ESP32 - Keypad - Solenoid Lock
In this tutorial, we are going to learn how to use a keypad, solenoid lock, and ESP32 together. In detail, If a user inputs the password on the keypad correctly, ESP32 turns the solenoid lock on.
The tutorial also provides the code that turns on a solenoid lock in a period of time and then turns off without using delay() function. The ESP32 code also supports multiple passwords.
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to Keypad and Solenoid Lock
Unfamiliar with keypad and Solenoid Lock, including their pinouts, functionality, and programming? Explore comprehensive tutorials on these topics below:
- ESP32 - Keypad tutorial
- ESP32 - Solenoid Lock tutorial
Wiring Diagram
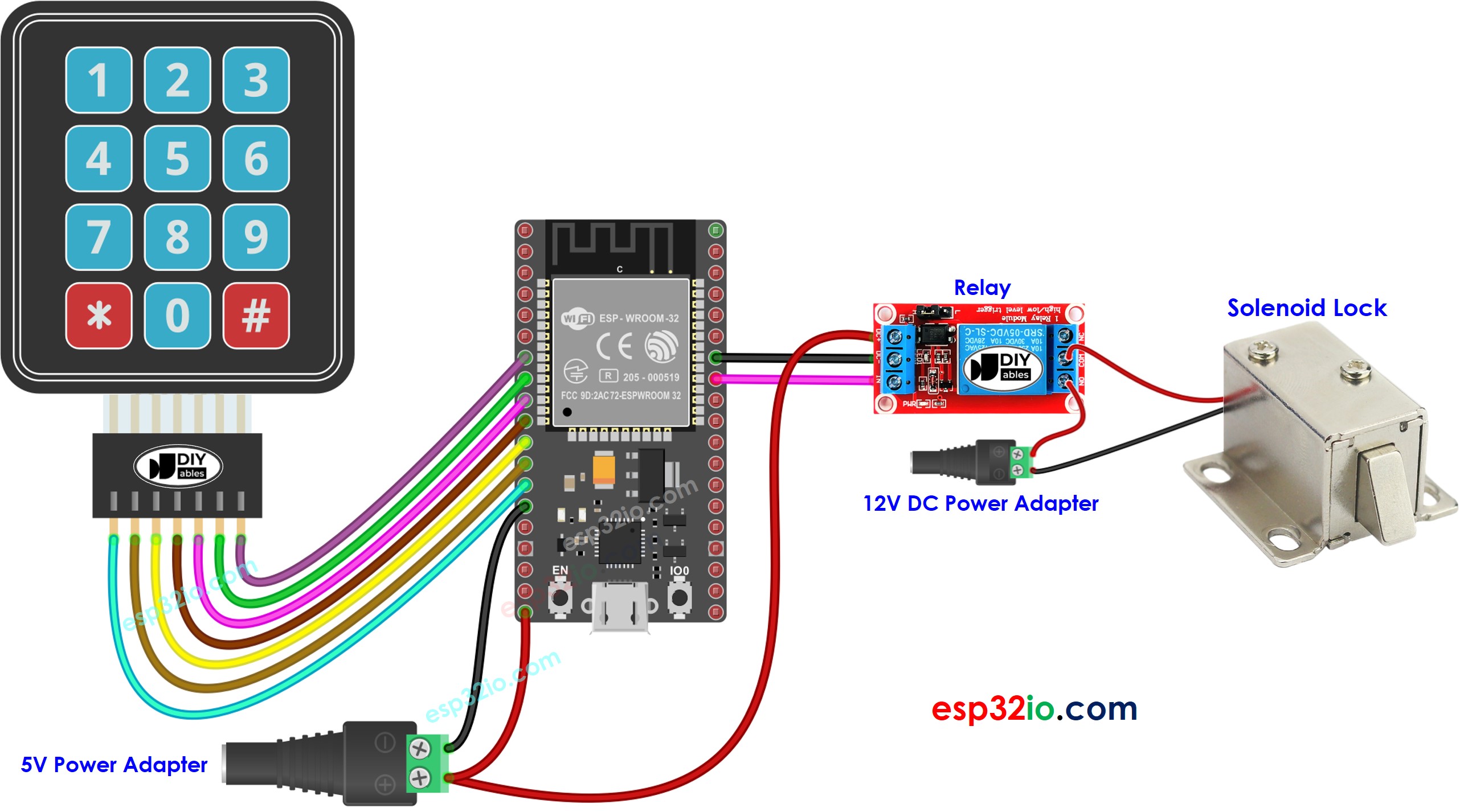
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
ESP32 Code - turn solenoid lock on if the password is correct
The below codes turn a solenoid lock on if the password is correct
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Connect ESP32 to PC via USB cable
- Open Arduino IDE, select the right board and port
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search “keypad”, then find the keypad library by Mark Stanley, Alexander Brevig
- Click Install button to install keypad library.
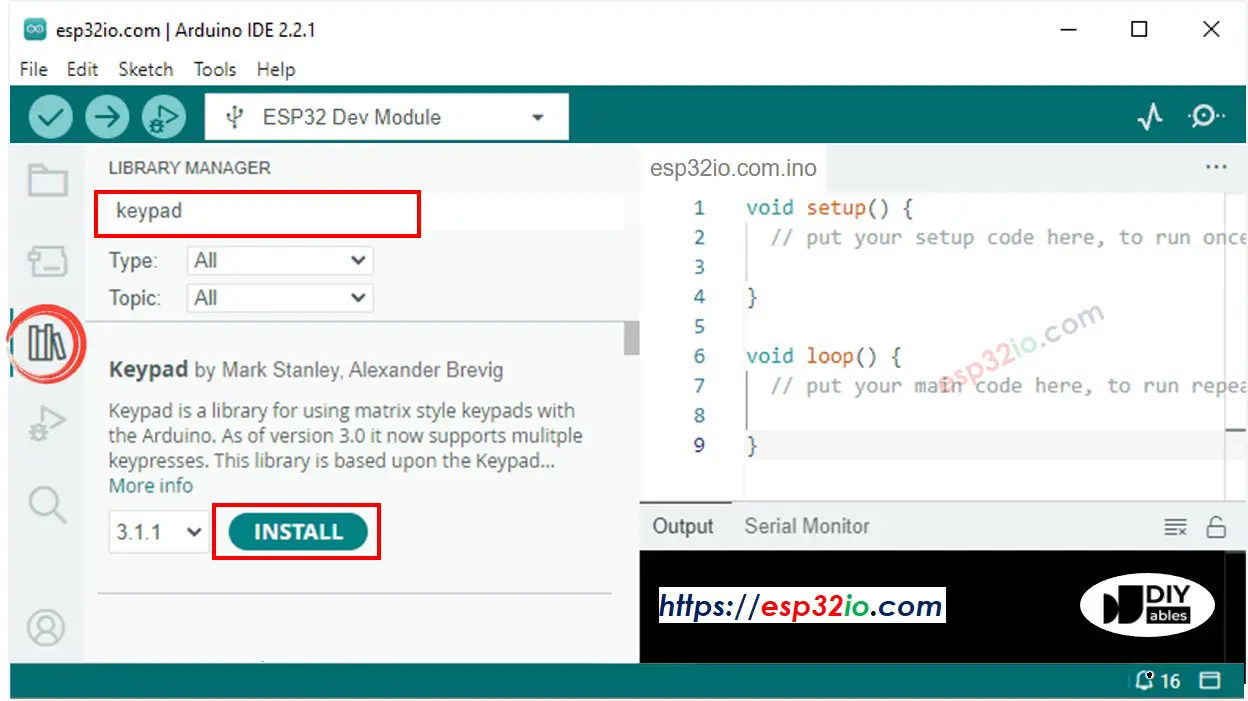
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
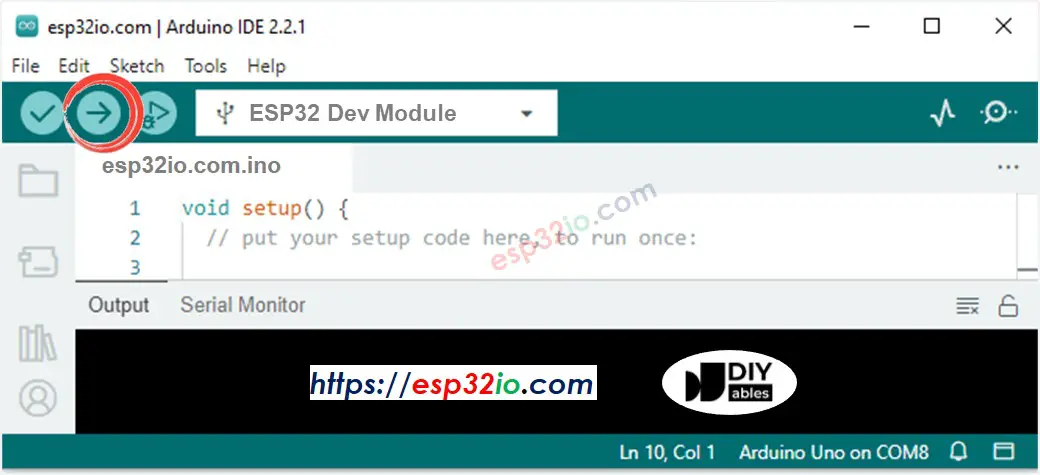
- Press 7124 keys and press #
- Press 1234 keys and press #
- See the result on Serial Monitor and the state of solenoid lock
Code Explanation
Authorized passwords are pre-defined in the ESP32 code.
A string is used to store the password inputted by users, called input string. In keypad, two keys (* and #) are used for special purposes: clear password and terminate password. When a key on keypad is pressed:
- If the pressed key is not two special keys, it is appended to the input string
- If the pressed key is *, input string is clear. You can use it to start or re-start inputing the password
- If the pressed key is #:
- The input string is compared with the pre-defined passwords. If it matched with one of the pre-defined passwords, the solenoid lock is turned on.
- No matter the password is correct or not, the input string is clear for the next input
ESP32 Code - turn a solenoid lock on in a period of time if the password is correct
The below code turns the solenoid lock on for 5 seconds if the password is correct. After 5 seconds, the solenoid lock is turned off.
Please note that, the above code use ezOutput library, which makes it easy to manage time in non-blocking maner. You can refer to ezOutput Library Installization Guide
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.