ESP32 - RFID - Servo Motor
In this tutorial, we are going to learn how to use RFID/NFC tag to control servo motor using ESP32, It works as follows:
- If an authorized tag is tapped, ESP32 rotates servo motor to 90°
- If an authorized tag is tapped again, ESP32 rotates servo motor back to 0°
- The above process is repeated infinitely
This can be applied to lock/unlock cabinet, drawer, door, or open/clock the pet feeder...
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to RFID/NFC RC522 Module and Servo Motor
If you do not know about RFID/NFC RC522 Module and Servo Motor (pinout, how it works, how to program ...), learn about them in the following tutorials:
- ESP32 - RFID/NFC RC522 tutorial
- ESP32 - Servo Motor tutorial
How It Works
- The UIDs of some RFID/NFC tags are preset in ESP32 code
- User taps an RFID/NFC tag on RFID/NFC reader
- The reader reads UID from the tag.
- ESP32 gets the UID from the reader
- ESP32 compares the read UID with the predefined UIDs
- If the UID is matched with one of the predefined UIDs, ESP32 controls servo motor to 90°.
- If the tag is tapped again, ESP32 controls servo motor back to 0°
- This process is repeated infinitely.
Wiring Diagram
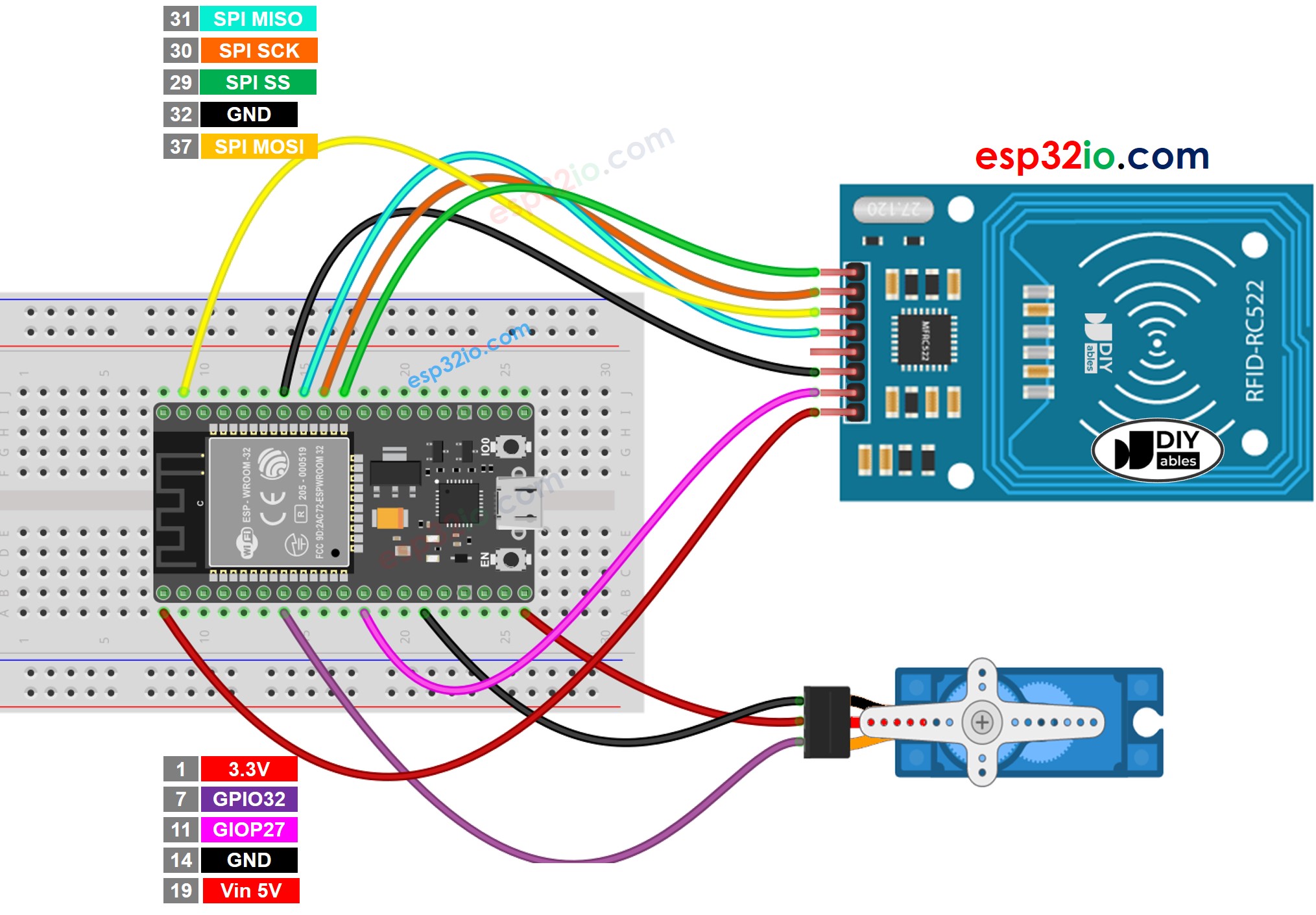
This image is created using Fritzing. Click to enlarge image
※ NOTE THAT:
The order of pins can vary according to manufacturers. ALWAYS use the labels printed on the module. The above image shows the pinout of the modules from DIYables manufacturer.
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
ESP32 Code - Single RFID/NFC Tag
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search “MFRC522”, then find the library by GithubCommunity
- Click Install button to install MFRC522 library.
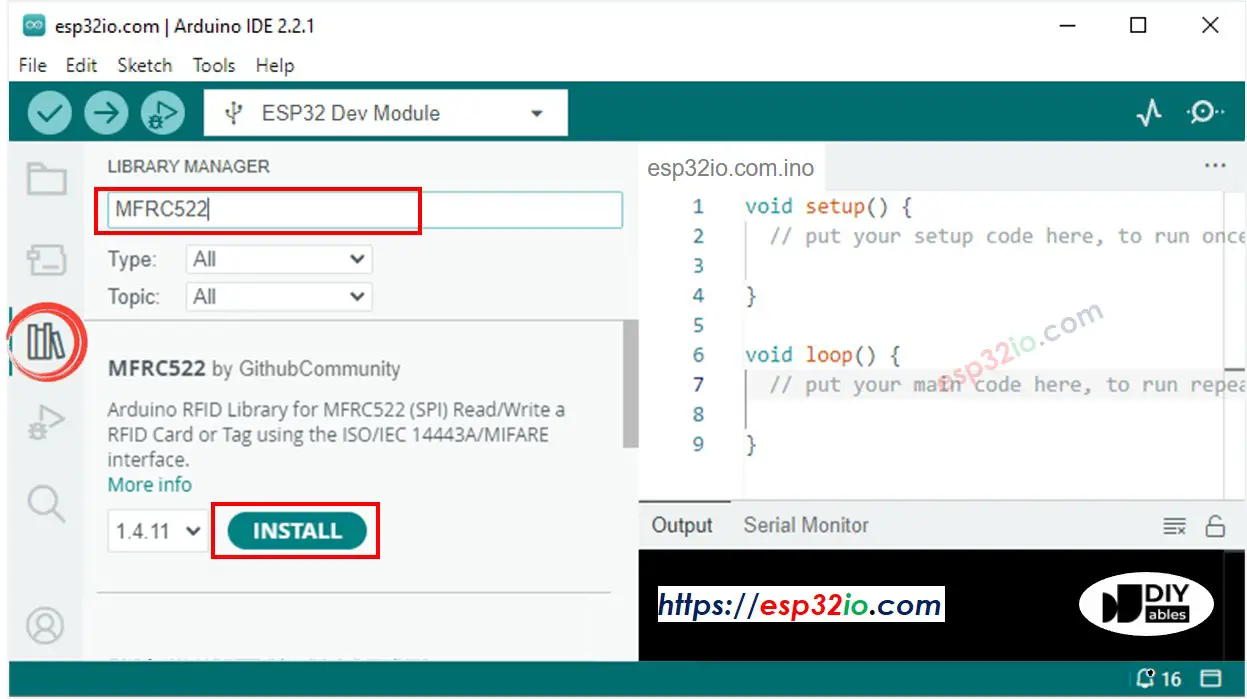
- Type ServoESP32 on the search box, then look for the servo library by Jaroslav Paral. Please be aware that both version 1.1.1 and 1.1.0 are affected by bugs. Kindly choose a different version.
- Click Install button to install servo motor library for ESP32.
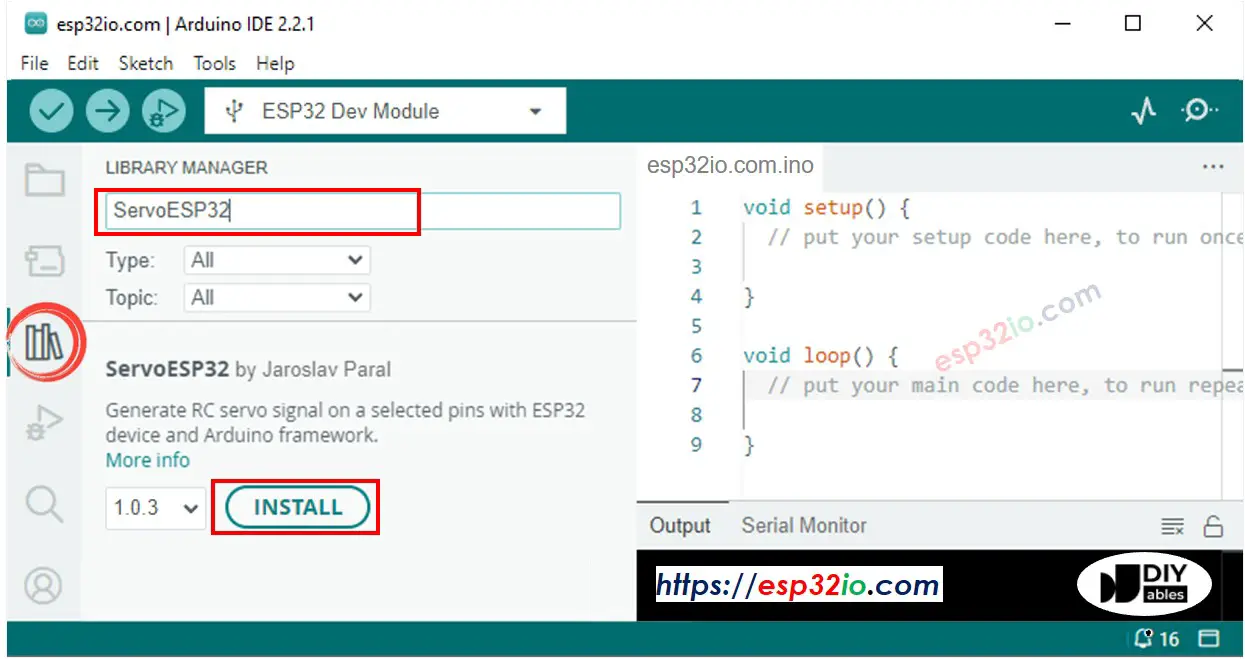
Because UID is usually not printed on RFID/NFC tag, The first step we need to do is to find out the tag's UID. This can be done by:
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
- Open Serial Monitor
- Tap an RFID/NFC tag on RFID-RC522 module
- Get UID on Serial Monitor
After having UID:
- Update UID in the line 20 of the above code. For example, change byte authorizedUID[4] = {0xFF, 0xFF, 0xFF, 0xFF}; TO byte authorizedUID[4] = {0x3A, 0xC9, 0x6A, 0xCB};
- Upload the code to ESP32 again
- Tap an RFID/NFC tag on RFID-RC522 module
- You will see the servo motor rotate to 90°
- See output on Serial Monitor
- Tap the same RFID/NFC tag on RFID-RC522 module again
- You will see the servo motor rotate to 0°
- See output on Serial Monitor
- Tap another RFID/NFC tag on RFID-RC522 module
- See output on Serial Monitor
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.