ESP32 - OLED
This tutorial instructs you how to use ESP32 to display data on OLED. In detail, we will learn how to print characters and number, draw line and shape, and print image on OLED display.
This tutorial shows how to program the ESP32 using the Arduino language (C/C++) via the Arduino IDE. If you’d like to learn how to program the ESP32 with MicroPython, visit this ESP32 MicroPython - OLED tutorial.
Hardware Used In This Tutorial
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to OLED Display
There are several different kinds of OLED display that can work with ESP32. They are different from each other in communication interface ( I2C, SPI), colors (white, blue, dual color...), and sizes (128x64, 128×32...)
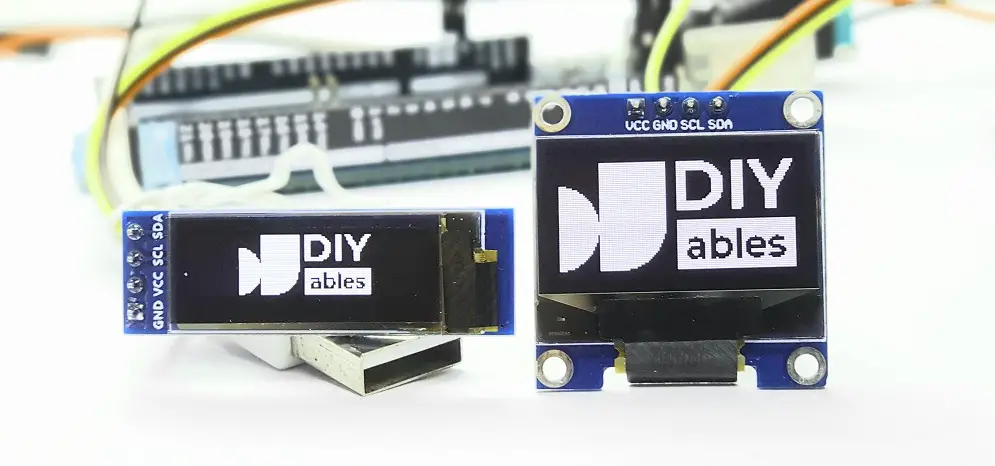
This tutorial uses an SSD1306 128x64 I2C OLED Display
I2C OLED Display Pinout
I2C OLED Display includes 4 pins:
- GND pin: should be connected to the ground of ESP32
- VCC pin: should be connected to the 5 volts pin of ESP32.
- SCL pin: is a I2C clock pin.
- SDA pin: is a I2C data pin.
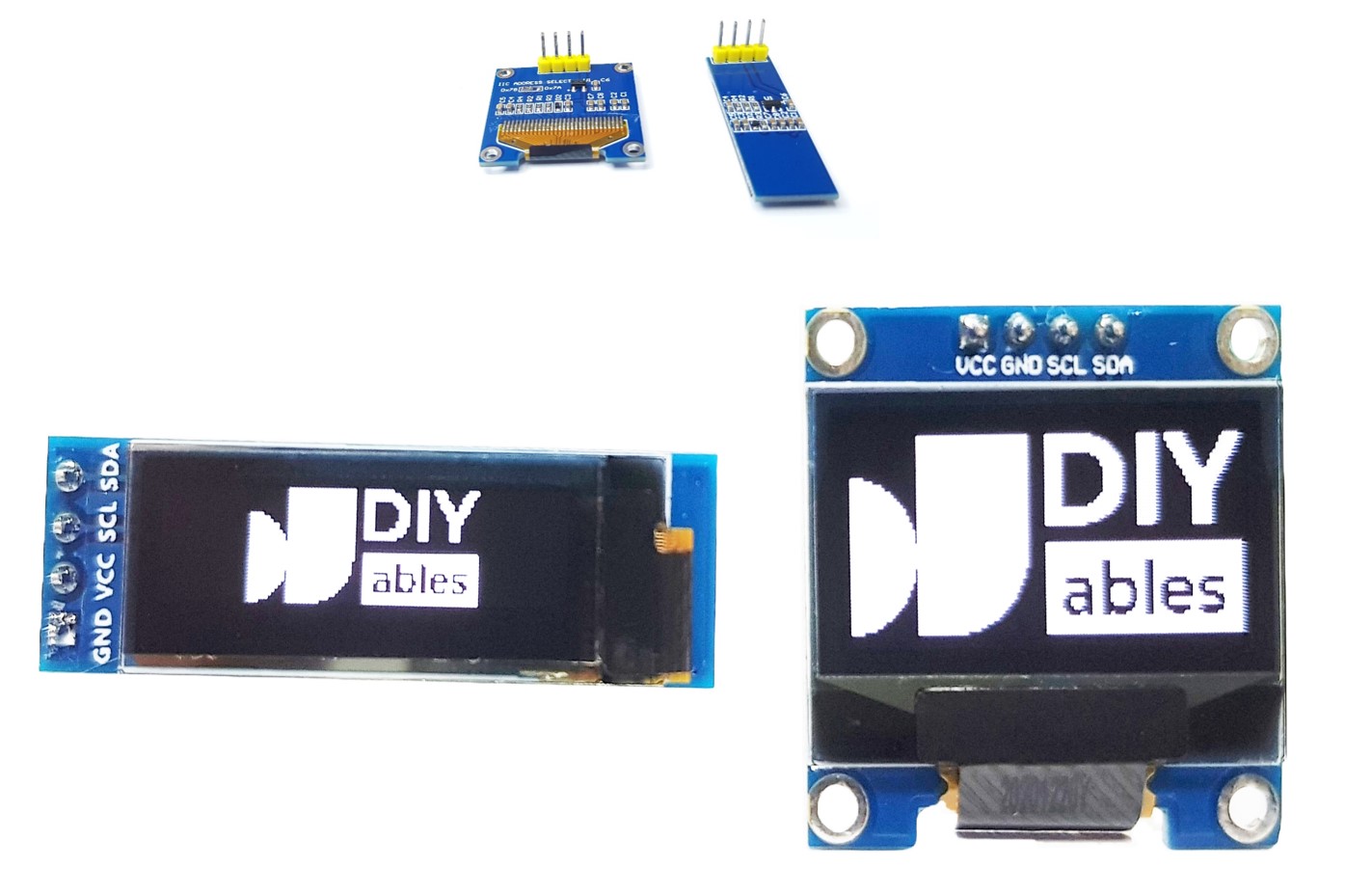
Wiring Diagram
- How to connect ESP32 to OLED 128x64 display using breadboard
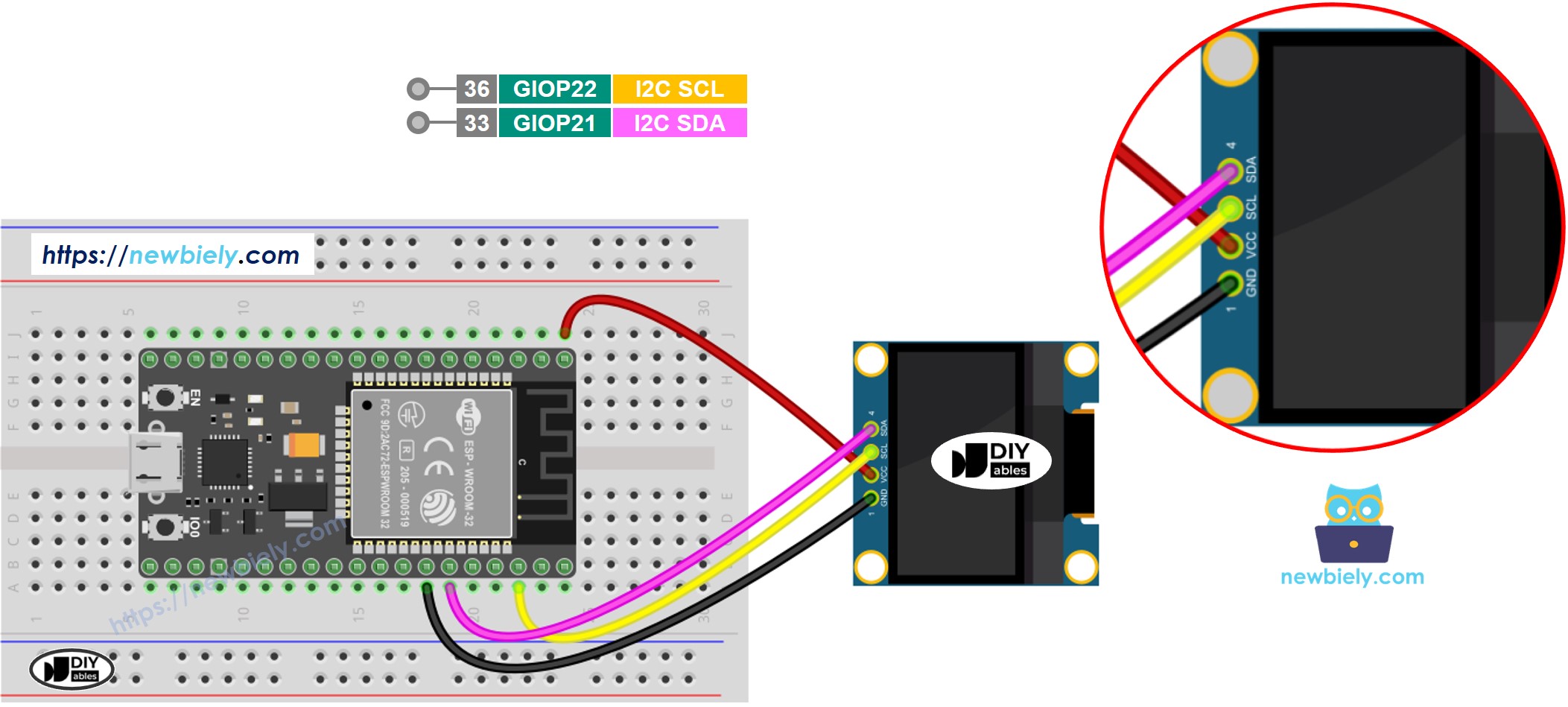
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 to OLED 128x64 display using screw terminal block breakout board
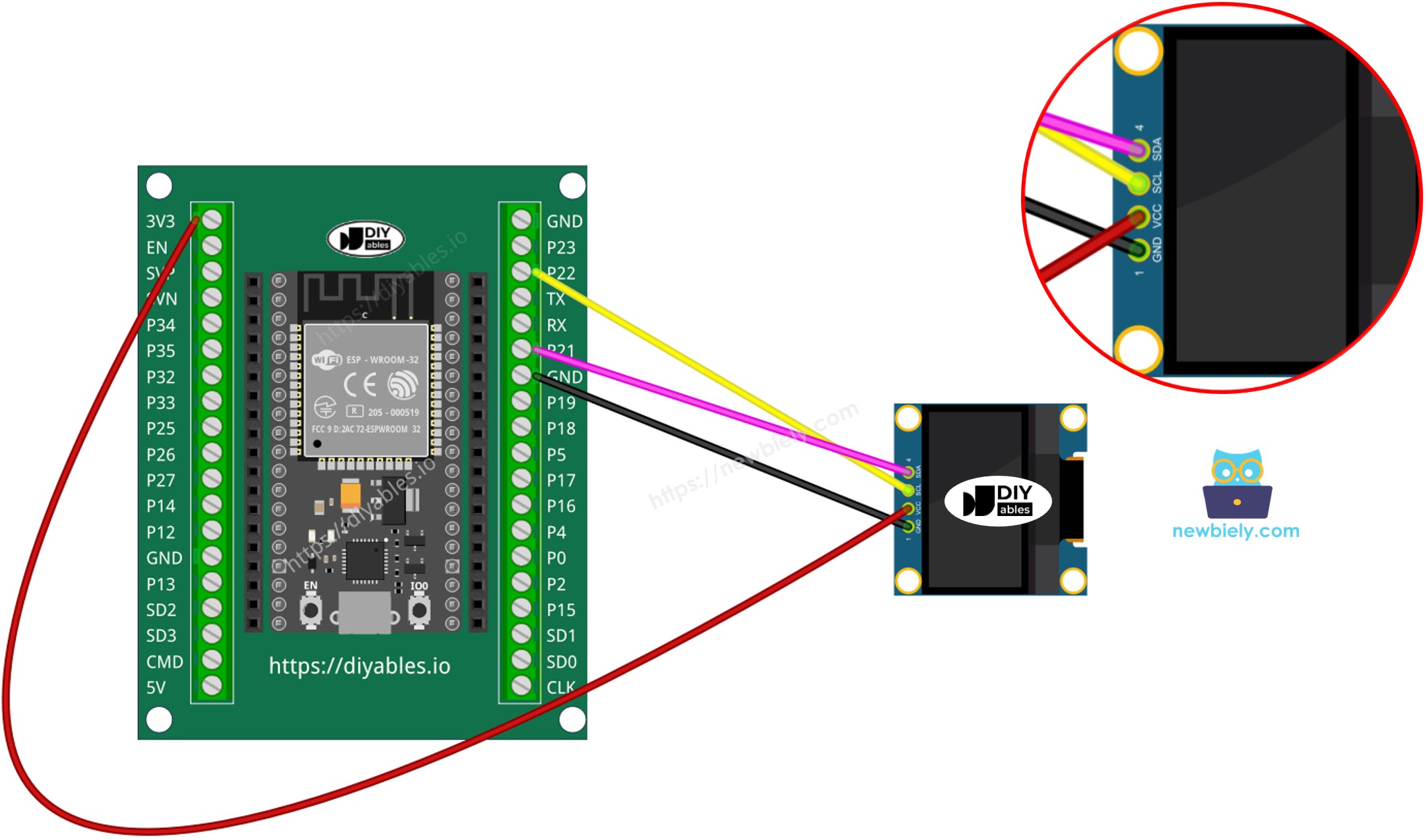
※ NOTE THAT:
- The order of the OLED module's pins can vary between manufacturers. ALWAYS use the labels printed on the OLED module. Look closely!
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
How To Use OLED with ESP32
Install SSD1306 OLED library
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Type SSD1306 on the search box, then look for the SSD1306 library by Adafruit
- Install the library by clicking on Install button.
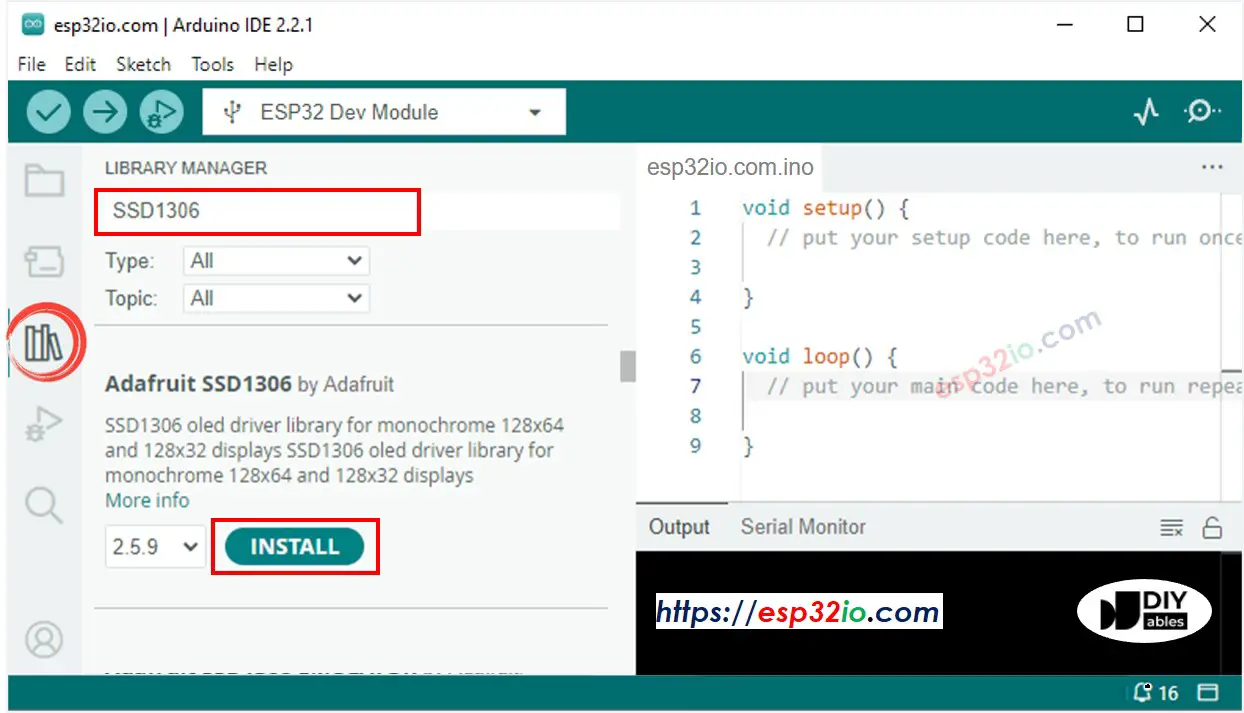
- A window appears to ask you to install dependencies for the library
- Install all dependencies for the library by clicking on Install All button.
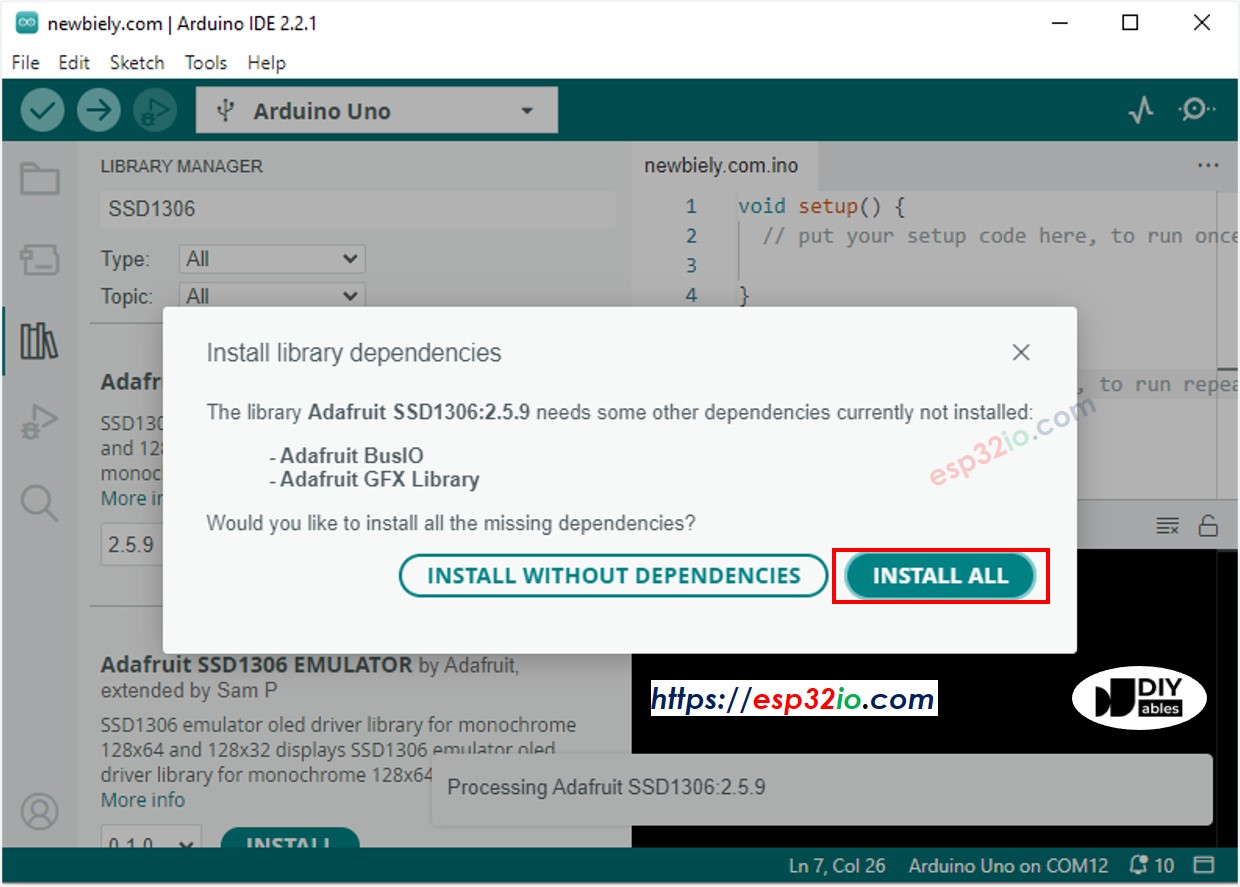
How to program for OLED
- Include library
- Define the screen size (123x64 or 128 x32)
- Declare an SSD1306 OLED object
- In setup() function, initialize OLED display
- And then you can display text, images, draw line ...
ESP32 Code - Display Text on OLED
The below ESP32 code display a text on OLED
We can do more by using the following functions:
- oled.clearDisplay(): this function is used to clear the display
- oled.setTextSize(n): this function is used to set the font size, supports sizes from 1 to 8
- oled.setTextColor(WHITE): this function is used to set the text color
- oled.setCursor(x,y): this function is used to set the coordinates to start displaying text
- oled.drawPixel(x,y, color): this function is used to plot a pixel in the x,y coordinates
- oled.setTextColor(BLACK, WHITE): this function is used to set the text color and background color, respectively
- oled.println("message"): this function is used to print a string
- oled.println(number): this function is used to print a number
- oled.println(number, HEX): this function is used to print a number in hex format
- oled.display(): this function is used to apply the changes
- oled.startscrollright(start, stop): this function is used to scroll text from the left to the right
- oled.startscrollleft(start, stop): this function is used to scroll text from the right to the left
- oled.startscrolldiagright(start, stop): this function is used to scroll text from left bottom corner to right upper corner
- oled.startscrolldiagleft(start, stop): this function is used to scroll text from right bottom corner to left upper corner
- oled.stopscroll(): this function is used to stop scrolling
ESP32 Code - Drawing Shapes on OLED
The below code show how to draw several shapes on OLED
After running the code, you'll see a rectangle, circle, and triangle on the OLED screen.
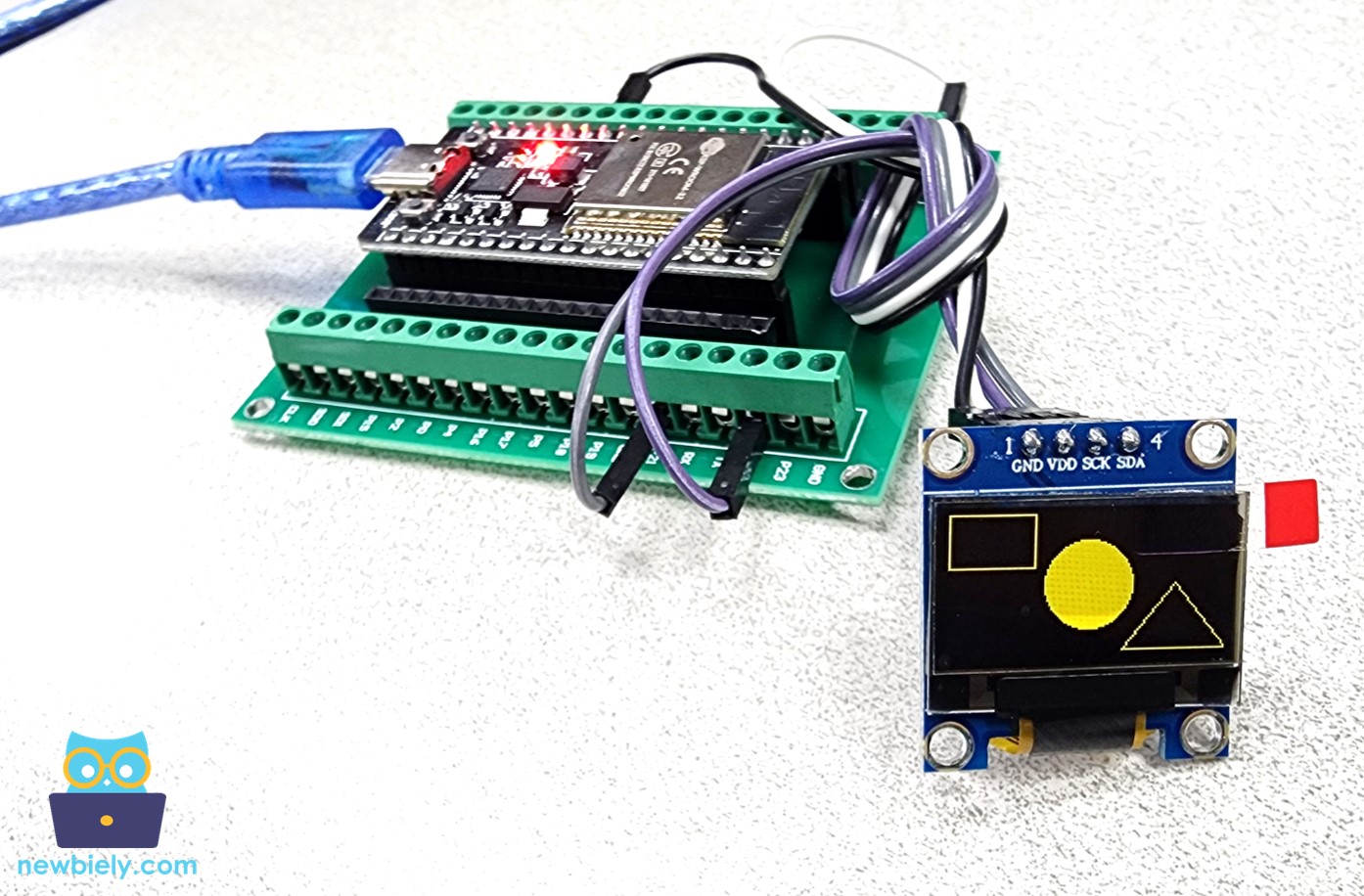
ESP32 Code – Display Image on OLED
To display an image on OLED, it needs to convert the image to the bitmap array. There is a online tool to convert the image to bitmap array. The below image show how to do it. I converted the ESP32 icon to bitmap array.
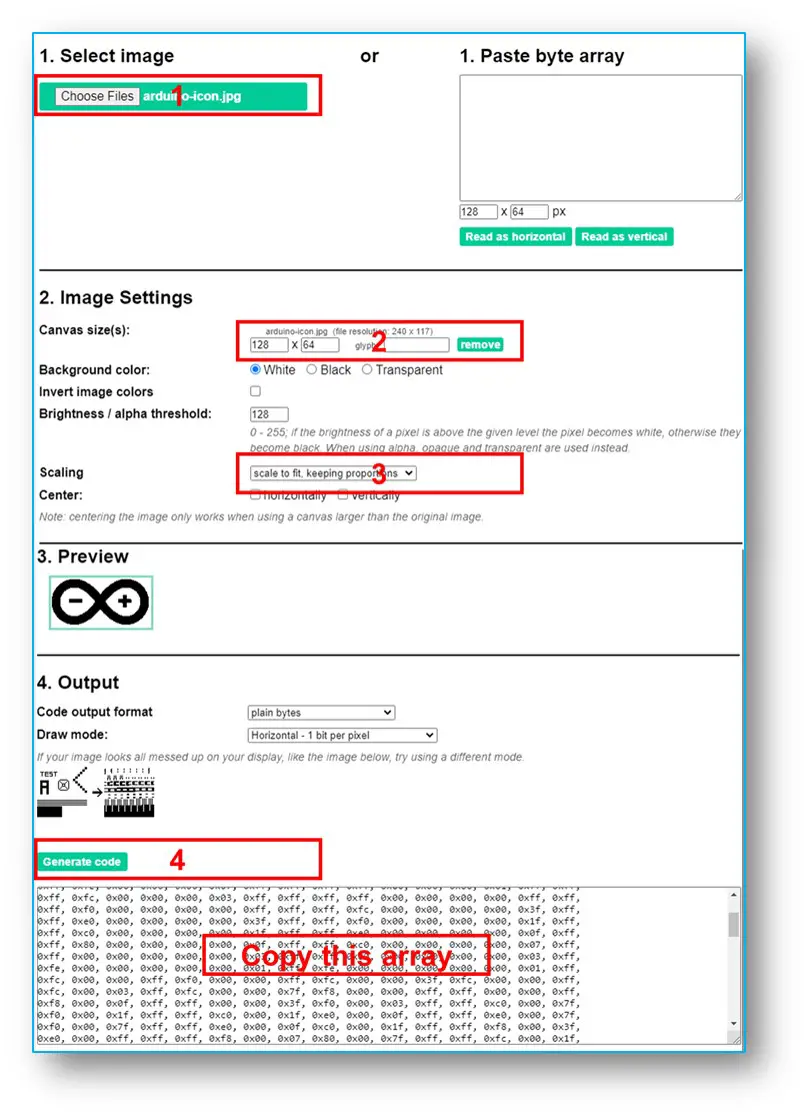
After converting, copy the array code and update it in the bitmap_image array in the following code:
By running the above code, you'll see the image displayed on the OLED as shown below.
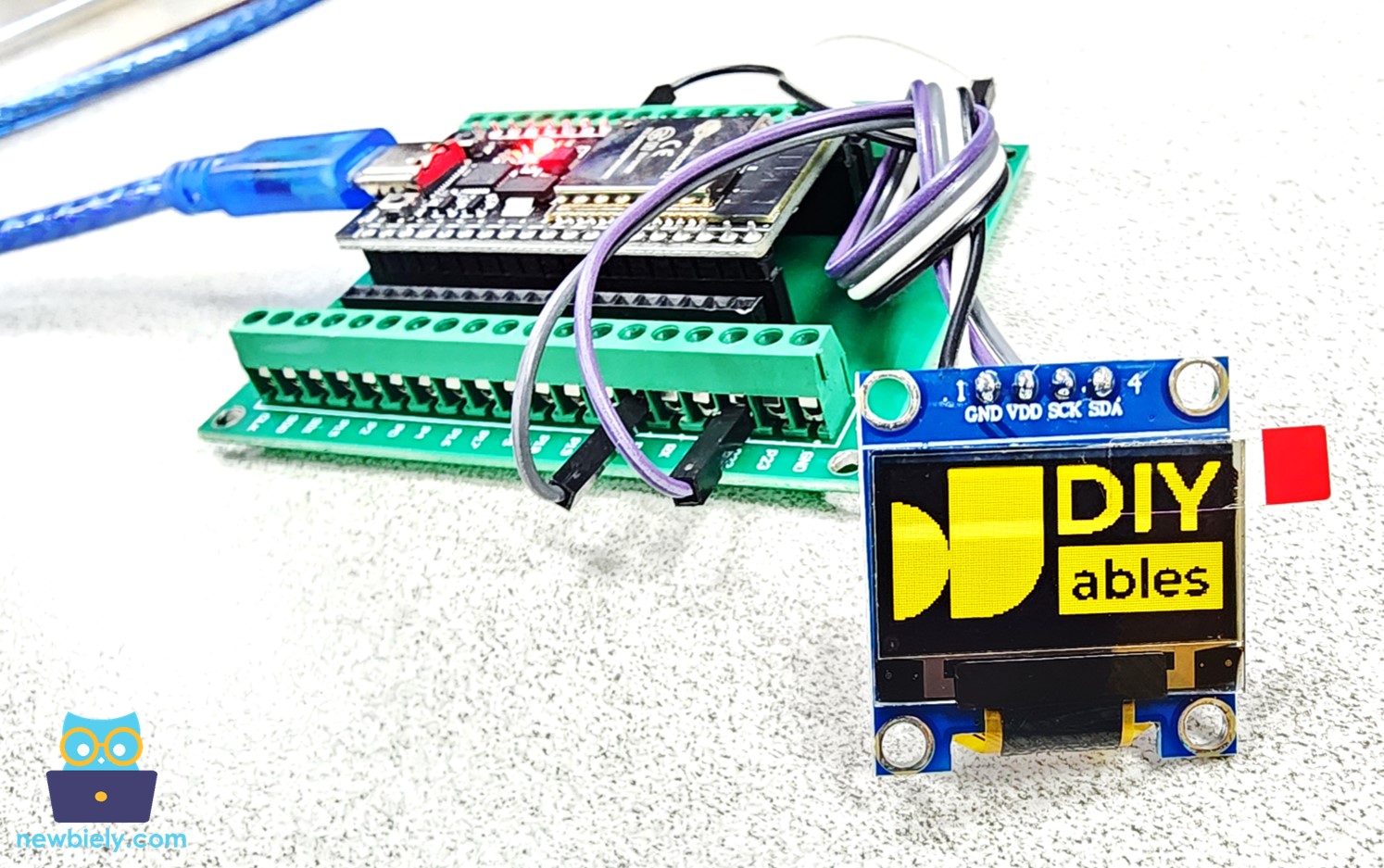