ESP32 - Button Count - LCD
In this tutorial, we will explore the capabilities of ESP32 to achieve the following objectives:
- Counting the number of times a button is pressed.
- Displaying the count number on an LCD I2C display.
- Implementing automatic vertical and horizontal center alignment for the count number on the LCD I2C display.
Furthermore, this tutorial addresses the debouncing of the button without relying on the delay() function. For a comprehensive understanding of why debouncing is crucial, you can refer to the explanation provided in Why do we need debouncing?.
This tutorial will guide you through the process of seamlessly integrating button press counting, LCD I2C display functionality, and debouncing techniques with your ESP32 project.
Hardware Used In This Tutorial
1 | × | ESP-WROOM-32 Dev Module | |
1 | × | USB Cable Type-C | |
1 | × | Push Button | |
1 | × | (Optional) Panel-mount Push Button | |
1 | × | LCD I2C | |
1 | × | Breadboard | |
1 | × | Jumper Wires | |
1 | × | (Recommended) ESP32 Screw Terminal Adapter |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Wiring Diagram
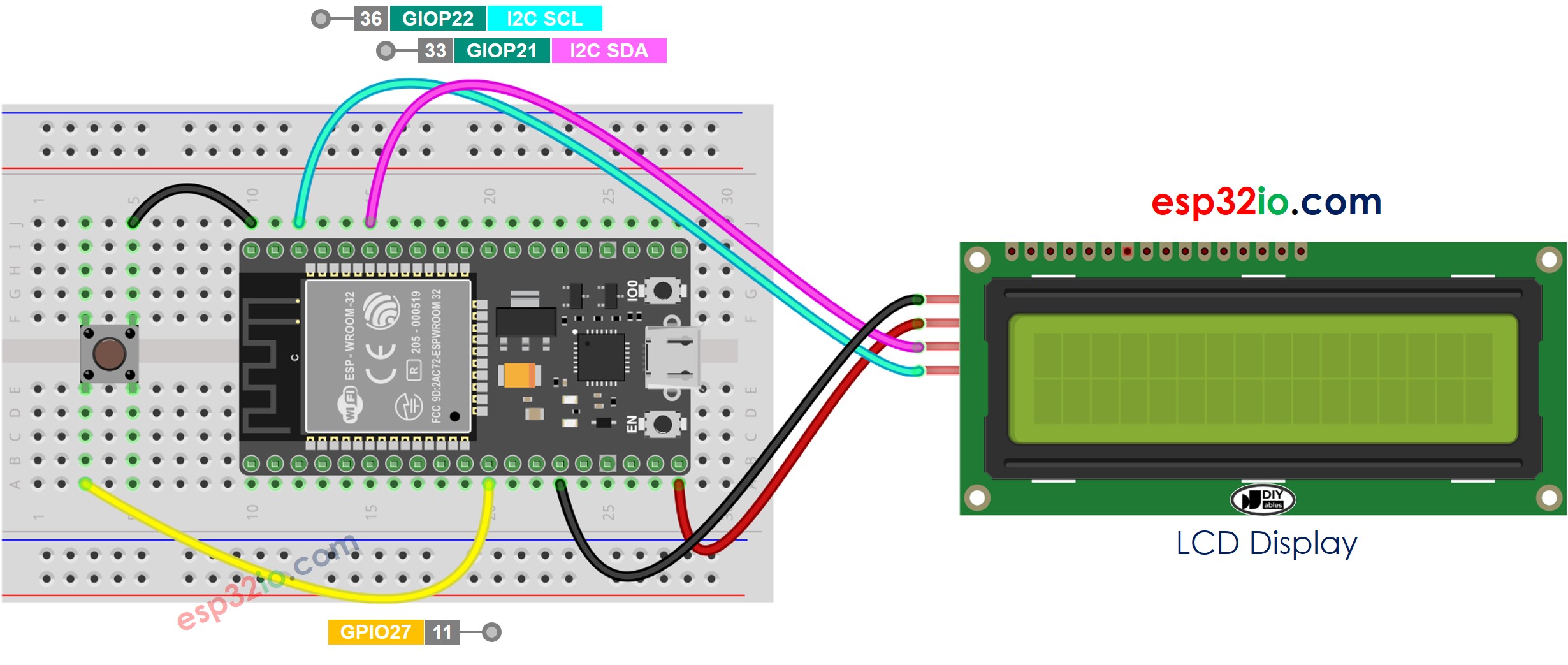
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: How to Power ESP32.
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.