ESP32 - Switch
An ON/OFF or toggle switch has two states: ON (closed) and OFF (open). Each time the switch is pressed, its state is toggled between ON and OFF and it remains in that state even when released. This tutorial will guide you on how to use an ON/OFF switch with an ESP32 microcontroller.
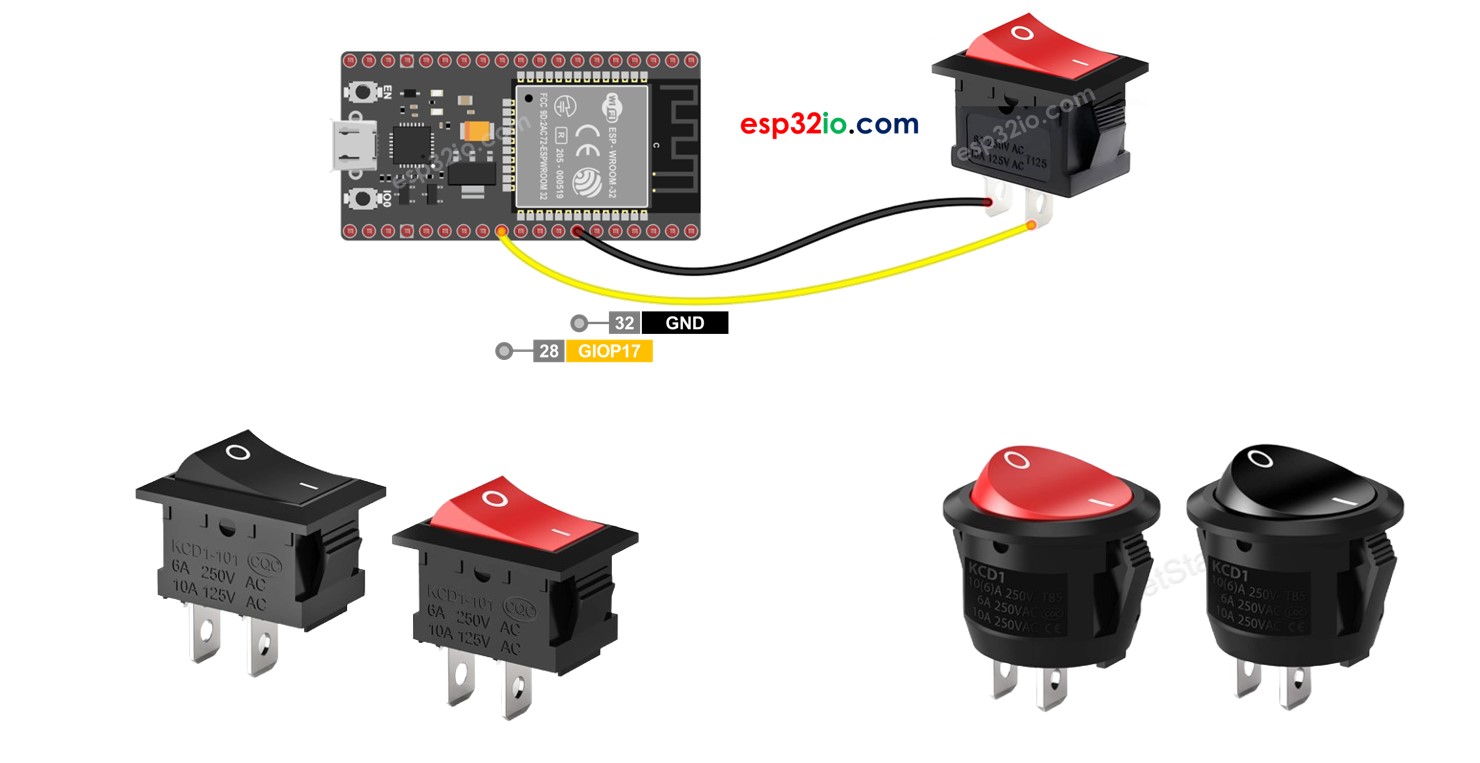
It is important to note that the ON/OFF switch is distinct from the following and should not be confused with them:
This tutorial shows how to program the ESP32 using the Arduino language (C/C++) via the Arduino IDE. If you’d like to learn how to program the ESP32 with MicroPython, visit this ESP32 MicroPython - Switch tutorial.
Hardware Used In This Tutorial
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to ON/OFF Switch
An ON/OFF Switch is a switch that, when pressed, changes its state between ON and OFF, and then maintains that state even when released. To changes the state again, the switch must be pressed again.
Pinout
There are generally two types of ON/OFF Switches, the two-pin switch and the three-pin switch. This tutorial will focus on using the two-pin switch, where the distinction between the two pins is not necessary.
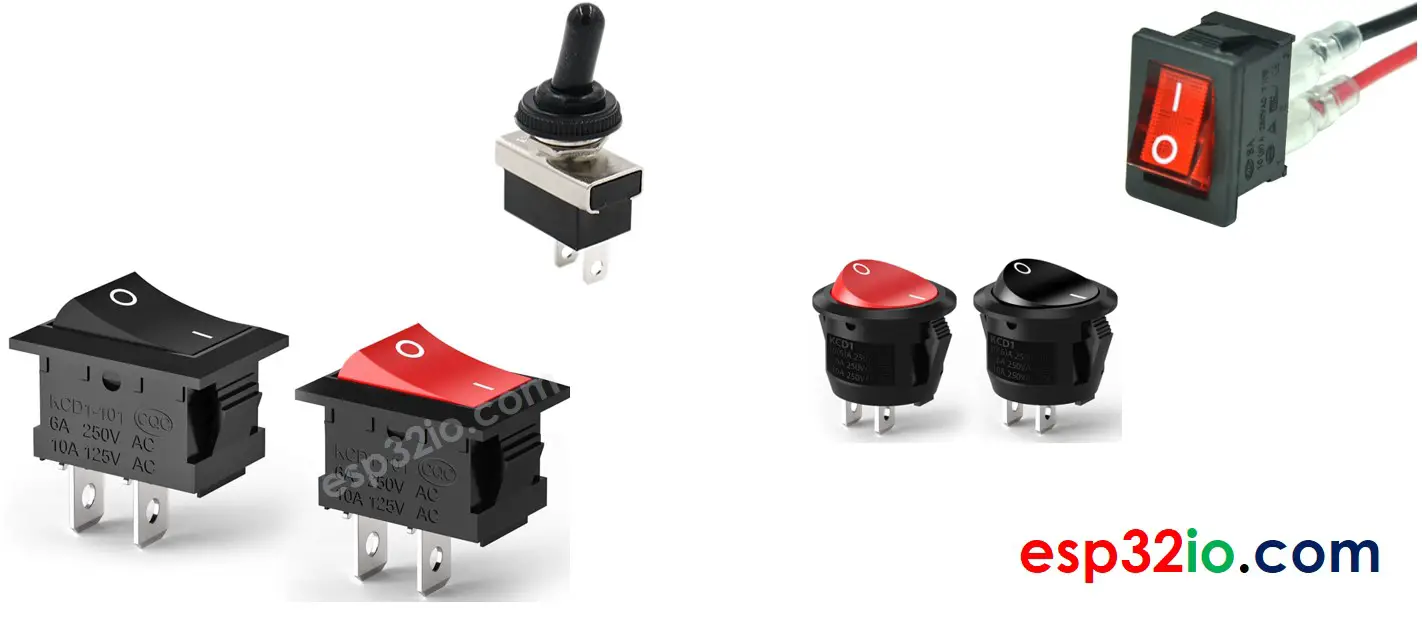
How It Works
There are two methods for using an ON/OFF switch. The table below illustrates the wiring and reading state on an ESP32 for each of these two methods:
pin 1 | pin 2 | ESP32 Input Pin's State | |
---|---|---|---|
1 | GND | ESP32 Input Pin (with pull-up) | HIGH ⇒ OFF, LOW ⇒ ON |
2 | VCC | ESP32 Input Pin (with pull-down) | HIGH ⇒ ON, LOW ⇒ OFF |
Out of the two methods, the tutorial will focus on one, specifically the first method will be used as an example.
Wiring Diagram
- How to connect ESP32 and switch using breadboard
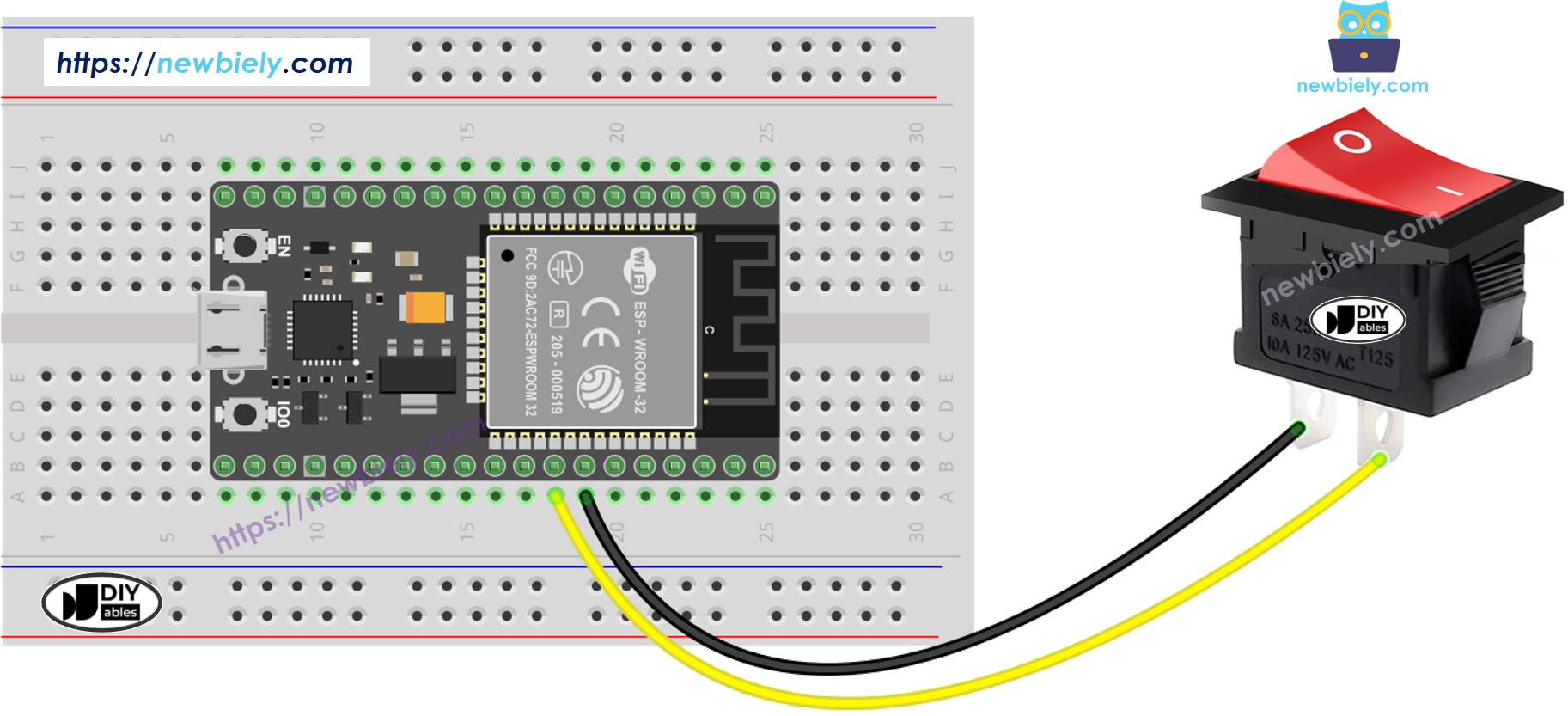
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and switch using screw terminal block breakout board
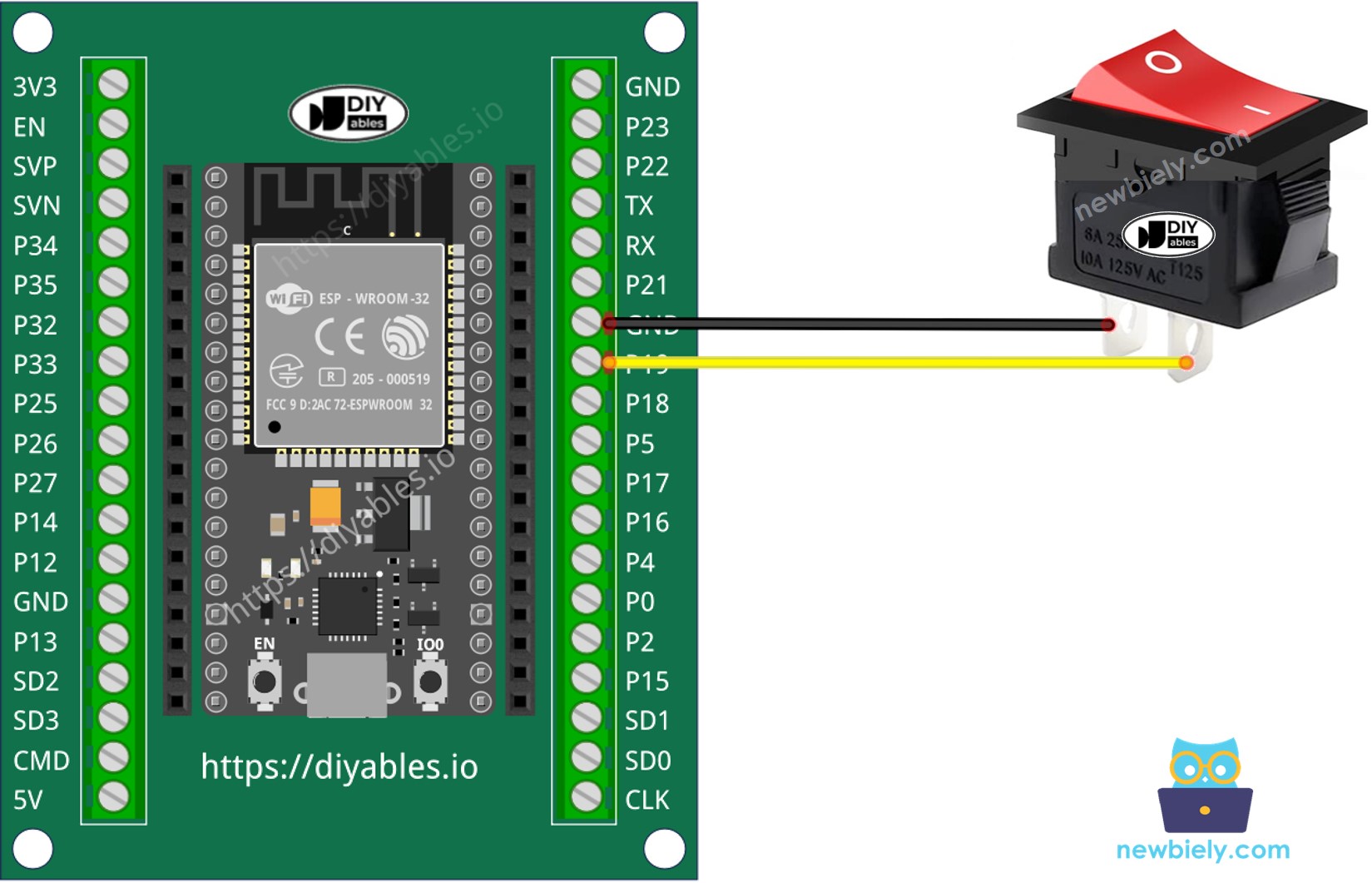
To make the wiring connection stable and firm, we recommend using to solder wires and ON/OFF switch's pin together, and then use to make it safe.
For a stable and secure connection, it is recommended to use a soldering iron to solder the wires and ON/OFF Switch pins together, and then use heat shrink tubes for added safety.
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
ESP32 Code - ON/OFF Switch
Just like a button, a ON/OFF switch also needs to be debounced (See more at ). Debouncing make the code complicated. Fortunately, the supports the debouncing functionm, The library also uses internal pull-up register. These make easy to us to program.
Similar to a button, an ON/OFF switch also requires debouncing (more information can be found at Why needs debounce for the button, ON/OFF switch?). Debouncing can make the code more complex. Fortunately, the ezButton library offers debouncing functionality and utilizes internal pull-up registers, making programming easier.
※ NOTE THAT:
Two common use cases for an ON/OFF switch are:
- The first use case: If the switch is in the ON state, perform a certain action. If the input state is OFF, perform the opposite action.
- The second use case: If the switch's state changes from ON to OFF (or OFF to ON), perform a specific action.
Quick Instructions
- Follow the wiring diagram provided above to connect the ON/OFF switch to ESP32.
- Connect your ESP32 to your PC using a USB cable.
- Open the Arduino IDE software.
- Install the ezButton library. Refer to the instructions
- Select the appropriate board and port in the Arduino IDE.
- Compile and upload the code to the ESP32 board by clicking the Upload button in the Arduino IDE.
- Test the ON/OFF switch by turning it ON.
- Observe the output on the Serial Monitor in the Arduino IDE.
- Turn the switch OFF.
- Observe the output on the Serial Monitor in the Arduino IDE.
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.