ESP32 - Gmail
In this tutorial, we will learn how to program the ESP32 to send an email from your Gmail account. The email sender will be a Gmail account, and the recipient can be any type of email account.
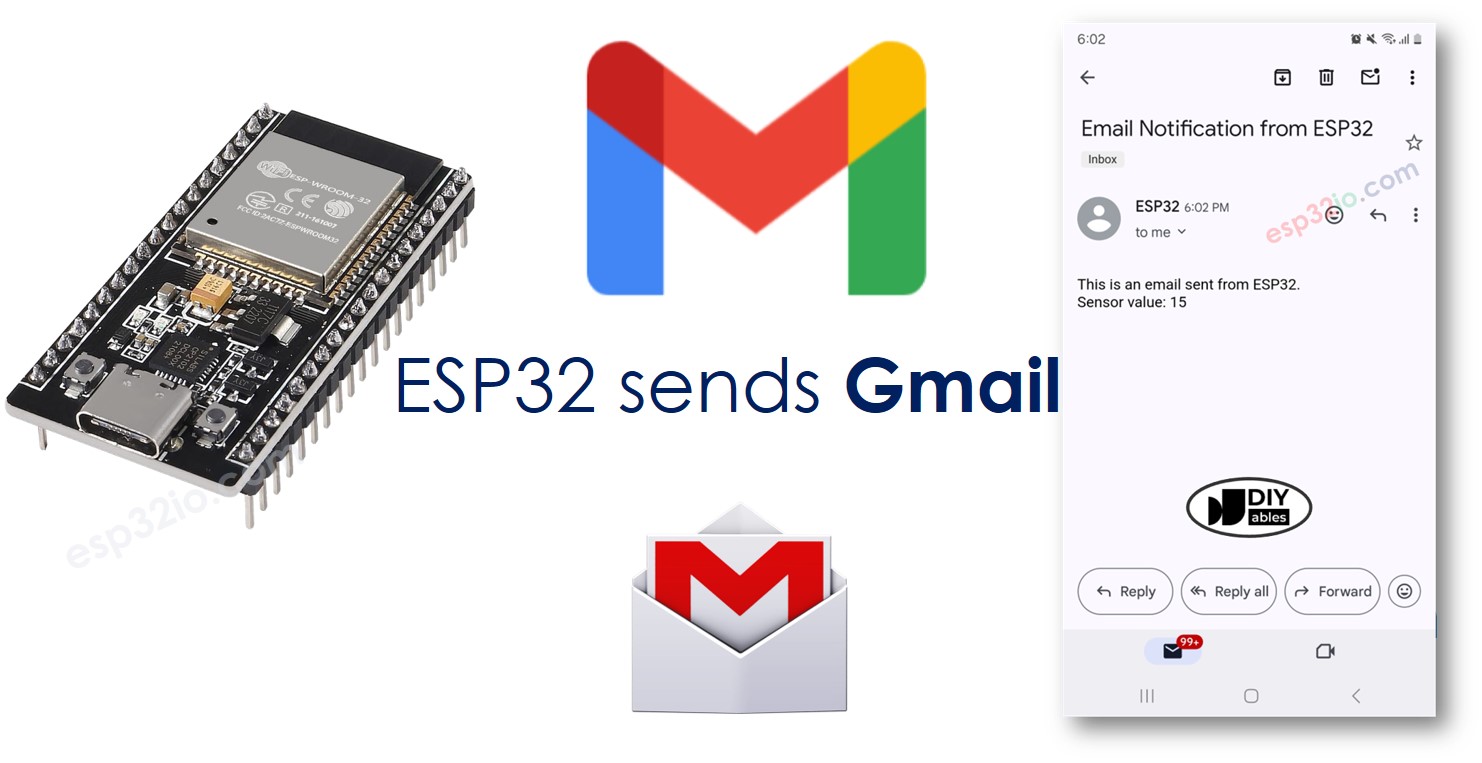
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Pre-Preparation
We need a Gmail account and its corresponding app password to be used in the code. Here are the key points to note:
- Do not use your current Gmail account. Create a new one specifically for testing to avoid any potential issues.
- The password used in the ESP32 code is not the same as the one you use to log in to Gmail via a web browser. You need to follow certain steps to obtain an "App Password" from your Google Account.
Here is a step-by-step guide:
- Create a new Gmail account.
- Log in to the newly created account.
- Go to your Google Account.
- Navigate to the "Security" section.
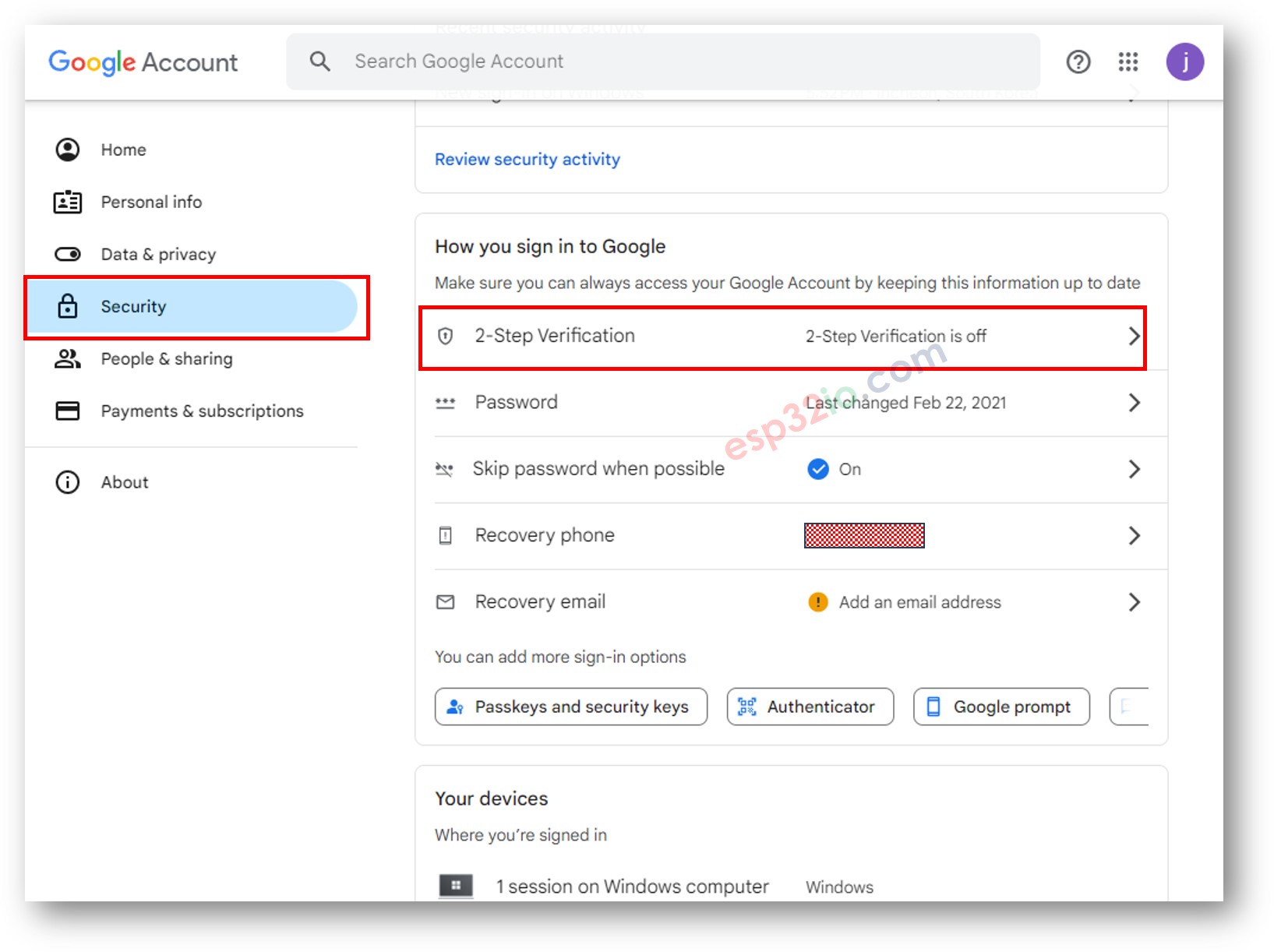
- Enable 2-Step Verification (App passwords can only be used with accounts that have 2-Step Verification turned on).
- Visit the Google App Passwords page and create an app password. You can give it any name.
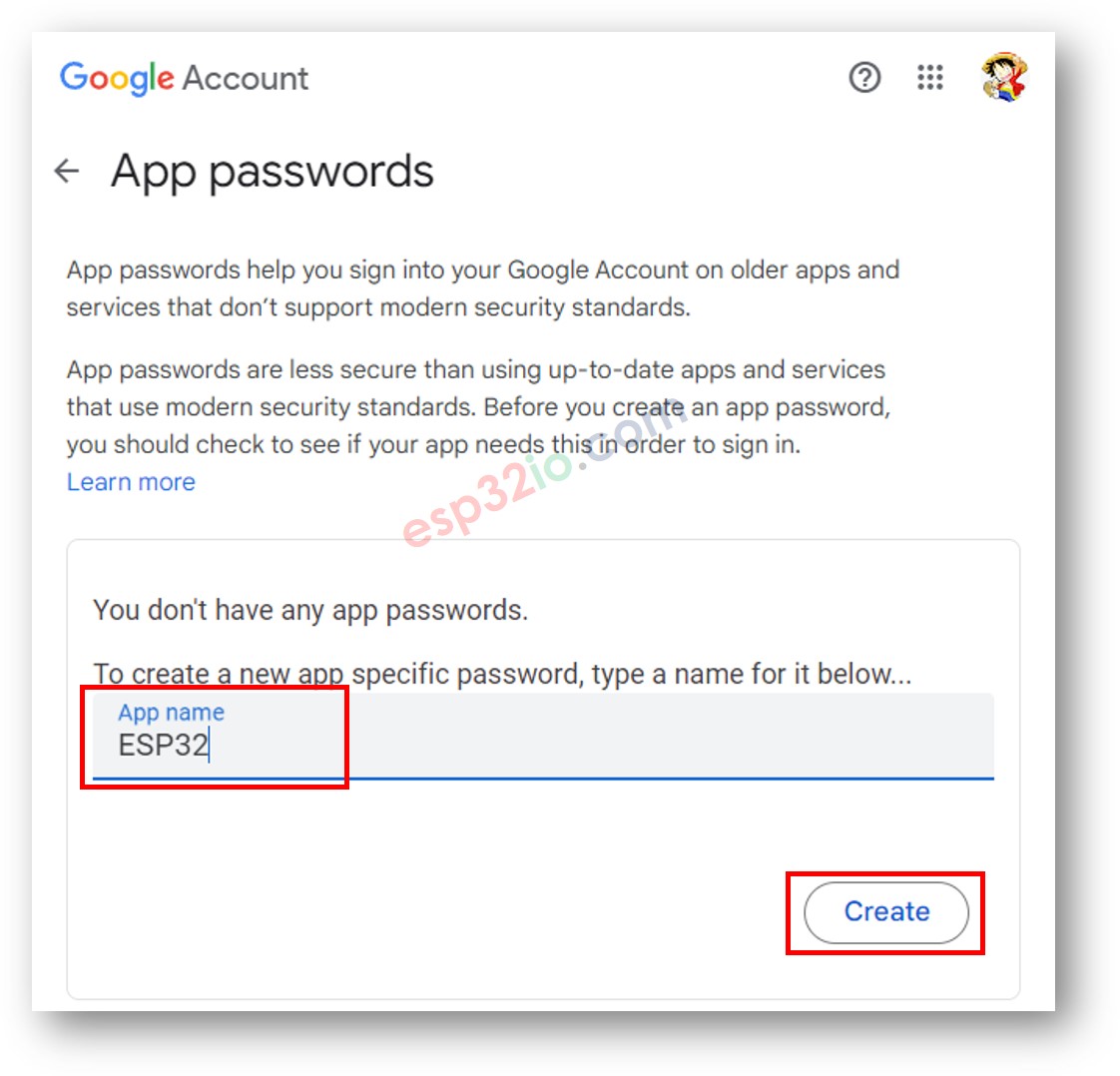
- Click the Create button. You will receive a 16-digit password that looks like this:
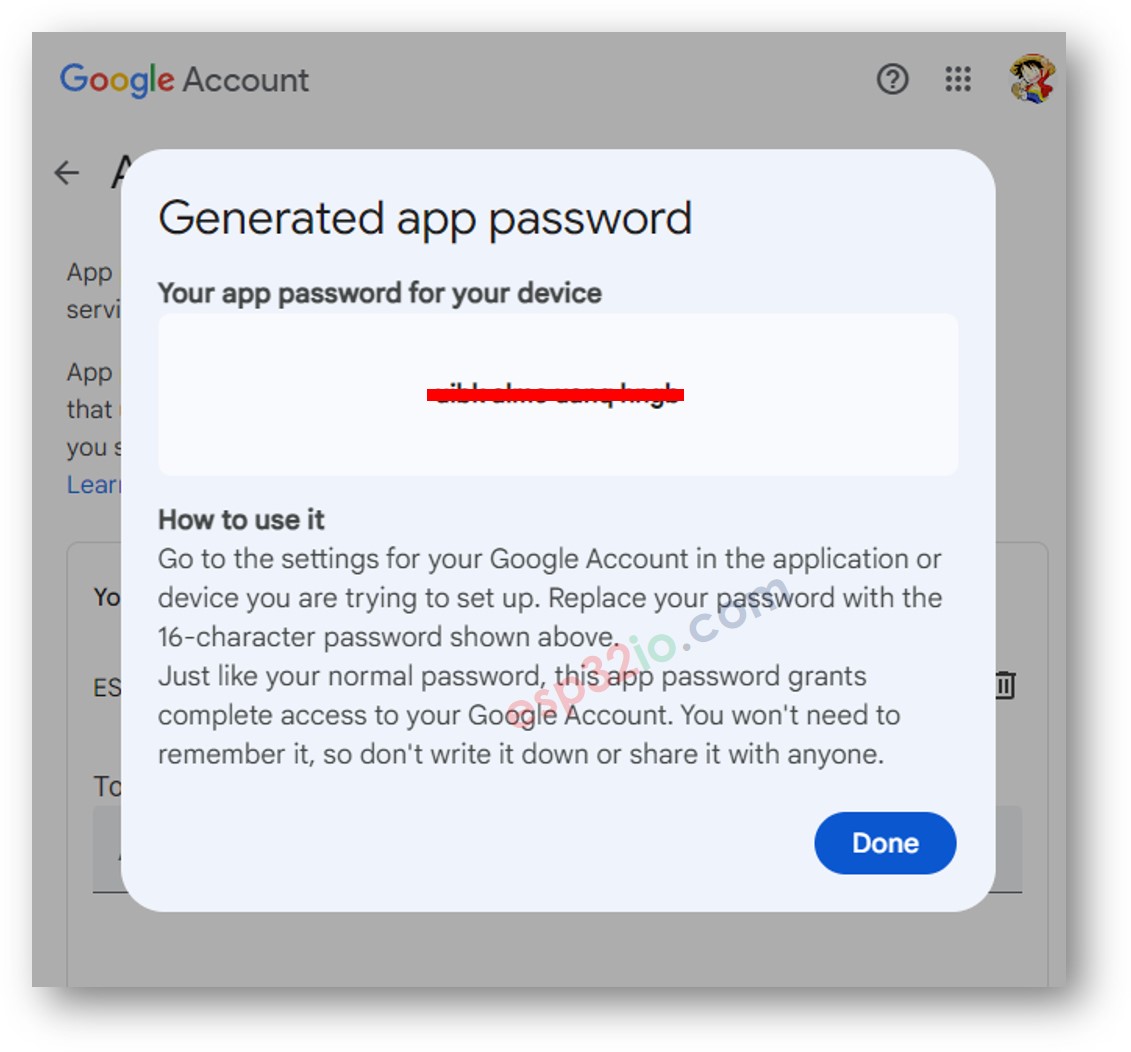
- Copy and store this 16-digit number. It will be used in the ESP32 code in the next part.
※ NOTE THAT:
Google may change its user interface over time. If you follow the steps above but cannot obtain the "App Passwords," try searching for "How to get Google App Passwords" for the latest instructions.
ESP32 Code
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Connect the ESP32 board to your PC via a USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Open the Library Manager by clicking on the Library Manager icon on the left navigation bar of Arduino IDE.
- Search ESP Mail Client, then find the ESP Mail Client created by Mobizt.
- Click Install button to install ESP Mail Client library.
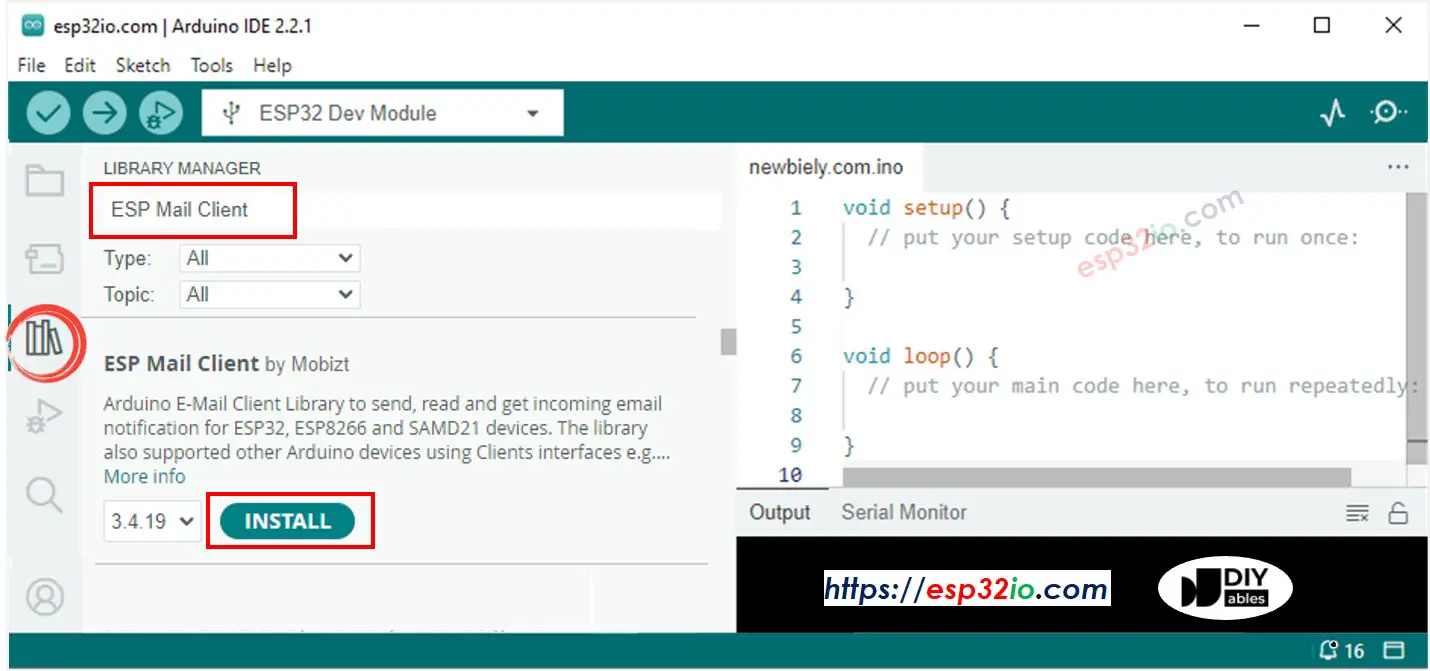
- Copy the above code and open with Arduino IDE
- Change the WiFi information (SSID and password) in the code to yours by changing two contanst WIFI_SSID and WIFI_PASSWORD
- Change the sender email and password in the code to yours by changing two contanst SENDER_EMAIL and SENDER_PASSWORD
- Change the recipient email in the code to yours by changing two contanst RECIPIENT_EMAIL. recipient email can be the same as sender
※ NOTE THAT:
- Sender email MUST be Gmail
- Sender password is the App passwords you got from previous step
- Recipient email can be any type of email
- Click Upload button on Arduino IDE to upload code to ESP32
- Open the Serial Monitor
- Check out the result on Serial Monitor.
- Check your recipient email box. You will get an email as below:
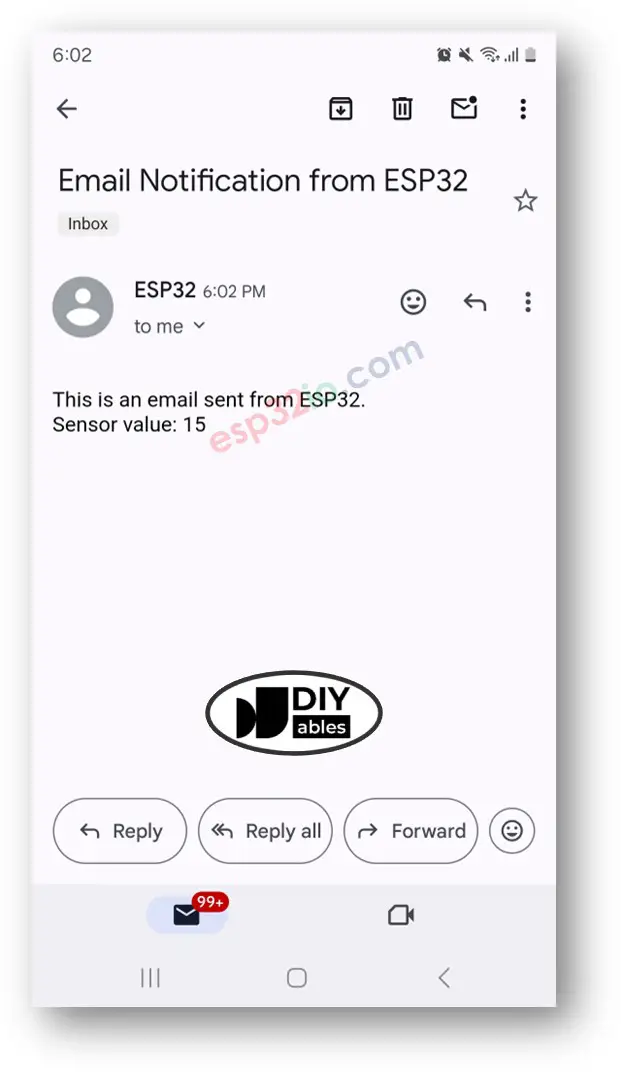
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.