ESP32 - Potentiometer
This tutorial instructs you how to use ESP32 with the potentiometer. In detail, we will learn:
- How the potentiometer works
- How to interface ESP32 with a potentiometer
- How to program ESP32 to read the value from a potentiometer
- How to convert the value read from a potentiometer to the useful value
This tutorial shows how to program the ESP32 using the Arduino language (C/C++) via the Arduino IDE. If you’d like to learn how to program the ESP32 with MicroPython, visit this ESP32 MicroPython - Potentiometer tutorial.
Hardware Used In This Tutorial
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to Potentiometer
The potentiometer (also known as rotary angle sensor) is used to change the settings (e.g. the speaker's volume, room's temperature, lamp's brightness...)
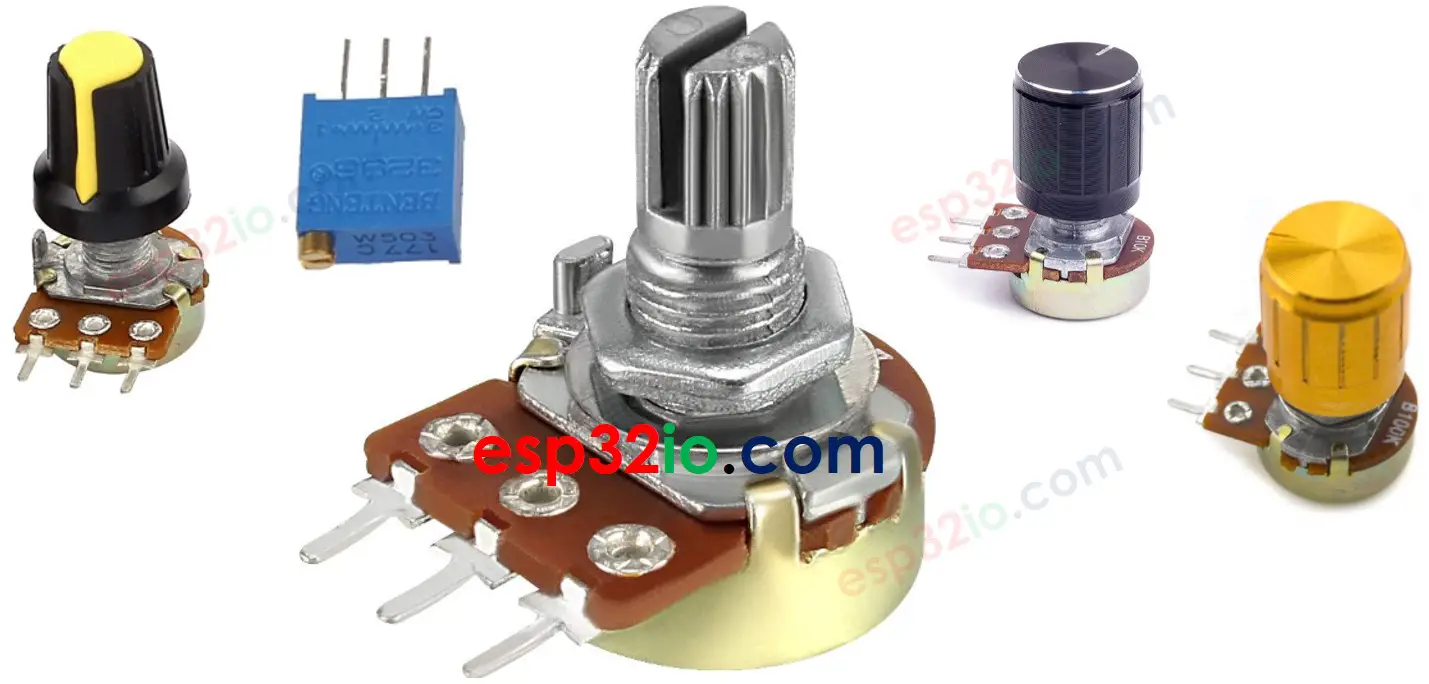
Potentiometer Pinout
A potentiometer usually has 3 pins:
- VCC pin: connect this pin to VCC (5V or 3.3v)
- GND pin: connect this pin to GND (0V)
- Output pin: outputs the voltage to ESP32's input pin.
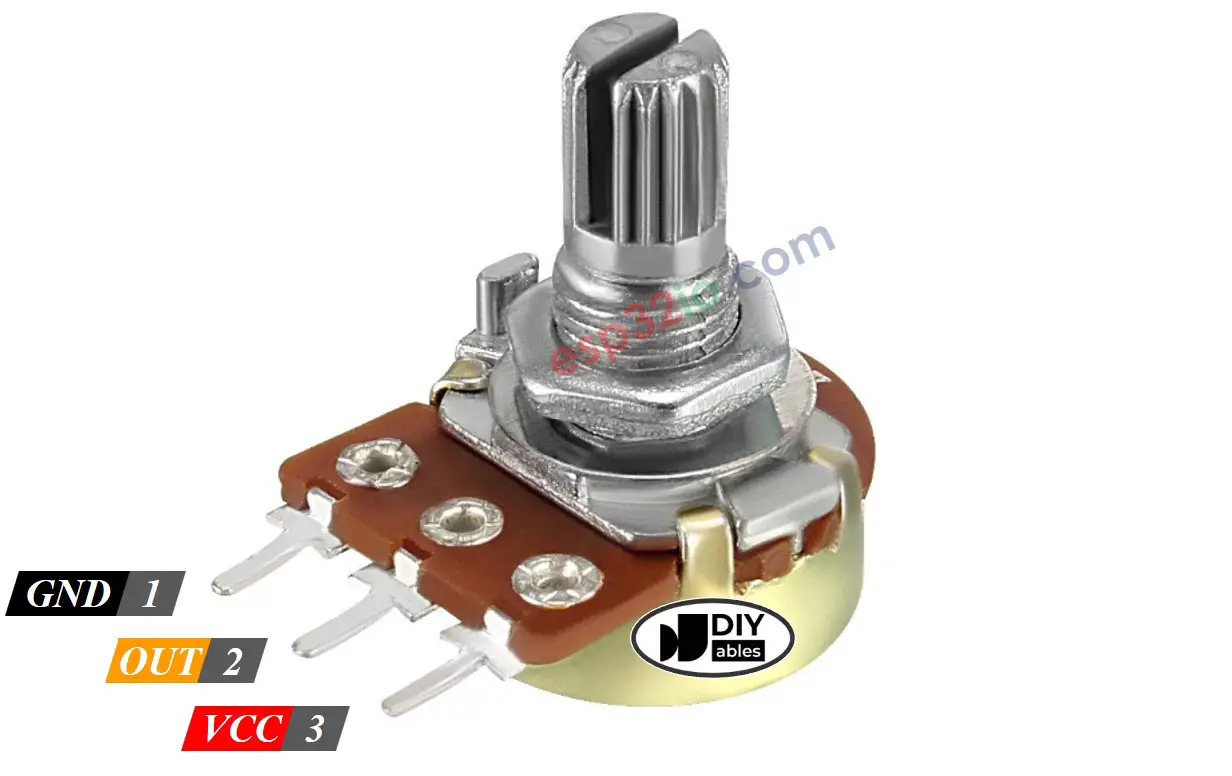
※ NOTE THAT:
The GND pin and VCC pin are interchangeable
How Potentiometer Works
The potentiometer's shaft can be rotated from 0° (closest to GND pin) to an angle (closest to VCC pin), called ANGLE_MAX.
The voltage in the output pin is in direct proportion to the angle position of the shaft, varing from 0 to VCC. The below table shows the relation between the angle and the voltage on the output pin:
Angle | Voltage |
---|---|
0° | 0v |
ANGLE_MAX° | VCC |
angle° | angle° × VCC / ANGLE_MAX° |
※ NOTE THAT:
The ANGLE_MAX value is depended on manufacturers.
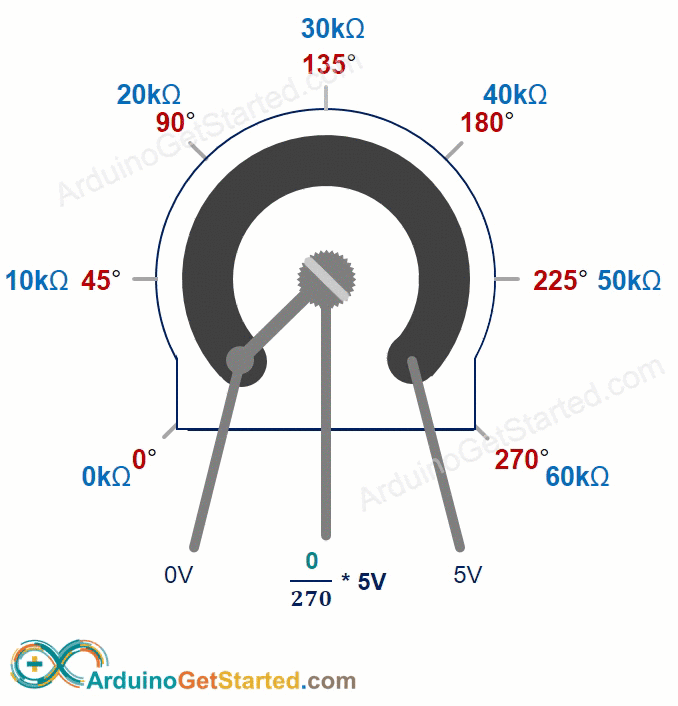
ESP32 - Rotary Potentiometer
The ESP32's analog input pin converts the voltage (between 0v and 3.3V) into integer values (between 0 and 4095), called ADC value or analog value.
We can connect the potentiometer's output pin to an ESP32's analog input pin, and then read the analog value from the pin.
The analog value from input pin can be rescaled into another value. Let's see the use cases:
- Rescale the analog value to the potentiometer's angle.
- Rescale the analog value to the potentiometer's voltage.
- Rescale the analog value to the the setting value (e.g. the speaker's volume, room's temperature, lamp's brightness...)
Rescale Range
FROM | TO | |||
---|---|---|---|---|
Angle | rotated by user | 0° | → | ANGLE_MAX° |
Voltage | from potentiometer's pin | 0V | → | 3.3V |
ADC value | read by ESP32 | 0 | → | 4095 |
Setting value | converted by ESP32 | VALUE_MIN | → | VALUE_MAX |
Wiring Diagram between Potentiometer and ESP32
- How to connect ESP32 and potentiometer using breadboard
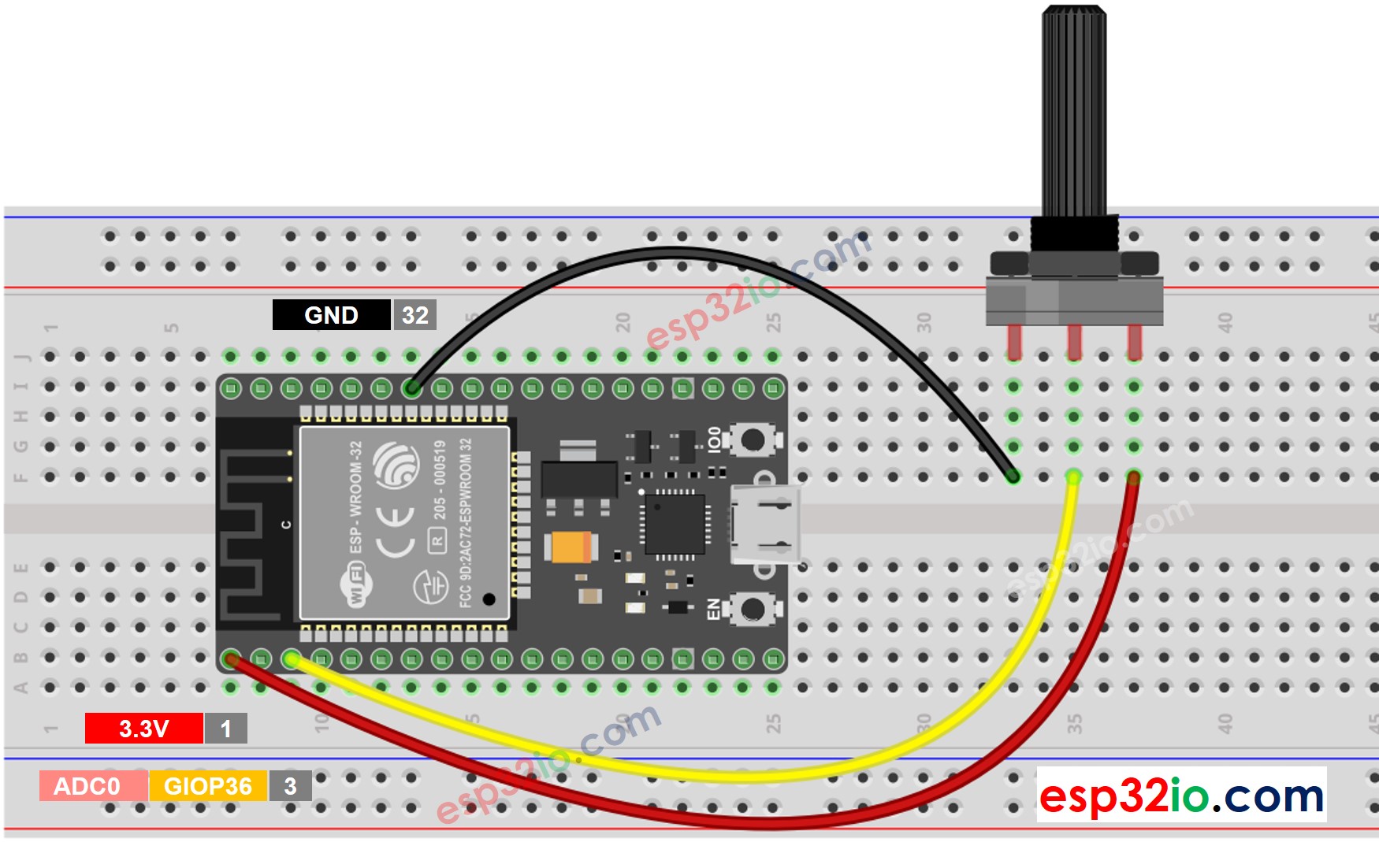
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and potentiometer using screw terminal block breakout board
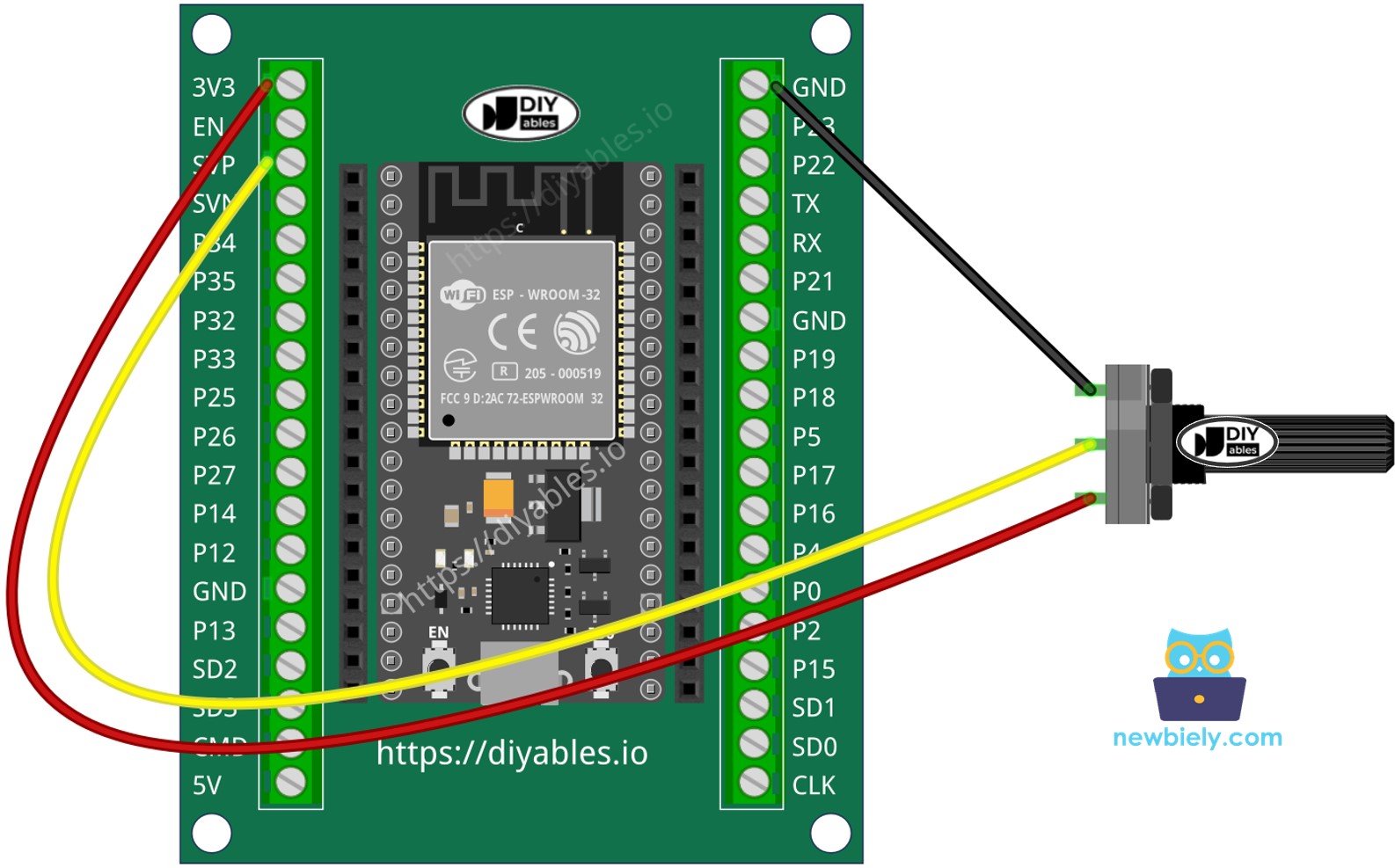
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
How To Program Potentiometer
- Read the value from an input pin, which connected to the output pin of the potentiometer by using analogRead() function.
- Rescale to the potentiometer's angle by using map() function.
- Rescale to the potentiometer's voltage:
- Rescale to the controllable value (e.g volume of stereo, brightness, speed of DC motor... )
- For example, rescaling to the brightness of LED. As mentioned in this tutorial, the brightness of LED can be controlled by using PWM value from 0 (always OFF) to 255 (always ON). Therefore, we can map the analog value to the brightness of LED (from OFF to the brightest) as follows:
If you want to dim LED from the nightlight to the brightest,
※ NOTE THAT:
The map() function can only be used to rescale the analog value to the int or long type value. If the controllable value is float type, you need to use the floatMap() function instead of the map() function.
floatMap() function:
ESP32 Code
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Copy the above code and paste it to Arduino IDE.
- Compile and upload code to ESP32 board by clicking Upload button on Arduino IDE
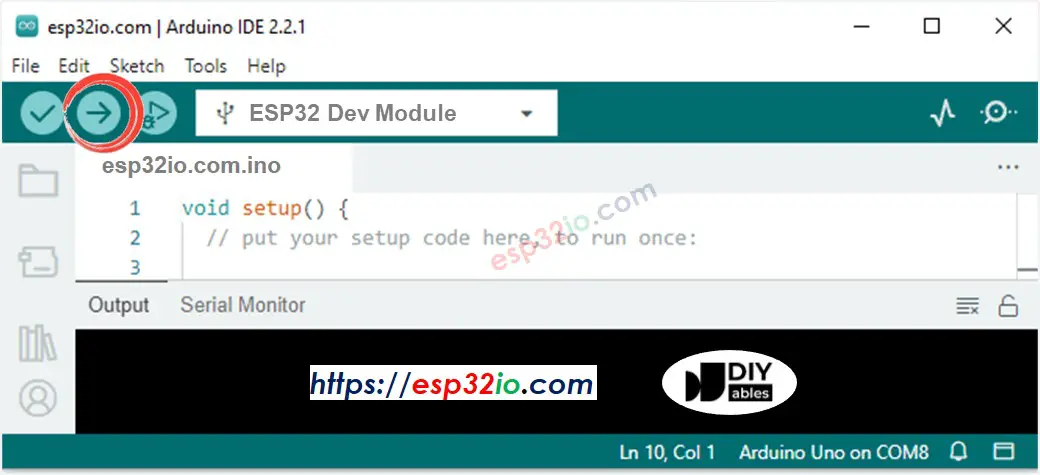
- Open Serial Monitor on Arduino IDE
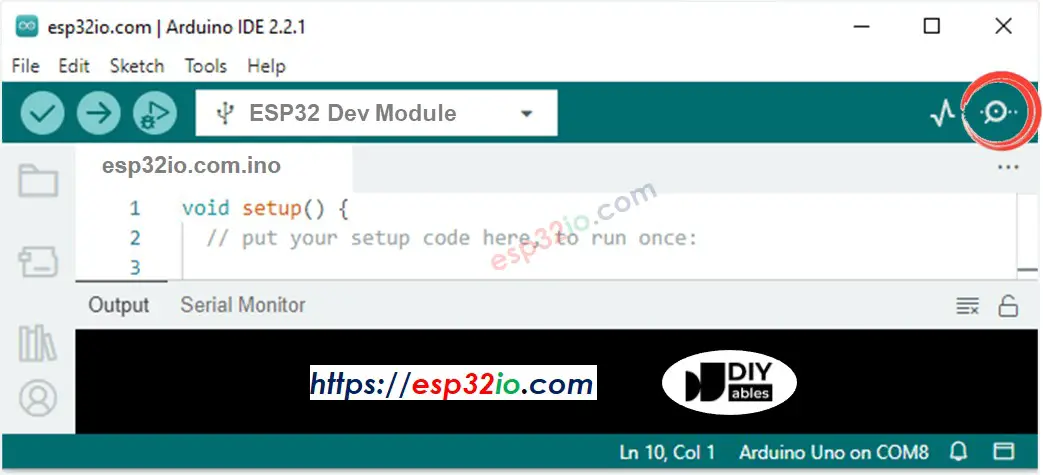
- Rotate the potentiometer
- See the result on Serial Monitor. It looks like the below:
※ NOTE THAT:
This tutorial uses the analogRead() function to read values from an ADC (Analog-to-Digital Converter) connected to a potentiometer. The ESP32 ADC is good for projects that do NOT need high accuracy. However, for projects that need precise measurements, please note:
- The ESP32 ADC is not perfectly accurate and might need calibration for correct results. Each ESP32 board can be a bit different, so you need to calibrate the ADC for each individual board.
- Calibration can be difficult, especially for beginners, and might not always give the exact results you want.
For projects that need high precision, consider using an external ADC (e.g ADS1115) with the ESP32 or using an Arduino, which has a more reliable ADC. If you still want to calibrate the ESP32 ADC, refer to ESP32 ADC Calibration Driver
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.