ESP32 - Button - Debounce
When a button is pressed/released or when a switch is toggled between ON and OFF, its state is changed from LOW to HIGH ( or HIGH to LOW) once. Is this correct?
⇒ No, it is not. That is because in the physical world. when you do a single press on a button, the state of the button is quickly toggled between LOW and HIGH several times rather than once. This is the mechanical and physical characteristic. This phenomenon is known with a name: chattering. The chattering phenomenon makes MCU (e.g. ESP32) read multiple button presses in response to a single actual press. This results in a malfunction. The process to eliminate this phenomenon is called debounce. This tutorial shows how to do it.
This tutorial provides:
How to debounce for a button on ESP32 code
How to debounce for a button on ESP32 code using library
How to debounce for multiple buttons on ESP32 code using library
This tutorial shows how to program the ESP32 using the Arduino language (C/C++) via the Arduino IDE. If you’d like to learn how to program the ESP32 with MicroPython, visit this ESP32 MicroPython - Button - Debounce tutorial.
Or you can buy the following kits:
Disclosure: Some of the links in this section are Amazon affiliate links, meaning we may earn a commission at no additional cost to you if you make a purchase through them. Additionally, some links direct you to products from our own brand,
DIYables .
We have specific tutorials about button. The tutorial contains detailed information and step-by-step instructions about hardware pinout, working principle, wiring connection to ESP32, ESP32 code... Learn more about them at the following links:
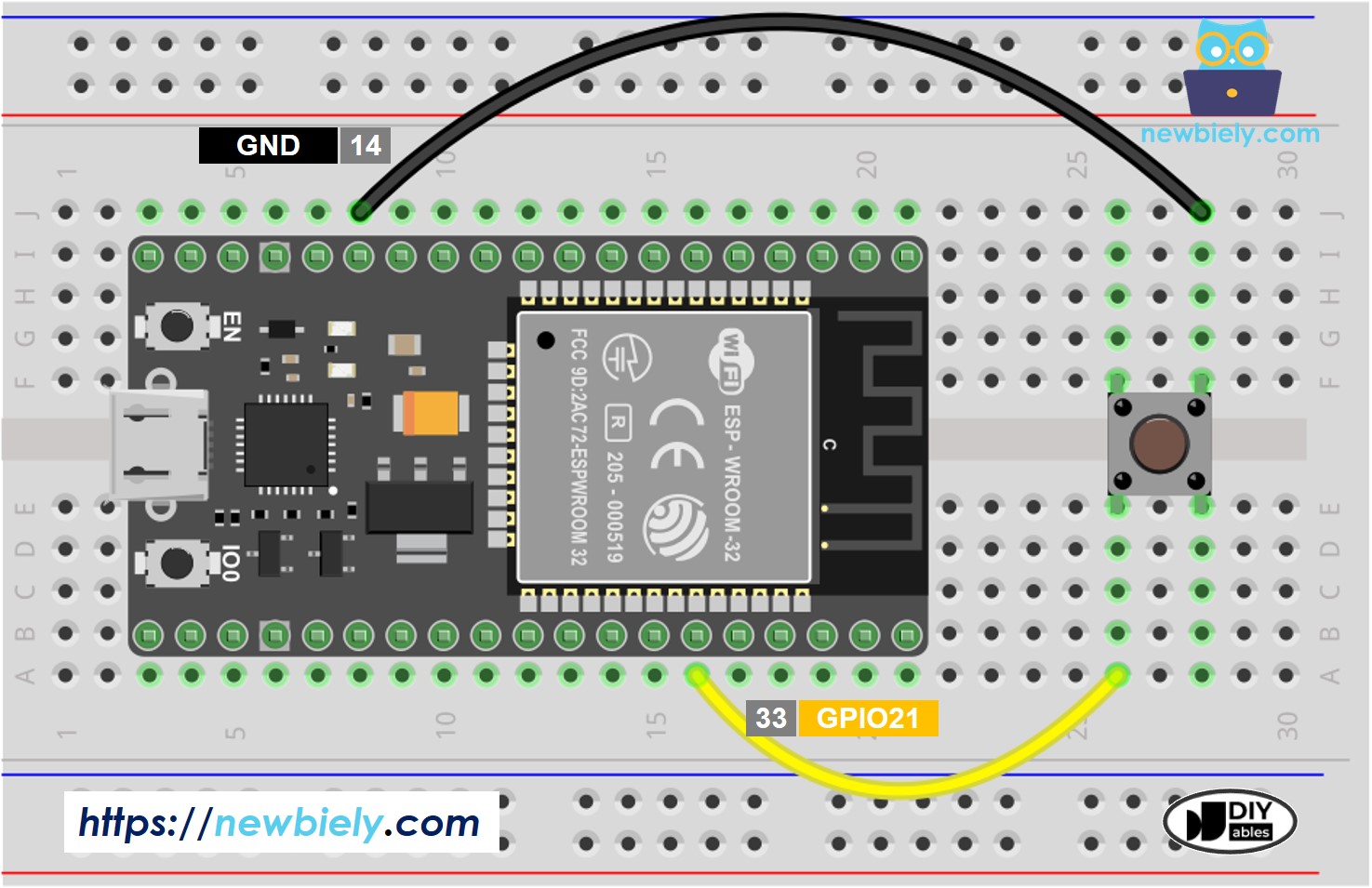
This image is created using Fritzing. Click to enlarge image
To make it clear, let's run ESP32 code WITHOUT and WITH debounce, and compare their results
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
Do the wiring as above image.
Connect the ESP32 board to your PC via a micro USB cable
Open Arduino IDE on your PC.
Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
Copy the below code and paste it to Arduino IDE.
#define BUTTON_PIN 21
int lastState = LOW;
int currentState;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
currentState = digitalRead(BUTTON_PIN);
if (lastState == HIGH && currentState == LOW)
Serial.println("The button is pressed");
else if (lastState == LOW && currentState == HIGH)
Serial.println("The button is released");
lastState = currentState;
}
Press the button once but keep it several seconds, and then release it.
See the result on Serial Monitor. It looks like the below:
The button is pressed
The button is pressed
The button is pressed
The button is released
The button is released
⇒ As you can see, you did only a single press and release, but ESP32 read multiple presses and releases.
※ NOTE THAT:
The chattering phenomenon does not happen all times. If it does not happen, please try the above test several time.
#define BUTTON_PIN 21
#define DEBOUNCE_TIME 50
int lastSteadyState = LOW;
int lastFlickerableState = LOW;
int currentState;
unsigned long lastDebounceTime = 0;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
currentState = digitalRead(BUTTON_PIN);
if (currentState != lastFlickerableState) {
lastDebounceTime = millis();
lastFlickerableState = currentState;
}
if ((millis() - lastDebounceTime) > DEBOUNCE_TIME) {
if(lastSteadyState == HIGH && currentState == LOW)
Serial.println("The button is pressed");
else if(lastSteadyState == LOW && currentState == HIGH)
Serial.println("The button is released");
lastSteadyState = currentState;
}
}
The button is pressed
The button is released
⇒ As you can see, you did one press and release, and ESP32 read one press and release. The chattering is eliminated.
To make it easy for newbies, especially when deboucing for multiple buttons, we made a button library, called ezButton. You can learn about ezButton library here.
#include <ezButton.h>
#define DEBOUNCE_TIME 50
ezButton button(21);
void setup() {
Serial.begin(9600);
button.setDebounceTime(DEBOUNCE_TIME);
}
void loop() {
button.loop();
if (button.isPressed())
Serial.println("The button is pressed");
if (button.isReleased())
Serial.println("The button is released");
}
Let's write debounce code for three buttons.
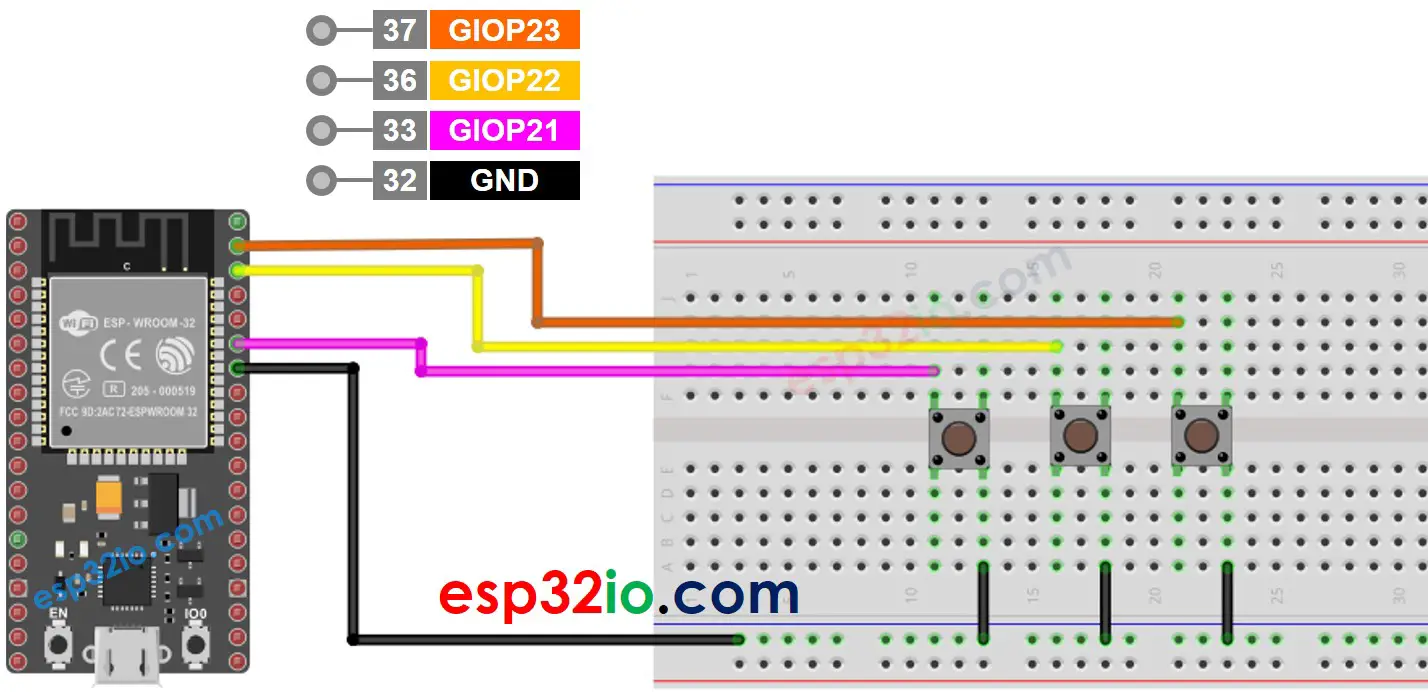
This image is created using Fritzing. Click to enlarge image
#include <ezButton.h>
#define DEBOUNCE_TIME 50
ezButton button1(21);
ezButton button2(22);
ezButton button3(23);
void setup() {
Serial.begin(9600);
button1.setDebounceTime(DEBOUNCE_TIME);
button2.setDebounceTime(DEBOUNCE_TIME);
button3.setDebounceTime(DEBOUNCE_TIME);
}
void loop() {
button1.loop();
button2.loop();
button3.loop();
if (button1.isPressed())
Serial.println("The button 1 is pressed");
if (button1.isReleased())
Serial.println("The button 1 is released");
if (button2.isPressed())
Serial.println("The button 2 is pressed");
if (button2.isReleased())
Serial.println("The button 2 is released");
if (button3.isPressed())
Serial.println("The button 3 is pressed");
if (button3.isReleased())
Serial.println("The button 3 is released");
}
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.
DEBOUNCE_TIME value depends on the hardware. Different hardware may use different values.
The debounce should also apply for on/off switch, limit switch, reed switch, touch sensor ...