ESP32 - LED - Blink Without Delay
One of the first program that beginners run is to blink an LED. The simplest way to blink an LED is to use the delay() function. This function blocks ESP32 from doing other things. It will be ok if you just want to blink only a single LED. However, If you want to blink more LED or do other works in parallel, you cannot use the delay() function. We need another solution. This tutorial instructs you how to do multiple task without using delay function. More specifically, We will learn how to blink an LED and checks the button's state.
We will run though three below examples and compare the difference between them.
- ESP32 blinks LED by using delay() function
- ESP32 blinks LED by using millis() function
- ESP32 blinks LED by using ezLED library
This method can be applied to let ESP32 do several tasks at the same time. Blinking LED is just an example.
This tutorial shows how to program the ESP32 using the Arduino language (C/C++) via the Arduino IDE. If you’d like to learn how to program the ESP32 with MicroPython, visit this ESP32 MicroPython - LED - Blink Without Delay tutorial.
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Wiring Diagram
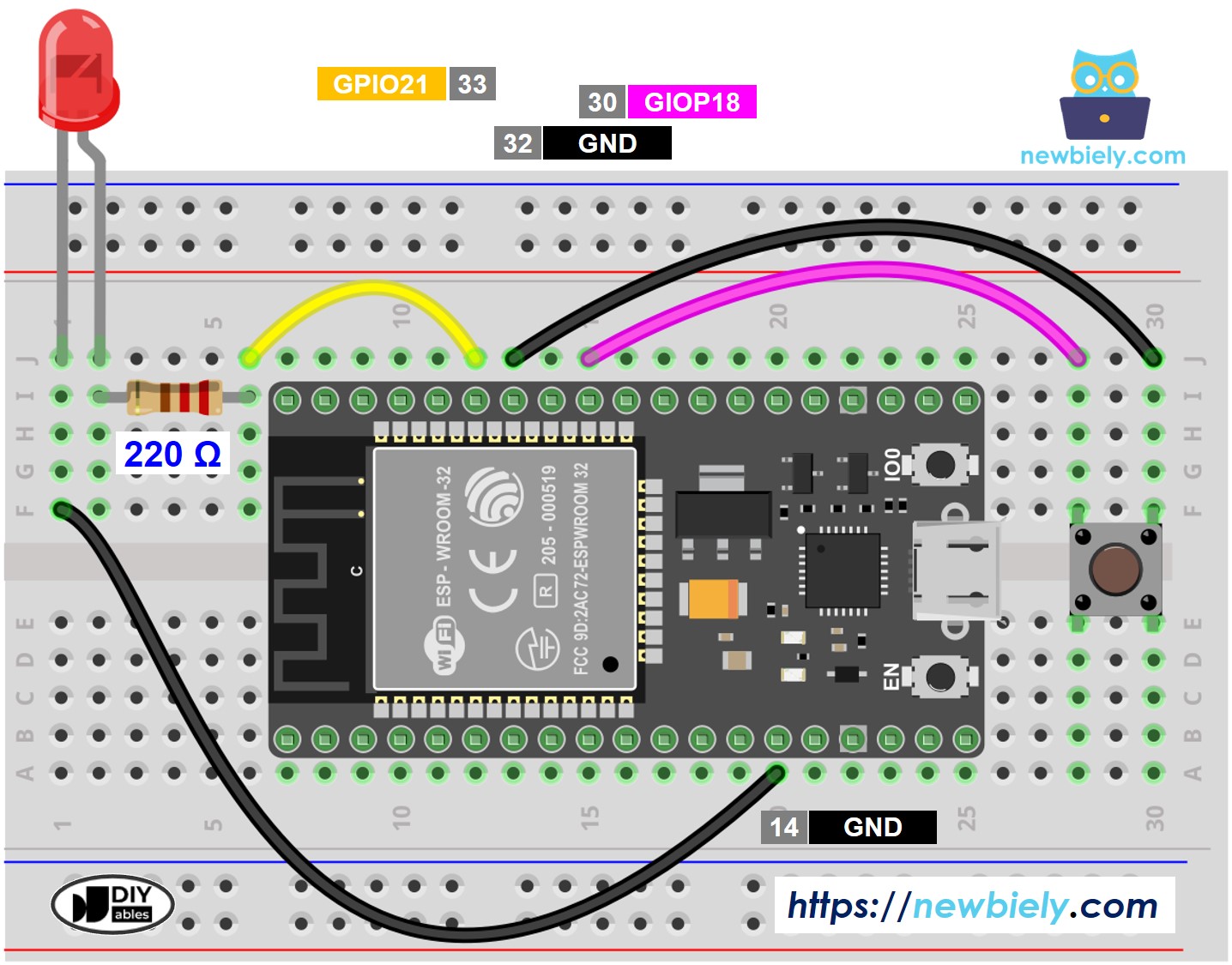
This image is created using Fritzing. Click to enlarge image
Let's compare the ESP32 code that blinks LED with and without using delay() function
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
ESP32 Code - With Delay
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Copy the above code and paste it to Arduino IDE.
- Compile and upload code to ESP32 board by clicking Upload button on Arduino IDE
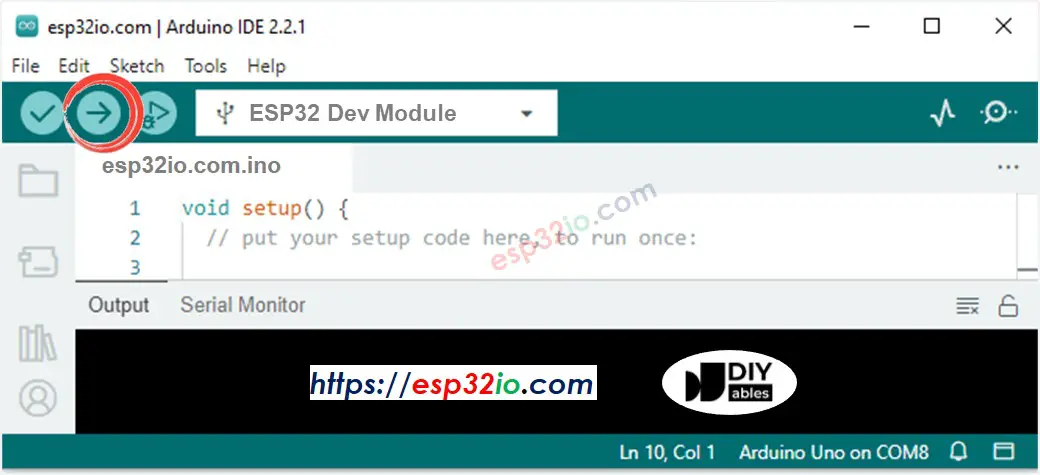
- Open Serial Monitor on Arduino IDE
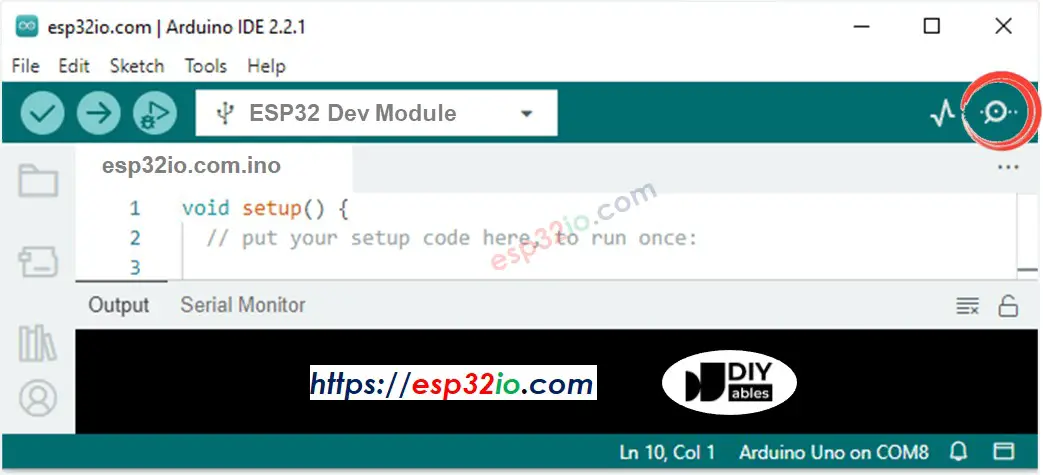
- Press the button 4 times
- See the LED: The LED toggles between ON/OFF periodically every second
- See the output in Serial Monitor
- On Serial Monitor, you will not see enough four times that the state changes to 0 (4 presses). That is because, during delay time, ESP32 CANNOT detect the changing.
ESP32 Code - Without Delay
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Run the above code and press the button 4 times
- See the LED: The LED toggles between ON/OFF periodically every second
- See the output in Serial Monitor
- All pressing events were detected.
Line-by-line Code Explanation
The above ESP32 code contains line-by-line explanation. Please read the comments in the code!
Adding More Tasks
The below code blinks two LEDs with different intervals and checks the state of the button.
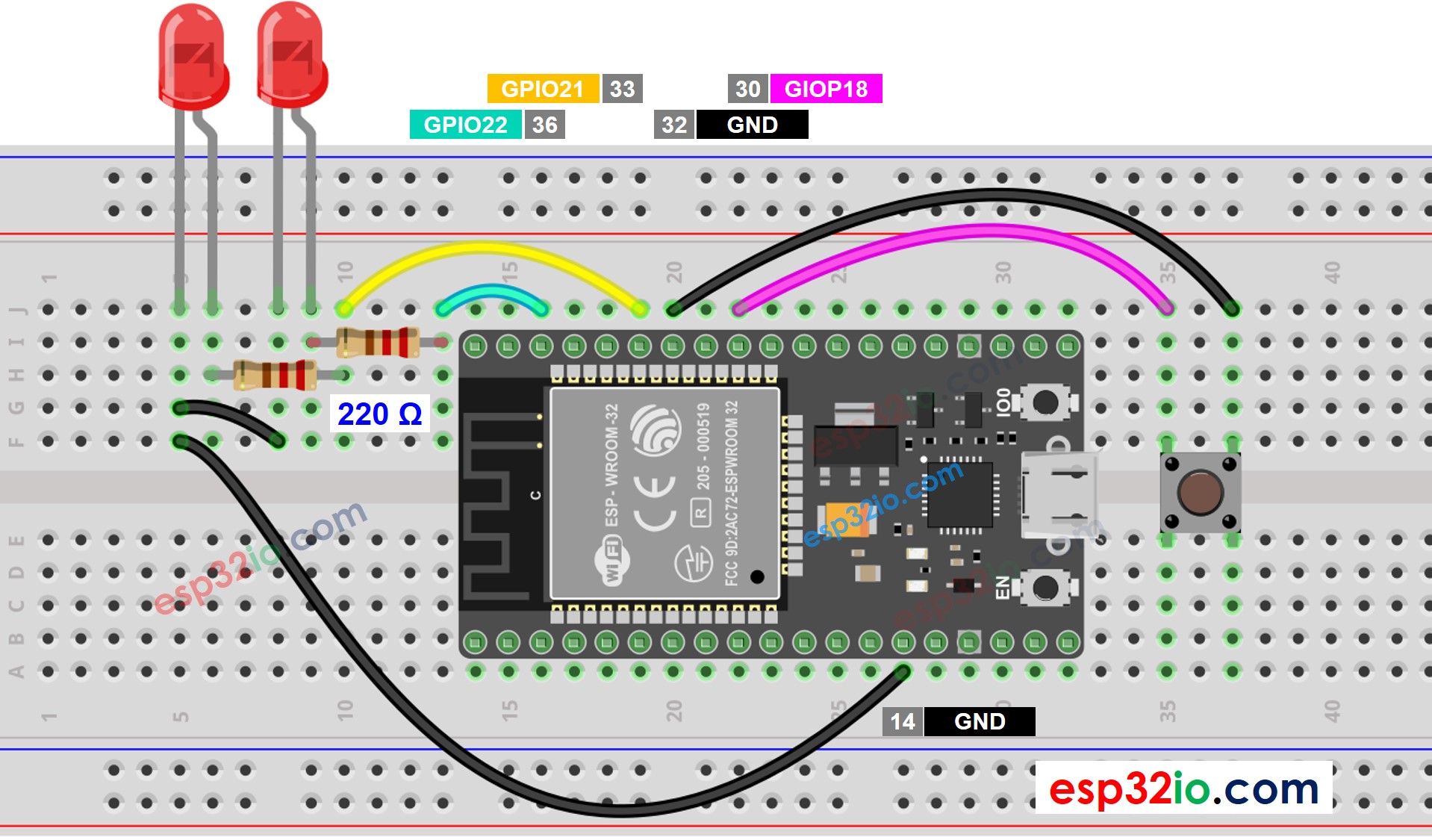
This image is created using Fritzing. Click to enlarge image
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.