ESP32 - TMP36 Temperature Sensor
In this guide, we are going to learn how to program ESP32 to read the temperature from the TMP36 sensor.
Hardware Used In This Tutorial
1 | × | ESP-WROOM-32 Dev Module | |
1 | × | USB Cable Type-C | |
1 | × | TMP36 Temperature Sensor | |
1 | × | Breadboard | |
1 | × | Jumper Wires | |
1 | × | (Recommended) Screw Terminal Expansion Board for ESP32 | |
1 | × | (Recommended) Power Splitter For ESP32 |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to TMP36 Temperature Sensor
Pinout
TMP36 temperature sensor has three pins:
- GND pin: needs to be connected to GND (0V)
- VCC pin: needs to be connected to VCC (5V)
- +Vs pin: is the power supply for the sensor which can vary between 2.7V to 5.5V.
- Vout pin: signal pin gives the output voltage that is linearly proportional to the temperature, should be connected to a analog pin on ESP32.
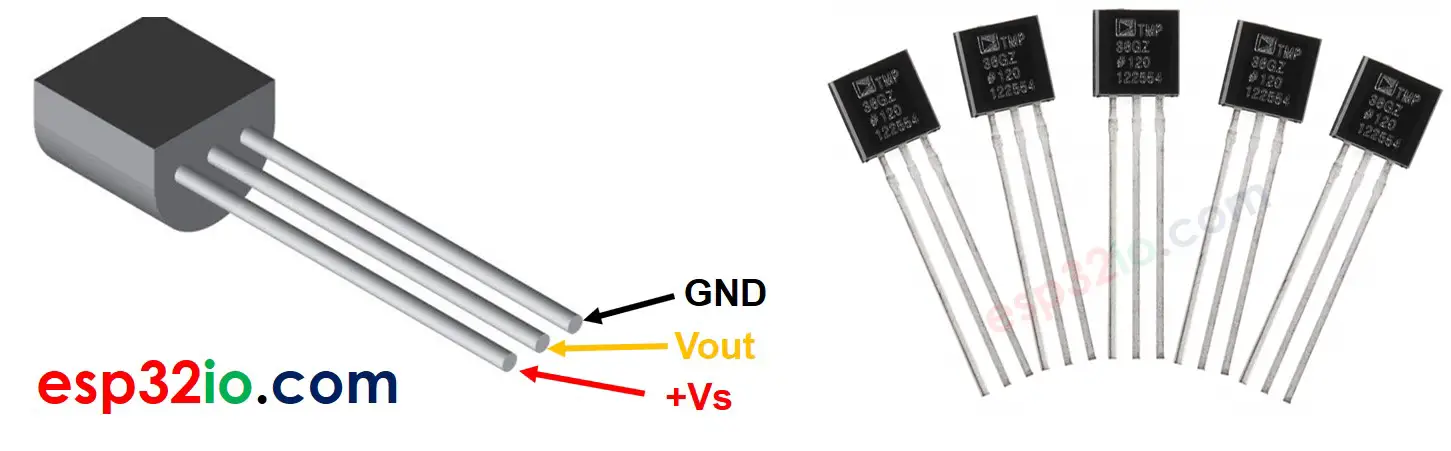
How It Works
The TMP36 outputs the voltage linearly proportional to the Centigrade temperature. The output scale factor of the TMP36 is 10 mV/°C. It means that the temperature is calculated by dividing the voltage (mV) in output pin by 10.
Wiring Diagram
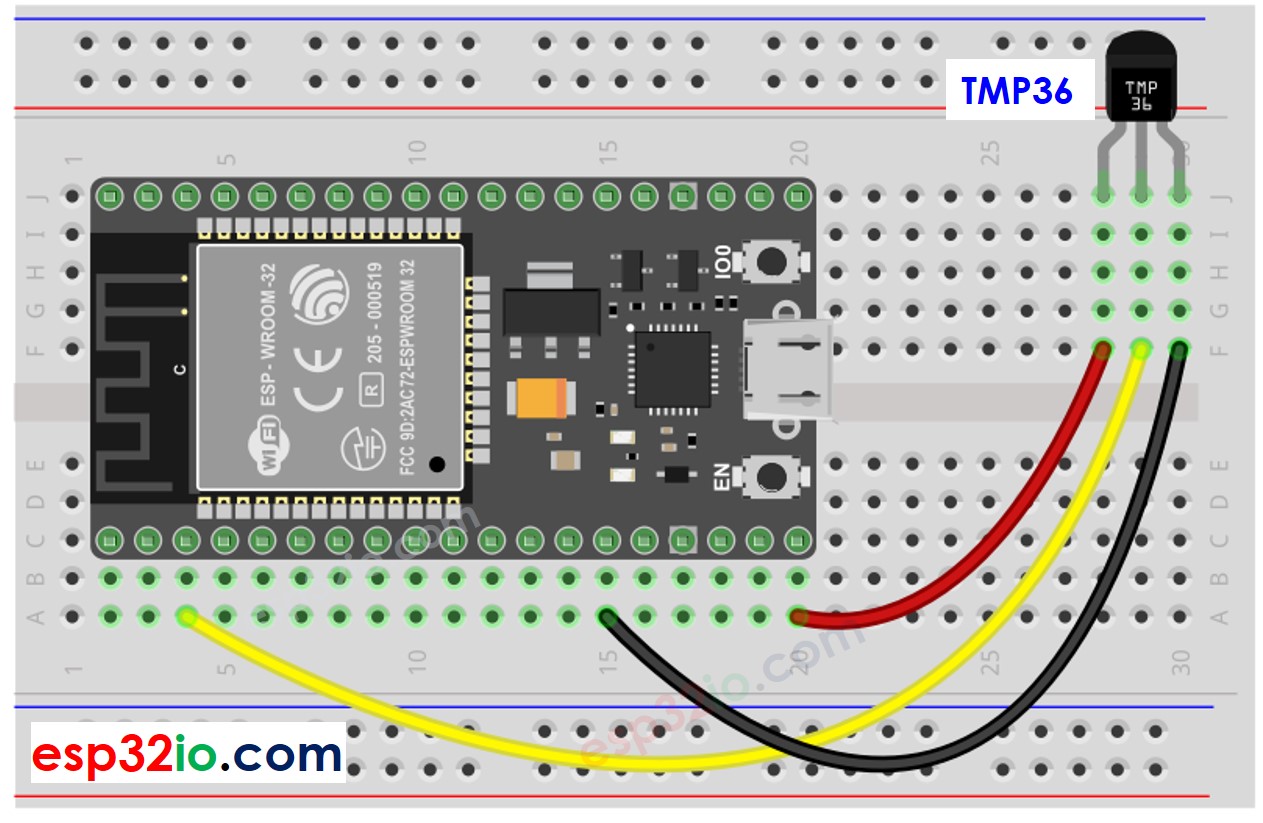
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: How to Power ESP32.
How To Program For TMP36 Temperature Sensor
- Get the ADC value from the temperature sensor by using analogRead() function.
- Convert the ADC value to voltage in voltage
- Convert the voltage to the temperature in Celsius
- (Optional) Convert the Celsius to Fahrenheit
ESP32 Code
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
- Grasp the sensor by your hand
- See the result on Serial Monitor.
※ NOTE THAT:
This tutorial uses the analogRead() function to read values from an ADC (Analog-to-Digital Converter) connected to a TMP36 sensor. The ESP32 ADC is good for projects that do NOT need high accuracy. However, for projects that need precise measurements, please note:
- The ESP32 ADC is not perfectly accurate and might need calibration for correct results. Each ESP32 board can be a bit different, so you need to calibrate the ADC for each individual board.
- Calibration can be difficult, especially for beginners, and might not always give the exact results you want.
For projects that need high precision, consider using an external ADC (e.g ADS1115) with the ESP32 or using an Arduino, which has a more reliable ADC. If you still want to calibrate the ESP32 ADC, refer to ESP32 ADC Calibration Driver
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.