ESP32 - Automatic Irrigation System
This tutorial instructs you how to make an automatic irrigation system for the garden using ESP32, a soil moisture sensor, relay, and pump. In detail:
- When soil moisture is dry, ESP32 automatically turns a pump on to water plants.
- When soil moisture is wet, ESP32 automatically turns a pump off.
Hardware Used In This Tutorial
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to Soil Moisture Sensor and Pump
We have specific tutorials about soil moisture sensor and pump. Each tutorial contains detailed information and step-by-step instructions about hardware pinout, working principle, wiring connection to ESP32, ESP32 code... Learn more about them at the following links:
- ESP32 - Soil Moisture Sensor tutorial
- ESP32 - Controls Pump tutorial
Wiring Diagram
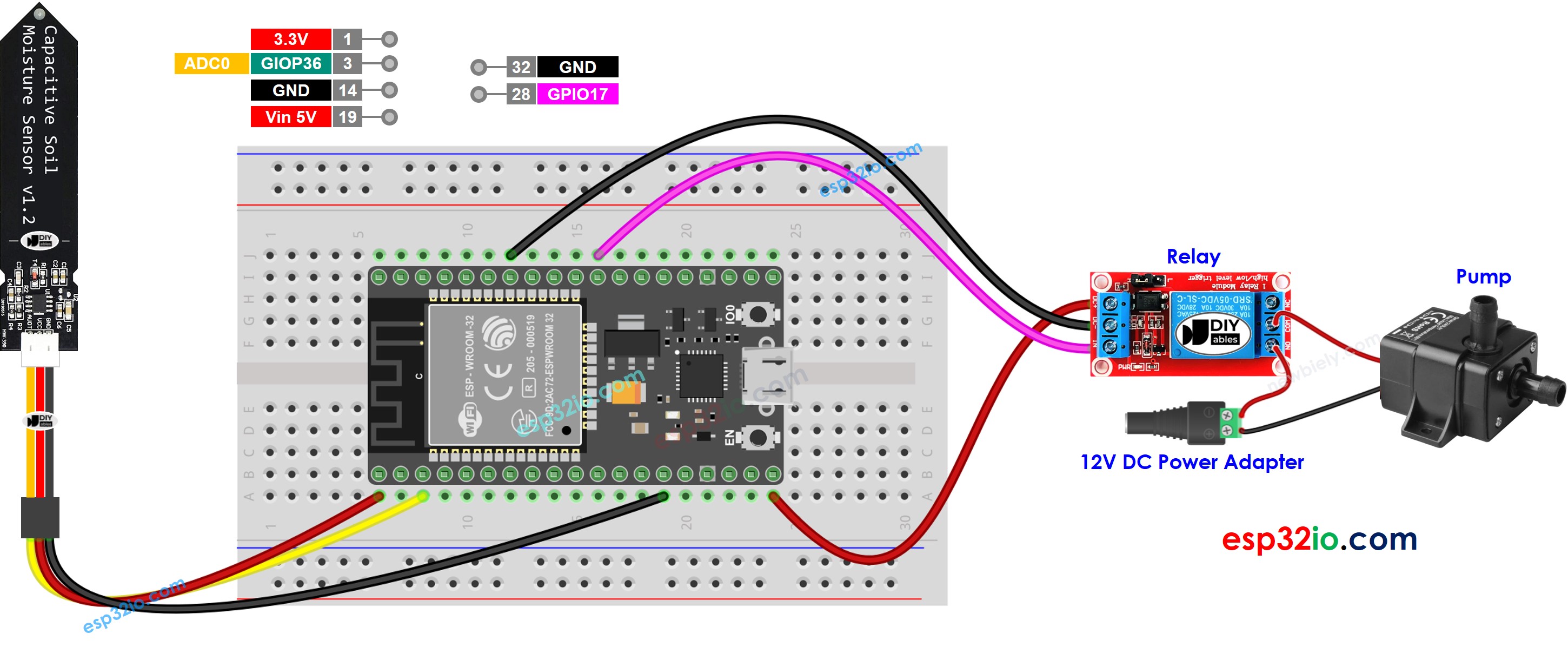
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
ESP32 Code
Quick Instructions
- Do calibration to determine the wet-dry THRESHOLD, see ESP32 - Calibrates Soil Moisture Sensor
- Update the calibrated THRESHOLD value in the code
- Open Serial Monitor on Arduino IDE
- Upload the code to ESP32 board
- See the result on Serial Monitor. It looks like the below:
Code Explanation
Read the line-by-line explanation in the comment lines of the source code!
※ NOTE THAT:
This tutorial uses the analogRead() function to read values from an ADC (Analog-to-Digital Converter) connected to a soil moisture sensor. The ESP32 ADC is good for projects that do NOT need high accuracy. However, for projects that need precise measurements, please note:
- The ESP32 ADC is not perfectly accurate and might need calibration for correct results. Each ESP32 board can be a bit different, so you need to calibrate the ADC for each individual board.
- Calibration can be difficult, especially for beginners, and might not always give the exact results you want.
For projects that need high precision, consider using an external ADC (e.g ADS1115) with the ESP32 or using an Arduino, which has a more reliable ADC. If you still want to calibrate the ESP32 ADC, refer to ESP32 ADC Calibration Driver
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.