ESP32 - Button - Long Press Short Press
This tutorial instructs you how to use ESP32 to detect the long press and short press, In detail, we will learn:
- How to detect the short press on the button
- How to detect the long press on the button
- How to detect both the long press and short press
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Wiring Diagram
- Wiring Diagram between ESP32 and PCB-mount button
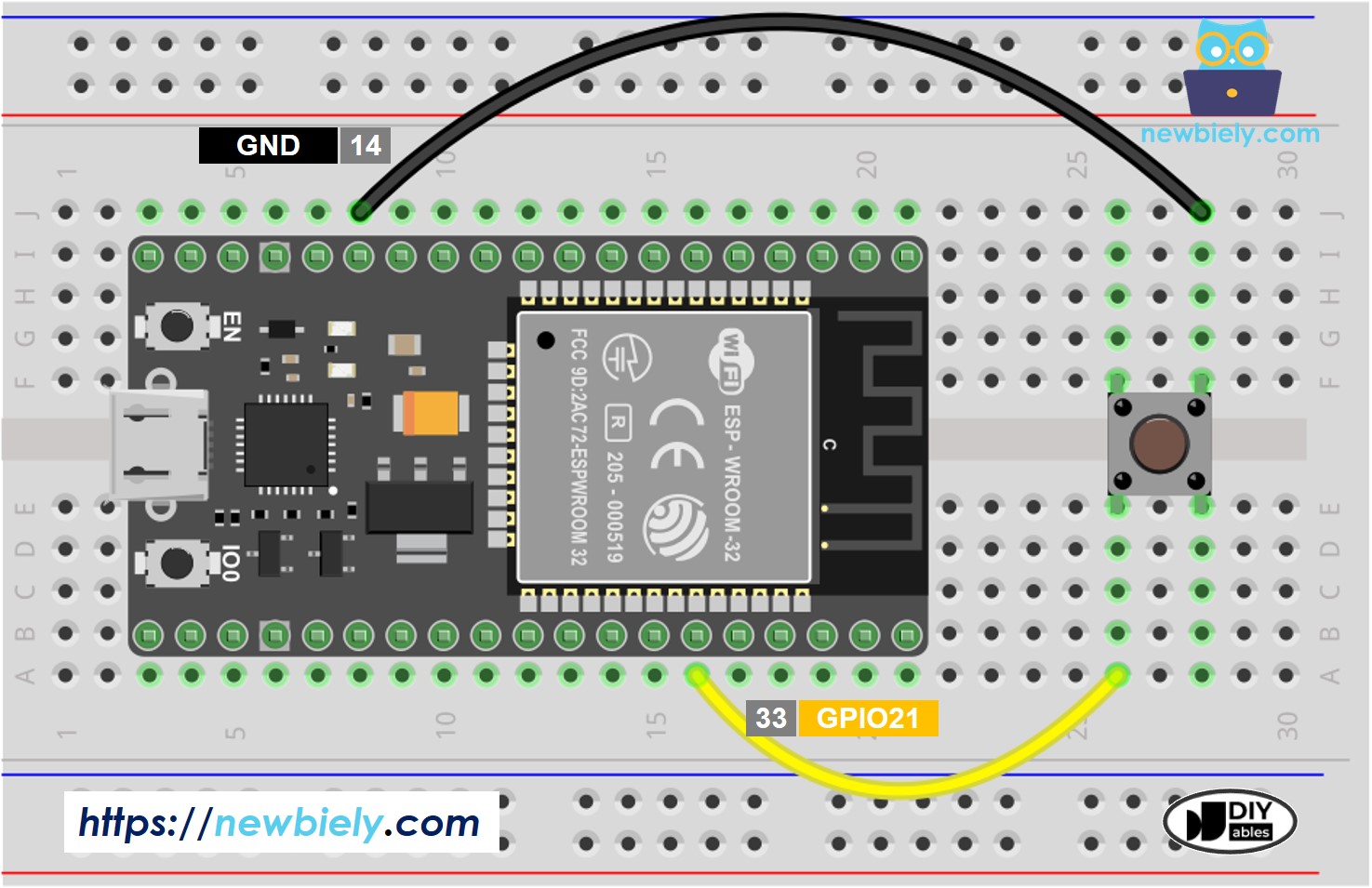
This image is created using Fritzing. Click to enlarge image
- Wiring Diagram between ESP32 and panel-mount button
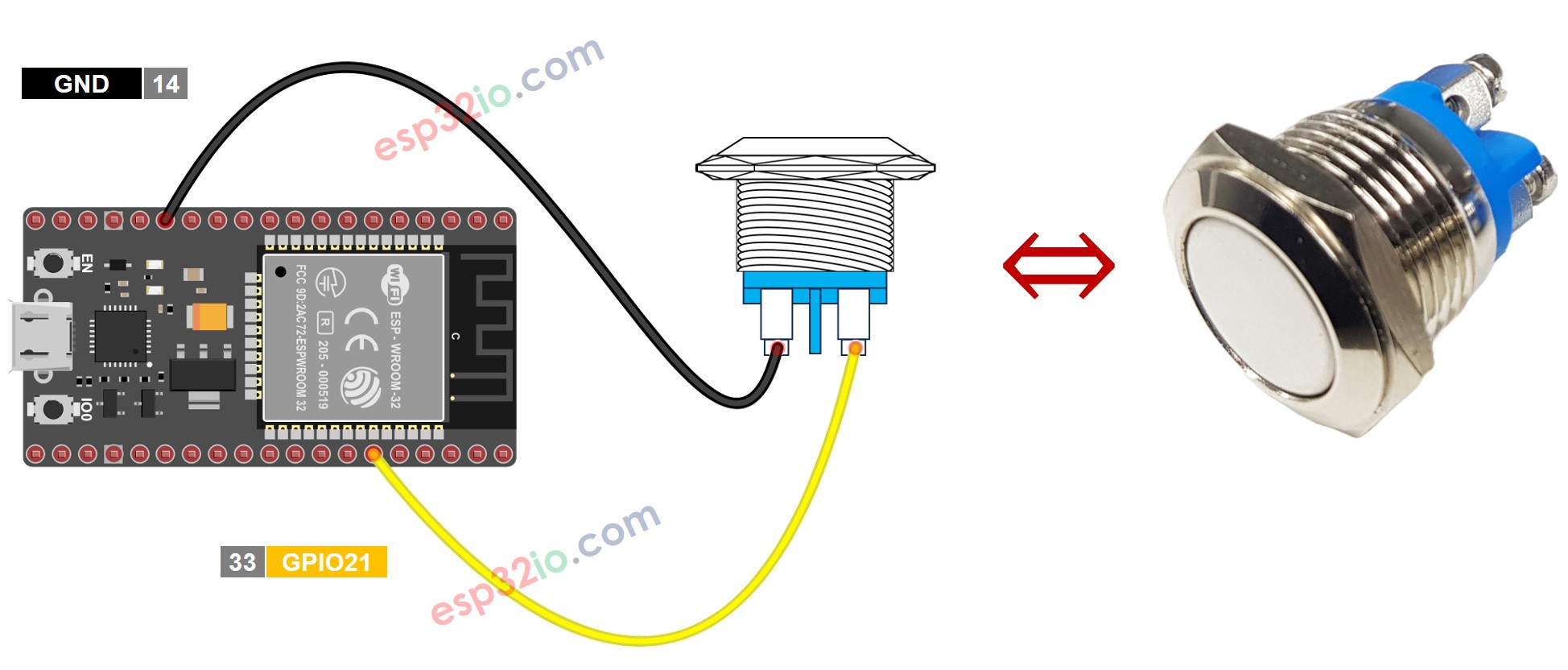
This image is created using Fritzing. Click to enlarge image
This tutorial will use the internal pull-up resistor. The state of the button is HIGH when normal and LOW when pressed.
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
How To Detect Short Press
- Measure the time between the pressed and released events.
- If the duration is shorter than a pre-defined time, the short press event is detected.
Let's see step by step:
- Define how long the maximum of short press lasts
- Detect the button is pressed and save the pressed time
- Detect the button is released and save the released time
- Calculate press duration and
- Determine the short press by comparing the press duration with the defined short press time.
ESP32 Code for detecting the short press
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Upload the above code to ESP32 via Arduino IDE
- Press the button shortly several times.
- See the result on Serial Monitor. It looks like the below:
※ NOTE THAT:
The Serial Monitor may print several short presses for a single press. This is a normal behavior of the button. This behavior is called the “chattering phenomenon”. We will learn how to eliminate this issue later in this tutorial.
How To Detect Long Press
There are two use cases for detecting the long press.
- The long-press event is detected right after the button is released
- The long-press event is detected while the button is being pressed.
In the first case:
- Measure the time duration between the pressed event and released event.
- If the duration is longer than a pre-defined time, the long-press event is detected.
In the second case: during the time the button is being pressed, do the below process repteatedly:
- Measure the pressing time.
- If the duration is longer than the pre-defined time, the long-press event is detected.
- Otherwise, repeat the process until the button is released
ESP32 Code for detecting long press when released
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Upload the above code to ESP32 via Arduino IDE
- Press and release the button after one second.
- See the result on Serial Monitor. It looks like the below:
ESP32 Code for detecting long press during pressing
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Upload the above code to ESP32 via Arduino IDE
- Press and release the button after several seconds.
- See the result on Serial Monitor. It looks like the below:
How To Detect Both Long Press and Short Press
Short Press and Long Press after released
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Upload the above code to ESP32 via Arduino IDE
- Long and short press the button.
- See the result on Serial Monitor. It looks like the below:
※ NOTE THAT:
The Serial Monitor may show several short press detection when long press. This is the normal behavior of the button. This behavior is called the “chattering phenomenon”. The issue will be solved in the last part of this tutorial.
Short Press and Long Press During pressing
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Upload the above code to ESP32 via Arduino IDE
- Long and short press the button.
- See the result on Serial Monitor. It looks like the below:
Long Press and Short Press with Debouncing
It is very important to debounce the button in many applications.
Debouncing is a little complicated, especially when using multiple buttons. To make it simple for newbies, we created a library, called ezButton.
We will use this library in below codes
Short Press and Long Press with debouncing after released
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Install ezButton library. See How To
- Upload the above code to ESP32 via Arduino IDE
- Long and short press the button.
- See the result on Serial Monitor. It looks like the below:
Short Press and Long Press with debouncing During Pressing
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Install ezButton library. See How To
- Upload the above code to ESP32 via Arduino IDE
- Long and short press the button.
- See the result on Serial Monitor. It looks like the below:
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.
Why Needs Long Press and Short Press
- To save the number of buttons and digital input pin. A single button can keep two or more functionalities. For example, short press for turning on the light, long press for turn on the fan.
- Use the long press instead the short press to avoid pressing by accident. For example, some kinds of devices use the button for factory reset. If the button is pressed by accident, it is dangerous.