ESP32 - SD Card
In this guide, we'll explore how to use a Micro SD Card with the ESP32. We'll delve into the following topics:
- How to open a file on the Micro SD Card using the ESP32, and also how to create a new one if it doesn't already exist.
- How to write data to a file on the Micro SD Card using the ESP32.
- How to read a file on the Micro SD Card character by character using the ESP32.
- How to read a file on the Micro SD Card line by line using the ESP32.
- How to add more content to an existing file on the Micro SD Card using the ESP32.
- How to replace the content of a file on the Micro SD Card using the ESP32.
Hardware Used In This Tutorial
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to Micro SD Card Module
The Micro SD Card Module can interface with ESP32 and it can carry a Micro SD Card. In another word, the Micro SD Card Module is a bridge between ESP32 and Micro SD Card
Pinout
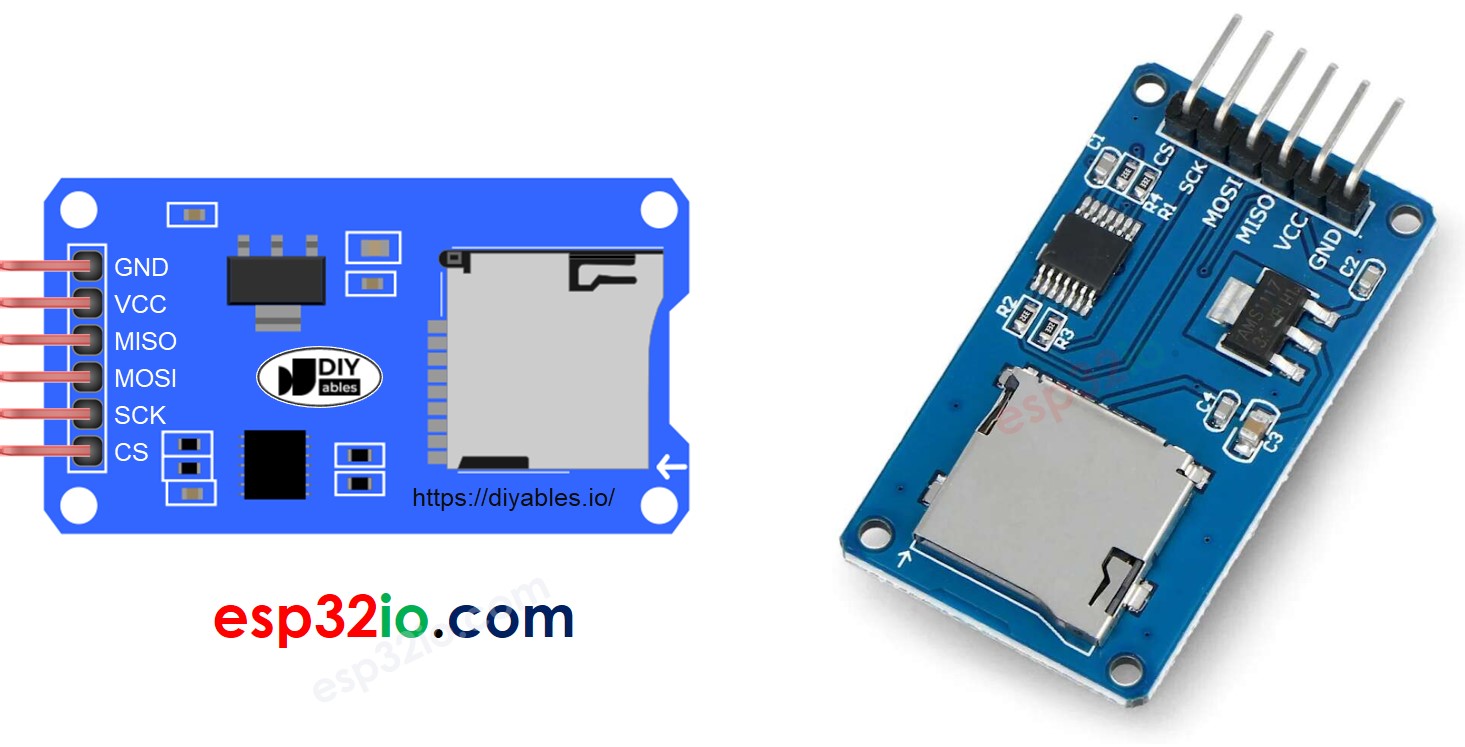
Micro SD Card Module includes 6 pins:
- VCC pin: connect to the ESP32's 5V pin.
- GND pin: connect this pin to the ESP32's GND.
- MISO pin: (Master In Slave Out) connect this pin to the ESP32's MISO pin.
- MOSI pin: (Master Out Slave In) connect this pin to the ESP32's MOSI pin.
- SCK pin: connect this pin to the ESP32's SCK pin.
- SS pin: (Slave Select) connect this pin to the pin specified in ESP32 code as a SS pin.
Preparation
- Connect the Micro SD Card to the PC via USB 3.0 SD Card Reader
- Make sure that the Micro SD Card is formatted FAT16 or FAT32 (Google for it)
Wiring Diagram
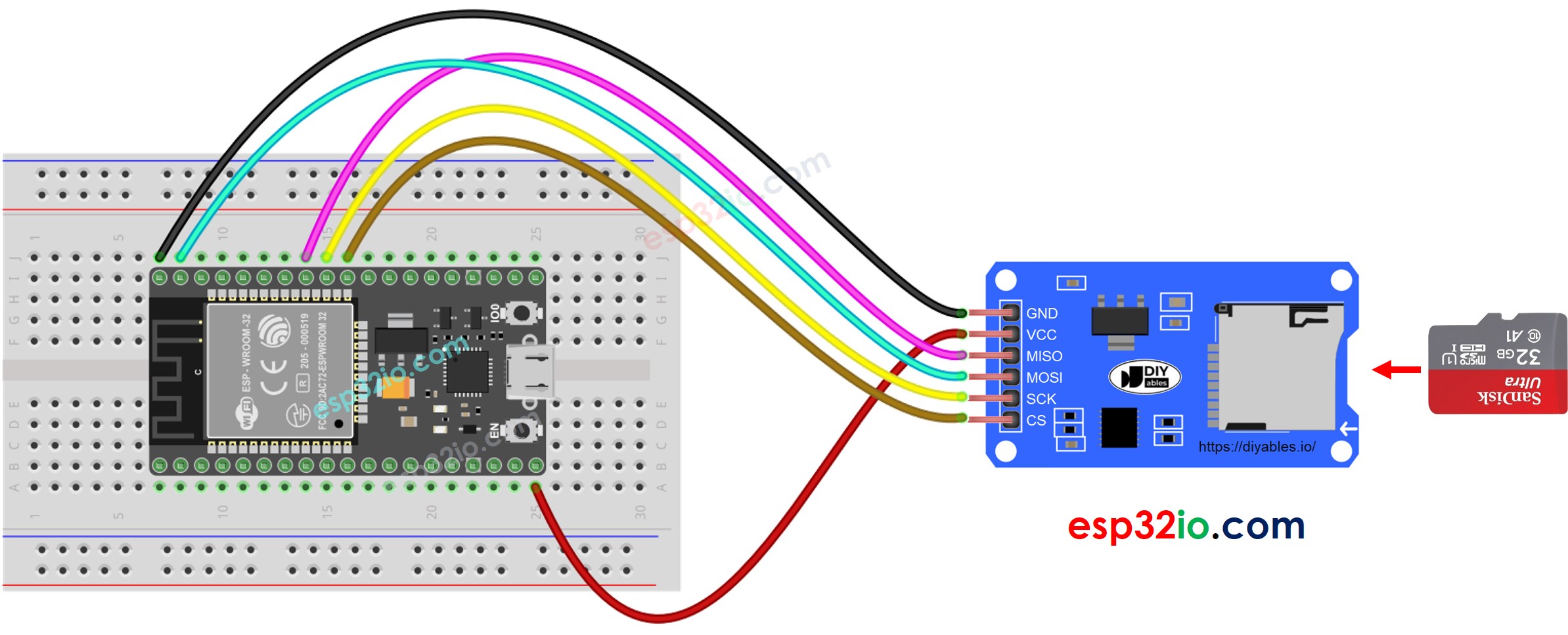
This image is created using Fritzing. Click to enlarge image
※ NOTE THAT:
If you use an Ethernet shield or any shield that has a Micro SD Card Holder, you do not need to use the Micro SD Card Module. You just need to insert the Micro SD Card to the Micro SD Card Holder on the shield.
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
ESP32 - How to open a file on Micro SD Card and create if not existed
ESP32 Code
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Insert the Micro SD Card to the Micro SD Card module
- Do the wiring between the Micro SD Card module and ESP32 as the above wiring diagram
- Connect ESP32 to PC via USB cable
- Open Arduino IDE, select the right board and port
- Open Serial On Arduino IDE
- Copy the above code and paste it to Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
- The result on Serial Monitor for the first run
- The result on Serial Monitor for the next runs
※ NOTE THAT:
You may NOT see the output on Serial Monitor for the first run of your first upload is done before opening Serial Monitor.
- Detach the Micro SD Card from the module
- Insert the Micro SD Card to an USB SD Card reader
- Connect the USB SD Card reader to the PC
- Check if the file exists or not
ESP32 - How to write/read data to/from a file on Micro SD Card
The below code does:
- Write data to a file
- Read the content of the a file character-by-character and print it to Serial Monitor
- The Serial Monitor shown the content of the file
※ NOTE THAT:
The data will be appended to the end of file by default. If you reboot ESP32 with above code, the text will be appended to the file again ⇒ the Serial Monitor will shows more lines as below:
You can also detach the Micro SD Card from the module, and open it on your PC to check the content (USB SD Card reader is needed)
ESP32 - How to read a file on Micro SD Card line-by-line
- The result on Serial Monitor
※ NOTE THAT:
You may see more lines on Serial Monitor if the content of the file is not deleted before.
ESP32 - How to overwrite a file on Micro SD Card
By default, the content will append to the end of the file. The simplest way to overwrite a file is: delete the exsiting file and create new one with the same name
- The result on Serial Monitor
- Reboot ESP32
- Check if the content of the file on Serial Monitor is appended or not.
You can also detach the Micro SD Card from the module, and open it on your PC to check the content (USB SD Card reader is needed)
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.