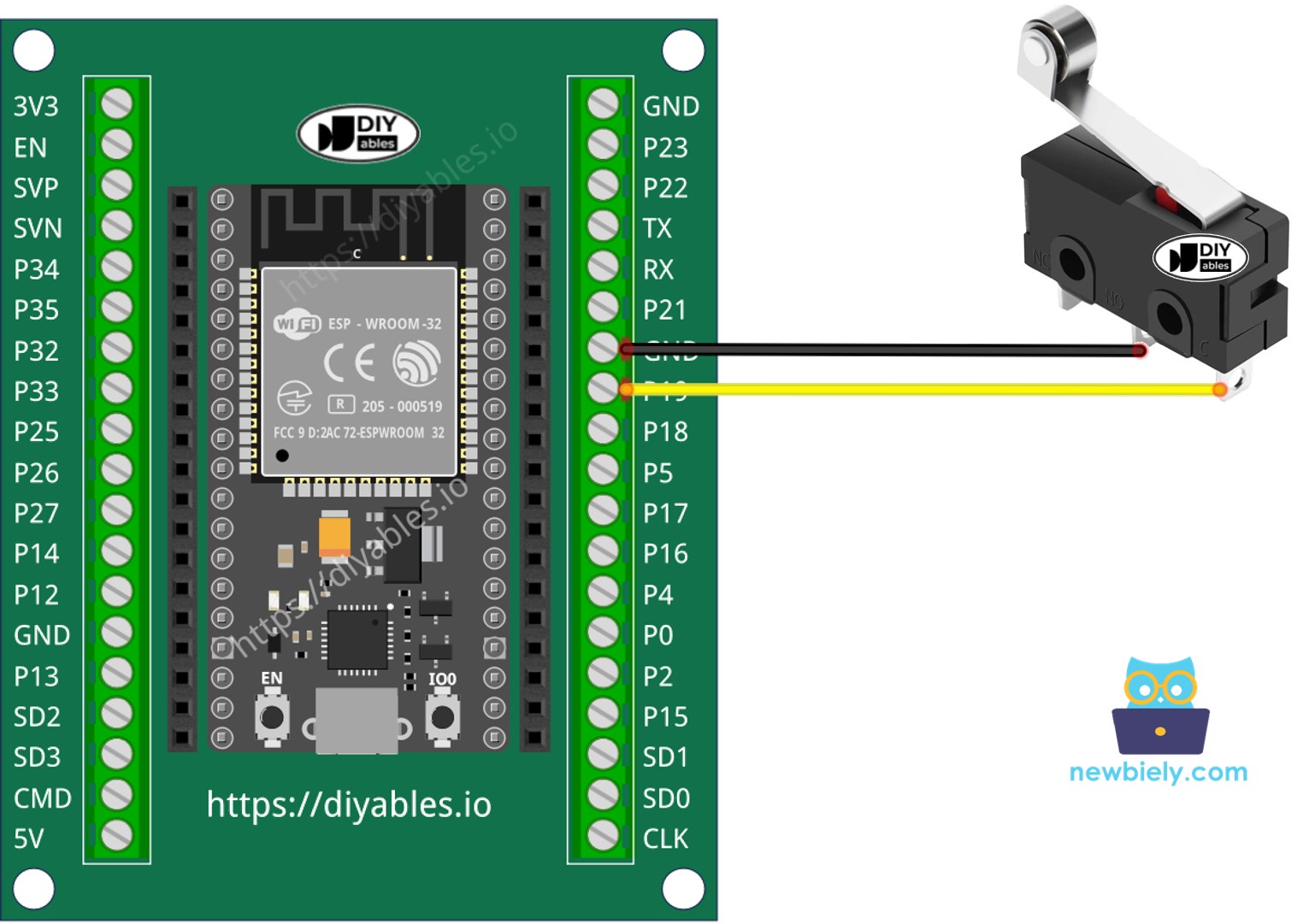
To make the wiring connection stable and firm, we recommend using to solder wires and limit switch's pin together, and then use to make it safe.
For a stable and secure connection, it is recommended to use a soldering iron to solder the wires and Limit Switch pins together, and then use heat shrink tubes for added safety.
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
ESP32 Code - Limit Switch
Just like a button, a limit switch also needs to be debounced (See more at ). Debouncing make the code complicated. Fortunately, the ezButton library supports the debouncing functionm, The library also uses internal pull-up register. These make easy to us to program
Similar to a button, a Limit Switch also requires debouncing (more information can be found at Why needs debounce for the button/limit switch?). Debouncing can make the code more complex. However, the ezButton library offers debouncing functionality and utilizes internal pull-up registers, making programming easier.
※ NOTE THAT:
Two common use cases for a Limit Switch are:
- The first use case: If the switch is TOUCHED, perform a certain action. If the input state is UNTOUCHED, perform the opposite action.
- The second use case: If the switch's state changes from UNTOUCHED to TOUCHED (or TOUCHED to UNTOUCHED), perform a specific action.
Quick Instructions
- Follow the wiring diagram provided above to connect your Limit Switch to ESP32.
- Connect your ESP32 to your PC using a USB cable.
- Open the Arduino IDE software.
- Install the ezButton library. Refer to the instructions
- Select the appropriate board and port in the Arduino IDE.
- Compile and upload the code to the ESP32 board by clicking the Upload button in the Arduino IDE.
- Test the Limit Switch by touching and releasing it.
- Observe the output on the Serial Monitor in the Arduino IDE.
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.