ESP32 - Motion Sensor - LED Strip
In this guide, we will delve into creating a seamless lighting automation system using an ESP32, an HC-SR501 motion sensor, and an LED strip. This system is expertly designed to activate the LED strip upon detecting human presence, making it versatile and well-suited for a variety of applications, including:
- Implementing the system on staircases for automatic lighting as individuals approach.
- Installing the system beneath your bed, with the light triggered as you step down from the bed to the floor.
- Establishing automatic illumination for a Christmas tree whenever humans are present.
Hardware Used In This Tutorial
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Introduction to LED Strip and Motion Sensor
Unfamiliar with LED strip and motion sensor, including their pinouts, functionality, and programming? Explore comprehensive tutorials on these topics below:
You have the flexibility to use either NeoPixel, WS2812B, or DotStar LED Strips. For the sake of simplicity in wiring, this tutorial specifically uses the DotStar LED Strip. Adapting the code for other LED strip types is straightforward, simply refer to the tutorials above for guidance.
Wiring Diagram
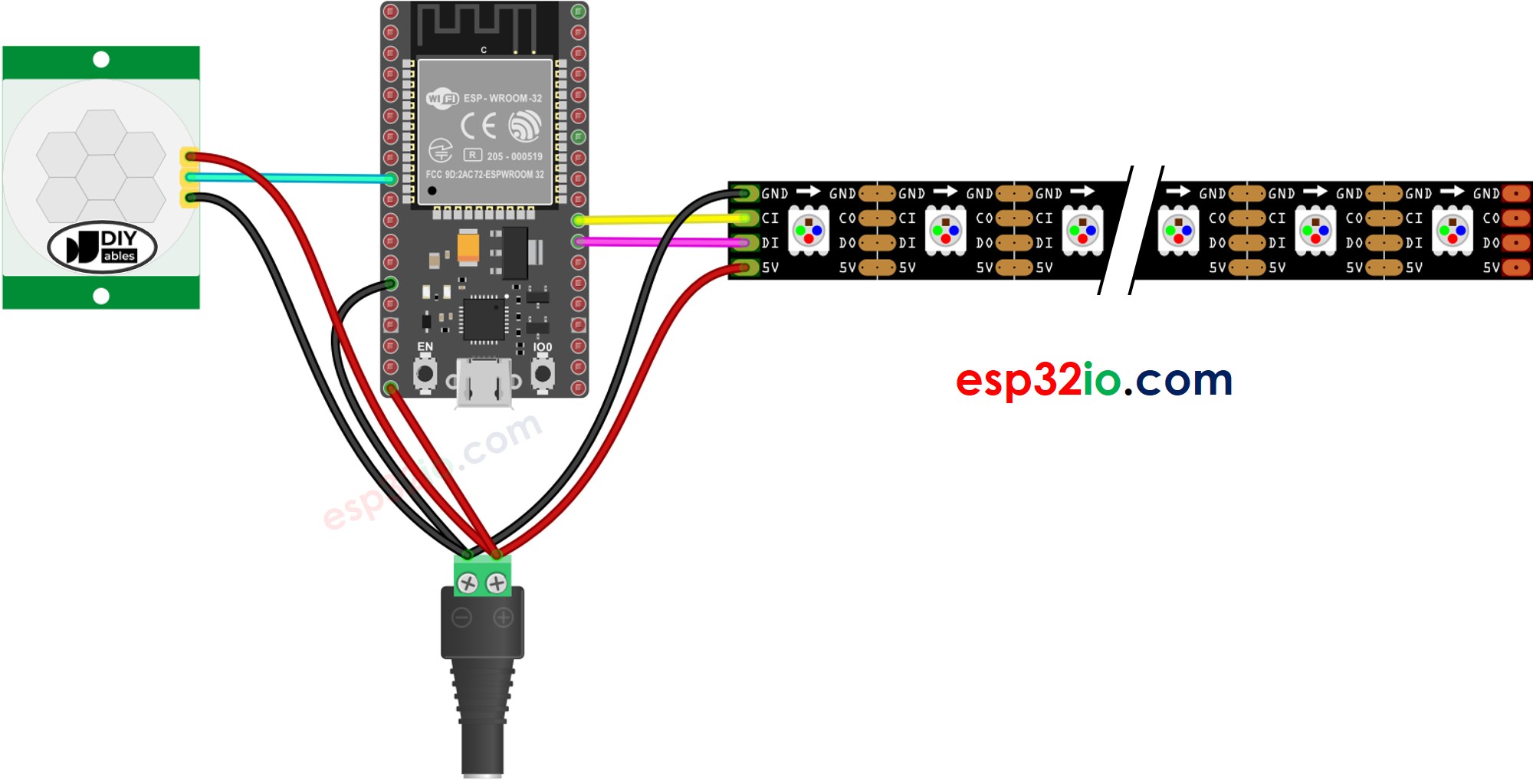
This image is created using Fritzing. Click to enlarge image
If you're unfamiliar with how to supply power to the ESP32 and other components, you can find guidance in the following tutorial: The best way to Power ESP32 and sensors/displays.
Initial Setting
Time Delay Adjuster | Screw it in anti-clockwise direction fully. |
Detection Range Adjuster | Screw it in clockwise direction fully. |
Repeat Trigger Selector | Put jumper as shown on the image. |
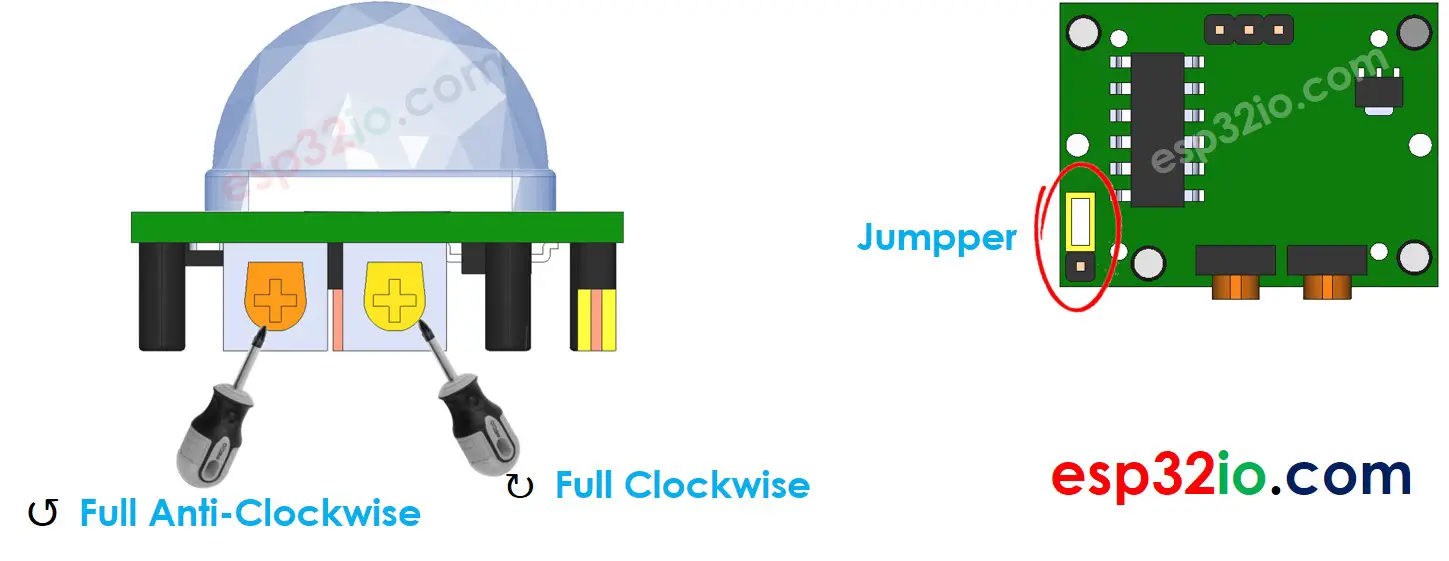
ESP32 Code - Motion Sensor Controls LED strip
Quick Instructions
- If this is the first time you use ESP32, see how to setup environment for ESP32 on Arduino IDE.
- Do the wiring as above image.
- Connect the ESP32 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP32 board (e.g. ESP32 Dev Module) and COM port.
- Connect ESP32 to PC via USB cable
- Open Arduino IDE, select the right board and port
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search “Adafruit DotStar”, then find the DotStar library by Adafruit
- Click Install button to install DotStar library.
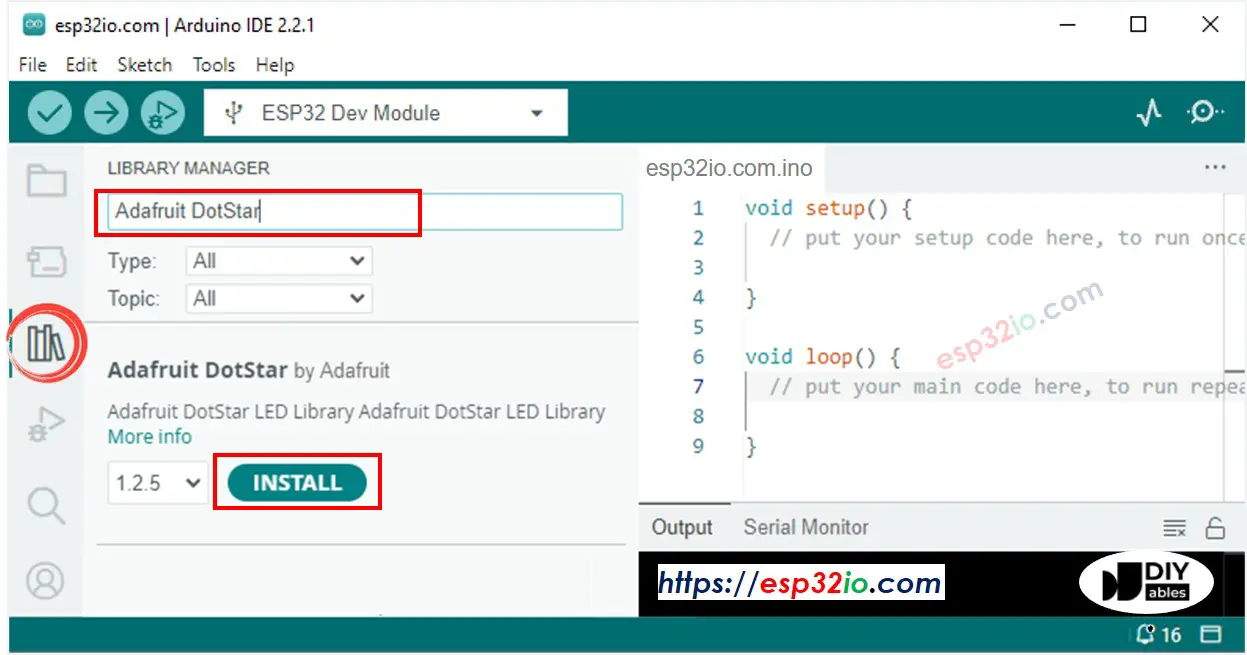
- You will be asked to install the dependency. Click Install All button.
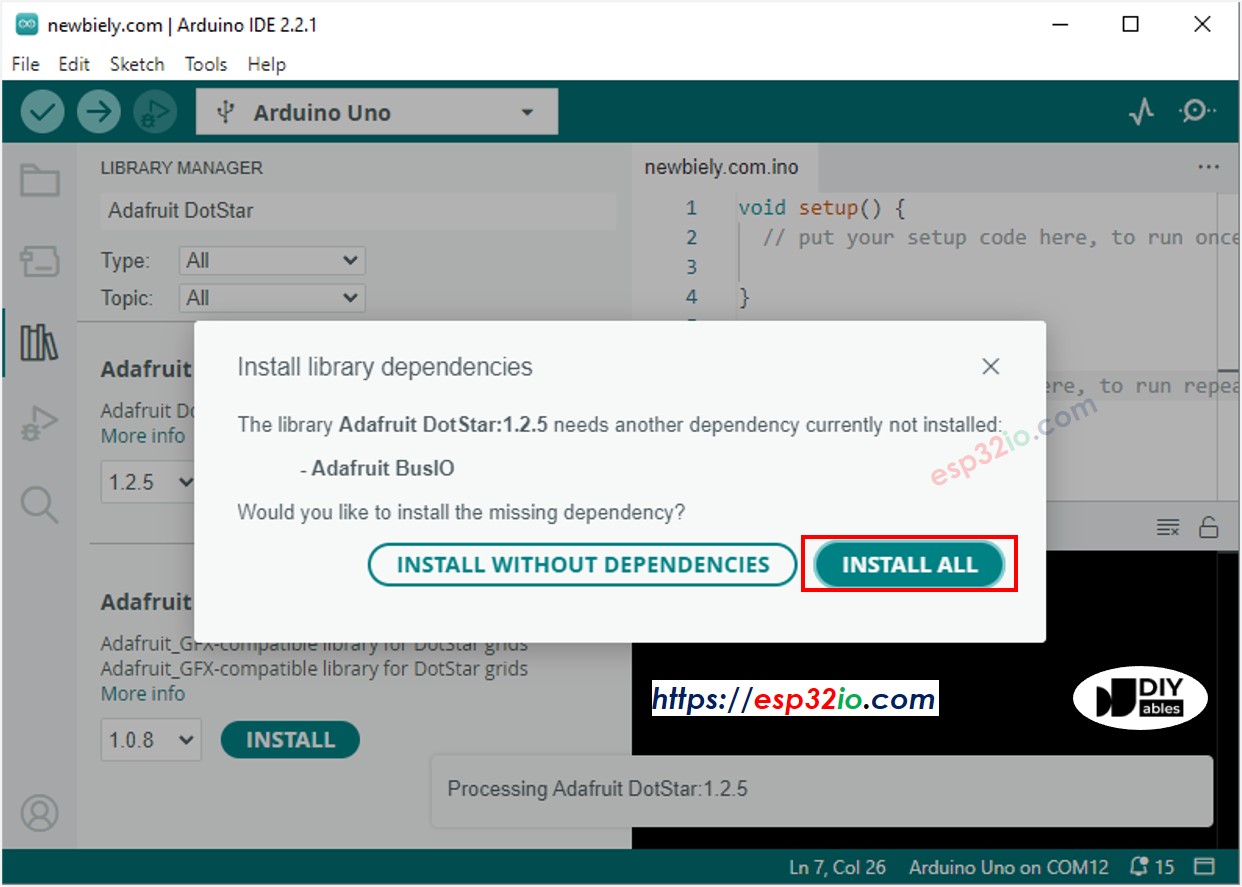
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP32
- Move your hand in front of sensor
- Check out the LED strip
You can modify the code to add a lighting effect.
Video Tutorial
Making video is a time-consuming work. If the video tutorial is necessary for your learning, please let us know by subscribing to our YouTube channel , If the demand for video is high, we will make the video tutorial.